Summary of usage of C# List
Namespace: System.Collections.Generic
public class List
List< T>Class is the generic equivalent of the ArrayList class. This class implements the IList
Benefits of generics: It adds great efficiency and flexibility to writing object-oriented programs using the C# language. There is no forced boxing and unboxing of value types, or downcasting of reference types, so performance is improved.
Performance Notes:
When deciding to use IList
If you use a reference type for type T of the IList
"Any reference or value type added to the ArrayList will be implicitly cast up to Object. If the item is a value type, it must be boxed when it is added to the list. Unboxing operations are performed during retrieval. Casts and boxing and unboxing operations all reduce performance; the impact of boxing and unboxing is significant in situations where large collections must be iterated over.”
1. Basic and common methods of List:
Declaration:
1. List
T is the element type in the list , now take the string type as an example
E.g.: List
2, List
Create a List with a collection as a parameter
E.g.:
string[] temArr = { "Ha", "Hunter", "Tom", "Lily ", "Jay", "Jim", "Kuku", "Locu" };
List
Add elements:
1. List. Add(T item) Add an element
E.g.:mList.Add("John");
2. List. AddRange(IEnumerable
E.g.:
string[] temArr = { "Ha","Hunter", "Tom", "Lily", "Jay", "Jim", "Kuku" , "Locu" };
mList.AddRange(temArr);
3. Insert(int index, T item); Add an element at the index position
E.g.: mList.Insert (1, "Hei");
Traverse the elements in the List:
foreach (T element in mList) T的类型与mList声明时一样 { Console.WriteLine(element); }
E.g.:
foreach (string s in mList) { Console.WriteLine(s); }
Delete the element:
1. List. Remove(T item) deletes a value
E.g.: mList.Remove("Hunter");
2. List. RemoveAt(int index); Delete the next Element marked index
E.g.: mList.RemoveAt(0);
3. List.RemoveRange(int index, int count);
Start from the subscript index , delete count elements
E.g.: mList.RemoveRange(3, 2);
Determine whether an element is in the List:
List. Contains(T item ) Returns true or false, very practical
E.g.:
if (mList.Contains("Hunter")) { Console.WriteLine("There is Hunter in the list"); } else { mList.Add("Hunter"); Console.WriteLine("Add Hunter successfully."); }
Sort the elements in the List:
Clear List: List. Clear ()
E.g.: mList.Clear() ;
int count = mList.Count();
Console.WriteLine("The num of elements in the list: " +count);
2. Advanced and powerful methods of List:
List.Find method: Searches for elements that match the conditions defined by the specified predicate and returns the first matching element in the entire List.
public T Find(Predicate
string listFind = mList.Find(name => //name是变量,代表的是mList { //中元素,自己设定 if (name.Length > 3) { return true; } return false; }); Console.WriteLine(listFind); //输出是Hunter
E.g.:
string listFind1 = mList.Find(ListFind); //委托给ListFind函数
Console.WriteLine(listFind); //输出是Hunter
ListFind函数:
public bool ListFind(string name) { if (name.Length > 3) { return true; } return false; }
List.FindLast 方法:搜索与指定谓词所定义的条件相匹配的元素,并返回整个 List 中的最后一个匹配元素。
public T FindLast(Predicate
用法与List.Find相同。
List.TrueForAll方法: 确定是否 List 中的每个元素都与指定的谓词所定义的条件相匹配。
public bool TrueForAll(Predicate
委托给拉姆达表达式:
E.g.:
bool flag = mList.TrueForAll(name => { if (name.Length > 3) { return true; } else { return false; } } ); Console.WriteLine("True for all: "+flag); //flag值为false
委托给一个函数,这里用到上面的ListFind函数:
E.g.:
bool flag = mList.TrueForAll(ListFind); //委托给ListFind函数
Console.WriteLine("True for all: "+flag); //flag值为false
这两种方法的结果是一样的。
List.FindAll方法:检索与指定谓词所定义的条件相匹配的所有元素。
public List
E.g.:
List<string> subList = mList.FindAll(ListFind); //委托给ListFind函数 foreach (string s in subList) { Console.WriteLine("element in subList: "+s); }
这时subList存储的就是所有长度大于3的元素
List.Take(n): 获得前n行 返回值为IEnumetable
E.g.:
IEnumerable<string> takeList= mList.Take(5); foreach (string s in takeList) { Console.WriteLine("element in takeList: " + s); }
这时takeList存放的元素就是mList中的前5个
List.Where方法:检索与指定谓词所定义的条件相匹配的所有元素。跟List.FindAll方法类似。
E.g.:
IEnumerable<string> whereList = mList.Where(name => { if (name.Length > 3) { return true; } else { return false; } }); foreach (string s in subList) { Console.WriteLine("element in subList: "+s); }
这时subList存储的就是所有长度大于3的元素
List.RemoveAll方法:移除与指定的谓词所定义的条件相匹配的所有元素。
public int RemoveAll(Predicate
E.g.:
mList.RemoveAll(name => { if (name.Length > 3) { return true; } else { return false; } }); foreach (string s in mList) { Console.WriteLine("element in mList: " + s); }
这时mList存储的就是移除长度大于3之后的元素。
List
比如
List
intList.Add(34); //添加
intList.Remove(34);//删除
intList.RemoveAt(0); //删除位于某处的元素
intList.Count; //链表长度
还有Insert,Find,FindAll,Contains等方法,也有索引方法 intList[0] = 23;
1.减少了装箱拆箱
2.便于编译时检查数据类型
List
更多C# List

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
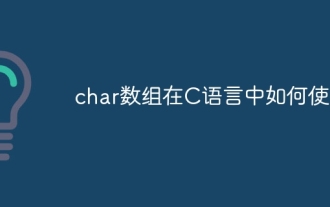
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
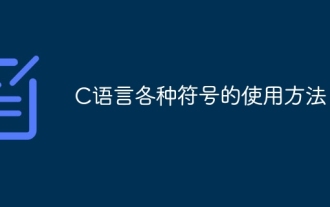
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
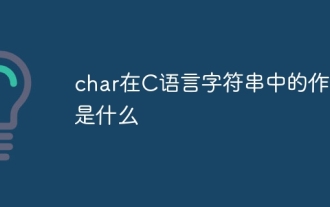
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
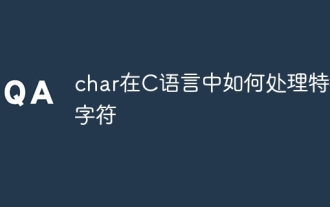
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
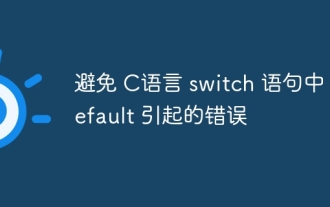
A strategy to avoid errors caused by default in C switch statements: use enums instead of constants, limiting the value of the case statement to a valid member of the enum. Use fallthrough in the last case statement to let the program continue to execute the following code. For switch statements without fallthrough, always add a default statement for error handling or provide default behavior.
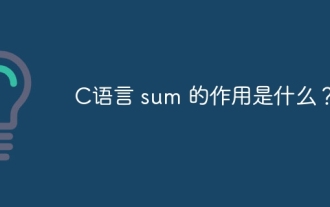
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
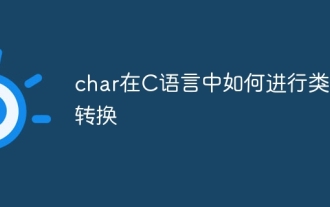
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
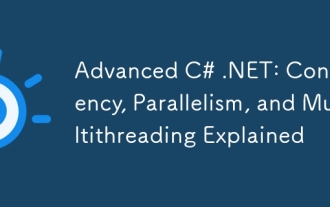
C#.NET provides powerful tools for concurrent, parallel and multithreaded programming. 1) Use the Thread class to create and manage threads, 2) The Task class provides more advanced abstraction, using thread pools to improve resource utilization, 3) implement parallel computing through Parallel.ForEach, 4) async/await and Task.WhenAll are used to obtain and process data in parallel, 5) avoid deadlocks, race conditions and thread leakage, 6) use thread pools and asynchronous programming to optimize performance.
