Memory management of C# value types and reference types
In this blog, we will focus on how to improve performance during the software development process. This is a deep-seated problem in the software development or research and development process. This article mainly explains how calculations work in the process of writing our software code from two aspects: memory allocation and memory recycling. Here you can understand the process and methods of memory management so that you can pay attention to it and utilize it in future software development.
Value types include: int, float, double, bool, structure, reference, variables representing object instances
Reference types include: classes and arrays; more special reference types string, object
Generally: value type storage In the stack (excluding value types contained in references, such as value fields of classes, reference fields in classes, elements of arrays, which are stored in the regulated heap with references); reference types are stored in the regulated heap Why the heap is said to be a controlled heap will be discussed in detail below.
A few concepts:
Virtual memory: On a 32-bit computer, each process has 4G of virtual memory.
Regulated heap (managed heap): regulated by whom? The garbage collector, of course, is the garbage collector. How to manage it will be discussed below?
Useless unit collector: In addition to compressing the managed heap, updating the reference address, the garbage collector also maintains the information list of the managed heap, etc.
Regarding the storage of value types, first look at the following code:
{
int age=20;
double salary=2000;
}
The two variables defined above, int age, tell the compiler that they need to be given to me Allocate 4 bytes of memory space to store the age value, which is stored on the stack. The stack is a first-in, last-out data structure, and the stack stores data from high-order addresses to low-order addresses. The computer register maintains a stack pointer, which always points to the free space address at the bottom of the stack. When we define a value of type int, the stack pointer decrements by four bytes of the address; when the variable goes out of scope, the stack The pointer accordingly increments the address by four bytes. It just moves the stack pointer up and down, so the performance of the stack is quite high.
Let’s take a look at the storage of reference types, let’s look at the code first:
{
Customer customerA;
customerA=new Customer(); //Assume that the Customer instance occupies 32 bytes
}
The above code The first line first declares a Customer reference, the name of the reference is customerA, and allocates storage space for this reference on the stack. The size of the storage space is 4 bytes, because it only stores one reference, and the reference pointed to is It is the space address where the Customer instance will be stored. Note that customerA does not point to a specific space at this time, it just allocates a space.
During the execution of the second line, the .net environment will search the managed heap to find the first unused, continuous 32-byte space allocated to the instance of the class, and set customerA to point to the top position of this space (heap The space is used from low to high). When a reference variable goes out of scope, the reference in the stack becomes invalid while the instance remains in the managed heap until the garbage collector cleans it up.
Careful readers here may have some questions. When defining a reference type, does the computer have to search the entire heap to find a memory space large enough to store the object? Will this be very inefficient? What if there is not enough continuous space? This is about "custody". The heap is managed by the garbage collector. When the garbage collector is executed, .net releases all objects that can be released, compresses other objects, and then combines all free space and moves it to the top of the heap to form a continuous block and update it at the same time. References to other mobile objects. If there is another object definition, you can quickly find a suitable space. It seems that the managed heap works similarly to the stack. It allocates and reclaims space through heap pointers.
The above has talked about the management process and methods of .net's memory space allocation. Next, let's talk about the memory recycling process. When it comes to cleaning up resources, we have to mention two concepts and three methods.
Two concepts are: managed resources and unmanaged resources.
Managed resources are managed by the CLR (Common Language Runtime) of the .net framework; unmanaged resources are not managed by it.
The three methods are: Finalize(), Dispose(), Close().
1. Finalize() is a destructor method that clears unmanaged resources.
Defined in the class:
public ClassName{
~ClassName()
{
//Clean up unmanaged resources (such as closing files and database connections, etc.)
}
}
Its features Yes:
1. Operational uncertainty.
It is managed by the garbage collector. When the garbage collector works, this method will be called.
2. High performance overhead.
The way the garbage collector works is that if the object executes the Finalize() method, the first time the garbage collector executes it, it will be placed in a special queue; the object will not be deleted until the second execution.
3. The definition and call cannot be displayed, and the definition is in the form of a destructor method.
Based on the above characteristics, it is best not to execute the Finalize() method unless the class really needs it or it is used in combination with the other two methods.
2. The Dispose() method can clear all resources that need to be cleared, including managed and unmanaged resources.
is defined as follows:
public void Dispose()
{
//Clean up the resources that should be cleaned up (including managed and unmanaged resources)
System.GC.SuppressFinalize(this); //This sentence is very important, and the reasons will be explained below.
}
Its features:
1. Any client code should explicitly call this method to release resources.
2. Due to the first reason, a backup is generally required, and this backup is usually played by the destructor method.
3. The class that defines this method must inherit the IDisposable interface.
4. The syntax keyword using is equivalent to calling Dispose(). When using using, it calls the Dispose() method by default. Therefore, classes using using must also inherit the IDisposable interface.
The Dispose() method is more flexible and releases resources immediately when they are no longer needed. It is the final disposal of the resource, calling it means that the object will eventually be deleted.
3. Close() method, which temporarily disposes the status of resources and may be used in the future. Generally handles unmanaged resources.
is defined as follows:
public viod Close()
{
//Set the status of unmanaged resources, such as closing files or database connections
}
Its features:
Set the status of unmanaged resources Setting, usually closing the file or database connection.
Let’s write a comprehensive and classic example below, using code to demonstrate the function of each part: (To save trouble, this example is made up from the Internet, just explain the problem, what do you think. In We should thank the original author of the code for writing such a classic and easy-to-understand code. We have benefited a lot!
Dispose(true);
}
protected virtual void Dispose(bool disposing)
{
if (disposing)
{
// Here is the user code snippet to clean up "managed resources".
~ResourceHolder()
{
Dispose(false); // This is to clean up "unmanaged resources"
}
}
If you don't understand the above code, Be sure to read the following explanation carefully, it is a classic, you will regret it if you don’t read it.
Here, we must be clear that the user needs to call the method Dispose() instead of the method Dispose(bool). However, the method that actually performs the release work here is not Dispose(), but Dispose(bool)! Why? What? Look carefully at the code. In Dispose(), Dispose(true) is called, and when the parameter is "true", the function is to clean up all managed resources and unmanaged resources; you must still remember what I said earlier, " "The destructor method is used to release unmanaged resources." So since Dispose() can complete the work of releasing unmanaged resources, what is the purpose of the destructor method? In fact, the role of the destructor method is just a "backup" !
Why?
To put it formally, any class that implements the interface "IDisposable", as long as the programmer uses the object instance of this class in the code, sooner or later the Dispose() method of this class must be called. At the same time, if the class contains non- If managed resources are used, unmanaged resources must also be released! Unfortunately, if the code to release unmanaged resources is placed in the destructor method (the above example corresponds to "~ResourceHolder()"), then the programmer wants to call this section It is impossible to release the code (because the destructor method cannot be called by the user, but can only be called by the system, to be precise, the "garbage collector"), so everyone should know why the above example "cleans up the user code of unmanaged resources" "Section" is in Dispose(bool), not ~ResourceHolder()! Unfortunately, not all programmers are always careful to remember to call the Dispose() method. In case the programmer forgets to call this method, the managed Of course there is no problem with the resources. Sooner or later there will be a "garbage collector" to collect them (it will just be delayed for a while), but what about the unmanaged resources? It is not under the control of the CLR! Could it be that the unmanaged resources it occupies can never be released? Of course not! We still have a "destruction method"! If you forget to call Dispose(), then the "garbage collector" will also call the "destruction method" to release unmanaged resources! (One more nonsense, if If the programmer remembers to call Dispose(), then the code "System.GC.SuppressFinalize(this);" can prevent the "garbage collector" from calling the destructor method, so that there is no need to release "unmanaged resources" one more time) So we You are not afraid that programmers forget to call the Dispose() method.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


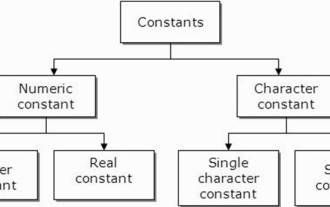
A constant is also called a variable and once defined, its value does not change during the execution of the program. Therefore, we can declare a variable as a constant referencing a fixed value. It is also called text. Constants must be defined using the Const keyword. Syntax The syntax of constants used in C programming language is as follows - consttypeVariableName; (or) consttype*VariableName; Different types of constants The different types of constants used in C programming language are as follows: Integer constants - For example: 1,0,34, 4567 Floating point constants - Example: 0.0, 156.89, 23.456 Octal and Hexadecimal constants - Example: Hex: 0x2a, 0xaa.. Octal
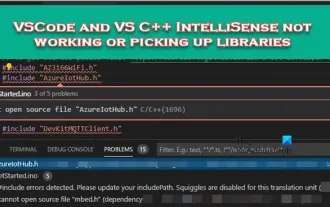
VS Code and Visual Studio C++ IntelliSense may not be able to pick up libraries, especially when working on large projects. When we hover over #Include<wx/wx.h>, we see the error message "CannotOpen source file 'string.h'" (depends on "wx/wx.h") and sometimes, autocomplete Function is unresponsive. In this article we will see what you can do if VSCode and VSC++ IntelliSense are not working or extracting libraries. Why doesn't my Intellisense work in C++? When working with large files, IntelliSense sometimes
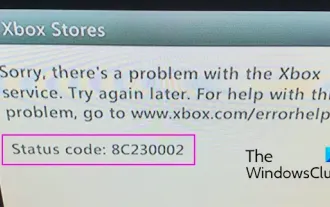
Are you unable to purchase or watch content on your Xbox due to error code 8C230002? Some users keep getting this error when trying to purchase or watch content on their console. Sorry, there's a problem with the Xbox service. Try again later. For help with this issue, visit www.xbox.com/errorhelp. Status Code: 8C230002 This error code is usually caused by temporary server or network problems. However, there may be other reasons, such as your account's privacy settings or parental controls, that may prevent you from purchasing or viewing specific content. Fix Xbox Error Code 8C230002 If you receive error code 8C when trying to watch or purchase content on your Xbox console
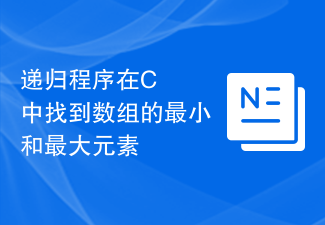
We take the integer array Arr[] as input. The goal is to find the largest and smallest elements in an array using a recursive method. Since we are using recursion, we will iterate through the entire array until we reach length = 1 and then return A[0], which forms the base case. Otherwise, the current element is compared to the current minimum or maximum value and its value is updated recursively for subsequent elements. Let’s look at various input and output scenarios for this −Input −Arr={12,67,99,76,32}; Output −Maximum value in the array: 99 Explanation &mi
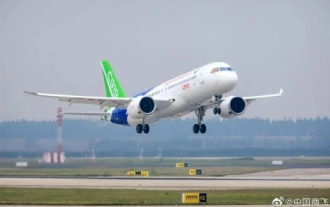
According to news on May 25, China Eastern Airlines disclosed the latest progress on the C919 passenger aircraft at the performance briefing meeting. According to the company, the C919 purchase agreement signed with COMAC has officially come into effect in March 2021, and the first C919 aircraft has been delivered by the end of 2022. It is expected that the aircraft will be officially put into actual operation soon. China Eastern Airlines will use Shanghai as its main base for commercial operations of the C919, and plans to introduce a total of five C919 passenger aircraft in 2022 and 2023. The company stated that future introduction plans will be determined based on actual operating conditions and route network planning. According to the editor's understanding, the C919 is China's new generation of single-aisle mainline passenger aircraft with completely independent intellectual property rights in the world, and it complies with internationally accepted airworthiness standards. Should
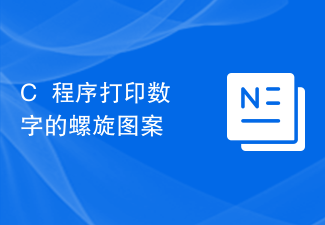
Displaying numbers in different formats is one of the basic coding problems of learning. Different coding concepts like conditional statements and loop statements. There are different programs in which we use special characters like asterisks to print triangles or squares. In this article, we will print numbers in spiral form, just like squares in C++. We take the number of rows n as input and start from the top left corner and move to the right, then down, then left, then up, then right again, and so on and so on. Spiral pattern with numbers 123456724252627282982340414243309223948494431102138474645321120373635343312191817161514
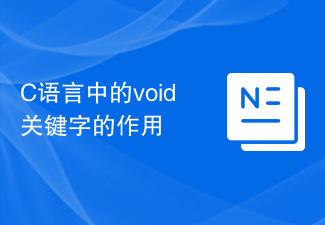
void in C is a special keyword used to represent an empty type, which means data without a specific type. In C language, void is usually used in the following three aspects. The function return type is void. In C language, functions can have different return types, such as int, float, char, etc. However, if the function does not return any value, the return type can be set to void. This means that after the function is executed, it does not return a specific value. For example: voidhelloWorld()
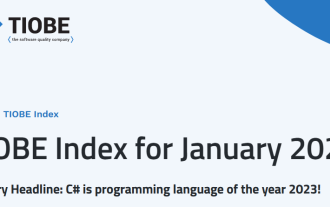
According to the TIOBE Programming Community Index, one of the benchmarks for measuring the popularity of programming languages, it is evaluated by collecting data from engineers, courses, vendors and search engines around the world. The TIOBE Index in January 2024 was released recently, and the official programming language rankings for 2023 were announced. C# won the TIOBE 2023 Programming Language of the Year. This is the first time that C# has won this honor in 23 years. TIOBE's official press release stated that C# has been in the top 10 for more than 20 years. Now it is catching up with the four major languages and has become the programming language with the largest growth in one year (+1.43%). It well-deserved to win this award. Ranked second are Scratch (+0.83%) and Fortran (+0
