


PHP tutorial: Combining cookies and arrays to implement a shopping cart
I found that the results obtained by many PHP functions are given by arrays. The most commonly used one is mysql_fetch_array(),
including the obtained string information to generate an array, and then the array is decomposed into a string using the functions explode(); implode() ;
I have never written an implementation like a shopping cart before. I feel that I am a stupid type. I wrote it intermittently for a week and figured out (I am allowed to be arrogant as a novice) the shopping cart processing program.
And because I have never entered the class, I still focus on the process.
The essence of the method I use is: store the cookie in the array, add, delete, and modify the array. Each set of records in the array is information about a product (number, price, etc.)
Solution The idea of the shopping cart is to use cookies to record a two-dimensional array. One dimension represents each product, and two dimensions include the product ID, the quantity of the product, etc. You can add it by yourself. Anyway, it is two-dimensional. You can add as many product attributes as you want.
The operations on items in the shopping cart generally include the following: adding items, modifying the quantity of items, deleting items, and clearing the shopping cart.
These operations are all for cookies. Each time, the array in the cookie is taken out, added, modified, deleted, and then recorded in the cookie. As for clearing the shopping cart, it's even simpler. Just set the cookie with the same name to empty.
The following is the specific operation function I wrote
//Add to cart
function addcart($goods_id,$goods_num){
$cur_cart_array = unserialize(stripslashes($_COOKIE['shop_cart_info']));
if($cur_cart_array==""){
$ cart_info[0][] = $goods_id;
$cart_info[0][] = $goods_num;
setcookie("shop_cart_info",serialize($cart_info));
}elseif($cur_cart_array<>"") {
//Return the largest array key name in reverse order
$ar_keys = array_keys($cur_cart_array);
rsort($ar_keys);
$max_array_keyid = $ar_keys[0]+1;
//Traverse the current shopping cart Array
//Traverse the 0 value of each product information array. If the key value is 0 and the item number is the same, the same item exists in the shopping cart
foreach($cur_cart_array as $goods_current_cart){
foreach($goods_current_cart as $key=>$ goods_current_id){
if($key == 0 and $goods_current_id == $goods_id){
echo "";
exit();
}
}
$cur_cart_array[$max_array_keyid][] = $goods_id;
$ cur_cart_array[$max_array_keyid ][] = $goods_num;
setcookie("shop_cart_info",serialize($cur_cart_array));
}
}
//Delete from shopping cart
function delcart($goods_array_id){
$cur_goods_array = unserialize (stripslashes($_COOKIE['shop_cart_info']));
//Delete the position of the product in the array
unset($cur_goods_array[$goods_array_id]);
setcookie("shop_cart_info",serialize($cur_goods_array));
}
//Modify the quantity of items in the shopping cart
function update_cart($up_id,$up_num,$goods_ids){
//Clear the cookie first so that it can be reset, and pass the three array parameters 1 the identifier of the array 2 the product Quantity array 3 product number array
//If the cookie is not cleared, products with a quantity of zero cannot be processed
setcookie("shop_cart_info","");
foreach($up_id as $song){
//Return the current array first unit; then move the pointer down one position
$goods_nums = current($up_num);
$goods_id = current($goods_ids);
next($up_num);
next($goods_ids);
//When the goods When the quantity is empty, unregister the array value here and use the continue 2 statement to avoid the following operations, and continue with the foreach loop
while($goods_nums == 0){
unset($song);
continue 2;
}
$cur_goods_array[$song][0] = $goods_id;
$cur_goods_array[$song][1] = $goods_nums;
}
setcookie("shop_cart_info",serialize($cur_goods_array));
}
For the subsequent order formation, you need to put it into storage according to your own needs, whether there are points, cash rewards, etc.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
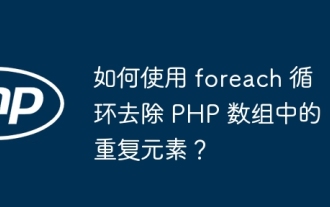
The method of using a foreach loop to remove duplicate elements from a PHP array is as follows: traverse the array, and if the element already exists and the current position is not the first occurrence, delete it. For example, if there are duplicate records in the database query results, you can use this method to remove them and obtain results without duplicate records.
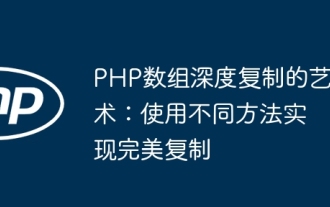
Methods for deep copying arrays in PHP include: JSON encoding and decoding using json_decode and json_encode. Use array_map and clone to make deep copies of keys and values. Use serialize and unserialize for serialization and deserialization.

The performance comparison of PHP array key value flipping methods shows that the array_flip() function performs better than the for loop in large arrays (more than 1 million elements) and takes less time. The for loop method of manually flipping key values takes a relatively long time.
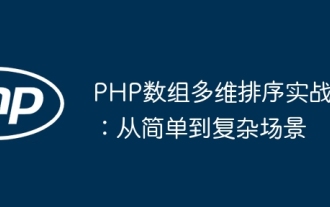
Multidimensional array sorting can be divided into single column sorting and nested sorting. Single column sorting can use the array_multisort() function to sort by columns; nested sorting requires a recursive function to traverse the array and sort it. Practical cases include sorting by product name and compound sorting by sales volume and price.
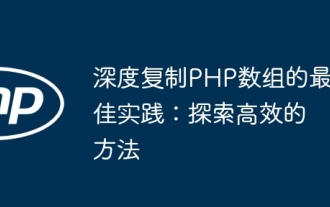
The best practice for performing an array deep copy in PHP is to use json_decode(json_encode($arr)) to convert the array to a JSON string and then convert it back to an array. Use unserialize(serialize($arr)) to serialize the array to a string and then deserialize it to a new array. Use the RecursiveIteratorIterator to recursively traverse multidimensional arrays.
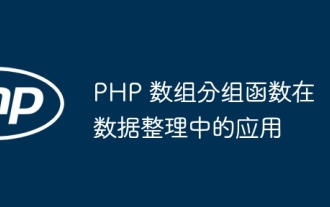
PHP's array_group_by function can group elements in an array based on keys or closure functions, returning an associative array where the key is the group name and the value is an array of elements belonging to the group.
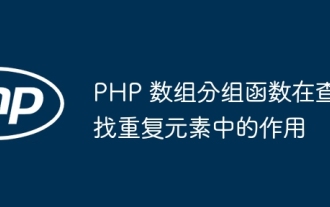
PHP's array_group() function can be used to group an array by a specified key to find duplicate elements. This function works through the following steps: Use key_callback to specify the grouping key. Optionally use value_callback to determine grouping values. Count grouped elements and identify duplicates. Therefore, the array_group() function is very useful for finding and processing duplicate elements.
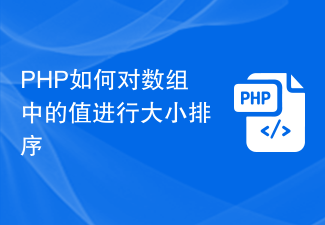
PHP is a commonly used server-side scripting language widely used in website development and data processing fields. In PHP, it is a very common requirement to sort the values in an array by size. By using the built-in sort function, you can easily sort arrays. The following will introduce how to use PHP to sort the values in an array by size, with specific code examples: 1. Sort the values in the array in ascending order:
