


C# basic knowledge compilation: basic knowledge (10) static
If you want to access the methods or properties of a certain class, you must first instantiate the class, and then use the object of the class plus the . sign to access it. For example:
There is a user class and a class that handles passwords (encryption and decryption). After a user instance is not generated, the password class needs to encrypt and decrypt the password.
using System; using System.Collections.Generic; using System.Text; using System.Security.Cryptography; using System.IO; namespace YYS.CSharpStudy.MainConsole.Static { /// <summary> /// 用户类 /// </summary> public class User { //加密解密用到的Key private string key = "20120719"; //加密解密用到的向量 private string ivalue = "12345678"; private string userName; private string userEncryptPassword; private string userDecryptPassword; /// <summary> /// 用户名 /// </summary> public string UserName { get { return userName; } } /// <summary> /// 用户密码,加密后的密码 /// </summary> public string UserEncryptPassword { get { return userEncryptPassword; } } /// <summary> /// 用户密码,解密后的密码 /// </summary> public string UserDecryptPassword { get { DES des = new DES(); this.userDecryptPassword = des.Decrypt(userEncryptPassword, key, ivalue); return userDecryptPassword; } } /// <summary> /// 构造函数 /// </summary> /// <param name="userName"></param> /// <param name="userPassword"></param> public User(string userName, string userPassword) { this.userName = userName; DES des = new DES(); this.userEncryptPassword = des.Encrypt(userPassword, key, ivalue); } } /// <summary> /// 处理密码的类 /// </summary> public class DES { /// <summary> /// 加密字符串 /// </summary> public string Encrypt(string sourceString, string key, string iv) { try { byte[] btKey = Encoding.UTF8.GetBytes(key); byte[] btIV = Encoding.UTF8.GetBytes(iv); DESCryptoServiceProvider des = new DESCryptoServiceProvider(); using (MemoryStream ms = new MemoryStream()) { byte[] inData = Encoding.UTF8.GetBytes(sourceString); try { using (CryptoStream cs = new CryptoStream(ms, des.CreateEncryptor(btKey, btIV), CryptoStreamMode.Write)) { cs.Write(inData, 0, inData.Length); cs.FlushFinalBlock(); } return Convert.ToBase64String(ms.ToArray()); } catch { return sourceString; } } } catch { } return sourceString; } /// <summary> /// 解密字符串 /// </summary> public string Decrypt(string encryptedString, string key, string iv) { byte[] btKey = Encoding.UTF8.GetBytes(key); byte[] btIV = Encoding.UTF8.GetBytes(iv); DESCryptoServiceProvider des = new DESCryptoServiceProvider(); using (MemoryStream ms = new MemoryStream()) { byte[] inData = Convert.FromBase64String(encryptedString); try { using (CryptoStream cs = new CryptoStream(ms, des.CreateDecryptor(btKey, btIV), CryptoStreamMode.Write)) { cs.Write(inData, 0, inData.Length); cs.FlushFinalBlock(); } return Encoding.UTF8.GetString(ms.ToArray()); } catch { return encryptedString; } } } } }
Call:
class Program { static void Main(string[] args) { User user = new User("yangyoushan", "000000"); Console.WriteLine(string.Format("用户名:{0}", user.UserName)); Console.WriteLine(string.Format("加密后的密码:{0}", user.UserEncryptPassword)); Console.WriteLine(string.Format("明文的密码:{0}", user.UserDecryptPassword)); Console.ReadKey(); } }
Result:
There are two problems in the code implemented by these two classes.
1. For every user instantiated,
DES des = new DES(); this.userEncryptPassword = des.Encrypt(userPassword, key, ivalue);
must be run, that is, a DES instance must be instantiated every time. This is not good. DES is instantiated just to call its methods, but it is inconvenient to instantiate it every time a method is called, and it also increases memory consumption.
2. For
//加密解密用到的Key private string key = "20120719"; //加密解密用到的向量 private string ivalue = "12345678";
these two variables are used by every user instance and will not change. However, space must be allocated every time a user is instantiated, which also consumes memory, and is not very reasonable in terms of object-oriented thinking.
In this case, it is best to share the two methods of DES and call them directly without instantiation. For example, all methods of Math (Math.Abs(1);). Another is to set the key and ivalue variables in User to public, and they can also be accessed directly, and the memory space is only allocated once, and there is no need to allocate it separately when instantiating the user.
This requires the use of static, that is, the static keyword. The so-called static means that members are shared by a class. In other words, members declared as static do not belong to objects of a specific class, but belong to all objects of this class. All members of a class can be declared static, and can declare static fields, static properties, or static methods. But here we need to distinguish between const and static. Const means that the value of a constant cannot be changed during the running of the program, while static can change the value during running. If it is changed in one place, the changed value will be accessed in other places.
In this way, you can use static to optimize the above code, as follows:
using System; using System.Collections.Generic; using System.Text; using System.Security.Cryptography; using System.IO; namespace YYS.CSharpStudy.MainConsole.Static { /// <summary> /// 用户类 /// </summary> public class User { //加密解密用到的Key private static string key = "20120719"; //加密解密用到的向量 private static string ivalue = "12345678"; private string userName; private string userEncryptPassword; private string userDecryptPassword; /// <summary> /// 用户名 /// </summary> public string UserName { get { return userName; } } /// <summary> /// 用户密码,加密后的密码 /// </summary> public string UserEncryptPassword { get { return userEncryptPassword; } } /// <summary> /// 用户密码,解密后的密码 /// </summary> public string UserDecryptPassword { get { //使用静态方法和静态字段 this.userDecryptPassword = DES.Decrypt(userEncryptPassword, key, ivalue); return userDecryptPassword; } } /// <summary> /// 构造函数 /// </summary> /// <param name="userName"></param> /// <param name="userPassword"></param> public User(string userName, string userPassword) { this.userName = userName; this.userEncryptPassword = DES.Encrypt(userPassword, key, ivalue); } } /// <summary> /// 处理密码的类 /// </summary> public class DES { /// <summary> /// 加密字符串 /// </summary> public static string Encrypt(string sourceString, string key, string iv) { try { byte[] btKey = Encoding.UTF8.GetBytes(key); byte[] btIV = Encoding.UTF8.GetBytes(iv); DESCryptoServiceProvider des = new DESCryptoServiceProvider(); using (MemoryStream ms = new MemoryStream()) { byte[] inData = Encoding.UTF8.GetBytes(sourceString); try { using (CryptoStream cs = new CryptoStream(ms, des.CreateEncryptor(btKey, btIV), CryptoStreamMode.Write)) { cs.Write(inData, 0, inData.Length); cs.FlushFinalBlock(); } return Convert.ToBase64String(ms.ToArray()); } catch { return sourceString; } } } catch { } return sourceString; } /// <summary> /// 解密字符串 /// </summary> public static string Decrypt(string encryptedString, string key, string iv) { byte[] btKey = Encoding.UTF8.GetBytes(key); byte[] btIV = Encoding.UTF8.GetBytes(iv); DESCryptoServiceProvider des = new DESCryptoServiceProvider(); using (MemoryStream ms = new MemoryStream()) { byte[] inData = Convert.FromBase64String(encryptedString); try { using (CryptoStream cs = new CryptoStream(ms, des.CreateDecryptor(btKey, btIV), CryptoStreamMode.Write)) { cs.Write(inData, 0, inData.Length); cs.FlushFinalBlock(); } return Encoding.UTF8.GetString(ms.ToArray()); } catch { return encryptedString; } } } } }
Running results:
But there is one problem to note, the general method Static properties or static methods can be accessed outside the method. But if you want to access properties or methods outside the method in a static method, the properties and methods being accessed must also be static. Because general attributes or methods can only be used after allocating space after instantiation, while statically allocates memory space directly during compilation, so it is not possible to call other attributes or methods in static methods. , only static ones can be called at the same time.
The above is the compilation of C# basic knowledge: basic knowledge (10) static content. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


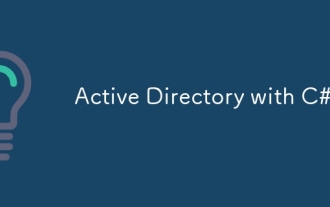
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
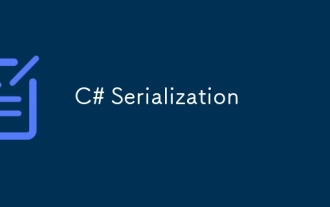
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
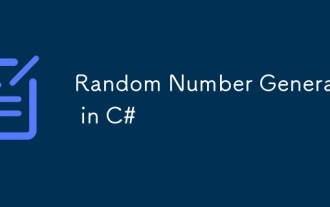
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
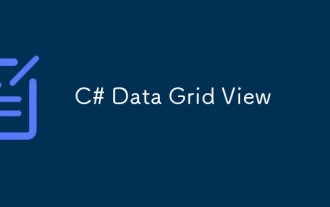
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
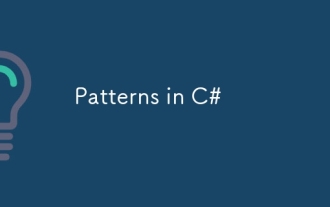
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
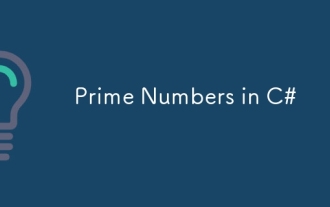
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
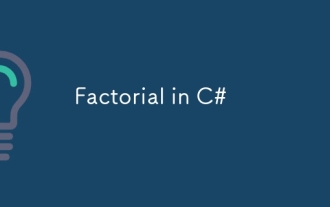
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
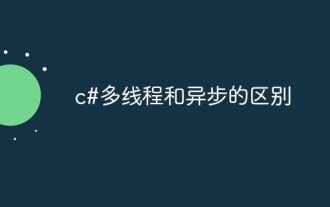
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
