Asp.net uses SignalR to send pictures
1. Introduction
In the previous article, we have introduced how to use SignalR to implement the chat room function. In this article, we will implement how to use SignalR to implement the function of sending pictures.
2. The idea of implementing the function of sending pictures
I will tell this article in the same way as before. First, let us clarify the idea of implementing the function of sending pictures.
In addition to directly specifying the path of the image (this implementation method is also called: http URI schema), the image can also be displayed through the Data Uri Schema. This method allows images to be embedded directly in web pages in the form of strings. The form is as follows:
<img src="/static/imghw/default1.png" data-src="~/Scripts/jquery-2.2.2.min.js" class="lazy" / alt="Asp.net uses SignalR to send pictures" >
The above code uses Data Url Schema to display images. The advantages and disadvantages of Data Uri Schema are:
Advantages:
It can reduce Http requests, because if you use http Uri Schema to specify the image address, the client needs to issue Http requests for each image, by using Data Uri This method can save bandwidth and HTTP requests
Disadvantages:
It is only supported by IE8 and above, and the size limit cannot exceed 32KB.
In addition, Base64 content will increase the size of the image by 33%, but GZIP compression can be enabled on the server to reduce the size of the content. Despite this, since sending an Http request will attach a lot of additional information (such as Http Header, etc.), the cumulative content size is still larger than the content added by using Base64 encoding.
Since SignalR is based on text transmission, it is necessary to send pictures.
You can only send the Base64-encoded string of the picture to the SignalR server, and then the server will push the Base64 string to the client that needs to receive the picture, and the client will then use the Data Uri method to display the picture on the page, thus Complete the transfer of pictures.
Of course, you can also upload pictures to Azure Bob Table like Jabbr (an open source project that uses SignalR to implement instant chat), and then return the Uri of the Blob to all clients to display the picture. In fact, this implementation is similar to our implementation here. The client can read the image and display it through the Uri of the blob. In short, the implementation idea is to indirectly convert the content of the image binary file into text for transmission.
3. Implementation code for sending pictures using SignalR
Before the specific implementation, here we need to introduce a file upload plug-in - boostrap-fileinput. This plug-in is used to provide image preview function. For specific usage of the plug-in, please refer to the github site or the implementation code of this article.
1. Implement our hub
public class ChatHub : Hub { /// <summary> /// 供客户端调用的服务器端代码 /// </summary> /// <param name="name"></param> /// <param name="message"></param> public void Send(string name,string message) { // 调用所有客户端的sendMessage方法 Clients.All.sendMessage(name, message); } // 发送图片 public void SendImage(string name,IEnumerable<ImageData> images) { foreach (var item in images ?? Enumerable.Empty<ImageData>()) { if(String.IsNullOrEmpty(item.Image)) continue; Clients.All.receiveImage(name, item.Image); // 调用客户端receiveImage方法将图片进行显示 } } /// <summary> /// 客户端连接的时候调用 /// </summary> /// <returns></returns> public override Task OnConnected() { Trace.WriteLine("客户端连接成功"); return base.OnConnected(); } }
2. The implementation code of HomeController mainly generates a random user name for each client, and then stores the user name in the Session.
public class HomeController : Controller { private static readonly char[] Constant = { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z' }; // GET: Home public ActionResult Index() { Session["username"] = GenerateRandomName(4); return View(); } /// <summary> /// 产生随机用户名函数 /// </summary> /// <param name="length">用户名长度</param> /// <returns></returns> private static string GenerateRandomName(int length) { var newRandom = new System.Text.StringBuilder(62); var rd = new Random(DateTime.Now.Millisecond); for (var i = 0; i < length; i++) { newRandom.Append(Constant[rd.Next(62)]); } return newRandom.ToString(); } }
3. The next step is to implement the front-end page.
<html> <head> <meta name="viewport" content="width=device-width" /> <title>使用SignalR实现发送图片</title> <link href="/Content/bootstrap.min.css" rel="stylesheet"> <link href="/Content/bootstrap-fileinput/css/fileinput.min.css" media="all" rel="stylesheet" type="text/css" /> </head> <body> <p class="container"> <p>用户名:<p id="username"></p></p> <input type="text" id="message" /> <br/> <br /> <input id="fileinput" type="file"> <br /> <input type="button" id="sendmessage" value="Send" /> <input type="hidden" id="displayname" /> <ul id="discussion"></ul> </p> <script type="text/javascript" ></script> <script src="~/Scripts/jquery.signalR-2.2.0.min.js"></script> <script src="~/signalr/hubs"></script> <script src="/Scripts/fileinput.js" type="text/javascript"></script> <script src="/Scripts/bootstrap.min.js" type="text/javascript"></script> <script> $(function () { var userName = '@Session["username"]'; $('#username').html(userName); // 引用自动生成的集线器代理 var chat = $.connection.chatHub; // 定义服务器端调用的客户端sendMessage来显示新消息 chat.client.sendMessage = function (name, message) { // 向页面添加消息 $('#discussion').append('<li><strong>' + htmlEncode(name) + '</strong>: ' + htmlEncode(message) + '</li>'); }; chat.client.receiveImage = function (name, base64) { // 向页面添加消息 $('#discussion').append('<image class = "file-preview-image" style="width:auto;height:100px;" src=' + base64 + '/>'); }; // 设置焦点到输入框 $('#message').focus(); // 开始连接服务器 $.connection.hub.start().done(function () { $('#sendmessage').click(function () { // 调用服务器端集线器的Send方法 chat.server.send(userName, $('#message').val()); // 清空输入框信息并获取焦点 $('#message').val('').focus(); }); }); $("#fileinput").fileinput({ allowedFileExtensions: ["jpg", "png", "gif", "jpeg"], maxImageWidth: 700, maxImageHeight: 700, resizePreference: 'height', maxFileCount: 1, resizeImage: true }); $("#fileinput").on('fileloaded', function (event, file, previewId, index, reader) { var readers = new FileReader(); readers.onloadend = function () { $(".file-preview-image").attr('src', readers.result); }; readers.readAsDataURL(file); }); $('#sendmessage').click(function() { var imagesJson = $('.file-preview-image').map(function() { var $this = $(this); return { image: $this.attr('src'), filename: $this.attr('data-filename') }; }).toArray(); chat.server.sendImage(userName, imagesJson); }); }); // 为显示的消息进行Html编码 function htmlEncode(value) { var encodedValue = $('<p />').text(value).html(); return encodedValue; } </script> </body> </html>
4. Operational effect
After the above three steps, the function of sending pictures using SignalR is already operational. Next, let's take a look at the specific operating effect.
Here, the introduction of all the contents of this article is over. Next, we will introduce how to use the Html5 Notification API to implement the reminder function of new messages.
For more articles related to Asp.net using SignalR to send pictures, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


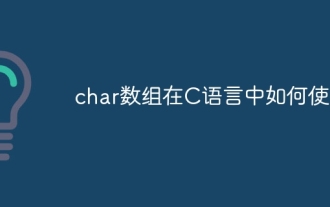
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
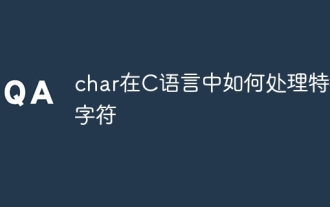
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
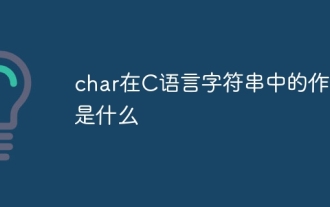
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
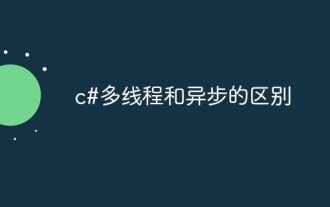
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
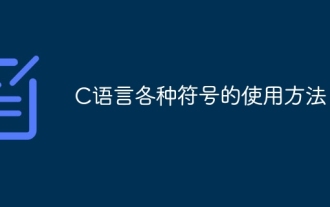
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
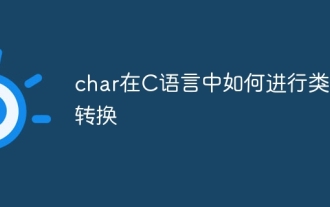
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
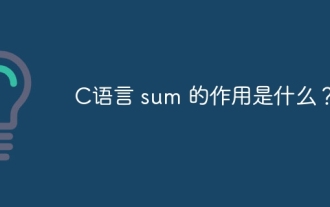
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
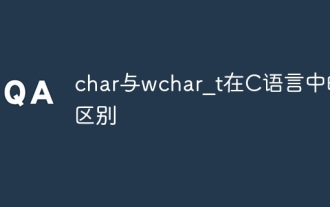
In C language, the main difference between char and wchar_t is character encoding: char uses ASCII or extends ASCII, wchar_t uses Unicode; char takes up 1-2 bytes, wchar_t takes up 2-4 bytes; char is suitable for English text, wchar_t is suitable for multilingual text; char is widely supported, wchar_t depends on whether the compiler and operating system support Unicode; char is limited in character range, wchar_t has a larger character range, and special functions are used for arithmetic operations.
