PHP Learning Guide-Chapter 5
Chapter 5
Syntax, variables and output
Key points of this chapter
◆ Understand the basic rules of PHP program code
◆ Store information in variables
◆ Display output in a Web page
In this chapter we will explain The basic syntax of PHP is the rules that all PHP program codes with regular format usage must follow. We'll also look at how to use variables to store and retrieve information in PHP code execution, and finally talk about the simplest way to display text in the user's browser window.
The looseness and flexibility of PHP syntax
About PHP, the first thing to mention is that it has strived to be as loose and flexible as possible. Programming languages vary considerably depending on the strictness of the grammatical rules to be followed. It's best to be selective, as this will help ensure that you write exactly what you need. If you were writing a program to control a nuclear reactor and you forgot to specify a variable, it would be much better to have the program refuse to execute than slowly deviate from the original design intent. However, the basic design principles of PHP are based on another specification template, because PHP itself is a tool for making fast and simple Web pages. Its focus is on making it convenient for designers to use it correctly, rather than making it easy to use. The program does extra redundant work to specify the meaning of the program code. PHP requires very little program code, but it can express the meaning of the program code to the greatest extent possible. This means that certain syntax features found in other languages, such as variable declarations and function prototypes, are not needed in PHP.
Having said that, PHP cannot read what you are thinking, it still has to be expressed by you and there are some grammatical rules to follow. If you see a "parse error" in the browser window instead of the Web page you originally intended to produce, it means that the program code you have written has violated the rules to a level that PHP cannot tolerate.
HTML is not PHP
Another important thing to remember is that this syntax should only be used within PHP. Because PHP is embedded in HTML, in such a file, each part will be interpreted by PHP or HTML, depending on the PHP tags in it.
PHP syntax is only valid within PHP, so we assume that the content in this chapter refers to PHP mode. That is to say, most program code snippets are assumed to be embedded in HTML web pages, and are distinguished by appropriate markup mark.
PHP has the syntax style of C language
The third important point to understand is that PHP is a programming language that is very similar to C language style. If the reader already knows C, you will learn PHP quickly: if that doesn't work, check the manual again. The rest of this chapter is for other people who don’t know C yet. If you are a C programmer, you can quickly read the title of this chapter. You can also refer to this book specifically for C. Appendices prepared by language programmers, saving you valuable time.
PHP is not sensitive to half-whitespace
Half-whitespace is the empty part on the screen typed by the programmer, including spaces (spacehar), Tab characters and Enter keys (terminal symbols at the end of lines), etc. PHP is not sensitive to such whitespace, but this does not mean that spaces and such content are not important (in fact, they are still very important for separating "words or words" in the PHP language), but in a line there are It doesn't matter how many spaces there are, one space character is the same as many such characters.
For example, every PHP syntax below that assigns the sum of 2+2 to the variable $four is equal
$four=2+2; //Single space
$four =2+2; // Space and tab keys
$four =
2
+
2; //Multi-line style
It is very convenient to use the line end symbol of the Enter key as a blank, because it means that there is no need to ensure The syntax must be on a single line, which makes it much easier to write programs.
PHP is sometimes case-sensitive
As mentioned before, PHP is not overly picky. Readers may be surprised that it is sometimes case-sensitive (that is, it distinguishes the difference between upper and lower case of English letters). This should be noted especially in all variable settings. If you embed program code like this in an HTML page:
<?php $capital=67; Print(“Variable capital is $capital<BR>”); Print(“Variable CaPiTaL is $ CaPiTaL<BR>”); ?>
The output result is
Variable capital is 67
Variable CaPiTaL is
This is because the variables use different cases, so they are divided into two different variables. (Surprisingly, under the default debugging settings, program code snippets like this do not generate PHP errors. See the "Unspecified variables" section later in this chapter.)
On the other hand, The difference between PHP and C language is that its function names are not case-sensitive, as are the basic syntax structures (if, then, else, while, etc.).
The description statement ends with a semicolon
The following is a typical statement in PHP, which assigns the string to the $greeting variable:
$greeting="Welcome to PHP!";
This section The rest of the chapter will introduce how to construct such a statement from smaller components, and how the PHP interpreter performs the evaluation operation of the representation (you can skip this content if you are already familiar with these statements and expressions).
PHP’s minimal structure is an indivisible token, such as a number (3.14159), a string (?tow?), a variable ($tow), a constant (TRUE), and special words (if, else) that constitute the syntax of PHP itself etc.), they are separated from each other by whitespace and other special characters (such as brackets and braces).
The most complex construct in PHP is an expression, which is any combination of tags with [value]. A single number is an expression, and a single variable is an expression. Simple expressions can be combined to form more complex expressions, usually by adding operators between expressions (for example, 2 + (2 + 2)), or by calling the expression as a function Input (for example, pow(2*3,3*2)). Expression numbers that take two inputs are treated as inputs, so a function with inputs puts the inputs in parentheses after the function name, and the inputs (called parameters) are separated by commas.
Expression evaluation operations
Whenever the PHP interpreter encounters an expression in the program code, the expression is evaluated immediately. This means that PHP starts with the value of the smallest element of the expression, and then continues to combine these values connected by operators or functions until the entire value of the expression is generated. For example, each step in the judgment process should be as follows in imagination:
$result=2*2+3*3+5;
(=4+3*3+5)//Imagination The estimation process
(=4+9+5)
(=13+5)
(=18)
The result will be the number 18 stored in the $result variable.
Priority, associativity and order of evaluation
There may be two types of problems in PHP expression evaluation: If subexpressions are combined or combined, what is the order of evaluation? ? For example, in the evaluation evaluation process just shown, multiplication is more tightly associative than addition, which has an impact on the final result.
The specific way of operating combined expressions is called the precedence rule. High-priority operators will first obtain other surrounding expressions for operation. If necessary, remember these rules, for example [*] always has higher priority than [+] (more on these rules in subsequent chapters). Or use this cardinal rule: When in doubt, use parentheses to join expressions together.
For example:
$resultl=2+3*4+5//The result is 19
$result2=(2+3)*(4+5)//The result is 45
The operator priority rule will Take away the ambiguity about how expressions combine, but what about when operators have the same precedence? Consider the following expression for example:
$how_much=3.0/4.0/5.0;
This expression is equal to 0.15 or 3.75 depending on which division operator processes the number 4.0 first. There is an extensive list of associativity rules in the online instructions, but the most important rule to remember is that associativity is usually in order from left to right. In other words, the above expression evaluates to 0.15 because The left-hand division operator of the two division operators has priority.
The last question is the order of evaluation, which is not the same as associativity. For example, the following arithmetic expression:
3*4+5*6
We know that multiplication should occur before addition, but this does not mean knowing which multiplication operation PHP will perform first. Generally speaking, users do not need to care about the order of evaluation, because in most cases it has no impact on the results. We can build some weird examples where the result depends on the order of evaluation, usually because of the specification of subexpressions in other parts of the expression. For example,
$hun=($this=$that+5)+($that+$this+3); //Bad example
But please don’t write like this, okay. PHP may have... dependencies on it, and we won't tell you the results (the only reasonable use of relying on left-to-right evaluation order is in the "short path" mode of Boolean expressions, which we'll cover in Chapter 7 explain).
Expressions and types
通常程序设计师会很小心地配合表达式的类型和将它们组合在一起的运算子或函式。常见的表达式是数学的表达式(用数学运算子结合数字)、Boolean表达式(用and或or结合true或false的语句)或字符串连算式(用运算子和函式来建构字符串)。与PHP的其余部分一样,对类型的处理会很宽松。例如,下面有表达式范例,很明显地它将两个运算试很不恰当地混合在一起使用了:
2 + 2 * ?nonsense? + TRUE
这个表达式不会产生错误,而是求值为数字「3」(你可以暂时把这个范例当作猜谜,下一章将解释为什么会出现这样的结果)。
指定表达式
最常见的表达式类型是指(assignment),在表达式中,把一个变数设置为等于某表达式的评算求值结果。表达式的形式是,先有一个变量名(是以「$」开头),后面是一个等号,然后是要评算求值的表达式。例如,
$eight=2 * (2 * 2)
$eight」将按照所我们所希望的方式被指定。
有一件要记住的重要事项是,指定表达式也是一种表达式,因此它们本身是有「值」的!运算工指定的对象无论是变量或值都是相同的。这意谓着可以在更复杂的表达式中间使用表达式。如果对下面的表达式求值:
$ten=($two = 2)+($eight = (2 * 2))
每个变量都将被指定为等于其名称的数值。
总而言之,PHP中的叙述语句(statement)可以是最后带带着分号(;)的任何表达式。如果把表达式看成是词组,则叙述语句则算是「整个句子」了,分号就算是句子结尾处的句号。任何括在PHP标记内有效PHP叙述语句都算是PHP的有效程序代码。
使用表达式和语句的理由
通常只有两个理由需要在PHP中编写表达式:为了取得它的值(value),或者为了取得次作用(side effect)。表达式的值传递给包含它的更复杂表达式;而次作用是除求值结果以外发生的任何事情。最典型的次作用包括指定或更改变量,在使用者屏幕是显示某些内容,或者对程序环境的一些其它持久性改变(诸如与数据库的互动)。
仅管叙述语句是表达式,但它们本身不能包含在更复杂的表达式中,这意味着使用叙述语句的唯一理由就是它的「次作用」!这也意味着可以编者编写一些合法但完全无用的语句,例如下面的第二条语句:
Print(“Hello”);//副加效应为输出显示至屏幕
2 * 3 + 4;//无用,没有效应产生
$value_num= 3 * 4 + 5;//副加效应为指定
Store_in_database(49.5);//副加效应到数据库
大括号的使用
虽然叙述句不能像表达式那样合并,但可以在能够使用语句的位置放入一串语句,把它们括在大括号中即可。
例如,PHP中的if结构有个测试条件(在圆括号中),条件后面是如果判断结果为真则应该执行的语句。如果在判断为真时要执行多条语句,可以使用大括号括住的这一堆叙述语句。下面的二个if程序代码段是相等的(都是判断一个恒真的叙述并印出相同讯息):
if(3==2+1) print(“Good – I haven′t totally lost my mind.<BR>”); if(3 ==2+1) { Print(“Good – I haven′t totally ”); Print(“lost my mind.<BR>”); }
在大括号包围住的中央可放置任何语句,其中包括本身带有大括号区块的if叙述。这表示if语句可包含另一个if叙述,这种巢状嵌套可依需要套入任意层。
注释
注释是程序的一部分,就是我们人类用来解释说明程序而使用,目的是帮助说明解释程序代码。程序执行器对程序代码做的第一件事情就是剔除注释,因此注释对程序的功能没有任何作用。但它对于帮助其它人在阅读程序代码时,更能理解原设计者在编写程序时的想法,因此注释也可算是无价的,即使就是程序设计师自己编写了一段时间之后,再回头看自己的程序也是如此。
PHP算是从几种不同程序设计语言(包括C、Perl和UNIX的shell script)获取灵感。因而PHP支持所有这些语言的注释风格,并且这些风格可在PHP程序代码中自由混合使用。
属于C语言风格的多行注释
多行的注释风格和C中的相同:注释从字符对「/*」开始,以字符对「*/」当作结束。例如:
* This is A comment in PHP */
关于多行注释,要记住的最重要一点是,注释不能巢状嵌套方式编写。不能在一个注释内放另一个注释。如果试图这样做,注释将在第一个「*/」字符对后结束,原本要作为注释的其余部分将被解释为程序代码,可能会导致错误的结果。例如:
/* This comment will /* fail horribly on the Last word of this */sentence */
这是一种很容易造成的无心错误,通常发生在试图「注释」一段已经有注释语言的程序时,是常遇到的,请读者小心。
单行注释:「#」和「//」
除了/*…*/这种多行注释之外,PHP还支持两种在给单行使用的不同注释方式,一种是从C和Java继承来的,另一种是则后Perl和shell script继承来的。Shell script风格的注释以「#」符号开始,而C++风格的注释以双斜线「//」开始。这两种方法都会将加注的该行叙述的剩余部分当成注释对待,如下所示的范例:
# This is a comment ,and # this is the second line of the comment //This is a comment too.Each style comments only //one line so the last word of this sentence will fail horribly.
非常机灵的读者认为单行注释与我们前面所介绍到的空白不敏感性并不相容。这是对的,如果采用单行注释并用Enter行结束符号替换了其中一个空格,结果全是不同的。以更精确的讲法是,PHP在从程序代码中剔除了注释后,程序代码才是对空白不敏感的。
变数
在PHP程序中间储存信息的主要方式是透过使用「变量」,这是取一个名称然后保存发后要使用的任意值的方式。
关于PHP中的变量,以下有几点正是要注意知道(后面会有更详细的讲解):
◆ PHP中的所有变量都要在最前面加上「$」符号标示。
◆ 变数中的值是它最近被指定的值。
◆ 变量用「=」运算子进行指定,变量在左边,要评算求值的表达式在右边。
◆ 变量不需用要在指定之前进行宣告。
◆ 除了目前值的型别之外,变量没有固有的型别。
◆ 指定前就被使用的变量有其默认值。
以上就是PHP学习宝典-第五章的内容,更多相关内容请关注PHP中文网(www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
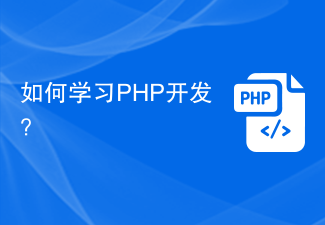
With the development of the Internet, the demand for dynamic web pages is increasing. As a mainstream programming language, PHP is widely used in web development. So, for beginners, how to learn PHP development? 1. Understand the basic knowledge of PHP. PHP is a scripting language that can be directly embedded in HTML code and parsed and run through a web server. Therefore, before learning PHP, you can first understand the basics of front-end technologies such as HTML, CSS, and JavaScript to better understand the operation of PHP.
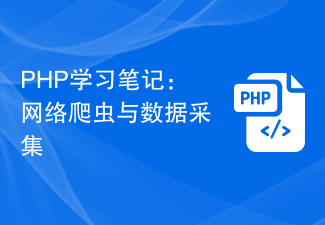
PHP study notes: Web crawler and data collection Introduction: A web crawler is a tool that automatically crawls data from the Internet. It can simulate human behavior, browse web pages and collect the required data. As a popular server-side scripting language, PHP also plays an important role in the field of web crawlers and data collection. This article will explain how to write a web crawler using PHP and provide practical code examples. 1. Basic principles of web crawlers The basic principles of web crawlers are to send HTTP requests, receive and parse the H response of the server.
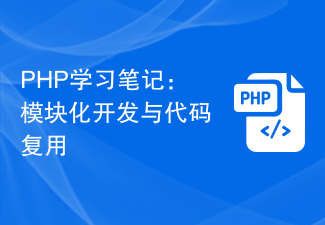
PHP study notes: Modular development and code reuse Introduction: In software development, modular development and code reuse are very important concepts. Modular development can decompose complex systems into manageable small modules, improving development efficiency and code maintainability; while code reuse can reduce redundant code and improve code reusability. In PHP development, we can achieve modular development and code reuse through some technical means. This article will introduce some commonly used technologies and specific code examples to help readers better understand and apply these concepts.
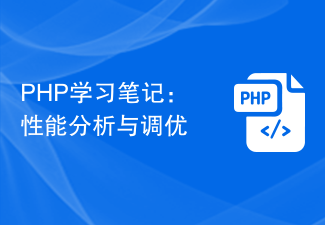
PHP study notes: Performance analysis and tuning Introduction: In web development, performance is a very critical factor. A high-performance website can provide a better user experience, improve user retention, and increase business revenue. In PHP development, performance optimization is a common and important issue. This article will introduce performance analysis and tuning methods in PHP, and provide specific code examples to help readers better understand and apply these techniques. 1. Performance analysis tool Xdebug extension Xdebug is a powerful P
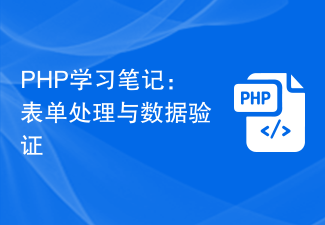
PHP study notes: Form processing and data validation In web development, forms are one of the important components for users to interact with the website. When users fill out forms and submit data on the website, the website needs to process and verify the submitted data to ensure the accuracy and security of the data. This article will introduce how to use PHP to process forms and perform data validation, and provide specific code examples. Form submission and data preprocessing In HTML, we need to use the <form> tag to create a form and specify the form
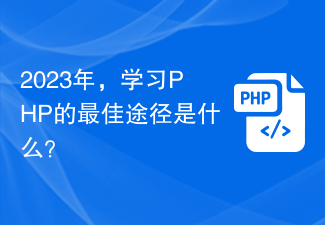
What is the best way to learn PHP in 2023? With the rapid development of the Internet, computer programming has become a skill with extremely high employment prospects. Among many programming languages, PHP is a language that is widely used in network development. If you want to learn PHP, it's important to know the best way to learn it. PHP is an open source, server-side scripting language used to develop dynamic websites and applications. Compared to other languages, PHP has a lower learning curve and a wide range of applications, making it an ideal choice for beginners.
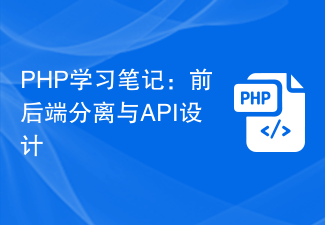
PHP study notes: Overview of front-end and back-end separation and API design: With the continuous development of the Internet and the increasing user needs, the development model of front-end and back-end separation has attracted more and more attention from developers. Front-end and back-end separation refers to separating the development of the front-end and the back-end, and conducting data interaction through APIs to achieve development efficiency and flexibility. This article will introduce the concept of front-end and back-end separation, and how to design an API. The concept of front-end and back-end separation: The traditional Web development model is front-end and back-end coupling, that is, the development of front-end and back-end is carried out in the same project.
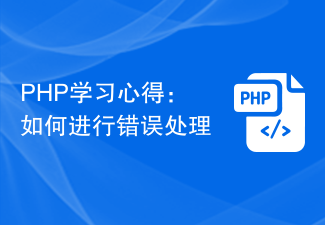
PHP learning experience: How to handle errors When developing PHP applications, handling errors is a very important aspect. Good error handling can improve the stability and reliability of the code, and can also better help us debug the code and solve problems. This article will introduce some common error types and how to handle them, with corresponding code examples. Syntax Errors Syntax errors are the most common and easiest to find errors in the coding process. It usually causes the PHP parser to not understand the code correctly, resulting in
