Python deduplicates values in the list structure
I encountered a problem today. I used the itertools.groupby function after a casual prompt from a colleague. However, this thing was not used in the end.
The problem is to deduplicate the news IDs in a list, and ensure that the order remains unchanged after deduplication.
Intuitive method
The simplest idea is:
ids = [1,2,3,3,4,2,3,4,5,6,1] news_ids = [] for id in ids: if id not in news_ids: news_ids.append(id) print news_ids
This is also the case It works, but it doesn't look cool enough.
Use set
Another solution is to use set:
ids = [1,4,3,3,4,2,3,4,5,6,1] ids = list(set(ids))
Sort again by index
Finally solved in this way:
ids = [1,4,3,3,4,2,3,4,5,6,1] news_ids = list(set(ids)) news_ids.sort(ids.index)
Use itertools .grouby
The article mentioned itertools.grouby at the beginning. If you don’t care about the order of the list, you can use this:
##
ids = [1,4,3,3,4,2,3,4,5,6,1] ids.sort() it = itertools.groupby(ids) for k, g in it: print k
About the principle of itertools.groupby, you can see here: http://www.php.cn/
Use reduce
Netizen reatlk left a message for another solution. I add and explain here:
In [5]: ids = [1,4,3,3,4,2,3,4,5,6,1] In [6]: func = lambda x,y:x if y in x else x + [y] In [7]: reduce(func, [[], ] + ids) Out[7]: [1, 4, 3, 2, 5, 6]
The above is the code I run in ipython, in which lambda x, y:x if y in x else x + [y] is equivalent to lambda x,y: y in x and x or x+[y] .
More Python performs operations on values in the list structure For articles related to deduplication, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


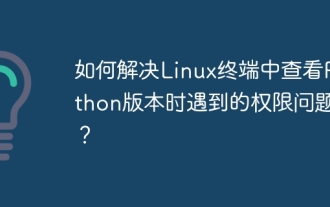
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
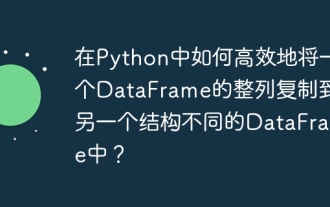
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
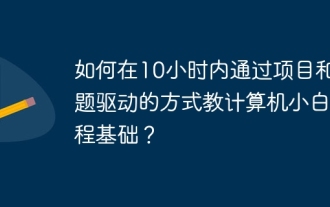
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
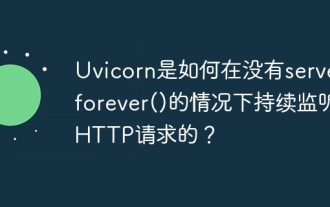
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
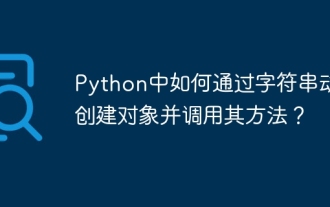
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
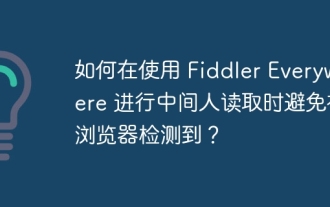
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
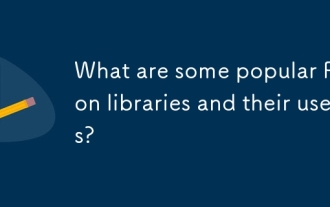
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
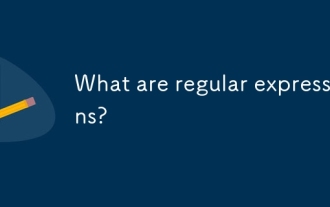
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
