


Android UI control series: DatePicker, TimePicker (date and time selection)
Date and time are functions available on any mobile phone platform, and the same is true for Android.
DatePicker: Used to implement date (year, month, day)
TimePicker: Used to implement time (hour, minute, second)
Calendar: Calendar is a set of annual date objects and an integer field. Abstract base class for conversion between, such as month, day, hour, etc.
For example
final Calendar calendar=Calendar.getInstance(); mYear=calendar.get(Calendar.YEAR);—获取年份 mMonth=calendar.get(Calendar.MONTH);—获取月份 mDay=calendar.get(Calendar.DAY_OF_MONTH);—获取日 mHour=calendar.get(Calendar.HOUR_OF_DAY);—获取时 mMinute=calendar.get(Calendar.MINUTE);—获取分
etc., you can refer to the API documentation
TimePickerDialog and DatePickerDialog are time classes in the form of dialog boxes.
Without further ado, the example is as follows:
DateTest.java file
package org.loulijun.datetest; import java.util.Calendar; import android.app.Activity; import android.app.DatePickerDialog; import android.app.TimePickerDialog; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.DatePicker; import android.widget.TextView; import android.widget.TimePicker; public class DateTest extends Activity { /** Called when the activity is first created. */ TextView textview; TimePicker timepicker; DatePicker datepicker; Button btn1; Button btn2; //JAVA中的Calendar类 Calendar c; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); /*Like other locale-sensitive classes, Calendar provides a class method, getInstance, *for getting a default instance of this class for general use. *Calendar's getInstance method returns a calendar whose locale is based on system settings *and whose time fields have been initialized with the current date and time: */ c=Calendar.getInstance(); textview=(TextView)findViewById(R.id.textview); btn1=(Button)findViewById(R.id.button1); btn2=(Button)findViewById(R.id.button2); //获取DatePicker对象 datepicker=(DatePicker)findViewById(R.id.datepicker1); //将日历初始化为当前系统时间,并设置其事件监听 datepicker.init(c.get(Calendar.YEAR),c.get(Calendar.MONTH),c.get(Calendar.DAY_OF_MONTH), new DatePicker.OnDateChangedListener() { @Override public void onDateChanged(DatePicker view, int year, int monthOfYear, int dayOfMonth) { // TODO Auto-generated method stub //当前日期更改时,在这里设置 c.set(year,monthOfYear,dayOfMonth); } }); //获取TimePicker对象 timepicker=(TimePicker)findViewById(R.id.timepicker1); //设置为24小时制显示时间 timepicker.setIs24HourView(true); //监听时间改变 timepicker.setOnTimeChangedListener(new TimePicker.OnTimeChangedListener() { @Override public void onTimeChanged(TimePicker view, int hourOfDay, int minute) { // TODO Auto-generated method stub //时间改变处理 //c.set(year,month,hourOfDay,minute,second); } }); btn1.setOnClickListener(new Button.OnClickListener() { @Override public void onClick(View v) { // TODO Auto-generated method stub new DatePickerDialog(DateTest.this, new DatePickerDialog.OnDateSetListener() { @Override public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) { //设置日历 } },c.get(Calendar.YEAR),c.get(Calendar.MONTH),c.get(Calendar.DAY_OF_MONTH)).show(); } }); btn2.setOnClickListener(new Button.OnClickListener() { @Override public void onClick(View v) { // TODO Auto-generated method stub new TimePickerDialog(DateTest.this, new TimePickerDialog.OnTimeSetListener() { @Override public void onTimeSet(TimePicker view, int hourOfDay, int minute) { // TODO Auto-generated method stub //设置时间 } },c.get(Calendar.HOUR_OF_DAY),c.get(Calendar.MINUTE),true).show(); } }); } }
main.xml file
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" > <TextView android:id="@+id/textview" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="时间控件使用" /> <DatePicker android:id="@+id/datepicker1" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TimePicker android:id="@+id/timepicker1" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="设置日期" android:layout_gravity="center" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="设置时间" android:layout_gravity="center" /> </LinearLayout>
Run result As follows:
When you click the "Set Date" button, the following dialog box style DatePickerDialog will be displayed
When you click the "Set Time" button, a TimePickerDialog with the following dialog box style will pop up
The above is the Android UI control series: DatePicker, TimePicker (date and time selection) Content, please pay attention to the PHP Chinese website (www.php.cn) for more related content!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


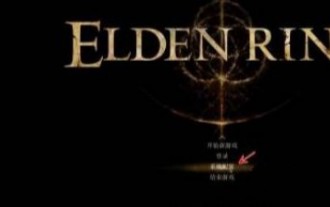
In Elden's Ring, the UI page of this game will be automatically hidden after a period of time. Many players do not know how the UI is always displayed. Players can select the gauge display configuration in the display and sound configuration. Click to turn it on. Why does the Elden Ring UI keep displaying? 1. First, after we enter the main menu, click [System Configuration]. 2. In the [Display and Sound Configuration] interface, select the meter display configuration. 3. Click Enable to complete.
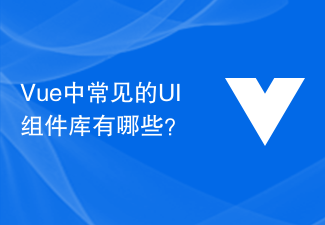
Vue is a popular JavaScript framework that uses a component-based approach to build web applications. In the Vue ecosystem, there are many UI component libraries that can help you quickly build beautiful interfaces and provide rich functions and interactive effects. In this article, we will introduce some common VueUI component libraries. ElementUIElementUI is a Vue component library developed by the Ele.me team. It provides developers with a set of elegant,
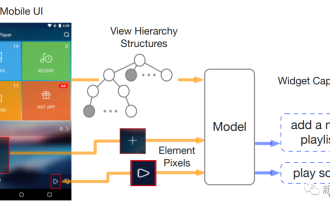
For AI, "playing with mobile phones" is not an easy task. Just identifying various user interfaces (UI) is a big problem: not only must the type of each component be identified, but also the symbols used , position to determine the function of the component. Understanding the UI of mobile devices can help realize various human-computer interaction tasks, such as UI automation. Previous work on mobile UI modeling usually relies on the view hierarchy information of the screen, directly utilizing the structural data of the UI, and thereby bypassing the problem of identifying components starting from the screen pixels. However, view hierarchy is not available in all scenarios. This method usually outputs erroneous results due to missing object descriptions or misplaced structural information, so even if you use
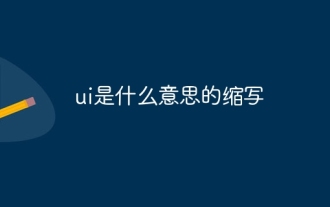
UI is the abbreviation of "User Interface", which is mainly used to describe the human-computer interaction, operation logic and beautiful interface of the software. The purpose of UI design is to make software operation easier and more comfortable, and fully reflect its positioning and characteristics. Common UI designs are divided into physical UI and virtual UI, among which virtual UI is widely used in the Internet field.

The jQuery mobile UI framework is a tool for developing mobile applications. It provides rich interface components and interactive effects, allowing developers to quickly build excellent mobile user interfaces. In this article, we will explore some of the most popular jQuery mobile UI frameworks and provide specific code examples to help readers better understand and use these frameworks. 1.jQueryMobiljQueryMobile is an open source mobile UI framework based on HTML5 and CSS3.
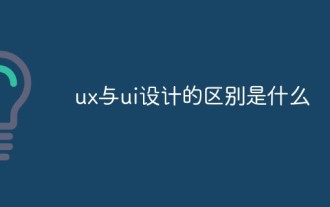
The difference between ux and ui design: 1. UX makes the interface easier to use, and UI makes the interface more beautiful; 2. UX allows users to achieve their goals, and UI makes the interface enhance the brand sense; 3. UX core goals guide users to complete tasks, UI does not; 4. The deliverables of UI and UX are different. The output of UX includes UX experience report, function definition, function planning, project progress, etc., while the output of UI includes visual and interaction, visual design, brand design, motion design, and component design. and design language, etc.
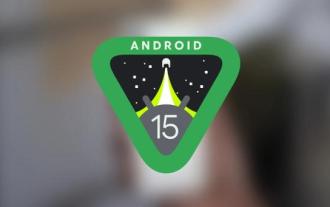
A few days ago, Google officially pushed the Android 15 Beta 4 update to eligible Pixel smartphone and tablet users. This marks that the Android 15 operating system has entered the platform stable stage, indicating that its stable version will be officially released with global users in the next few days. Meet. At the same time, this development also injects new vitality into Samsung Electronics' Galaxy device series to accelerate the development process of its OneUI7.0 version. 1.[Android15Beta4 promotes Samsung OneUI7.0 stable build](https://www.cnbeta.com/articles/tech/1427022.htm) With Android15Bet
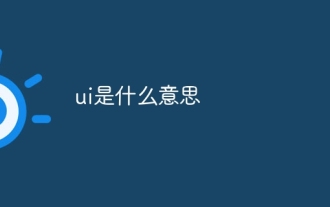
UI, the full name of user interface, refers to the design of human-computer interaction, operation logic and beautiful interface in software. It is divided into physical UI and virtual UI, among which virtual UI is widely used in mobile Internet. Good UI design not only makes the software look tasteful, but more importantly, makes software operation comfortable and easy, fully reflecting the software's positioning and characteristics.
