


[Java Getting Started Notes] Java Language Basics (1): Comments, Identifiers and Keywords
Annotations
What are annotations?
Comments are explanatory text for a certain piece of code, a certain method, or a certain class when we write code to facilitate everyone's reading of the code. The commented content will not be compiled or executed.
Java comments are divided into three types: single-line comments, multi-line comments, and document comments.
Single-line comments and multi-line comments
Single-line comments comment on a line of text or code in the program. In Java, use "//" for single-line comments. Multi-line comments can comment multiple lines of code at one time. In Java, "/*" is used to indicate the beginning of a multi-line comment, and "*/" is used to indicate the end of a multi-line comment.
Example:
public class CommentTest{ public static void main(String[] args){ //这是单行注释 /* 多行注释第一行 第二行 */ //System.out.PRintln("被注释的代码不会编译和运行"); System.out.println("未被注释的代码"); } }
Eclipse comment shortcut key: Ctrl+/ single-line comment and uncomment; Ctrl+Shift+/ to add /* */ comments; Ctrl+Shift+ to eliminate /* */ comments
Documentation comments
Comments added using documentation comments can be used to generate API documentation through the documentation generation tool javadoc, which is only processed by the javadoc tool Comments in front of classes, interfaces, methods, Fields, constructors and internal classes modified with public and protected in the document source text.
Documentation comments end with "/**"Start with "*/". The middle part is the documentation comment, which will be generated into the API documentation.
Example:
/** * 这是一个文档注释的测试类 * @author ping * */public class Test { /** 这是一个Filed */ public int i; /** * 这是程序的main方法 * @param args */ public static void main(String[] args) { } }
Regarding generating Java API documentation, you can refer to the following two articles:
Use the javadoc command to generate api help documentation
How to generate javadoc with eclipse
Identifiers and keywords
Separators
In Java Delimiters include semicolons (;), braces ({}), square brackets ([]), parentheses (()), spaces, and dots (.). All symbols are English symbols. This is when writing Pay special attention when coding.
Semicolon: In Java, a newline cannot represent the end of a statement. Only a semicolon represents the end of a statement. Therefore, it is theoretically possible to write multiple statements in one line, but this is not recommended as it will affect the readability of the code. Makes the code look cluttered.
Braces: A pair of curly braces represents a statement block and needs to appear in pairs.
Square brackets: used for arrays, used when defining arrays and accessing array elements. They also need to be used in pairs.
Parentheses: Used to include formal parameters when defining methods, calling methods and constructors, requiring parentheses.
Space: Used to separate multiple parts of a statement.
Dots: Used as members of objects and classes.
Identifiers
Identifiers are symbols used to name classes, methods, and variables in a program. Java identifiers have the following characteristics:
Case-sensitive.
Must start with a character, underscore, and dollar sign, and can be followed by four elements: characters, underscore, dollar sign, and numbers. Characters are not limited to English characters, but can be characters from various countries such as Chinese characters.
Cannot contain special symbols other than underscores and spaces.
cannot be a keyword in Java.
public class Test{ //Test is an identifier
public static void main(String[] args){ //main args is also an identifierint a = 1;
int A = 1; //a and A are Two different identifiers int $i = 2; //The identifier is correct int 123 = 123; //The identifier is wrong int i123 = 123; //The identifier is correct int i_1 = 1; //The identifier is correct int i.1 = 1; //Error int class = 2; //class is a keyword, error}
}
Keywords
Java contains a total of 48 keywords and two reserved words (goto/const).
The above is [Java Introduction Notes] Java Language Basics (1): Comments, identifiers and keywords. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


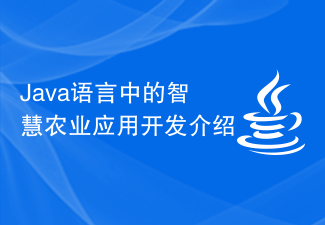
With the development of the times, the agricultural field has also begun to upgrade and transform with the help of modern scientific and technological means, and smart agriculture has emerged as the times require. As a computer programming language with excellent performance and strong portability, Java has high popularity and application value, and has become one of the important solutions for smart agricultural application development. This article aims to introduce the development process, application scenarios and advantages of smart agricultural applications in Java language. 1. Development process of smart agricultural applications in Java language. The development process of smart agricultural applications is divided into requirements analysis,
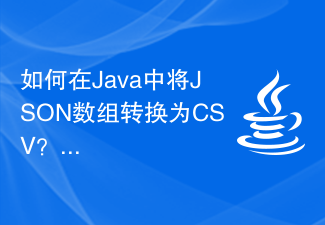
JSON can be used as a data exchange format, it is lightweight and language independent. A JSONArray can parse text strings to produce vector-like objects and supports the java.util.List interface. We can convert JSON array to CSV format using org.json.CDL class, which provides a static method toString() for converting JSONArray to comma-separated text. We need to import the org.apache.commons.io.FileUtils package to store data in a CSV file using the writeStringToFile() method. Syntaxpublicstaticj
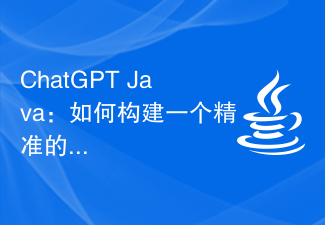
ChatGPTJava: How to build an accurate semantic search engine, requiring specific code examples. Introduction: With the rapid development of the Internet and the explosive growth of information, people often encounter poor quality and inconsistent search results in the process of obtaining the required information. Exact question. In order to provide more accurate and efficient search results, semantic search engines came into being. This article will introduce how to use ChatGPTJava to build an accurate semantic search engine and give specific code examples. 1. Understanding ChatGPTJ
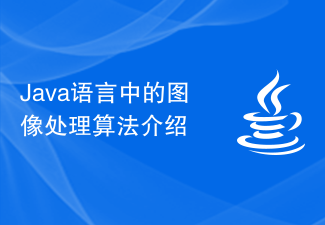
Introduction to image processing algorithms in Java language With the advent of the digital age, image processing has become an important branch of computer science. In computers, images are stored in digital form, and image processing changes the quality and appearance of the image by performing a series of algorithmic operations on these numbers. As a cross-platform programming language, Java language has rich image processing libraries and powerful algorithm support, making it the first choice of many developers. This article will introduce commonly used image processing algorithms in the Java language, and
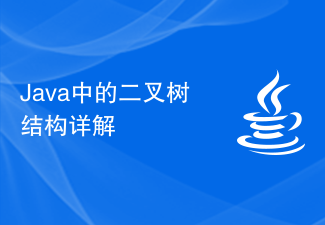
Binary trees are a common data structure in computer science and a commonly used data structure in Java programming. This article will introduce the binary tree structure in Java in detail. 1. What is a binary tree? In computer science, a binary tree is a tree structure in which each node has at most two child nodes. Among them, the left child node is smaller than the parent node, and the right child node is larger than the parent node. In Java programming, binary trees are commonly used to represent sorting, searching and improving the efficiency of data query. 2. Binary tree implementation in Java In Java, binary tree
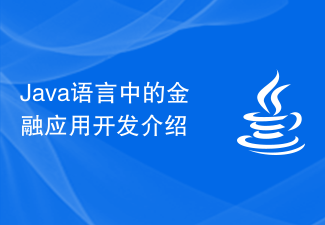
Java language is a programming language widely used in the financial field. Due to its powerful functions and efficient performance, Java language has become the preferred language for financial institutions to develop software. This article will introduce the importance of Java language in financial application development and introduce some common financial applications. 1. Application of Java language in the financial field Java language has been widely used in the financial field. Its main advantages include: 1. Cross-platform capability. The Java language has cross-platform capability, which means that the same Java language
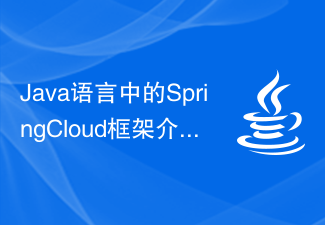
Introduction to the Spring Cloud framework in the Java language With the popularity of cloud computing and microservices, the Spring Cloud framework has become one of the preferred frameworks for building cloud native applications in the Java language. This article will introduce the concepts and features of the Spring Cloud framework, and how to use Spring Cloud to build a microservice architecture. Introduction to SpringCloud The SpringCloud framework is a microservice framework based on SpringBoot. it is

How to use Java language and Tencent Cloud API to implement SMS sending 1. Introduction With the rapid development of the mobile Internet, SMS notifications have become an important communication method between enterprises and users. Tencent Cloud provides a powerful SMS API service that can help developers quickly implement SMS sending functions. This article will introduce how to use Java language to connect with Tencent Cloud API to implement the SMS sending function. 2. Preparation: Register Tencent Cloud account, create SMS application, obtain API key (SecretId and SecretKey) 3
