


Java encryption algorithm sharing (rsa decryption, symmetric encryption, md5 encryption)
import java.io.UnsupportedEncodingException; import java.security.InvalidKeyException; import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; import java.security.PrivateKey; import java.security.PublicKey; import java.security.SecureRandom; import javax.crypto.BadPaddingException; import javax.crypto.Cipher; import javax.crypto.IllegalBlockSizeException; import javax.crypto.KeyGenerator; import javax.crypto.NoSuchPaddingException; import javax.crypto.SecretKey; import com.sun.mail.util.BASE64DecoderStream; import com.sun.mail.util.BASE64EncoderStream; public class util { /** * 传入名文和公钥钥对数据进行RSA解密 * <br>返回值:String * <br>@param src * <br>@param pubkey * <br>@return */ public static String rsaEncoding(String src,PublicKey pubkey){ try { Cipher cip = Cipher.getInstance("RSA"); cip.init(cip.ENCRYPT_MODE, pubkey); byte[] by = cip.doFinal(src.getBytes()); return new String(BASE64EncoderStream.encode(by)); } catch (NoSuchAlgorithmException e) { throw new RuntimeException(e); } catch (NoSuchPaddingException e) { throw new RuntimeException(e); } catch (InvalidKeyException e) { throw new RuntimeException(e); } catch (IllegalBlockSizeException e) { throw new RuntimeException(e); } catch (BadPaddingException e) { throw new RuntimeException(e); } } /** * 传入RSA密文和私钥对数据进行解密 * <br>返回值:String * <br>@param sec * <br>@param privkey * <br>@return */ public static String rsaDeEncoding(String sec,PrivateKey privkey){ try { Cipher cip = Cipher.getInstance("RSA"); cip.init(cip.DECRYPT_MODE, privkey); byte[] by = BASE64DecoderStream.decode(sec.getBytes()); return new String(cip.doFinal(by)); } catch (NoSuchAlgorithmException e) { throw new RuntimeException(e); } catch (NoSuchPaddingException e) { throw new RuntimeException(e); } catch (InvalidKeyException e) { throw new RuntimeException(e); } catch (IllegalBlockSizeException e) { throw new RuntimeException(e); } catch (BadPaddingException e) { throw new RuntimeException(e); } } /** * 传入字符串、密钥,并加密字符串(对称加密加密),支持:DES、AES、DESede(3DES) * <br>返回值:String 密文 * <br>@param src * <br>@param key * <br>@param method(DES、AES、DESede) * <br>@return */ //对称加密加密 public static String doubKeyEncoding(String src,String keysrc,String method) { SecretKey key; try { //生成密钥 KeyGenerator kg = KeyGenerator.getInstance(method); //初始化此密钥生成器。 kg.init(new SecureRandom(keysrc.getBytes("utf-8"))); key = kg.generateKey(); //加密 Cipher ciph = Cipher.getInstance(method); ciph.init(Cipher.ENCRYPT_MODE, key); ciph.update(src.getBytes("utf-8")); //使用64进行编码,一避免出现丢数据情景 byte[] by = BASE64EncoderStream.encode(ciph.doFinal()); return new String(by); } catch (NoSuchAlgorithmException e) { throw new RuntimeException(e); } catch (NoSuchPaddingException e) { throw new RuntimeException(e); } catch (InvalidKeyException e) { throw new RuntimeException(e); } catch (IllegalBlockSizeException e) { throw new RuntimeException(e); } catch (BadPaddingException e) { throw new RuntimeException(e); } catch (UnsupportedEncodingException e) { throw new RuntimeException(e); } } /** * 传入字符串、密钥、加密方式,并解密字符串(对称加密解密密),支持:DES、AES、DESede(3DES) * <br>生成时间:2014年5月2日 下午1:12:13 * <br>返回值:String 密钥原文 * <br>@param sec * <br>@param key * <br>@param method(DES、AES、DESede) * <br>@return */ public static String doubKeyDencoding(String sec,String keysrc,String method) { SecretKey key; try { //生成密钥 KeyGenerator kg = KeyGenerator.getInstance(method); //初始化此密钥生成器。 kg.init(new SecureRandom(keysrc.getBytes("utf-8"))); key = kg.generateKey(); //加密 Cipher ciph = Cipher.getInstance(method); ciph.init(ciph.DECRYPT_MODE, key); //使用64进行解码,一避免出现丢数据情景 byte[] by = BASE64DecoderStream.decode(sec.getBytes()); ciph.update(by); return new String(ciph.doFinal()); } catch (NoSuchAlgorithmException e) { throw new RuntimeException(e); } catch (NoSuchPaddingException e) { throw new RuntimeException(e); } catch (InvalidKeyException e) { throw new RuntimeException(e); } catch (IllegalBlockSizeException e) { throw new RuntimeException(e); } catch (BadPaddingException e) { throw new RuntimeException(e); } catch (UnsupportedEncodingException e) { throw new RuntimeException(e); } } /** * 单向信息加密(信息摘要),支持:md5、md2、SHA(SHA-1,SHA1)、SHA-256、SHA-384、SHA-512, * <br>返回值:String 加密后的密文 * <br>@param src 传入加密字符串(明文) * <br>@param method 指定算法(md5、md2、SHA(SHA-1,SHA1)、SHA-256、SHA-384、SHA-512) * <br>@return */ public static String ecodingPasswd(String src,String method){ try { //信息摘要算法 MessageDigest md5 = MessageDigest.getInstance(method); md5.update(src.getBytes()); byte[] encoding = md5.digest(); //使用64进行编码,一避免出现丢数据情景 return new String(BASE64EncoderStream.encode(encoding)); } catch (NoSuchAlgorithmException e) { throw new RuntimeException(e+"加密失败!!"); } } }
For more java encryption algorithm sharing (rsa decryption, symmetric encryption, md5 encryption) related articles, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


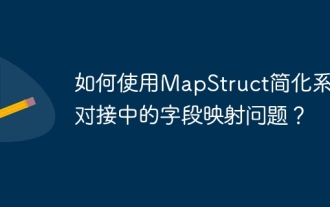
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
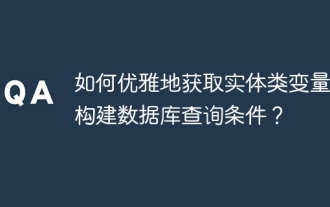
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
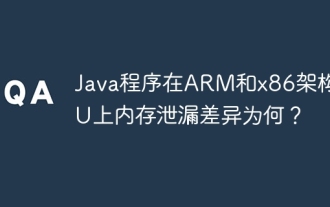
Analysis of memory leak phenomenon of Java programs on different architecture CPUs. This article will discuss a case where a Java program exhibits different memory behaviors on ARM and x86 architecture CPUs...
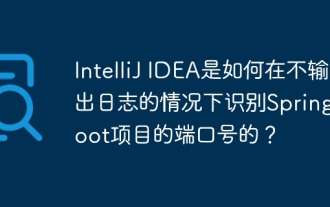
Start Spring using IntelliJIDEAUltimate version...
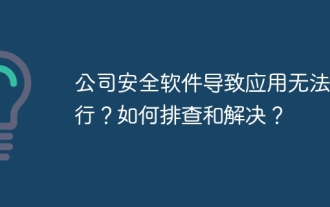
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
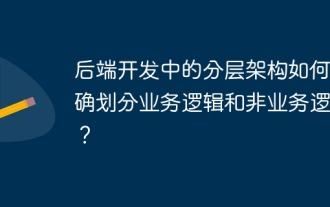
Discussing the hierarchical architecture problem in back-end development. In back-end development, common hierarchical architectures include controller, service and dao...
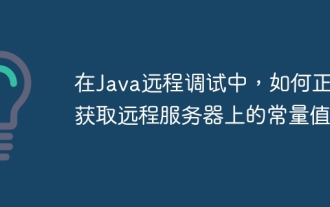
Questions and Answers about constant acquisition in Java Remote Debugging When using Java for remote debugging, many developers may encounter some difficult phenomena. It...
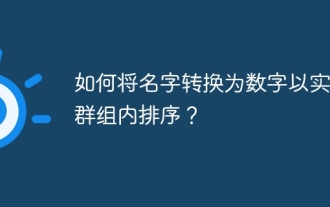
How to convert names to numbers to implement sorting within groups? When sorting users in groups, it is often necessary to convert the user's name into numbers so that it can be different...
