.NET Decoration Mode Explanation
Definition of decoration mode:
Decoration mode is the ability to dynamically extend the functionality of an object without changing the original class file and using inheritance. It wraps the real object by creating a wrapping object, that is, decoration.
Decorator pattern structure diagram:
Decorator pattern role:
(1) Abstract component (Component) role: Gives an abstract interface to standardize objects that are prepared to receive additional responsibilities.
(2) Concrete Component role: Define a class that will receive additional responsibilities.
(3) Decorator role: Holds an instance of a Component object and implements an interface consistent with the abstract component interface.
(4) Concrete Decorator role: Responsible for adding additional responsibilities to component objects.
Implementation:
Quoting examples from life, we bought a new mobile phone and slowly added new decorations to the phone, such as stickers and widgets...
1. First abstract Mobile phone class, as an abstract component in the decorator pattern:
/// <summary> /// 手机抽象类,即抽象者模式中的抽象组件类 /// </summary> public abstract class Phone { /// <summary> /// 打印方法 /// </summary> public abstract void Print(); }
2. Suppose we buy an Apple phone now and inherit from the abstract class to implement the specific component class:
/// <summary> /// 苹果手机,即装饰着模式中的具体组件类 /// </summary> public class ApplePhone:Phone { /// <summary> /// 重写基类的方法 /// </summary> public override void Print() { Console.WriteLine("我有一部苹果手机"); } }
3 .Now I want to add new decorations to this phone, let’s abstract the decoration class first:
/// <summary> /// 装饰抽象类,让装饰完全取代抽象组件,所以必须继承Phone /// </summary> public abstract class Decorator:Phone { private Phone p ; //该装饰对象装饰到的Phone组件实体对象 public Decorator(Phone p) { this.p = p; } public override void Print() { if (this.p != null) { p.Print(); } } }
4. Specific decoration objects, inherit the decoration abstract class: here are the specific film decoration, and pendant decoration:
/// <summary> /// 贴膜,具体装饰者 /// </summary> public class Sticker:Decorator { public Sticker(Phone p) : base(p) { } public override void Print() { base.Print(); //添加行为 AddSticker(); } /// <summary> /// 新的行为方法 /// </summary> public void AddSticker() { Console.WriteLine("现在苹果手机有贴膜了"); } }
/// <summary> /// 手机挂件,即具体装饰者 /// </summary> public class Accessories:Decorator { public Accessories(Phone p) : base(p) { } public override void Print() { base.Print(); // 添加新的行为 AddAccessories(); } /// <summary> /// 新的行为方法 /// </summary> public void AddAccessories() { Console.WriteLine("现在苹果手机有漂亮的挂件了"); } }
5. Call:
/// <summary> /// 设计模式-装饰者模式 /// </summary> class Program { static void Main(string[] args) { Phone ap = new ApplePhone(); //新买了个苹果手机 Decorator aps = new Sticker(ap); //准备贴膜组件 aps.Print(); Decorator apa = new Accessories(ap); //过了几天新增了挂件组件 apa.Print(); Sticker s = new Sticker(ap); //准备贴膜组件 Accessories a = new Accessories(s);//同时准备挂件 a.Print(); } }
(1) Abstract component (Component) role: Give an abstract interface to standardize objects that are prepared to receive additional responsibilities. >Here is the Phone interface
(2) Concrete Component role: Define a class that will receive additional responsibilities. ApplePhone
(3) Decorator role: Holds an instance of a component (Component) object and implements an interface consistent with the abstract component interface. > Refers to Decorator
(4) Concrete Decorator role: Responsible for adding additional responsibilities to component objects. & Gt; Refers to the purpose of Accessories and Sticker
:
1. Decorator mode and inheritance the purpose is to expand the function of the object, but Decorator can provide more flexibility than inheritance.
2. By using different specific decoration classes and the arrangement and combination of these decoration classes, designers can create many combinations of different behaviors.
Disadvantages:
1. This feature, which is more flexible than inheritance, also means more complexity.
2. Decoration mode will lead to many small classes in the design. If overused, the program will become very complicated.
3. Decoration mode is for abstract component (Component) type programming. However, if you are programming for specific components, you should rethink your application architecture and whether decorators are appropriate. Of course, you can also change the Component interface, add new public behaviors, and implement the "translucent" decorator mode. Make the best choice in actual projects.
The above is the entire content of this article. I hope it will be helpful to everyone’s learning. I also hope that everyone will support the PHP Chinese website.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
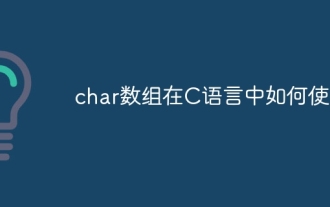
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
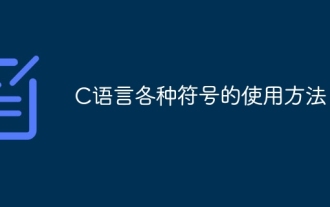
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
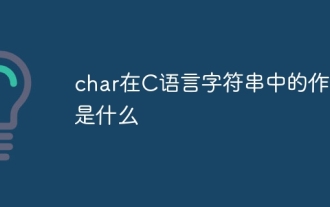
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
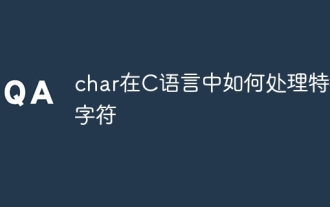
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
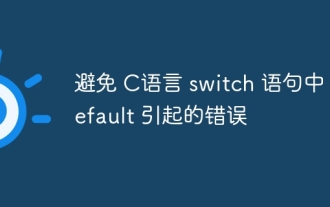
A strategy to avoid errors caused by default in C switch statements: use enums instead of constants, limiting the value of the case statement to a valid member of the enum. Use fallthrough in the last case statement to let the program continue to execute the following code. For switch statements without fallthrough, always add a default statement for error handling or provide default behavior.
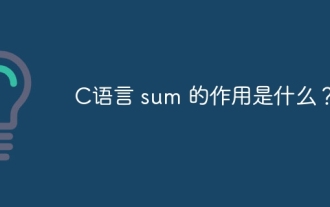
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
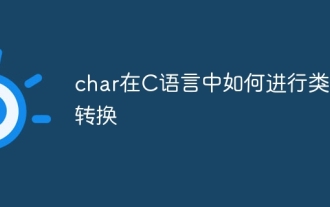
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
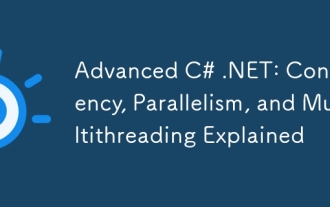
C#.NET provides powerful tools for concurrent, parallel and multithreaded programming. 1) Use the Thread class to create and manage threads, 2) The Task class provides more advanced abstraction, using thread pools to improve resource utilization, 3) implement parallel computing through Parallel.ForEach, 4) async/await and Task.WhenAll are used to obtain and process data in parallel, 5) avoid deadlocks, race conditions and thread leakage, 6) use thread pools and asynchronous programming to optimize performance.
