


Three ways to get table field names and field information in php mysql
php mysql three methods to obtain table field names and field information
First give the information about the table used in this example:
Use desc to obtain table field information
The php code is as follows:
<?php mysql_connect("localhost","root",""); mysql_select_db("test"); $query = "desc student"; $result = mysql_query($query); while($row=mysql_fetch_assoc($result)){ print_r($row); } ?>
Running results:
Array ( [Field] => student_id [Type] => int(4) [Null] => NO [Key] => PRI [Default] => [Extra] => auto_increment ) Array ( [Field] => student_name [Type] => varchar(50) [Null] => NO [Key] => [Default] => [Extra] => ) Array ( [Field] => class_id [Type] => int(4) [Null] => NO [Key] => [Default] => [Extra] => ) Array ( [Field] => total_score [Type] => int(4) [Null] => NO [Key] => [Default] => [Extra] => )
Use SHOW FULL FIELDS to obtain table field information
The php code is as follows:
<?php mysql_connect("localhost","root",""); mysql_select_db("test"); $query = "SHOW FULL COLUMNS FROM student"; $result = mysql_query($query); while($row=mysql_fetch_assoc($result)){ print_r($row); } ?>
Run results:
Array ( [Field] => student_id [Type] => int(4) [Collation] => [Null] => NO [Key] => PRI [Default] => [Extra] => auto_increment [Privileges] => select,insert,update,references [Comment] => ) Array ( [Field] => student_name [Type] => varchar(50) [Collation] => latin1_swedish_ci [Null] => NO [Key] => [Default] => [Extra] => [Privileges] => select,insert,update,references [Comment] => ) Array ( [Field] => class_id [Type] => int(4) [Collation] => [Null] => NO [Key] => [Default] => [Extra] => [Privileges] => select,insert,update,references [Comment] => ) Array ( [Field] => total_score [Type] => int(4) [Collation] => [Null] => NO [Key] => [Default] => [Extra] => [Privileges] => select,insert,update,references [Comment] => )
Use the mysql_fetch_field method to obtain table field information
php code is as follows:
<?php mysql_connect("localhost","root",""); mysql_select_db("test"); $query = "SELECT * FROM student LIMIT 1"; $result = mysql_query($query); $fields = mysql_num_fields($result); for($count=0;$count<$fields;$count++) { $field = mysql_fetch_field($result,$count); print_r($field); } ?>
The running results are as follows:
stdClass Object ( [name] => student_id [table] => student [def] => [max_length] => 1 [not_null] => 1 [primary_key] => 1 [multiple_key] => 0 [unique_key] => 0 [numeric] => 1 [blob] => 0 [type] => int [unsigned] => 0 [zerofill] => 0 ) stdClass Object ( [name] => student_name [table] => student [def] => [max_length] => 5 [not_null] => 1 [primary_key] => 0 [multiple_key] => 0 [unique_key] => 0 [numeric] => 0 [blob] => 0 [type] => string [unsigned] => 0 [zerofill] => 0 ) stdClass Object ( [name] => class_id [table] => student [def] => [max_length] => 1 [not_null] => 1 [primary_key] => 0 [multiple_key] => 0 [unique_key] => 0 [numeric] => 1 [blob] => 0 [type] => int [unsigned] => 0 [zerofill] => 0 ) stdClass Object ( [name] => total_score [table] => student [def] => [max_length] => 3 [not_null] => 1 [primary_key] => 0 [multiple_key] => 0 [unique_key] => 0 [numeric] => 1 [blob] => 0 [type] => int [unsigned] => 0 [zerofill] => 0 )
The above are three ways for php mysql to obtain table field names and field information Method content, please pay attention to the PHP Chinese website (www.php.cn) for more related content!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


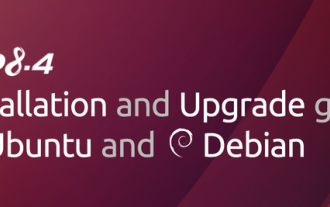
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
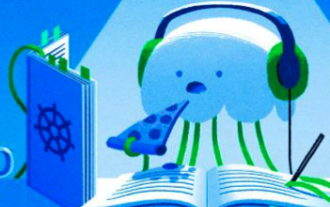
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
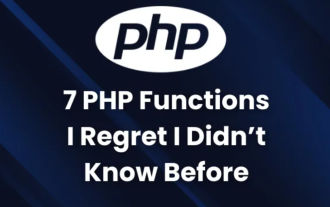
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
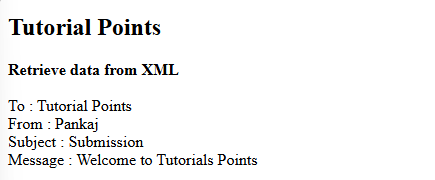
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
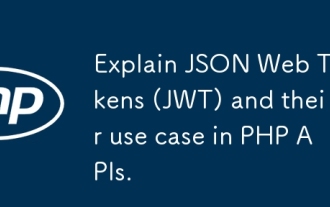
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
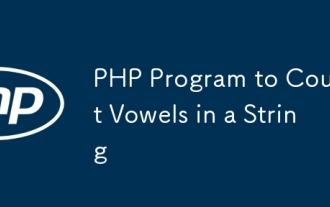
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
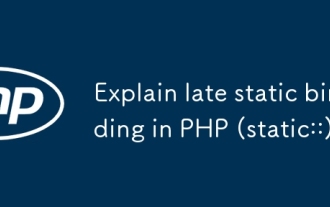
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
