A brief discussion on JavaScript for loop closure
A netizen asked a question, why is the output of the following html always 5, instead of clicking on each p, the corresponding 1, 2, 3, 4, 5 will be alerted.
<html > <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>闭包演示</title> <script type="text/javascript"> function init() { var pAry = document.getElementsByTagName("p"); for( var i=0; i<pAry.length; i++ ) { pAry[i].onclick = function() { alert(i); } } } </script> </head> <body onload="init();"> <p>产品一</p> <p>产品二</p> <p>产品三</p> <p>产品四</p> <p>产品五</p> </body> </html>
The solutions are as follows
1. Save the variable i on each paragraph object (p)
function init() { var pAry = document.getElementsByTagName("p"); for( var i=0; i<pAry.length; i++ ) { pAry[i].i = i; pAry[i].onclick = function() { alert(this.i); } } }
2. Save the variable i in the anonymous function itself
function init2() { var pAry = document.getElementsByTagName("p"); for( var i=0; i<pAry.length; i++ ) { (pAry[i].onclick = function() { alert(arguments.callee.i); }).i = i; } }
3. Add a layer of closure, and pass i to the inner function in the form of a function parameter
function init3() { var pAry = document.getElementsByTagName("p"); for( var i=0; i<pAry.length; i++ ) { (function(arg){ pAry[i].onclick = function() { alert(arg); }; })(i);//调用时参数 } }
4. Add a layer of closure, i is passed to the memory function in the form of a local variable
function init4() { var pAry = document.getElementsByTagName("p"); for( var i=0; i<pAry.length; i++ ) { (function () { var temp = i;//调用时局部变量 pAry[i].onclick = function() { alert(temp); } })(); } }
5. Add A layer of closure that returns a function as a response event (note the subtle difference from 3)
function init5() { var pAry = document.getElementsByTagName("p"); for( var i=0; i<pAry.length; i++ ) { pAry[i].onclick = function(arg) { return function() {//返回一个函数 alert(arg); } }(i); } }
#6. Use Function to implement. In fact, every time a function instance is generated, a closure will be generated. Package
function init6() { var pAry = document.getElementsByTagName("p"); for( var i=0; i<pAry.length; i++ ) { pAry[i].onclick = new Function("alert(" + i + ");");//new一次就产生一个函数实例 } }
7. Use Function to implement, pay attention to the difference from 6
function init7() { var pAry = document.getElementsByTagName("p"); for( var i=0; i<pAry.length; i++ ) { pAry[i].onclick = Function('alert('+i+')') } }
The above is a brief talk about JavaScript brought to you by the editor The entire content of the for loop closure is here. I hope you will pay more attention to the PHP Chinese website~
For more articles related to JavaScript for loop closure, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


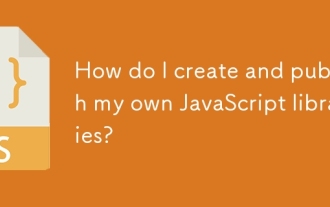
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
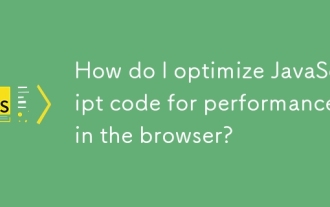
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
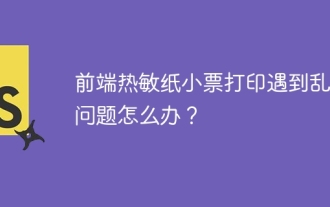
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
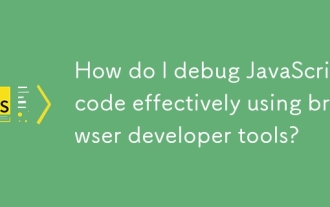
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
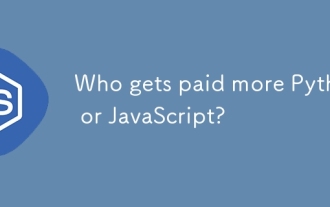
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
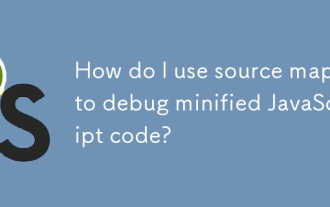
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
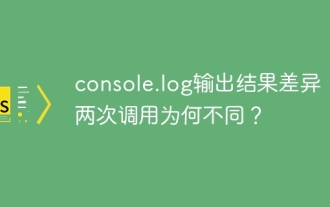
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
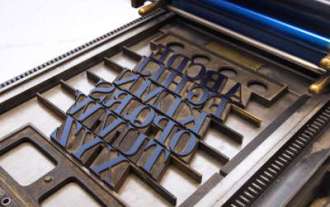
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
