


Detailed explanation of how ASP.NET generates graphic verification codes
The example in this article describes how ASP.NET generates graphical verification codes. Share it with everyone for your reference, the details are as follows:
Usually there are three main steps to generate a graphic verification code:
(1) Randomly generate a random string of length N, N The value can be set by the developer. The string can contain numbers, letters, etc.
(2) Create a randomly generated string into a picture and display it.
(3) Save the verification code.
Create a new page as default.aspx, place a TextBox control and an Image control. The TextBox control is used to input the generated string, the Image control is used to display the string, and its picture is used to verify the generated graphic. Code imageUrl="/default.aspx";
The source code of the default.aspx page is:
<form id="form1" runat="server"> <div> <asp:TextBox ID="TextBox1" runat="server"></asp:TextBox> <asp:Image ID="Image1" imageUrl=“/default.aspx” runat="server" /> </div> </form>
The code of the graphic verification code is :
using System; using System.Configuration; using System.Data; using System.Linq; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.HtmlControls; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Xml.Linq; using System.Drawing; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { string validateNum = CreateRandomNum(4); CreateImage(validateNum); Session["ValidateNum"] = validateNum; } } //生产随机数 private string CreateRandomNum(int NumCount) { string allChar = "0,1,2,3,4,5,6,7,8,9,A,B,C,D,E,F,G,H,I,J,K,O,P,Q,R,S,T,U,W,X,Y,Z,a,b,c,d,e,f,g,h,i,j,k,m,n,o,p,q,s,t,u,w,x,y,z"; string[] allCharArray = allChar.Split(',');//拆分成数组 string randomNum = ""; int temp = -1; //记录上次随机数的数值,尽量避免产生几个相同的随机数 Random rand = new Random(); for (int i = 0; i < NumCount; i++) { if (temp != -1) { rand = new Random(i*temp*((int)DateTime.Now.Ticks)); } int t = rand.Next(35); if (temp == t) { return CreateRandomNum(NumCount); } temp = t; randomNum += allCharArray[t]; } return randomNum; } //生产图片 private void CreateImage(string validateNum) { if (validateNum == null || validateNum.Trim() == string.Empty) return; //生成BitMap图像 System.Drawing.Bitmap image = new System.Drawing.Bitmap(validateNum.Length*12+12,22); Graphics g = Graphics.FromImage(image); try { //生成随机生成器 Random random = new Random(); //清空图片背景 g.Clear(Color.White); //画图片的背景噪音线 for (int i = 0; i < 25; i++) { int x1 = random.Next(image.Width); int x2 = random.Next(image.Width); int y1 = random.Next(image.Height); int y2 = random.Next(image.Height); g.DrawLine(new Pen(Color.Silver),x1,x2,y1,y2); } Font font = new System.Drawing.Font("Arial",12,(System.Drawing.FontStyle.Bold|System.Drawing.FontStyle.Italic)); System.Drawing.Drawing2D.LinearGradientBrush brush=new System.Drawing.Drawing2D.LinearGradientBrush(new Rectangle(0,0,image.Width,image.Height),Color.Blue,Color.DarkRed,1.2f,true); g.DrawString(validateNum,font,brush ,2,2); //画图片的前景噪音点 for( int i=0;i<100;i++) { int x=random.Next(image.Width); int y=random.Next(image.Height); image.SetPixel(x,y,Color.FromArgb(random.Next())); } //画图片的边框线 g.DrawRectangle(new Pen(Color.Silver),0,0,image.Width-1,image.Height-1); System.IO.MemoryStream ms=new System.IO.MemoryStream(); //将图像保存到指定流 image.Save(ms,System.Drawing.Imaging.ImageFormat.Gif); Response.ClearContent(); Response.ContentType="image/Gif"; Response.BinaryWrite(ms.ToArray()); } finally { g.Dispose(); image.Dispose(); } } }
I hope this article will be helpful to everyone in asp.net programming.
For more detailed explanations of how ASP.NET generates graphic verification codes and related articles, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


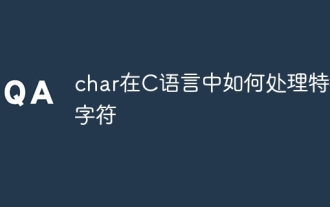
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
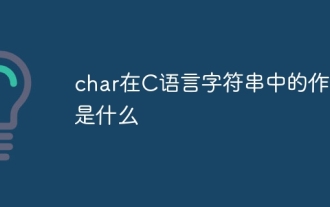
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
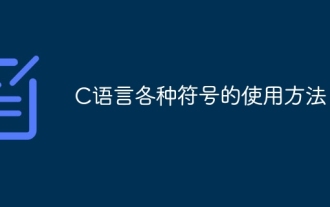
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
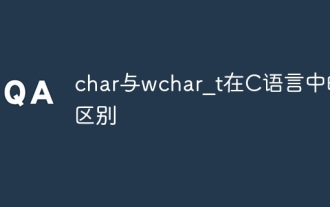
In C language, the main difference between char and wchar_t is character encoding: char uses ASCII or extends ASCII, wchar_t uses Unicode; char takes up 1-2 bytes, wchar_t takes up 2-4 bytes; char is suitable for English text, wchar_t is suitable for multilingual text; char is widely supported, wchar_t depends on whether the compiler and operating system support Unicode; char is limited in character range, wchar_t has a larger character range, and special functions are used for arithmetic operations.
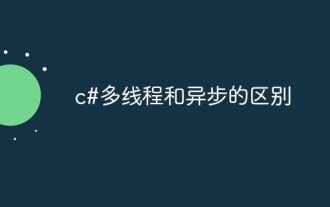
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
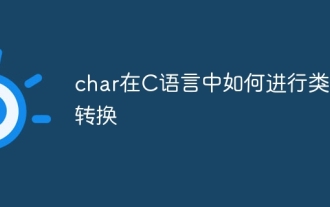
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
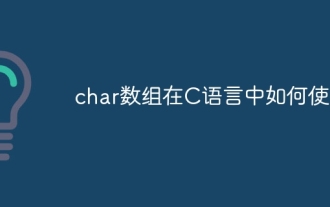
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
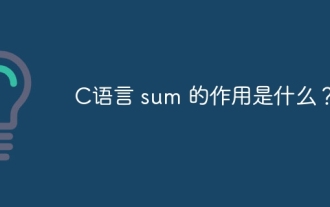
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
