Getting Started with MyBatis (6)---Integration of mybatis and spring
1. Integration needs
1.1, method
The data in the previous chapter
requires sPRing to manage SqlsessionFactory through a singleton
spring and mybatis integration generate proxy objects, use SqlSessionFactory to create SqlSession
(spring and mybatis integration automatically Completed)
The mappers of the persistence layer need to be managed by spring
2. Create project integration environment
2.1, create project
2.2, data
db.properties
#database configuration information
#driver
driverClass=com.MySQL.jdbc.Driver
#Connection url
jdbcUrl=jdbc:mysql://localhost:3306/mybatis?character=utf8#Username
user=root
#Password
passWord=root
#Connection pool The minimum number of connections reserved in
minPoolSize=10#The maximum number of connections reserved in the connection pool. Default: 15 maxPoolSize=20#Maximum idle time, the connection will be discarded if it is not used within 1800 seconds. If it is 0, it will never be discarded. Default: 0 maxIdletime=1800#The number of connections c3p0 obtains at the same time when the connections in the connection pool are exhausted. Default: 3acquireIncrement=3#The number of initial connections in the connection pool should be between minPoolSize and maxPoolSize. The default is 3
initialPoolSize=15
2.3, confinguration
br/> PUBLIC "-//mybatis.org //DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
2.4 POJO classes and interfaces
package com.pb.ssm.po;import java.util.Date;/**
*
* @ClassName: Author
* @Description: TODO(author)
* @author Liu Nan
* @date 2015-10-31 12:39:33 pm
**/public class Author { //作者id
private Integer authorId; //作者姓名
private String authorUserName; //作者密码
private String authorPassword; //作者邮箱
private String authorEmail; //作者介绍
private String authroBio; //注册时间
private Date registerTime;
public Integer getAuthorId() { return authorId;
} public void setAuthorId(Integer authorId) { this.authorId = authorId;
} public String getAuthorUserName() { return authorUserName;
} public void setAuthorUserName(String authorUserName) { this.authorUserName = authorUserName;
} public String getAuthorPassword() { return authorPassword;
} public void setAuthorPassword(String authorPassword) { this.authorPassword = authorPassword;
} public String getAuthorEmail() { return authorEmail;
} public void setAuthorEmail(String authorEmail) { this.authorEmail = authorEmail;
} public String getAuthroBio() { return authroBio;
} public void setAuthroBio(String authroBio) { this.authroBio = authroBio;
} public Date getRegisterTime() { return registerTime;
} public void setRegisterTime(Date registerTime) { this.registerTime = registerTime;
}
@Override public String toString() { return "Author [authorId=" + authorId + ", authorUserName="
+ authorUserName + ", authorPassword=" + authorPassword + ", authorEmail=" + authorEmail + ", authroBio=" + authroBio + ", registerTime=" + registerTime + "]";
}
}
接口
package com.pb.ssm.mapper;import com.pb.ssm.po.Author;public interface AuthorMapper { /**
* *
* @Title: findAuthorById
* @Description: TODO (search based on id)
* @param @param id
* @param @return Setting file
* @return Author Return type
* @ throws
*/
public Author findAuthorById(int id);
/**
* *
* @Title: addAuthor
* @Description: TODO(Add)
* @param @param author
* @param @return Setting file
* @return int Return type
* @throws
* /
public int addAuthor(Author author); /**
* *
* @Title: updateAuthor
* @Description: TODO(update)
* @param @param author
* @param @return Setting file
* @return int Return type
* @throws
*/
public int updateAuthor(Author author);
/**
* * Delete
* @Title: delteAuthor
* @Description: TODO (delete by ID)
* @param @param id
* @param @return Setting file
* @return int Return type
* @throws
*/
public int delteAuthor(int id);
}
mapper.xml
br/> PUBLIC "-//mybatis.org//DTD Mapper 3.0/ /EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
VALUES(#{authorUserName},#{authorPassword},#{authorEmail},#{authroBio})
3. Use Mybatis configuration file.xml integration
3.1、写applicationContext.xml
3.2、测试
package com.pb.ssm.mapper;import java.io.InputStream;import org.apache.ibatis.io.Resources;import org.apache.ibatis.session.SqlSession;import org.apache.ibatis.session.SqlSessionFactory;import org.apache.ibatis.session.SqlSessionFactoryBuilder;import org.junit.Before;import org.junit.Test;import org.springframework.context.ApplicationContext;import org.springframework.context.support.ClassPathXmlApplicationContext;import com.pb.ssm.po.Author;public class AuthorMapperTest { private ApplicationContext applicationContext;
@Before public void setUp() throws Exception {
applicationContext=new ClassPathXmlApplicationContext("ApplicationContext.xml");
}
@Test public void testFindAuthorById() {
AuthorMapper authorMapper = (AuthorMapper) applicationContext.getBean("authorMapper");
Author author = authorMapper.findAuthorById(2);
System.out.println(author);
}
@Test public void testAddAuthor() { // 获取会话工厂
AuthorMapper authorMapper = (AuthorMapper) applicationContext.getBean("authorMapper");
Author author=new Author();
author.setAuthorUserName("程序猿");
author.setAuthorPassword("QWERdlfdad");
author.setAuthorEmail("QWER@QQ.com");
int num = authorMapper.addAuthor(author);
System.out.println("num="+num);
System.out.println("添加后的ID:"+author.getAuthorId());
}
@Test public void testUpdateAuthor() { // 获取会话工厂
AuthorMapper authorMapper = (AuthorMapper) applicationContext.getBean("authorMapper");
Author author = authorMapper.findAuthorById(13);
author.setAuthroBio("天天写代码");
author.setAuthorUserName("码农"); int num=authorMapper.updateAuthor(author);
System.out.println("num="+num);
System.out.println(author);
}
@Test public void testDeleteAuthor() { // 获取会话工厂
AuthorMapper authorMapper = (AuthorMapper) applicationContext.getBean("authorMapper"); int num= authorMapper.delteAuthor(13);
}
}
四、不使用mybatis配置文件
4.1、写ApplicationContext.xml
更改Mapper.xml,因为不能使用别名,所以type要写POJO类的全路径
br/> PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
VALUES(#{authorUserName},#{authorPassword},#{authorEmail},#{authroBio})
测试类同上
以上就是MyBatis入门(六)---mybatis与spring的整合的内容,更多相关内容请关注PHP中文网(www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
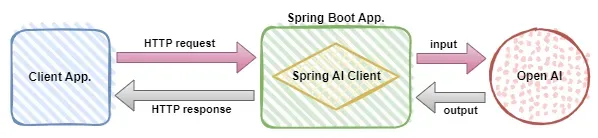
As an industry leader, Spring+AI provides leading solutions for various industries through its powerful, flexible API and advanced functions. In this topic, we will delve into the application examples of Spring+AI in various fields. Each case will show how Spring+AI meets specific needs, achieves goals, and extends these LESSONSLEARNED to a wider range of applications. I hope this topic can inspire you to understand and utilize the infinite possibilities of Spring+AI more deeply. The Spring framework has a history of more than 20 years in the field of software development, and it has been 10 years since the Spring Boot 1.0 version was released. Now, no one can dispute that Spring
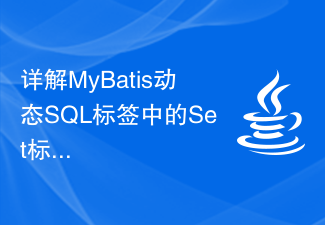
Interpretation of MyBatis dynamic SQL tags: Detailed explanation of Set tag usage MyBatis is an excellent persistence layer framework. It provides a wealth of dynamic SQL tags and can flexibly construct database operation statements. Among them, the Set tag is used to generate the SET clause in the UPDATE statement, which is very commonly used in update operations. This article will explain in detail the usage of the Set tag in MyBatis and demonstrate its functionality through specific code examples. What is Set tag Set tag is used in MyBati
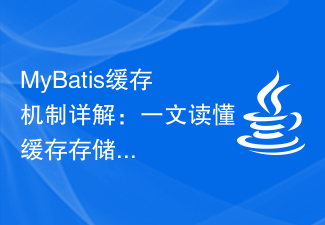
Detailed explanation of MyBatis caching mechanism: One article to understand the principle of cache storage Introduction When using MyBatis for database access, caching is a very important mechanism, which can effectively reduce access to the database and improve system performance. This article will introduce the caching mechanism of MyBatis in detail, including cache classification, storage principles and specific code examples. 1. Cache classification MyBatis cache is mainly divided into two types: first-level cache and second-level cache. The first-level cache is a SqlSession-level cache. When
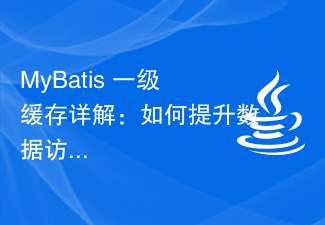
Detailed explanation of MyBatis first-level cache: How to improve data access efficiency? During the development process, efficient data access has always been one of the focuses of programmers. For persistence layer frameworks like MyBatis, caching is one of the key methods to improve data access efficiency. MyBatis provides two caching mechanisms: first-level cache and second-level cache. The first-level cache is enabled by default. This article will introduce the mechanism of MyBatis first-level cache in detail and provide specific code examples to help readers better understand

Analysis of MyBatis' caching mechanism: The difference and application of first-level cache and second-level cache In the MyBatis framework, caching is a very important feature that can effectively improve the performance of database operations. Among them, first-level cache and second-level cache are two commonly used caching mechanisms in MyBatis. This article will analyze the differences and applications of first-level cache and second-level cache in detail, and provide specific code examples to illustrate. 1. Level 1 Cache Level 1 cache is also called local cache. It is enabled by default and cannot be turned off. The first level cache is SqlSes
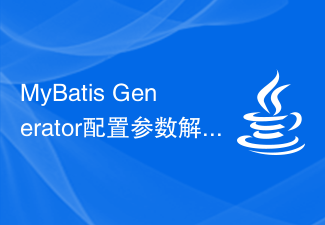
MyBatisGenerator is a code generation tool officially provided by MyBatis, which can help developers quickly generate JavaBeans, Mapper interfaces and XML mapping files that conform to the database table structure. In the process of using MyBatisGenerator for code generation, the setting of configuration parameters is crucial. This article will start from the perspective of configuration parameters and deeply explore the functions of MyBatisGenerator.

As network technology continues to develop, database attacks are becoming more and more common. SQL injection is one of the common attack methods. Attackers enter malicious SQL statements into the input box to perform illegal operations, causing data leakage, tampering or even deletion. In order to prevent SQL injection attacks, developers must pay special attention when writing code, and when using an ORM framework such as MyBatis, they need to follow some best practices to ensure the security of the system. 1. Parameterized query Parameterized query is the anti-
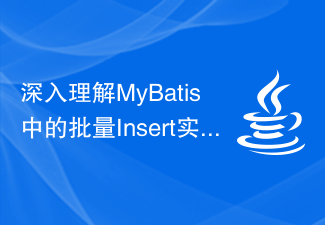
MyBatis is a popular Java persistence layer framework that is widely used in various Java projects. Among them, batch insertion is a common operation that can effectively improve the performance of database operations. This article will deeply explore the implementation principle of batch Insert in MyBatis, and analyze it in detail with specific code examples. Batch Insert in MyBatis In MyBatis, batch Insert operations are usually implemented using dynamic SQL. By constructing a line S containing multiple inserted values
