


[Java Introduction Notes] Java Language Basics (2): Constants, Variables and Data Types
Constants and variables
What are constants and variables?
Constants and variables are a memory space used by the program to store data while the program is running.
Constant:
The value of the constant cannot be changed while the program is running. Constants are declared in Java The final keyword must be used. Constants can also be divided into two meanings:
The first meaning is a value. This value itself can be called a constant. Here are a few examples:
Integer constant: 123
Real constant: 3.14
Character constants: 'a'
Logical constants: true, false
String constants: "helloworld"
This is just a matter of saying. For example, for the number 7, we can say "a constant of type int 7"
The second meaning represents an immutable variable. This type is also called a constant. From a grammatical perspective, it means adding final and using the final keyword to modify a variable. Then as long as the value is assigned, it cannot be changed. It cannot be assigned again
//Declare a constant i of int type final int i = 1; //Declare a constant s of String object type, the "Hello" string is also a constant final String s = "Hello";
It should be noted that a constant must be assigned a value when it is declared.
Variable:
is a memory space used to store data when the program is running. It is the most basic storage unit in the program. We can access variables using variable names.
Classification of Java variables:
According to declaration position:
Local variables
Member variables
The parameters of the method are local variables.
public class Test{ int i1 = 1; //Member variables public class static main(String[] args){ //args are the parameters of the method, so they are also local variables int i2 = 2; //Local variables} }
By data type:
Basic data type variables
Reference data type variables
public class Test{ int i = 1; //Basic data type variables String s = new String(); //Reference data type}
Steps to use variables:
Step 1: Declare a variable - open up space in memory according to type Step 2: Assign value - store data in space Step 3: Use variables - get memory The data in
We must declare and assign values before using variables. Declaration and assignment can be separated or combined into one step;
If a member variable is not explicitly assigned a value when it is declared, it will be assigned a value. default value.
public class Test{ int i3; //If it is a member variable, the system will assign a value of 0 by default to it
public static void main(String[] args){ int i1; //Declare i1 = 1; //Assign value int i2 = 2; //Assign a value to it when declaring it. The value 2 is also called a "direct quantity" System.out.PRintln(i1); //Use to output the value of the variable to the Java console} }
Basic data types
Java is a strongly typed language. The so-called strong type means that each variable expression must have a certain data type at compile time. Java data types are divided into two major categories: basic types and reference types.
Reference data types are: class, interface, array. The basic data types in 基java are divided into four types of eight types, which are:
Basic data types
Numerous type
character type Char
occupation space: 2 Byte
Boolean type boolean
Occupied space: 1 bit
Integer type
Floating point type
Byte byte
short integer short
integer int
long integer long
single precision long
double double
1 byte
2 bytes
4 bytes
8 bytes
4 bytes
8 bytes
- 128~127
-32768~ 32767
-2147483648
~2147483647
-9223372036854774808
~9223372036854774807
-3.403E38
~3.403E38
-1.798E308
~1.798
Integer type
Java integer constants default to int type. To declare long type constants, you need to add L at the end.
Three expressions of Java language integer constants
Decimal integers: such as 12, -15, 0
Octal integers: requirements Starting with 0, such as 012
Hexadecimal number: It is required to start with 0x or 0X, such as 0x12
Note: Because hexadecimal value can be assigned in Java, it is similar to decimal 10 and hexadecimal A There is no difference in memory.
After Java 7, you can also use binary method to assign integer values in Java.
byte b1 = 127; //Correct byte b2 = 128; //Error, out of range short = b1; //Correct, assign the value of another variable to this variable, and it does not exceed the range int i1 = 12;/ /Correct int i2 = 012; //Correct int i3 = 0x12; //Correct System.out.println(i2); //Output 10, because i2 is assigned in octal System.out.println(i3) ; //Output 18, because i3 is assigned in hexadecimal long lo = 2147483648L; //Long type declaration needs to be added
Floating point type
There are two types of Java floating point type constants Expression form:
Decimal number form: for example 3.14 314.0 .314
Scientific notation form: such as 3.14e2 3.14E2 3.14E-2
Java floating point type constant defaults to double. If you want to declare a constant as float, you need to Add F at the end because of accuracy issues, so don’t use floating point types for equality comparisons
float f1 = 123; float f2 = 123.4F; double d1 = 1234.5; double d = 3.141_592_65_36 //Correct, in order to make comparisons after java7 Long data is more readable, you can use underscores between numbers
Character type
Character type is a single character enclosed in single quotes
Java characters are encoded in Unicode, and each character occupies two bytes, so it can be expressed in hexadecimal encoding, such as char c = 'u0061';
allows the use of the escape character '' to convert the following characters into other meanings, such as char c = 'n'; newline
Commonly used escape characters in Java
char c1 = 'you'; //Correct char c2 = 'Hello'; //Incorrect char c3 = 'u9999'; //Correct System.out.println (c3); //Output '香'
The Boolean type
is used to store the "true" and "false" types, with only two values: true and false.
boolean b = true;
Data type conversion
Precision sorting: (low to high)
Byte
from low to high The system will automatically convert when high-precision is converted; when high-precision is converted to low-precision, there will be a loss of precision and must be forced to convert; during operation, different types will be automatically converted to high-precision types.
byte b = 127; short s = b; //It will be automatically converted to shortb = (byte)s; //It will not be automatically converted, it needs to be forced to convert
double constant is converted to float constant and cannot be converted when overflow occurs
public class Test {
public static void main(String[] args) throws Exception { double d = 122222222222222222222222222222222222222222222222.3; float f = ( float) d; //The value of d has exceeded the range of float, so the conversion will "overflow" , but no error will be reported during compilation System.out.println(f); //Because overflow occurs, "Infinity" is output }
}
The system will automatically convert the value to int type during operation, and the operation Casting is required when assigning the result to the original type.
byte b1 = 1;byte b2 = (byte) (b1 + 2); //Numeric operations will be automatically converted to int, so after calculating the result, you need to force conversion when assigning the value to the byte type variable b2
If the constant value exceeds the default type, you must add the first letter of the numerical type that is larger than the default type at the end, such as long num=30000000000L; because the integer type defaults to int, but 30000000000 cannot be placed in int, so it must be added at the end Add lowercase l or uppercase L to convert to long type.
(data type name) Variable or value to be converted and Variable or value to be converted + conversion character These two conversion methods are essentially different. The former means that it is its original type in the computer, and then the It is forced to the current type, which means that it was already converted to this type in the computer from the beginning.
long lo = (long)2;long lo2 = 2L; //These two declarations or conversions are essentially different
The above is [Java entry notes] Java language basics (2): constants, variables and data types Content, please pay attention to the PHP Chinese website (www.php.cn) for more related content!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


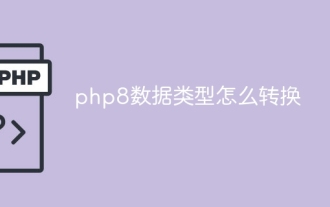
The methods of the php8 data type include converting strings to integers, converting integers to strings, converting strings to floating point numbers, converting floating point numbers to strings, converting arrays to strings, converting strings to arrays, and converting Boolean values to integers. Integer conversion to Boolean value and variable type determination and conversion. Detailed introduction: 1. Converting a string to an integer includes the intval() function and (int) forced type conversion; 2. Converting an integer to a string includes the strval() function and (string) forced type conversion; 3. Converting a string to a float Points and so on.
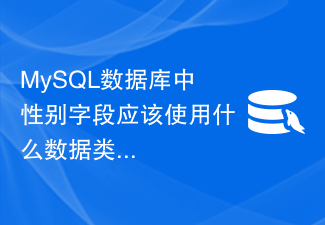
In a MySQL database, gender fields can usually be stored using the ENUM type. ENUM is an enumeration type that allows us to select one as the value of a field from a set of predefined values. ENUM is a good choice when representing a fixed and limited option like gender. Let's look at a specific code example: Suppose we have a table called "users" that contains user information, including gender. Now we want to create a field for gender, we can design the table structure like this: CRE
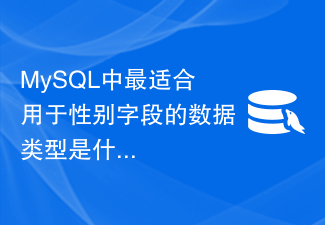
In MySQL, the most suitable data type for gender fields is the ENUM enumeration type. The ENUM enumeration type is a data type that allows the definition of a set of possible values. The gender field is suitable for using the ENUM type because gender usually only has two values, namely male and female. Next, I will use specific code examples to show how to create a gender field in MySQL and use the ENUM enumeration type to store gender information. The following are the steps: First, create a table named users in MySQL, including
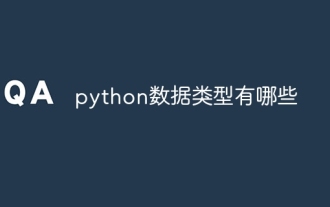
Python data types are: 1. Integer type; 2. Floating point type; 3. Complex number; 4. Boolean type; 5. String; 6. List; 7. Tuple; 8. Set; 9. Dictionary. Detailed introduction: 1. Integer type, used to represent integers, which can be positive, negative, or zero. In Python, the range of values that integers can represent is platform-specific; 2. Floating point type, used to represent numbers with decimal parts. Numbers, floating point type can represent positive numbers, negative numbers and zero; 3. Complex numbers, used to represent complex numbers, including real and imaginary parts; 4. Boolean type, used to represent Boolean values, etc.
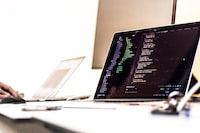
Python is widely used in a wide range of fields with its simple and easy-to-read syntax. It is crucial to master the basic structure of Python syntax, both to improve programming efficiency and to gain a deep understanding of how the code works. To this end, this article provides a comprehensive mind map detailing various aspects of Python syntax. Variables and Data Types Variables are containers used to store data in Python. The mind map shows common Python data types, including integers, floating point numbers, strings, Boolean values, and lists. Each data type has its own characteristics and operation methods. Operators Operators are used to perform various operations on data types. The mind map covers the different operator types in Python, such as arithmetic operators, ratio
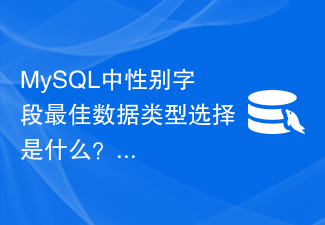
When designing database tables, choosing the appropriate data type is very important for performance optimization and data storage efficiency. In the MySQL database, there is really no so-called best choice for the data type to store the gender field, because the gender field generally only has two values: male or female. But for efficiency and space saving, we can choose a suitable data type to store the gender field. In MySQL, the most commonly used data type to store gender fields is the enumeration type. An enumeration type is a data type that can limit the value of a field to a limited set.
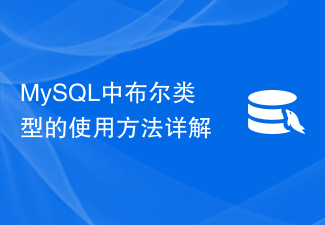
Detailed explanation of how to use Boolean types in MySQL MySQL is a commonly used relational database management system. In practical applications, it is often necessary to use Boolean types to represent logical true and false values. There are two representation methods of Boolean type in MySQL: TINYINT(1) and BOOL. This article will introduce in detail the use of Boolean types in MySQL, including the definition, assignment, query and modification of Boolean types, and explain it with specific code examples. 1. The Boolean type is defined in MySQL and can be
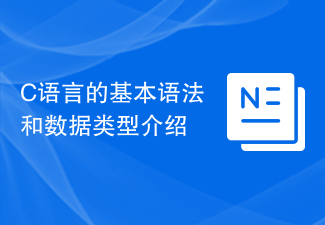
C language is a widely used computer programming language that is efficient, flexible and powerful. To be proficient in programming in C language, you first need to understand its basic syntax and data types. This article will introduce the basic syntax and data types of C language and give examples. 1. Basic syntax 1.1 Comments In C language, comments can be used to explain the code to facilitate understanding and maintenance. Comments can be divided into single-line comments and multi-line comments. //This is a single-line comment/*This is a multi-line comment*/1.2 Keyword C language
