Use of Python attributes and descriptors
About the @property decorator
In Python we use the @property decorator to disguise calls to functions as access to properties.
So why do this? Because @property allows us to tie custom code to variable access/setting, while maintaining a simple interface for accessing properties for your class.
For example, if we have a class that needs to represent a movie:
class Movie(object): def __init__(self, title, description, score, ticket): self.title = title self.description = description self.score = scroe self.ticket = ticket
You start using this class in other places in the project, But then you realize: What if you accidentally give a movie a negative score? You think this is wrong behavior and hope that the Movie class can prevent this error. The first thing you think of is to modify the Movie class to look like this:
class Movie(object): def __init__(self, title, description, score, ticket): self.title = title self.description = description self.ticket = ticket if score < 0: raise ValueError("Negative value not allowed:{}".format(score)) self.score = scroe
But that won’t work. Because other parts of the code are assigned directly through Movie.score. This newly modified class will only capture erroneous data in the __init__ method, but it will not be able to do anything about existing class instances. If someone tries to run m.scrore= -100, there's nothing you can do to stop it. so what should I do now?
Python's property solves this problem.
We can do this
class Movie(object): def __init__(self, title, description, score): self.title = title self.description = description self.score = score self.ticket = ticket @property def score(self): return self.__score @score.setter def score(self, score): if score < 0: raise ValueError("Negative value not allowed:{}".format(score)) self.__score = score @score.deleter def score(self): raise AttributeError("Can not delete score")
In this way, modifying the score anywhere will detect whether it is less than 0.
Disadvantages of property
The biggest disadvantage of properties is that they cannot be reused. For example, let's say you want to add a non-negative check to the ticket field as well.
The following is the modified new class:
class Movie(object): def __init__(self, title, description, score, ticket): self.title = title self.description = description self.score = score self.ticket = ticket @property def score(self): return self.__score @score.setter def score(self, score): if score < 0: raise ValueError("Negative value not allowed:{}".format(score)) self.__score = score @score.deleter def score(self): raise AttributeError("Can not delete score") @property def ticket(self): return self.__ticket @ticket.setter def ticket(self, ticket): if ticket < 0: raise ValueError("Negative value not allowed:{}".format(ticket)) self.__ticket = ticket @ticket.deleter def ticket(self): raise AttributeError("Can not delete ticket")
You can see that the code has increased a lot, but there are also many repeated logics. few. Although property can make the interface of a class look neat and beautiful from the outside, it cannot be as neat and beautiful from the inside.
Descriptor appears
What is a descriptor?
Generally speaking, a descriptor is an object property with binding behavior, and access to its properties is overridden by the descriptor protocol method. These methods are __get__(), __set__() and __delete__(). As long as an object contains at least one of these three methods, it is called a descriptor.
What does the descriptor do?
The default behavior for attribute access is to get, set, or delete the attribute from an object's dictionary. For instance, a.x has a lookup chain starting with a.__dict__['x'], then type(a) .__dict__['x'], and continuing through the base classes of type(a) excluding metaclasses. If the looked-up value is an object defining one of the descriptor methods, then Python may override the default behavior and invoke the descriptor method instead. Where this occurs in the precedence chain depends on which descriptor methods were defined. —–Excerpted from the official documentation
Simply put, descriptors will change the basic way of getting, setting, and deleting an attribute.
Let’s first look at how to use descriptors to solve the problem of repeated property logic above.
class Integer(object): def __init__(self, name): self.name = name def __get__(self, instance, owner): return instance.__dict__[self.name] def __set__(self, instance, value): if value < 0: raise ValueError("Negative value not allowed") instance.__dict__[self.name] = value class Movie(object): score = Integer('score') ticket = Integer('ticket')
Because the descriptor has a high priority and will change the default get and set behavior, in this way, when we access or set Movie().score It is always limited by the descriptor Integer.
But we can’t always create instances in the following way.
a = Movie() a.score = 1 a.ticket = 2 a.title = ‘test' a.descript = ‘…'
This is too blunt, so we still lack a constructor.
class Integer(object): def __init__(self, name): self.name = name def __get__(self, instance, owner): if instance is None: return self return instance.__dict__[self.name] def __set__(self, instance, value): if value < 0: raise ValueError('Negative value not allowed') instance.__dict__[self.name] = value class Movie(object): score = Integer('score') ticket = Integer('ticket') def __init__(self, title, description, score, ticket): self.title = title self.description = description self.score = score self.ticket = ticket
In this way, the __get__ and __set__ of Integer will be entered when obtaining, setting and deleting score and ticket, thereby reducing repeated logic.
Now that the problem has been solved, you may be curious about how this descriptor works. Specifically, what is accessed in the __init__ function is its own self.score and self.ticket. How is it related to the class attributes score and ticket?
How descriptors work
See the official description
If an object defines both __get__() and __set__(), it is considered a data descriptor. Descriptors that only define __get__() are called non-data descriptors (they are typically used for methods but other uses are possible).
Data and non-data descriptors differ in how overrides are calculated with respect to entries in an instance's dictionary. If an instance's dictionary has an entry with the same name as a data descriptor, the data descriptor takes precedence. If an instance's dictionary has an entry with the same name as a non-data descriptor, the dictionary entry takes precedence.
The important points to remember are:
descriptors are invoked by the __getattribute__() method
overriding __getattribute__() prevents automatic descriptor calls
object.__getattribute__() and type.__getattribute__() make different calls to __get__().
data descriptors always override instance dictionaries.
non-data descriptors may be overridden by instance dictionaries.
When a class calls __getattribute__() it is probably It looks like this:
def __getattribute__(self, key): "Emulate type_getattro() in Objects/typeobject.c" v = object.__getattribute__(self, key) if hasattr(v, '__get__'): return v.__get__(None, self) return v
The following is excerpted from a foreign blog.
Given a Class “C” and an Instance “c” where “c = C(…)”, calling “c.name” means looking up an Attribute “name” on the Instance “c” like this :
Get the Class from Instance
Call the Class's special method getattribute__. All objects have a default __getattribute
Inside getattribute
Get the Class's mro as ClassParents
For each ClassParent in ClassParents
If the Attribute is in the ClassParent's dict
If is a data descriptor
Return the result from calling the data descriptor's special method __get__()
Break the for each (do not continue searching the same Attribute any further)
If the Attribute is in Instance's dict
Return the value as it is (even if the value is a data descriptor)
For each ClassParent in ClassParents
If the Attribute is in the ClassParent's dict
If is a non-data descriptor
Return the result from calling the non-data descriptor's special method __get__()
If it is NOT a descriptor
Return the value
If Class has the special method getattr
Return the result from calling the Class's special method__getattr__.
我对上面的理解是,访问一个实例的属性的时候是先遍历它和它的父类,寻找它们的__dict__里是否有同名的data descriptor如果有,就用这个data descriptor代理该属性,如果没有再寻找该实例自身的__dict__ ,如果有就返回。任然没有再查找它和它父类里的non-data descriptor,最后查找是否有__getattr__
描述符的应用场景
python的property、classmethod修饰器本身也是一个描述符,甚至普通的函数也是描述符(non-data discriptor)
django model和SQLAlchemy里也有描述符的应用
class User(db.Model): id = db.Column(db.Integer, primary_key=True) username = db.Column(db.String(80), unique=True) email = db.Column(db.String(120), unique=True) def __init__(self, username, email): self.username = username self.email = email def __repr__(self): return '<User %r>' % self.username
总结
只有当确实需要在访问属性的时候完成一些额外的处理任务时,才应该使用property。不然代码反而会变得更加啰嗦,而且这样会让程序变慢很多。以上就是本文的全部内容,由于个人能力有限,文中如有笔误、逻辑错误甚至概念性错误,还请提出并指正。
更多Python属性和描述符的使用相关文章请关注PHP中文网!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


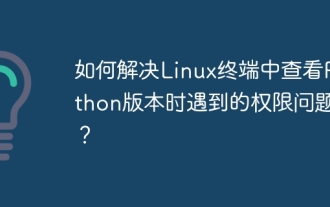
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
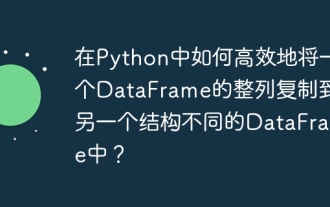
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
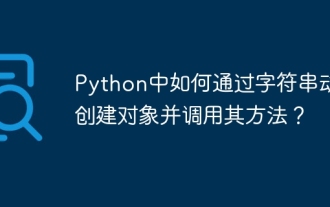
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
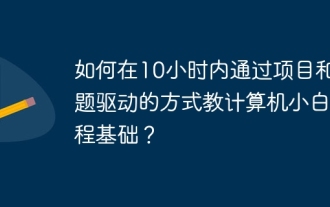
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
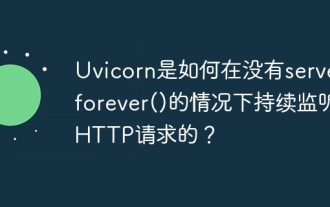
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
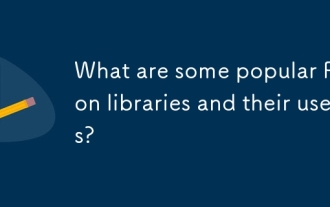
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
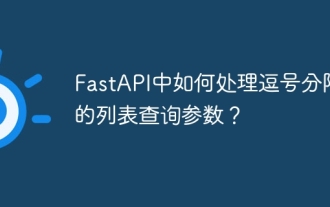
Fastapi ...
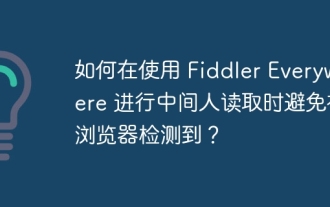
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
