New Java Movement: Test Driven Development 3---User Registration 3
Up to now, we have not touched on the substantive issue of user registration, that is, adding users to the database. Let's deal with this requirement now.
First you need to determine the technology used for database access. You can choose Hibernate, JPA or JDBC here. I believe that the vast majority of applications use Hibernate as the database access technology. Others may choose JPA, but we choose JDBC here. The reason is relatively simple. The underlying things seem to be more complicated, but once mastered, it is relatively easier to master because of its less content. This O-R mapping model adds many abstract concepts and details. We usually only look at the tip of the iceberg of these architectures. If you want to master the things under the iceberg, the difficulty is several orders of magnitude more difficult than directly mastering JDBC.
In addition, due to our test-driven development architecture, if we want to convert to other architectures, we can refactor the existing code. Under the guarantee of test cases, we can safely modify the code.
Okay, we first use the DAO mode to define the database access interface class UserDao. The code is as follows:
public interface UserDao { public long registerUser(Map<String, Object> userInfo); }
Generally speaking, we use the Mysql database, so we define the Dao implementation class UserMysqlDao , the code is as follows:
public class UserMysqlDao implements UserDao { @Override public long registerUser(Map<String, Object> userInfo) { // TODO Auto-generated method stub return 0; }
Of course we do not want the user to determine the database used, and then instantiate the corresponding implementation class. For this reason, we need to adopt the factory mode and introduce the DaoFactory class. The code is as follows:
public class DaoFactory { public static UserDao getUserDao() { UserDao dao = null; switch (dbms) { case "mysql": dao = new UserMysqlDao(); break; } return dao; } public static String getDbms() { return dbms; } public static void setDbms(String dbms) { DaoFactory.dbms = dbms; } private static String dbms = "mysql"; }
As can be seen from the above code, the caller only needs to call DaoFactory.getUserDao() to obtain the implementation class.
The following is the problem of database connection. We hope to use the database connection pool when running on application servers such as Jboss, and use DriverManager in unit testing, so we need to define a DataSource encapsulation class JdbcDs to handle the above situation. The code is as follows:
public class JdbcDs { public static Connection getConnection() throws SQLException { Connection conn = null; if (iDebug <= 0) { conn = appDs.getConnection(); } else { try { Class.forName("com.mysql.jdbc.Driver").newInstance(); } catch (InstantiationException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IllegalAccessException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (ClassNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } Properties connectionProps = new Properties(); connectionProps.put("user", "root"); connectionProps.put("password", "yantao"); conn = DriverManager.getConnection( "jdbc:" + "mysql" + "://" + "localhost" + ":" + 3306 + "/XrcjDb", connectionProps); } return conn; } public static int iDebug = 1; public final static String APP_DS = "java:jboss/datasources/XcgDS"; private static DataSource appDs = null; static{ if (null == appDs) { Properties env = new Properties(); try { InitialContext ictx = new InitialContext(env); appDs = (DataSource)ictx.lookup(APP_DS); } catch (NamingException e1) { // TODO Auto-generated catch block e1.printStackTrace(); } } } }
When doing unit testing, just set iDebug to 1. Otherwise the database connection pool will be used.
After all the preparation work is done, the database function development can be officially carried out.
The above is the content of the New Java Movement: Test Driven Development 3---User Registration 3. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


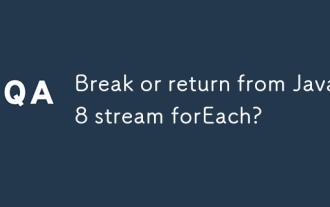
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is

DeepSeek's official website is now launching multiple discount activities to provide users with a shopping experience. New users sign up to get a $10 coupon, and enjoy a 15% limited time discount for the entire audience. Recommend friends can also earn rewards, and you can accumulate points for redemption of gifts when shopping. The event deadlines are different. For details, please visit the DeepSeek official website for inquiries.
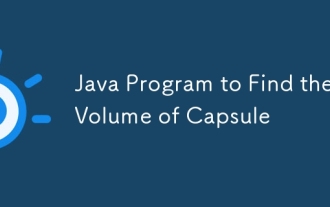
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
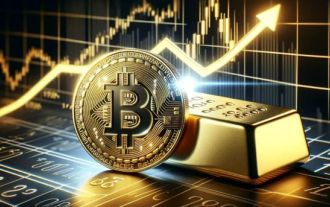
Gate.io Sesame Open is the world's leading blockchain digital asset trading platform, including fiat currency trading, currency trading, leveraged trading, perpetual contracts, ETF leveraged tokens, wealth management, Startup initial public offering and other sections, providing users with security, stability, openness and transparency.
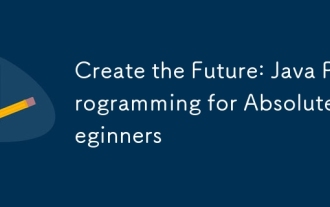
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
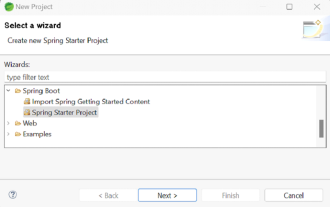
Spring Boot simplifies the creation of robust, scalable, and production-ready Java applications, revolutionizing Java development. Its "convention over configuration" approach, inherent to the Spring ecosystem, minimizes manual setup, allo
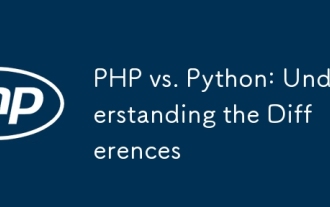
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
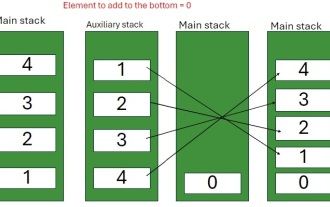
A stack is a data structure that follows the LIFO (Last In, First Out) principle. In other words, The last element we add to a stack is the first one to be removed. When we add (or push) elements to a stack, they are placed on top; i.e. above all the
