Java thread pool framework
This article mainly introduces the relevant knowledge of Java thread pool framework. It has a very good reference value. Let’s take a look at it with the editor
1. Thread pool structure diagram
2. Example
Define thread interface
public class MyThread extends Thread { @Override publicvoid run() { System.out.println(Thread.currentThread().getName() + "正在执行"); } }
1: newSingleThreadExecutor
ExecutorService pool = Executors. newSingleThreadExecutor(); Thread t1 = new MyThread(); Thread t2 = new MyThread(); Thread t3 = new MyThread(); //将线程放入池中进行执行 pool.execute(t1); pool.execute(t2); pool.execute(t3); //关闭线程池 pool.shutdown();
Input result:
pool-1-thread-1正在执行 pool-1-thread-1正在执行 pool-1-thread-1正在执行
2: newFixedThreadPool
ExecutorService pool = Executors.newFixedThreadPool(3); Thread t1 = new MyThread(); Thread t2 = new MyThread(); Thread t3 = new MyThread(); Thread t4 = new MyThread(); Thread t5 = new MyThread(); //将线程放入池中进行执行 pool.execute(t1); pool.execute(t2); pool.execute(t3); pool.execute(t4); pool.execute(t5); pool.shutdown();
Input result:
pool-1-thread-1正在执行 pool-1-thread-2正在执行 pool-1-thread-1正在执行 pool-1-thread-2正在执行
3 :newCachedThreadPool
ExecutorService pool = Executors.newCachedThreadPool(); Thread t1 = new MyThread(); Thread t2 = new MyThread(); Thread t3 = new MyThread(); Thread t4 = new MyThread(); Thread t5 = new MyThread(); //将线程放入池中进行执行 pool.execute(t1); pool.execute(t2); pool.execute(t3); pool.execute(t4); pool.execute(t5); //关闭线程池 pool.shutdown();
Input result:
pool-1-thread-2正在执行 pool-1-thread-4正在执行 pool-1-thread-3正在执行 pool-1-thread-1正在执行 pool-1-thread-5正在执行
4 :ScheduledThreadPoolExecutor
##
ScheduledExecutorService pool = Executors.newScheduledThreadPool(2); pool.scheduleAtFixedRate(new Runnable() {//每隔一段时间就触发异常 @Override public void run() { //throw new RuntimeException(); System.out.println("================"); } }, 1000, 2000, TimeUnit.MILLISECONDS); pool.scheduleAtFixedRate(new Runnable() {//每隔一段时间打印系统时间,证明两者是互不影响的 @Override public void run() { System.out.println("+++++++++++++++++"); } }, 1000, 2000, TimeUnit.MILLISECONDS);
================ +++++++++++++++++ +++++++++++++++++ +++++++++++++++++
3. Thread pool core parameters
corePoolSize: The number of core threads in the poolmaximumPoolSize: The maximum thread allowed in the pool number. keepAliveTime: When the number of threads is greater than the core, this is the maximum time for excess idle threads to wait for new tasks before terminating. unit: The time unit of the keepAliveTime parameter. workQueue: Queue used to hold tasks before execution. This queue only holds Runnable tasks submitted by the execute method. threadFactory: Factory used by the executor to create new threads. handler: The handler used when execution is blocked due to exceeding the thread scope and queue capacity. ThreadPoolExecutor: The underlying implementation of the Executors class.3.1 Task queuing mechanism
SynchonousQueue: Synchronous queue, the queue is directly submitted to thread execution without maintaining them. At this time, the thread pool is usually UnboundedLinkedBlockingQueue: Unbounded queue. When the number of thread pool threads reaches the maximum number, new tasks will wait in the queue for execution, which may cause the queue to expand infinitelyArrayBlockingQueue: Bounded Queue helps prevent resource exhaustion. Once the upper limit is reached, new tasks may be lostNote:
newSingleThreadExecutor, newFixedThreadPool use is LinkedBlockingQueuenewCachedThreadPool uses SynchonousQueuenewScheduledThreadPool uses DelayedWorkQueue3.2 Thread execution process
3.3 Thread size determination:
cpu-intensive: Open as few threads as possible, the optimal number of threads is Ncpu+ 1io-intensive: multiple threads, 2NcpuMixed: depending on the situation, it can be split into io-intensive and cou-intensiveMore Java thread pools For framework-related articles, please pay attention to the PHP Chinese website!
Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
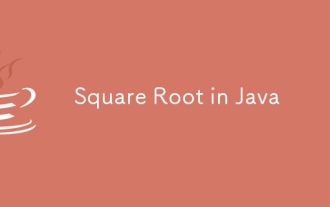
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
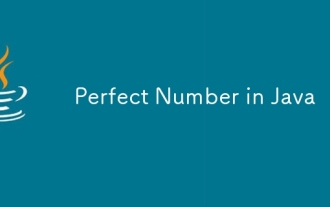
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
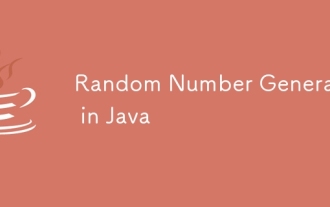
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
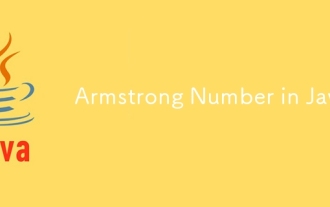
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
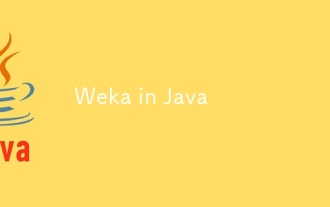
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
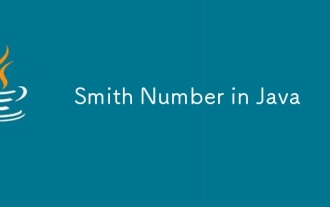
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
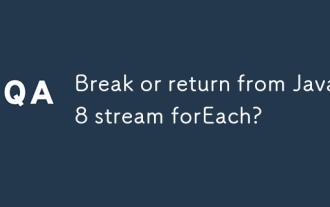
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
