Code examples of four methods for adding event listening in Java
Several ways to add events in Java (reprinted from codebrother's article with slight changes):
/** * Java事件监听处理——自身类实现ActionListener接口,作为事件监听器 * * @author codebrother */ class EventListener1 extends JFrame implements ActionListener { private JButton btBlue, btDialog; public EventListener1() { setTitle("Java GUI 事件监听处理"); setBounds(100, 100, 500, 350); setLayout(new FlowLayout()); btBlue = new JButton("蓝色"); btDialog = new JButton("弹窗"); // 将按钮添加事件监听器 btBlue.addActionListener(this); btDialog.addActionListener(this); add(btBlue); add(btDialog); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } // ***************************事件处理*************************** @Override public void actionPerformed(ActionEvent e) { if (e.getSource() == btBlue) { Container c = getContentPane(); c.setBackground(Color.BLUE); } else if (e.getSource() == btDialog) { JDialog dialog = new JDialog(); dialog.setBounds(300, 200, 400, 300); dialog.setVisible(true); } } } /** * Java事件监听处理——内部类处理 * * @author codebrother */ class EventListener3 extends JFrame { private JButton btBlue, btDialog; // 构造方法 public EventListener3() { setTitle("Java GUI 事件监听处理"); setBounds(100, 100, 500, 350); setLayout(new FlowLayout()); btBlue = new JButton("蓝色"); btDialog = new JButton("弹窗"); // 添加事件监听器对象(面向对象思想) btBlue.addActionListener(new ColorEventListener()); btDialog.addActionListener(new DialogEventListener()); add(btBlue); add(btDialog); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } // 内部类ColorEventListener,实现ActionListener接口 class ColorEventListener implements ActionListener { @Override public void actionPerformed(ActionEvent e) { Container c = getContentPane(); c.setBackground(Color.BLUE); } } // 内部类DialogEventListener,实现ActionListener接口 class DialogEventListener implements ActionListener { @Override public void actionPerformed(ActionEvent e) { JDialog dialog = new JDialog(); dialog.setBounds(300, 200, 400, 300); dialog.setVisible(true); } } } /** * Java事件监听处理——匿名内部类处理 * * @author codebrother */ class EventListener2 extends JFrame { private JButton btBlue, btDialog; public EventListener2() { setTitle("Java GUI 事件监听处理"); setBounds(100, 100, 500, 350); setLayout(new FlowLayout()); btBlue = new JButton("蓝色"); btDialog = new JButton("弹窗"); // 添加事件监听器(此处即为匿名类) btBlue.addActionListener(new ActionListener() { // 事件处理 @Override public void actionPerformed(ActionEvent e) { Container c = getContentPane(); c.setBackground(Color.BLUE); } }); // 并添加事件监听器 btDialog.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { JDialog dialog = new JDialog(); dialog.setBounds(300, 200, 400, 300); dialog.setVisible(true); } }); add(btBlue); add(btDialog); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } /** * Java事件监听处理——外部类处理 * * @author codebrother */ class EventListener4 extends JFrame { private JButton btBlue, btDialog; public EventListener4() { setTitle("Java GUI 事件监听处理"); setBounds(100, 100, 500, 350); setLayout(new FlowLayout()); btBlue = new JButton("蓝色"); btDialog = new JButton("弹窗"); // 将按钮添加事件监听器 btBlue.addActionListener(new ColorEventListener(this)); btDialog.addActionListener(new DialogEventListener()); add(btBlue); add(btDialog); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } // 外部类ColorEventListener,实现ActionListener接口 class ColorEventListener implements ActionListener { private EventListener4 el; ColorEventListener(EventListener4 el) { this.el = el; } @Override public void actionPerformed(ActionEvent e) { Container c = el.getContentPane(); c.setBackground(Color.BLUE); } } // 外部类DialogEventListener,实现ActionListener接口 class DialogEventListener implements ActionListener { @Override public void actionPerformed(ActionEvent e) { JDialog dialog = new JDialog(); dialog.setBounds(300, 200, 400, 300); dialog.setVisible(true); } } public class ActionListenerTest { public static void main(String args[]) { new EventListener2(); } }
For more code examples of four methods to add event listening in Java, please pay attention to the PHP Chinese website for related articles !

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


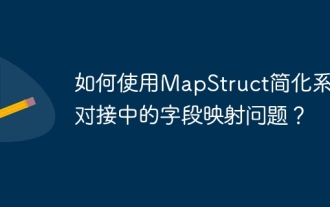
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
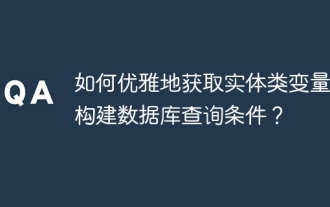
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
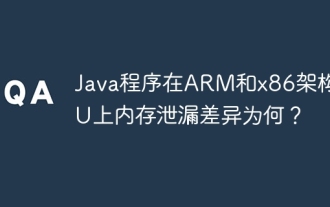
Analysis of memory leak phenomenon of Java programs on different architecture CPUs. This article will discuss a case where a Java program exhibits different memory behaviors on ARM and x86 architecture CPUs...
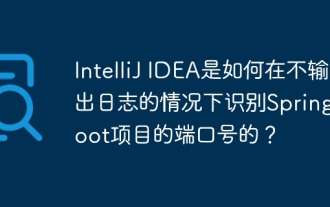
Start Spring using IntelliJIDEAUltimate version...
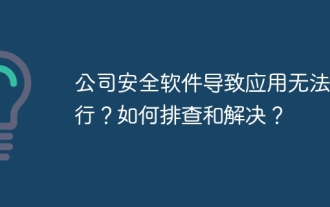
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
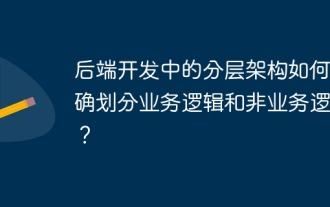
Discussing the hierarchical architecture problem in back-end development. In back-end development, common hierarchical architectures include controller, service and dao...
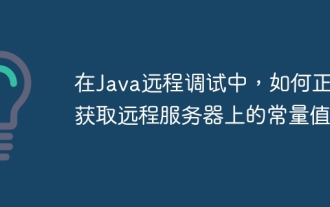
Questions and Answers about constant acquisition in Java Remote Debugging When using Java for remote debugging, many developers may encounter some difficult phenomena. It...
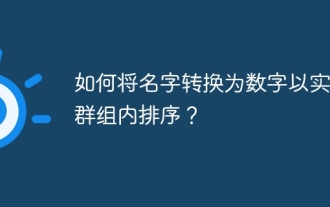
How to convert names to numbers to implement sorting within groups? When sorting users in groups, it is often necessary to convert the user's name into numbers so that it can be different...
