


ASP.NET inspired by 12306 dynamic verification code implements dynamic GIF verification code
12306 website launched a "color dynamic verification code mechanism". Not only did the new version of the verification code often have overlapping characters, but it also kept shaking. Many people shouted that they couldn't see clearly and said, "That verification code is an abstract painting by Picasso." What!" The customer service of China Railway Corporation said: This is the only way to be able to purchase tickets normally. Many ticket-grabbing software are on the verge of being scrapped, causing dissatisfaction from many netizens who complained that they were “too abstract and artistic.”
In the past, verification codes were sometimes used in projects, but they were basically static. This time I wanted to join in the fun of 12306. Without further ado, let’s get to the point and start with the code.
Implementation method:
public void ShowCode() { //对象实例化 Validate GifValidate = new Validate(); #region 对验证码进行设置(不进行设置时,将以默认值生成) //验证码位数,不小于4位 GifValidate.ValidateCodeCount = 4; //验证码字体型号(默认13) GifValidate.ValidateCodeSize = 13; //验证码图片高度,高度越大,字符的上下偏移量就越明显 GifValidate.ImageHeight = 23; //验证码字符及线条颜色(需要参考颜色类) GifValidate.DrawColor = System.Drawing.Color.BlueViolet; //验证码字体(需要填写服务器安装的字体) GifValidate.ValidateCodeFont = "Arial"; //验证码字符是否消除锯齿 GifValidate.FontTextRenderingHint = false; //定义验证码中所有的字符(","分离),似乎暂时不支持中文 GifValidate.AllChar = "1,2,3,4,5,6,7,8,9,0,A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U,W,X,Y,Z"; #endregion //输出图像(Session名称) GifValidate.OutPutValidate("GetCode"); }
Calling the main method:
public class Validate { public string AllChar = "1,2,3,4,5,6,7,8,9,0,A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U,W,X,Y,Z"; public Color DrawColor = Color.BlueViolet; public bool FontTextRenderingHint = false; public int ImageHeight = 0x17; private byte TrueValidateCodeCount = 4; protected string ValidateCode = ""; public string ValidateCodeFont = "Arial"; public float ValidateCodeSize = 13f; private void CreateImageBmp(out Bitmap ImageFrame) { char[] chArray = this.ValidateCode.ToCharArray(0, this.ValidateCodeCount); int width = (int) (((this.TrueValidateCodeCount * this.ValidateCodeSize) * 1.3) + 4.0); ImageFrame = new Bitmap(width, this.ImageHeight); Graphics graphics = Graphics.FromImage(ImageFrame); graphics.Clear(Color.White); Font font = new Font(this.ValidateCodeFont, this.ValidateCodeSize, FontStyle.Bold); Brush brush = new SolidBrush(this.DrawColor); int maxValue = (int) Math.Max((float) ((this.ImageHeight - this.ValidateCodeSize) - 3f), (float) 2f); Random random = new Random(); for (int i = 0; i < this.TrueValidateCodeCount; i++) { int[] numArray = new int[] { (((int) (i * this.ValidateCodeSize)) + random.Next(1)) + 3, random.Next(maxValue) }; Point point = new Point(numArray[0], numArray[1]); if (this.FontTextRenderingHint) { graphics.TextRenderingHint = TextRenderingHint.SingleBitPerPixel; } else { graphics.TextRenderingHint = TextRenderingHint.AntiAlias; } graphics.DrawString(chArray[i].ToString(), font, brush, (PointF) point); } graphics.Dispose(); } private void CreateImageGif() { AnimatedGifEncoder encoder = new AnimatedGifEncoder(); MemoryStream stream = new MemoryStream(); encoder.Start(); encoder.SetDelay(5); encoder.SetRepeat(0); for (int i = 0; i < 10; i++) { Bitmap bitmap; this.CreateImageBmp(out bitmap); this.DisposeImageBmp(ref bitmap); bitmap.Save(stream, ImageFormat.Png); encoder.AddFrame(Image.FromStream(stream)); stream = new MemoryStream(); } encoder.OutPut(ref stream); HttpContext.Current.Response.ClearContent(); HttpContext.Current.Response.ContentType = "image/Gif"; HttpContext.Current.Response.BinaryWrite(stream.ToArray()); stream.Close(); stream.Dispose(); } private void CreateValidate() { this.ValidateCode = ""; string[] strArray = this.AllChar.Split(new char[] { ',' }); int index = -1; Random random = new Random(); for (int i = 0; i < this.ValidateCodeCount; i++) { if (index != -1) { random = new Random((i * index) * ((int) DateTime.Now.Ticks)); } int num3 = random.Next(0x23); if (index == num3) { this.CreateValidate(); } index = num3; this.ValidateCode = this.ValidateCode + strArray[index]; } if (this.ValidateCode.Length > this.TrueValidateCodeCount) { this.ValidateCode = this.ValidateCode.Remove(this.TrueValidateCodeCount); } } private void DisposeImageBmp(ref Bitmap ImageFrame) { Graphics graphics = Graphics.FromImage(ImageFrame); Pen pen = new Pen(this.DrawColor, 1f); Random random = new Random(); Point[] pointArray = new Point[2]; for (int i = 0; i < 15; i++) { pointArray[0] = new Point(random.Next(ImageFrame.Width), random.Next(ImageFrame.Height)); pointArray[1] = new Point(random.Next(ImageFrame.Width), random.Next(ImageFrame.Height)); graphics.DrawLine(pen, pointArray[0], pointArray[1]); } graphics.Dispose(); } public void OutPutValidate(string ValidateCodeSession) { this.CreateValidate(); this.CreateImageGif(); HttpContext.Current.Session[ValidateCodeSession] = this.ValidateCode; } public byte ValidateCodeCount { get { return this.TrueValidateCodeCount; } set { if (value > 4) { this.TrueValidateCodeCount = value; } } } }
The above is the entire process of implementing ASP.NET, and the source code is also attached. I hope it can help everyone better Learn how to generate ASP.NET verification codes.
For more articles related to ASP.NET implementing dynamic GIF verification code inspired by 12306 dynamic verification code, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


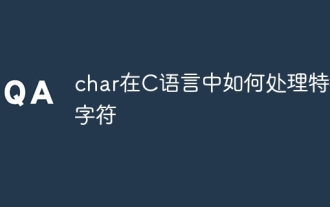
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
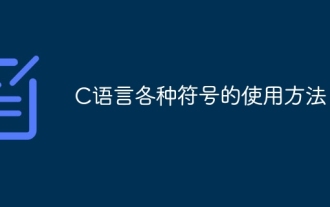
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
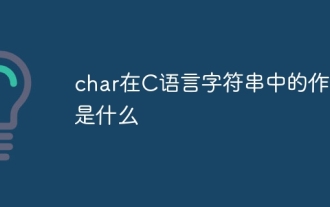
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
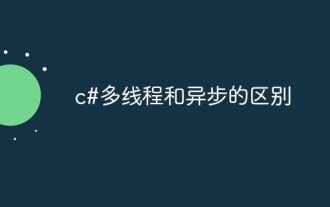
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
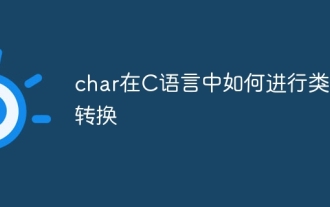
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
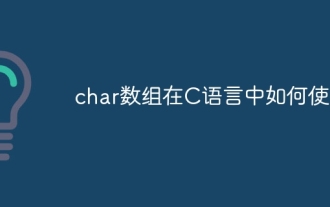
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
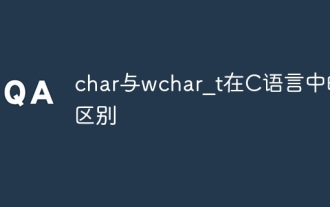
In C language, the main difference between char and wchar_t is character encoding: char uses ASCII or extends ASCII, wchar_t uses Unicode; char takes up 1-2 bytes, wchar_t takes up 2-4 bytes; char is suitable for English text, wchar_t is suitable for multilingual text; char is widely supported, wchar_t depends on whether the compiler and operating system support Unicode; char is limited in character range, wchar_t has a larger character range, and special functions are used for arithmetic operations.
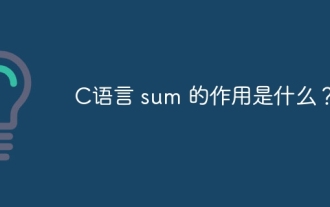
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
