


Detailed explanation of Shell arrays and associative arrays and example codes
Shell arrays and associative arrays
1. Array
1.1. Array definition
A pair of parentheses represents an array, and the array elements are separated by space symbols
1 2 3 4 5 6 |
|
1.2. Array length, elements, assignment and deletion
Length: Use ${#array name[@or*]} to get the array length
1 2 3 4 |
|
Get elements: Use ${array name[subscript]} to get the array elements (the subscript starts from 0), and the subscript is * or @ to get the entire array content
1 2 3 4 5 6 7 8 9 |
|
Assignment: Reference assignment can be made through the array name [subscript]. If the subscript does not exist, a new array element will be automatically added
1 2 3 4 5 6 |
|
Delete: through unset Array [subscript] can clear the corresponding elements. Without subscript, all data will be cleared.
1 2 3 4 5 6 7 |
|
1.3. Get elements in a certain range
Directly through ${array Name [@ or *]: starting position: length} Get the elements in the given range of the array and return a string, separated by spaces
1 2 3 4 5 6 7 |
|
1.4. Replace
${array name[@ or *]/search character/replace character} This operation will not change the original array content. If you need to modify it, you can see the above example
1 2 3 4 5 |
|
2. Association Array
Bash supports associative arrays, which can use strings as array indexes. Sometimes it is easier to understand using string indexes.
2.1 Define associative array
First you need to use a declaration statement to declare a variable as an associative array.
1 |
|
After declaration, there are two ways to add elements to an associative array.
(1) Method of using embedded index-value list
1 2 3 |
|
(2) Using independent index-value for assignment
1 2 3 4 5 6 |
|
2.2 List array index
Each array has an index for search. Use ${!array name[@or*]} to get the index list of the array
1 2 3 4 |
|
2.3 Get all key-value pairs
1 2 3 4 5 6 7 |
|
Result:
1 2 3 4 |
|
Thank you for reading, I hope it can help everyone, thank you everyone for your support of this site!
For more detailed explanations and example codes of Shell arrays and associative arrays, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


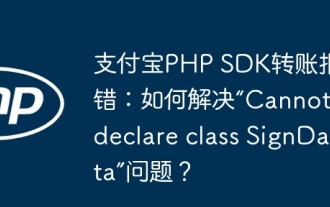
Alipay PHP...
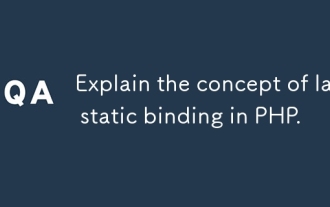
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
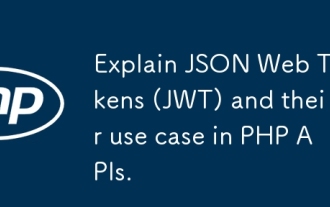
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
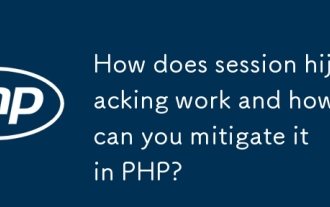
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
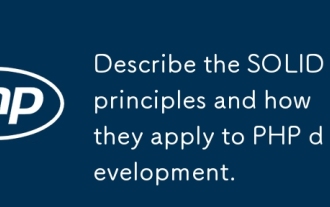
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
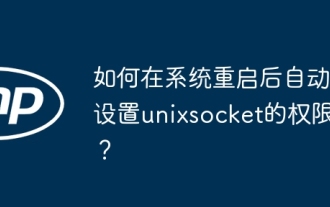
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
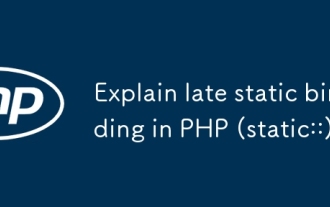
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
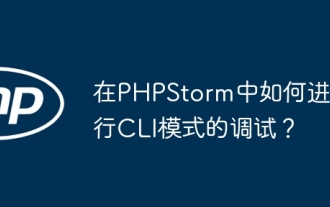
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
