[python tutorial] Python tuple
Python tuples
Python tuples are similar to lists, except that the elements of the tuple cannot be modified.
Use parentheses for tuples and square brackets for lists.
Tuple creation is very simple, just add elements in brackets and separate them with commas.
The following example:
tup1 = ('physics', 'chemistry', 1997, 2000); tup2 = (1, 2, 3, 4, 5 ); tup3 = "a", "b", "c", "d";
Create an empty tuple
tup1 = ();
When the tuple contains only one element, you need to add a comma after the element
tup1 = (50,);
element Groups are similar to strings. The subscript index starts from 0 and can be intercepted, combined, etc.
Accessing tuples
Tuples can use subscript indexes to access the values in the tuple, as shown in the following example:
#!/usr/bin/python tup1 = ('physics', 'chemistry', 1997, 2000); tup2 = (1, 2, 3, 4, 5, 6, 7 ); print "tup1[0]: ", tup1[0] print "tup2[1:5]: ", tup2[1:5]
The output result of the above example:
tup1[0]: physics tup2[1:5]: [2, 3, 4, 5]
Modify the tuple
The element values in the tuple are not allowed to be modified, but we can connect and combine the tuples, as shown in the following example:
#!/usr/bin/python tup1 = (12, 34.56); tup2 = ('abc', 'xyz'); # 以下修改元组元素操作是非法的。 # tup1[0] = 100; # 创建一个新的元组 tup3 = tup1 + tup2; print tup3;
The output result of the above example:
(12, 34.56, 'abc', 'xyz')
Delete a tuple
Element values in a tuple are not allowed to be deleted, but we can use the del statement to delete the entire tuple, as shown in the following example:
#!/usr/bin/python tup = ('physics', 'chemistry', 1997, 2000); print tup; del tup; print "After deleting tup : " print tup;
Above example After the tuple is deleted, the output variable will have exception information, and the output is as follows:
('physics', 'chemistry', 1997, 2000) After deleting tup : Traceback (most recent call last): File "test.py", line 9, in <module> print tup; NameError: name 'tup' is not defined
Tuple operator
Like strings, the + sign and * can be used between tuples number to perform operations. This means that they can be combined and copied, resulting in a new tuple.
Python expression
Result
Description
len((1, 2, 3) ) 3 Calculate the number of elements
(1, 2, 3) + (4, 5, 6) (1, 2, 3, 4, 5, 6) Connect
['Hi !'] * 4 ('Hi!', 'Hi!', 'Hi!', 'Hi!') Copy
3 in (1, 2, 3) True Whether the element exists
for x in (1, 2, 3): print x, 1 2 3 Iteration
Tuple index, interception
Because the tuple is also a sequence, we can access the tuple The element at the specified position in the index can also be intercepted, as shown below:
Tuple:
L = ('spam', 'Spam', 'SPAM!')
Python expression
Result
Description
L[2] 'SPAM!' Read the third element
L[-2] 'Spam' Reverse Read in the direction; read the penultimate element
L[1:] ['Spam', 'SPAM!'] Intercept element
No closing delimiter
Any unsigned object, separated by commas, defaults to a tuple, as shown in the following example:
#!/usr/bin/python print 'abc', -4.24e93, 18+6.6j, 'xyz'; x, y = 1, 2; print "Value of x , y : ", x,y;
The above example allows the result:
abc -4.24e+93 (18+6.6j) xyz Value of x , y : 1 2
tuple built-in function
Python element The group contains the following built-in functions
serial number
method and description
1 cmp(tuple1, tuple2)
Compares two tuple elements.
2 len(tuple)
Calculate the number of tuple elements.
3 max(tuple)
Returns the maximum value of the element in the tuple.
4 min(tuple)
Returns the minimum value of the element in the tuple.
5 tuple(seq)
Convert the list to a tuple.
The above is the content of [python tutorial] Python tuples. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


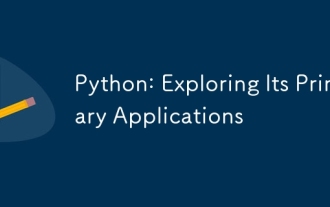
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
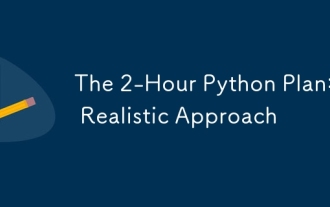
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
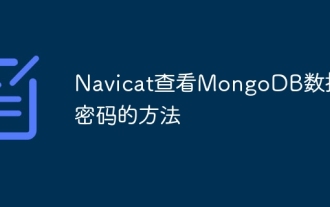
It is impossible to view MongoDB password directly through Navicat because it is stored as hash values. How to retrieve lost passwords: 1. Reset passwords; 2. Check configuration files (may contain hash values); 3. Check codes (may hardcode passwords).
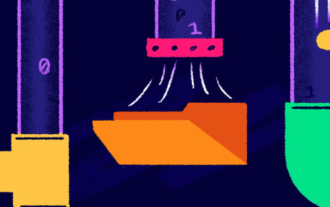
As a data professional, you need to process large amounts of data from various sources. This can pose challenges to data management and analysis. Fortunately, two AWS services can help: AWS Glue and Amazon Athena.
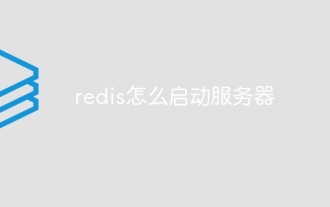
The steps to start a Redis server include: Install Redis according to the operating system. Start the Redis service via redis-server (Linux/macOS) or redis-server.exe (Windows). Use the redis-cli ping (Linux/macOS) or redis-cli.exe ping (Windows) command to check the service status. Use a Redis client, such as redis-cli, Python, or Node.js, to access the server.
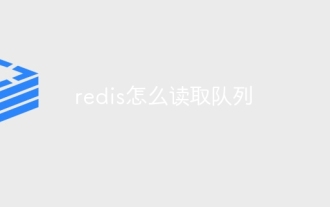
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
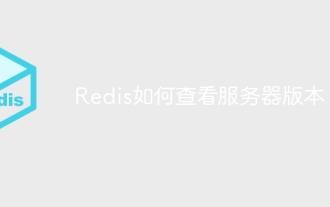
Question: How to view the Redis server version? Use the command line tool redis-cli --version to view the version of the connected server. Use the INFO server command to view the server's internal version and need to parse and return information. In a cluster environment, check the version consistency of each node and can be automatically checked using scripts. Use scripts to automate viewing versions, such as connecting with Python scripts and printing version information.
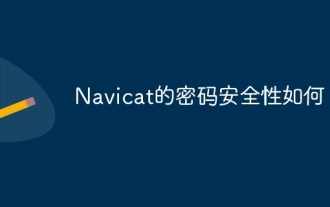
Navicat's password security relies on the combination of symmetric encryption, password strength and security measures. Specific measures include: using SSL connections (provided that the database server supports and correctly configures the certificate), regularly updating Navicat, using more secure methods (such as SSH tunnels), restricting access rights, and most importantly, never record passwords.
