Detailed explanation and sample code of Java Lambda expressions
Java Lambda expression is a new feature introduced in Java 8. It can be said to be a syntactic sugar for simulating functional programming. It is similar to closures in Javascript, but somewhat different. The main purpose is to provide a functional syntax to simplify our coding.
Lambda basic syntax
The basic structure of Lambda is (arguments) -> body, there are the following situations:
When the parameter type can be deduced, there is no need to specify the type , such as (a) -> System.out.println(a)
When there is only one parameter and the type can be deduced, writing () is not forced, such as a -> System.out.println(a )
When the parameter specifies the type, there must be parentheses, such as (int a) -> System.out.println(a)
The parameter can be empty, such as () -> System .out.println(“hello”)
body needs to use {} to include statements. When there is only one statement, {} can be omitted
Common writing methods are as follows:
(a) -> a * a
(int a, int b) -> a + b
(a, b) -> {return a - b;}
() -> ; System.out.println(Thread.currentThread().getId())
Functional InterfaceFunctionalInterface
Concept
Java Lambda expression in functional form interface-based. What is a functional interface (FunctionalInterface)? Simply put, it is an interface with only one method (function). The purpose of this type of interface is for a single operation, which is equivalent to a single function. Common interfaces such as Runnable and Comparator are functional interfaces and are annotated with @FunctionalInterface.
Example
Take Thread as an example to illustrate that it is easy to understand. The Runnable interface is an interface commonly used in our thread programming. It contains a method void run(), which is the running logic of the thread. According to the previous syntax, when we create a new thread, we generally use the anonymous class of Runnable, as follows:
new Thread(new Runnable() { @Override public void run() { System.out.println(Thread.currentThread().getId()); } }).start();
If you write too much, won’t it be boring? The writing rule based on Lambda becomes Concise and clear, as follows:
new Thread(() -> System.out.println(Thread.currentThread().getId())).start();
Pay attention to the parameters of Thread. The anonymous implementation of Runnable is realized in one sentence. It is better to understand it when written as follows
Runnable r = () -> System.out.println(Thread.currentThread().getId ());
new Thread(r).start();
Of course, the purpose of Lambda is not only to write concisely, but to summarize the higher-level purpose after understanding it.
Look at another example of a comparator. According to the traditional writing method, it is as follows:
Integer[] a = {1, 8, 3, 9, 2, 0, 5}; Arrays.sort(a, new Comparator<Integer>() { @Override public int compare(Integer o1, Integer o2) { return o1 - o2; } });
The Lambda expression is written as follows:
Integer[] a = {1, 8, 3, 9, 2, 0, 5};
Arrays.sort(a, (o1, o2) -> o1 - o2);
JDK Functional interface
In order for existing class libraries to directly use Lambda expressions, some interfaces existed before Java 8 that have been marked as functional interfaces:
java.lang.Runnable
java.util.Comparator
java.util.concurrent.Callable
java.io.FileFilter
java.security.PrivilegedAction
java.beans.PropertyChangeListener
Java 8 has added a new package java.util.function, which brings commonly used functional interfaces:
Function
BiFunction
Predicate
BiPredicate
Supplier
Consumer
BiConsumer
UnaryOperator
BinaryOperator
In addition, more specific functions and interfaces are added for basic type processing, including :BooleanSupplier, DoubleBinaryOperator, DoubleConsumer, DoubleFunction
Creating a functional interface
Sometimes we need to implement a functional interface ourselves. The method is also very simple. First, you must ensure that this interface can only have one function operation, and then on the interface type Just mark it with @FunctionalInterface.
Type derivation
Type derivation is the basis of Lambda expressions, and the process of type derivation is the compilation process of Lambda expressions. Take the following code as an example:
Function
编译期间,我理解的类型推导的过程如下:
先确定目标类型 Function
Function 作为函数式接口,其方法签名为:Integer apply(String t)
检测 str -> Integer.parseInt(str) 是否与方法签名匹配(方法的参数类型、个数、顺序 和返回值类型)
如果不匹配,则报编译错误
这里的目标类型是关键,通过目标类型获取方法签名,然后和 Lambda 表达式做出对比。
方法引用
方法引用(Method Reference)的基础同样是函数式接口,可以直接作为函数式接口的实现,与 Lambda 表达式有相同的作用,同样依赖于类型推导。方法引用可以看作是只调用一个方法的 Lambda 表达式的简化。
方法引用的语法为: Type::methodName 或者 instanceName::methodName , 构造函数对应的 methodName 为 new。
例如上面曾用到例子:
Function
对应的方法引用的写法为
Function
根据方法的类型,方法引用主要分为一下几种类型,构造方法引用、静态方法引用、实例上实例方法引用、类型上实例方法引用等
构造方法引用
语法为: Type::new 。 如下面的函数为了将字符串转为数组
方法引用写法
Function
Lambda 写法
Function
传统写法
Function<String, Integer> strToInt = new Function<String, Integer>() { @Override public Integer apply(String str) { return new Integer(str); } };
数组构造方法引用
语法为: Type[]::new 。如下面的函数为了构造一个指定长度的字符串数组
方法引用写法
Function
方法引用写法
Function
传统写法
Function<Integer, String[]> fixedArray = new Function<Integer, String[]>() { @Override public String[] apply(Integer length) { return new String[length]; } };
静态方法引用
语法为: Type::new 。 如下面的函数同样为了将字符串转为数组
方法引用写法
Function
Lambda 写法
Function
传统写法
Function<String, Integer> strToInt = new Function<String, Integer>() { @Override public Integer apply(String str) { return Integer.parseInt(str); } };
实例上实例方法引用
语法为: instanceName::methodName 。如下面的判断函数用来判断给定的姓名是否在列表中存在
List
Predicate
System.out.println(checkNameExists.test("张三"));
System.out.println(checkNameExists.test("张四"));
类型上实例方法引用
语法为: Type::methodName 。运行时引用是指上下文中的对象,如下面的函数来返回字符串的长度
Function<String, Integer> calcStrLength = String::length; System.out.println(calcStrLength.apply("张三")); List<String> names = Arrays.asList(new String[]{"zhangsan", "lisi", "wangwu"}); names.stream().map(String::length).forEach(System.out::println);<br>
又比如下面的函数已指定的分隔符分割字符串为数组
BiFunction
String[] names = split.apply("zhangsan,lisi,wangwu", ",");
System.out.println(Arrays.toString(names));
Stream 对象
概念
什么是 Stream ? 这里的 Stream 不同于 io 中的 InputStream 和 OutputStream,Stream 位于包 java.util.stream 中, 也是 java 8 新加入的,Stream 只的是一组支持串行并行聚合操作的元素,可以理解为集合或者迭代器的增强版。什么是聚合操作?简单举例来说常见的有平均值、最大值、最小值、总和、排序、过滤等。
Stream 的几个特征:
单次处理。一次处理结束后,当前Stream就关闭了。
支持并行操作
常见的获取 Stream 的方式
从集合中获取
Collection.stream();
Collection.parallelStream();
静态工厂
Arrays.stream(array)
Stream.of(T …)
IntStream.range()
这里只对 Stream 做简单的介绍,下面会有具体的应用。要说 Stream 与 Lambda 表达式有什么关系,其实并没有什么特别紧密的关系,只是 Lambda 表达式极大的方便了 Stream 的使用。如果没有 Lambda 表达式,使用 Stream 的过程中会产生大量的匿名类,非常别扭。
举例
以下的demo依赖于 Employee 对象,以及由 Employee 对象组成的 List 对象。
public class Employee { private String name; private String sex; private int age; public Employee(String name, String sex, int age) { super(); this.name = name; this.sex = sex; this.age = age; } public String getName() { return name; } public String getSex() { return sex; } public int getAge() { return age; } @Override public String toString() { StringBuilder builder = new StringBuilder(); builder.append("Employee {name=").append(name).append(", sex=").append(sex).append(", age=").append(age) .append("}"); return builder.toString(); } } List<Employee> employees = new ArrayList<>(); employees.add(new Employee("张三", "男", 25)); employees.add(new Employee("李四", "女", 24)); employees.add(new Employee("王五", "女", 23)); employees.add(new Employee("周六", "男", 22)); employees.add(new Employee("孙七", "女", 21)); employees.add(new Employee("刘八", "男", 20));
打印所有员工
Collection 提供了 forEach 方法,供我们逐个操作单个对象。
employees.forEach(e -> System.out.println(e));
或者
employees.stream().forEach(e -> System.out.println(e));
按年龄排序
Collections.sort(employees, (e1, e2) -> e1.getAge() - e2.getAge());
employees.forEach(e -> System.out.println(e));
或者
employees.stream().sorted((e1, e2) -> e1.getAge() - e2.getAge()).forEach(e -> System.out.println(e));
打印年龄最大的女员工
max/min 返回指定排序条件下最大/最小的元素
Employee maxAgeFemaleEmployee = employees.stream() .filter(e -> "女".equals(e.getSex())) .max((e1, e2) -> e1.getAge() - e2.getAge()) .get(); System.out.println(maxAgeFemaleEmployee);
打印出年龄大于20 的男员工
filter 可以过滤出符合条件的元素
employees.stream()
.filter(e -> e.getAge() > 20 && "男".equals(e.getSex()))
.forEach(e -> System.out.println(e));
打印出年龄最大的2名男员工
limit 方法截取有限的元素
employees.stream() .filter(e -> "男".equals(e.getSex())) .sorted((e1, e2) -> e2.getAge() - e1.getAge()) .limit(2) .forEach(e -> System.out.println(e));
打印出所有男员工的姓名,使用 , 分隔
map 将 Stream 中所有元素的执行给定的函数后返回值组成新的 Stream
String maleEmployeesNames = employees.stream() .map(e -> e.getName()) .collect(Collectors.joining(",")); System.out.println(maleEmployeesNames);
统计信息
IntSummaryStatistics, DoubleSummaryStatistics, LongSummaryStatistics 包含了 Stream 中的汇总数据。
IntSummaryStatistics stat = employees.stream() .mapToInt(Employee::getAge).summaryStatistics(); System.out.println("员工总数:" + stat.getCount()); System.out.println("最高年龄:" + stat.getMax()); System.out.println("最小年龄:" + stat.getMin()); System.out.println("平均年龄:" + stat.getAverage());
总结
Lambda 表达式确实可以减少很多代码,能提高生产力,当然也有弊端,就是复杂的表达式可读性会比较差,也可能是还不是很习惯的缘故吧,如果习惯了,相信会喜欢上的。凡事都有两面性,就看我们如何去平衡这其中的利弊了,尤其是在一个团队中。
以上就是对Java8 JavaLambda 的资料整理,后续继续补充相关资料谢谢大家对本站的支持!
更多Java Lambda 表达式详解及示例代码相关文章请关注PHP中文网!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
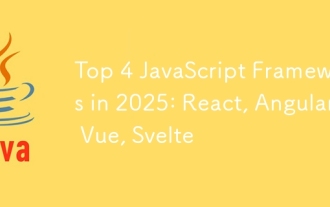
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
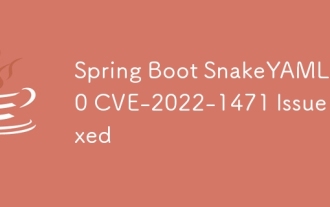
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
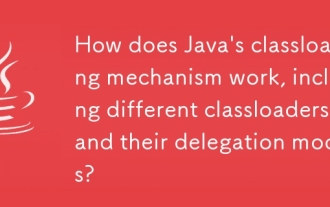
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
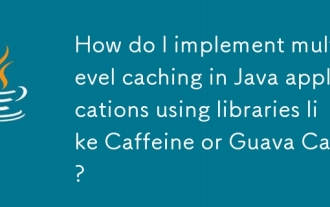
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
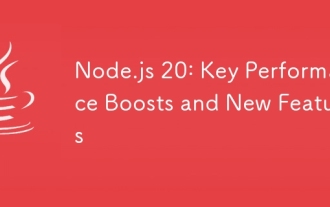
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
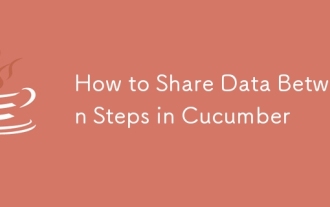
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
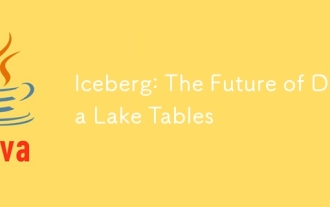
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
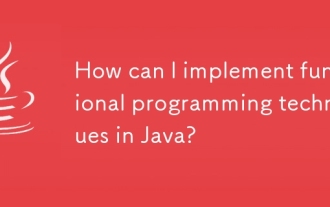
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
