New Java Movement: Test Driven Development 3---User Registration
The user registration process is for the user to enter the user name and password, and then correctly establish the user's basic information and account information into the database.
Let us start with a simple step. The first step is to write a test case, pass in cmd=registerUser, userName=Yan Tao, then call the Servlet, and finally in d:/ablog/app. The received user name is written back in the html file. The first name is the test code:
@Test public void testRegisterUser001() { HttpServletRequest request = new HttpJunitRequest(); Map<String, String[]>params = (Map<String, String[]>)request.getParameterMap(); String[] cmd = new String [1]; cmd[0] = "registerUser"; params.put("cmd", cmd); String[] userName = new String[1]; userName[0] = "y闫涛t"; params.put("userName", userName); MainServlet m = new MainServlet(); HttpServletResponse response = new HttpJunitResponse(); try { m.doGet(request, response); response.getWriter().close(); } catch (IOException | ServletException e) { // TODO Auto-generated catch block e.printStackTrace(); } assertTrue(1>0); }
The following is the code to pass this test case. First, when MainServlet jumps according to the command parameters, add the following code:
switch (cmd) { case "registerUser": registerUser(request, response); break;
The specific processing function is as follows Display:
private void registerUser(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException { PrintWriter out = response.getWriter(); String userName = null; if (request.getParameter("userName") != null) { userName = request.getParameter("userName"); } out.print("userName=" + userName + "!"); }
Run the test case, then open d:/ablog/app.html, you will find that the user name has been printed into the file.
But wait a minute, the above test steps are not only not automated, but also more convenient than directly opening the browser to access the URL, especially when the returned page contains a lot of content. So we need to transform the above test cases so that they can be tested automatically. As you can know from the previous article, we will store the content that needs to be displayed on the page in the request object, usually in the form of Map
Okay, let’s implement this feature first. In MainServlet.registerUser, define the Map
Map<String, Object> model = new HashMap<String, Object>(); long userId = 101; model.put("userId", "" + userId); request.setAttribute("model", model);
In the test case, we test whether the userId value is set correctly. The code is as follows Display:
Map<String, Object> model = (Map<String, Object>)request.getAttribute("model"); if (model.get("userId") != null && Long.parseLong("" + model.get("userId"))==101) { rst = true; }
At this time we will find that the test case cannot pass! This is normal, because the getAttribute and setAttribute methods are not implemented in our HttpJunitRequest object. In order to make the test case pass, we need to add the following code to HttpJunitRequest:
private final Map<String, Object> attributes = new HashMap<String, Object>(); @Override public Object getAttribute(String key) { return attributes.get(key); } @Override public void setAttribute(String key, Object value) { attributes.put(key, value); }
Run the test case again at this time, and finally you can It shows the green pass mark that makes us happy both physically and mentally.
So far, we have basically built a minimum runnable system and can develop it according to the concept of TDD.
As can be seen from the above example, we first think of a test case for a small function every time, and then code to try to pass this test case. After passing it, we continue to add new functions. Each test, development, and verification only takes up to 20 to 30 minutes. The code written in this way has basically been fully tested, and the code quality can be guaranteed to a certain extent.
The above is the content of the New Java Movement: Test Driven Development 3---user registration. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
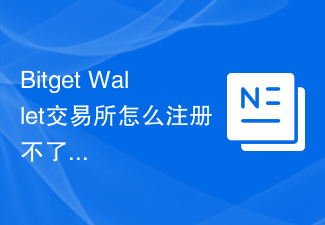
There are various reasons for being unable to register for the BitgetWallet exchange, including account restrictions, unsupported regions, network issues, system maintenance and technical failures. To register for the BitgetWallet exchange, please visit the official website, fill in the information, agree to the terms, complete registration and verify your identity.

DeepSeek's official website is now launching multiple discount activities to provide users with a shopping experience. New users sign up to get a $10 coupon, and enjoy a 15% limited time discount for the entire audience. Recommend friends can also earn rewards, and you can accumulate points for redemption of gifts when shopping. The event deadlines are different. For details, please visit the DeepSeek official website for inquiries.
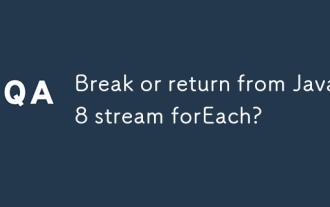
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
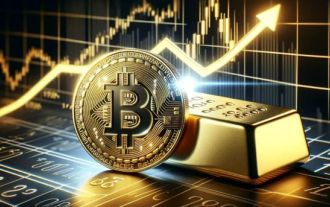
Gate.io Sesame Open is the world's leading blockchain digital asset trading platform, including fiat currency trading, currency trading, leveraged trading, perpetual contracts, ETF leveraged tokens, wealth management, Startup initial public offering and other sections, providing users with security, stability, openness and transparency.
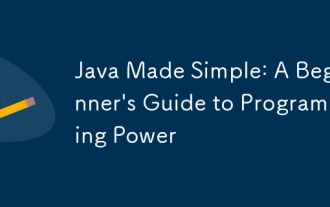
Java Made Simple: A Beginner's Guide to Programming Power Introduction Java is a powerful programming language used in everything from mobile applications to enterprise-level systems. For beginners, Java's syntax is simple and easy to understand, making it an ideal choice for learning programming. Basic Syntax Java uses a class-based object-oriented programming paradigm. Classes are templates that organize related data and behavior together. Here is a simple Java class example: publicclassPerson{privateStringname;privateintage;
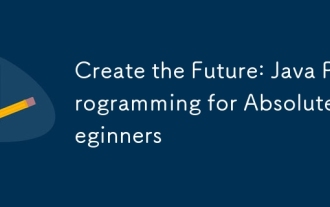
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
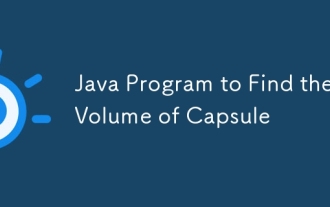
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
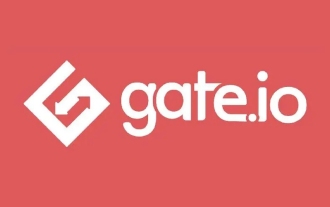
Gate.io Exchange is one of the world's leading cryptocurrency trading platforms. This guide provides step-by-step tutorials to help users register and trade with Gate.io. The registration process includes selecting the registration method (phone, email or social account), filling in information, setting a login password, and completing identity authentication. Trading tutorials include accessing trading pages, selecting trading pairs, entering trading information, placing an order, and viewing order status. With the guidance of this article, users can easily start trading cryptocurrency on Gate.io.
