python sorting sort() and sorted()
Application examples:
1. Output a sequence according to the alphabet
2. Sort multiple fields of records, etc.
Commonly used sorting functions:
sort()
sorted()
Comparison:
1.sorted() has a wider application range
sorted(iterable[, cmp[ , key[, reverse]]])
s.sorted([cmp[, key[, reverse]]])
Example:
>>> ; persons = [{'name':'Jon','age': 32}, {'name':'Alan','age': 50}, {'name': 'Bob', 'age':23 }]
>>> sorted(persons, key=lambda x: (x['name'], -x['age']))
[{'age': 50, 'name ': 'Alan'}, {'age': 23, 'name': 'Bob'}, {'age': 32, 'name': 'Jon'}]
sorted() can be used Any iterable object, sort() generally works on lists
>>> a = (1,2,4,2,3)
>>> a.sort( )
Traceback (most recent call last):
File "
AttributeError: 'tuple' object has no attribute 'sort'
> ;>> sorted(a)
[1, 2, 2, 3, 4]
2.sorted() returns the sorted list, and the original list remains unchanged , sort() directly modifies the original list.
sort() does not need to copy the original list, consumes less memory and is highly efficient
>>> a=['1',1,'a',3, 7,'n']
>>> sorted(a)
[1, 3, 7, '1', 'a', 'n']
>>> a
['1', 1, 'a', 3, 7, 'n']
>>> a.sort()
>>> a
[1 , 3, 7, '1', 'a', 'n']
3. For the sort() and sorted() functions, passing in the parameter key is more efficient than the parameter cmp . The function passed in by cmp is called multiple times during the entire sorting process, which is expensive; key is only processed once for each element.
>>> from timeit import Timer
>>> Timer(stmt="sorted(xs,key=lambda x:x[1])",setup="xs =range(100);xs=zip(xs,xs);").timeit(10000)
0.35391712188720703
>>> : cmp(a[1],b[1]))",setup="xs=range(100);xs=zip(xs,xs);").timeit(10000)
0.4931659698486328
4.sorted() can sort a variety of data structures
Dictionary:
Sort phonebook phone numbers by numerical size
> ;>> phonebook = {'Linda':'7750','Bob':'9345','Carol':'5834'}
>>> from operator import itemgetter
> >> sorted_pb = sorted(phonebook.iteritems(),key=itemgetter(1))
>>> sorted_pb
[('Carol', '5834'), ('Linda', '7750'), ('Bob', '9345')]
Multidimensional list:
Multi-field sorting of grades and grades
>>> from operator import itemgetter
>>> gameresult = [['Bob',95.00,'A'],['Alan',86.0,'C'],['Mandy',82.5,'A'] ,['Rob',86,'E']]
>>> sorted(gameresult, key=itemgetter(2, 1))
[['Mandy', 82.5, 'A'] , ['Bob', 95.0, 'A'], ['Alan', 86.0, 'C'], ['Rob', 86, 'E']]
Mixed list in dictionary:
>>> mydict = {'Li':['M',7],
... 'Zhang': ['E',2],
... ' Wang':['p',3],
... 'Du':['C',2]}
>>> from operator import itemgetter
>>> ; sorted(mydict.iteritems(),key=lambda(k,v):operator.itemgetter(1)(v))
[('Zhang', ['E', 2]), ('Du' , ['C', 2]), ('Wang', ['p', 3]), ('Li', ['M', 7])]
Mixed dictionary in List:
Sort multiple key values rating and name
>>> gameresult = [{"name":"Bob","wins":10, "losses":3,"rating":75.00},
... {"name":"David","wins":3,"loses":5,"rating":57.00}]
>>> from operator import itemgetter
>>> sorted(gameresult,key=itemgetter("rating","name"))
[{'wins': 3, 'rating' : 57.0, 'name': 'David', 'loses': 5}, {'wins': 10, 'losses': 3, 'name': 'Bob', 'rating': 75.0}]
> ;>>
For more python sorting sort() and sorted() related articles, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


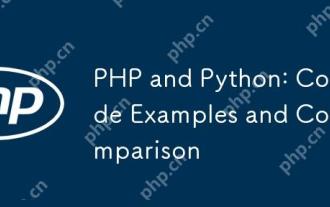
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
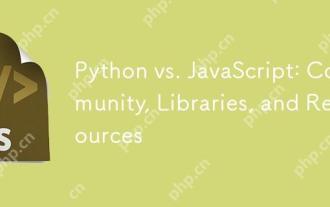
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
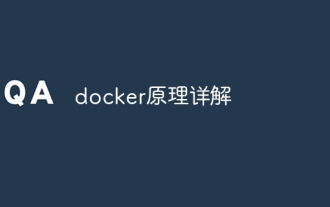
Docker uses Linux kernel features to provide an efficient and isolated application running environment. Its working principle is as follows: 1. The mirror is used as a read-only template, which contains everything you need to run the application; 2. The Union File System (UnionFS) stacks multiple file systems, only storing the differences, saving space and speeding up; 3. The daemon manages the mirrors and containers, and the client uses them for interaction; 4. Namespaces and cgroups implement container isolation and resource limitations; 5. Multiple network modes support container interconnection. Only by understanding these core concepts can you better utilize Docker.
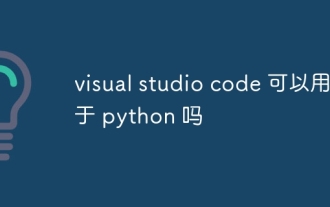
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
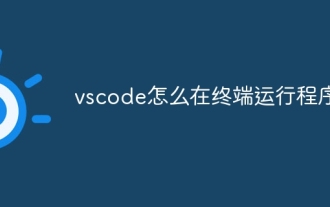
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
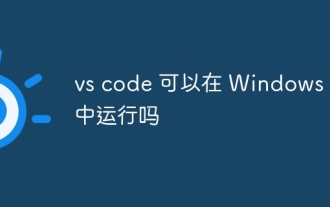
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
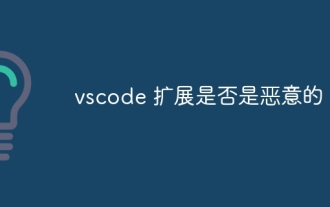
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
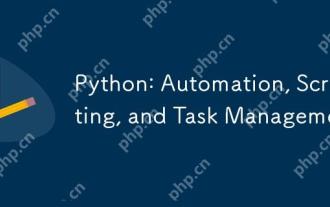
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
