Tutorial on writing graphical menus using Java
There are two types of menus: drop-down menus and pop-up menus. This chapter only discusses drop-down menu programming methods. Menus are different from JComboBox and JCheckBox in that they are always visible in the interface. The menu is similar to the JComboBox in that only one item can be selected at a time.
Selecting an option in the drop-down menu or pop-up menu generates an ActionEvent event. The event is sent to the monitor for that option, and the meaning of the event is interpreted by the monitor.
Menu Bar, Menu and Menu Items
The drop-down menu is visually represented by the name that appears on the menu bar. The menu bar (JMenuBar) usually appears at the top of the JFrame. A menu bar displays multiple drop-down menus. The name of the menu. There are two ways to activate drop-down menus. One is to press the mouse button, keep it pressed, and move the mouse until you release the mouse to complete the selection. The highlighted menu item is the selected one. Another way is to click the mouse when the cursor is over the menu name in the menu bar. In this case, the menu will expand and the menu item will be highlighted.
A menu bar can contain multiple menus (JMenu), and each menu can have many menu items (JMenuItem). For example, the menu bar of the Eclipse environment includes File, Edit, Source, Refactor and other menus, and each menu has many menu items. For example, the File menu has menu items such as New, Open File, Close, and Close All.
The method of adding a menu to a window is: first create a menu bar object, then create several menu objects, place these menu objects in the menu bar, and then add menu items to each menu object as required.
Menu items in the menu can also be a complete menu. Since a menu item can be another complete menu, a hierarchical menu structure can be constructed.
1. Menu bar
The instance of class JMenuBar is the menu bar. For example, the following code creates the menu bar object menubar:
JMenuBar menubar = new JMenuBar();
To add a menu bar to the window, you must use the setJMenuBar() method in the JFrame class. For example, code:
setJMenuBar(menubar);
Common methods of class JMenuBar are:
add(JMenu m): Add menu m to the menu bar.
countJMenus(): Get the number of menu items in the menu bar.
getJMenu(int p): Get the menu in the menu bar.
remove(JMenu m): Delete menu m in the menu bar.
2. Menu
The object created by class JMenu is the menu. Commonly used methods of the JMenu class are as follows:
JMenu(): Create a menu with an empty title.
JMenu(String s): Create a menu titled s.
add(JMenuItem item): Add the menu option specified by the parameter item to the menu.
add(JMenu menu): Add the menu specified by the parameter menu to the menu. Implement embedding submenus in menus.
addSeparator(): Draw a separator line between menu options.
getItem(int n): Get the menu item at the specified index.
getItemCount(): Get the number of menu items.
insert(JMenuItem item,int n): Insert menu item item at menu position n.
remove(int n): Delete menu item at menu position n
removeAll(): Remove all menu items of the menu.
3. Menu items
Instances of class JMenuItem are menu items. The common methods of class JMenuItem are as follows:
JMenuItem(): Construct an untitled menu item.
JMenuItem(String s): Constructs a titled menu item.
setEnabled(boolean b): Set whether the current single item can be selected.
isEnabled(): Returns whether the current menu item can be selected by the user.
getLabel(): Get the name of the menu item.
setLabel(): Set the name of the menu option.
addActionListener(ActionListener e): Set the monitor for the menu item. The monitor accepts action events when a menu is clicked.
4. Handle menu events
The event source of the menu is to click a menu item with the mouse. The interface to handle this event is ActionListener, the interface method to be implemented is actionPerformed(ActionEvent e), and the method to obtain the event source is getSource().
[Example] Implementation method of a small application indicating that the window has a menu bar. There is a button. When the button is in the open window state, clicking the button will open a window. The window has a menu bar with two menus, and each menu has three menu items. When a menu item is selected, the menu item monitoring method displays the words that the corresponding menu item is selected in the text box.
import java.applet.* import javax.swing.*; import java.awt.*; import java.awt.event.*; class MenuWindow extends JFrame implements ActionListener{ public static JtextField text; private void addItem(JMenu Menu,String menuName,ActionListener listener){ JMenuItem anItem = new JMenuItem(menuName); anItem.setActionCommand(menuName); anItem.addActionListener(listener); Menu.add(anItem); } public MenuWindow(String s,int w,int h){ setTitle(s); Container con = this.getContentPane(); con.setLayout(new BorderLayout()); this.setLocation(100,100); this.setSize(w,h); JMenu menu1 = new JMenu("体育"); addItem(menu1," 跑步",this); addItem(menu1," 跳绳",this); addItem(menu1,"打球",this); JMenu menu2 = JMenu("娱乐"); addItem(menu2,"唱歌",this); addItem(menu2,"跳舞",this); addItem(menu2,"游戏",this); JMenuBar menubar = new JMenuBar(); text = new JTextField(); menubar.add(menu1); menubar.add(menu2); setJMenuBar(MenuBar); con.add(text,BorderLayout.NORTH); } public void actionPerformed(ActionEvent e){ text.setText(e.getActionCommand()+"菜单项被选中!"); } } public class Example6_5 extends Applet implements ActionListener{ MenuWindow window; JButton button; boolean bflg; public void init(){ button = new JButton("打开我的体育娱乐之窗");bflg =true; window = new MenuWindow("体育娱乐之窗",100,100); button.addActionListener(this); add(button); } public void actionPerformed(ActionEvent e){ if(e.getSource()==button){ if(bflg){ window.setVisible(true); bflg = false; button.setLabel("关闭我的体育娱乐之窗"); } else{ window.setVisible(false); bflg = true; button.setLabel("打开我的体育娱乐之窗"); } } } }
5. Embed submenu
Create a menu and create multiple menu items, one of which Another menu (containing other menu items), which constitutes menu nesting. For example, change the relevant code in the above program to the following:
Menu menu1,menu2,item4; MenuItem item3,item5,item6,item41,item42;
Insert the following code to create item41 and item42 menu items, and add them to the item4 menu:
item41= new MenuItem(“东方红”); item42 = new MenuItem(“牡丹”); item4.add(item41); item4.add(item42);
Then click item4 menu, two more menu items will open for selection.
6. Add an exit item to the menu
Add a new menu item and add monitoring to the menu item. Use the System.exit() method in the corresponding monitoring method to click the menu. Exit the Java runtime environment when the item is selected. For example, the following code:
… item7 = new MenuItem(“退出”); item7.addActionListener(this); … public void actionPerformed(ActionEvent e){ if(e.getSource()==item7){ System.exit(0); } }
7.设置菜单项的快捷键
用MenuShortcut类为菜单项设置快捷键。构造方法是MenuShortcut(int key)。其中key可以取值KeyEvent.VK_A至KenEvent.VK_Z,也可以取 ‘a'到 ‘z'键码值。菜单项使用setShortcut(MenuShortcut k)方法来设置快捷键。例如,以下代码设置字母e为快捷键。
class Herwindow extends Frame implements ActionListener{ MenuBar menbar; Menu menu; MenuItem item; MenuShortcut shortcut = new MenuShortcut(KeyEvent.VK_E); … item.setShortcut(shortcut); … }
选择框菜单项
菜单也可以包含具有持久的选择状态的选项,这种特殊的菜单可由JCheckBoxMenuItem类来定义。JCheckBoxMenuItem对象像选择框一样,能表示一个选项被选中与否,也可以作为一个菜单项加到下拉菜单中。点击JCheckBoxMenuItem菜单时,就会在它的左边出现打勾符号或清除打勾符号。例如,在例6.5程序的类MenuWindow中,将代码
addItem(menu1,“跑步”,this);addItem(menu1,”跳绳”,this);
改写成以下代码,就将两个普通菜单项“跑步“和“跳绳”改成两个选择框菜单项:
JCheckBoxMenuItem item1 = new JCheckBoxMenuItem(“跑步”); JCheckBoxMenuItem item2 = new JCheckBoxMenuItem(“跳绳”); item1.setActionCommand(“跑步”); item1.addActionListener(this); menu1.add(item1); item2.setActionCommand(“跳绳”); item2.addActionListener(this); menu1.add(item2);
更多使用Java编写图形化的菜单的教程相关文章请关注PHP中文网!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




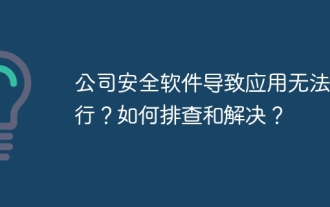
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
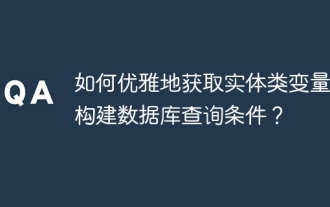
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
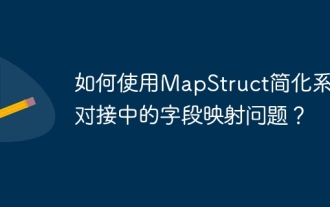
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
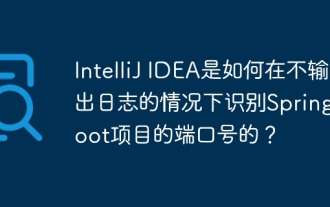
Start Spring using IntelliJIDEAUltimate version...
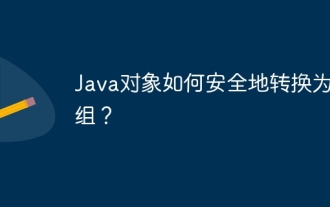
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
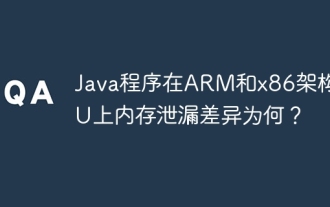
Analysis of memory leak phenomenon of Java programs on different architecture CPUs. This article will discuss a case where a Java program exhibits different memory behaviors on ARM and x86 architecture CPUs...
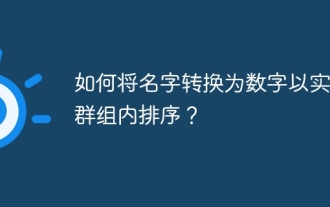
How to convert names to numbers to implement sorting within groups? When sorting users in groups, it is often necessary to convert the user's name into numbers so that it can be different...
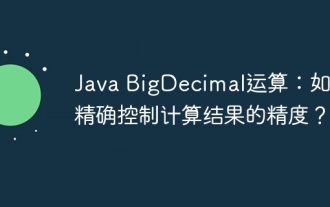
Java...
