python dict dictionary detailed description
The dictionary is implemented through the principle of hash table. Each element is a key-value pair. A unique hash value is calculated through the key of the element. This hash value determines the address of the element. Therefore, in order to ensure the address of the element No, it must be ensured that the key of each element and the corresponding hash value are completely different, and the key type must be unmodifiable, so the key type can be a numeric value, a string constant or a tuple, but it cannot be a list. Because the list can be modified.
So the dictionary has the following characteristics:
1. The query and insertion operations of elements are very fast, basically at a constant level
2. It takes up a large amount of memory, and uses Method of exchanging space for time
Dictionary initialization
The following methods are all equivalent
d={' a':1, 'b':2, 'c':3}
d=dict({'a':1, 'b':2, 'c':3})
d = dict([('a',1), ('b', 2), ('c', 3)])
d = dict(a=1, b=2 , c=3)
d = dict(zip(['a', 'b', 'c'], [1,2,3]))#This method can also be used to combine two Lists are merged into a dictionary
Assignment elements
1. e = d#Reference assignment, e and d are always the same
2. e = d.copy()# Value assignment, the two are not related
3. d.copy() is a shallow copy, when the value of the key-value pair encounters a dictionary or list , the dictionary or list will also change with the original change. At this time, the value is equivalent to the reference or pointer of the tuple or list, not itself. The tuple or list pointed to is actually the original one. This can be avoided by using the deepcopy() method of the copy module.
import copy dict1 = {'a': [1, 2], 'b': 3} dict2 = dict1 dict3 = dict1.copy() dict4 = copy.deepcopy(dict1) dict1['b'] = 'change'dict1['a'].append('change')print dict1 # {'a': [1, 2, 'change'], 'b': 'change'}print dict2 # {'a': [1, 2, 'change'], 'b': 'change'}print dict3 # {'a': [1, 2, 'change'], 'b': 3}print dict4 # {'a': [1, 2], 'b': 3}
Add element
1, d['d'] = 4# Add directly through the subscript. If the key value already exists, then the element is modified. Of course, you can also access the element
Delete the element
1. d.clear()#Delete all elements in d
2.d.pop('a')#Delete the element with key value 'a'
3.del d ['a']#Delete the element with key value 'a'
Traverse the elements
for k in d:
print 'd[%s]=' % k,d[k]
or
for k,v in d.items():
print ' d[%s]=' % k,v
or
for k,v in d.iteritems():
print 'd[%s]=' % k,v
or
for k,v in d.viewitems():
print 'd[%s]=' % k,v
The difference between items(), iteritems() and viewitems()
items() of python2.x returns a list containing all elements of dict like the above, but because This was too much of a waste of memory, so I later added a set of functions (note: iteritems(), iterkeys(), and itervalues() (which began to appear in Python 2.2) to return an iterator to save memory, but the iterator cannot reflect The change of dict after calling this function. So viewitems() was added, which always represents the latest element. There is only one items function in Python 3.x, which is equivalent to viewitems() in 2.x.
Dictionary merging
1. dd = dict(dict1.items() + dict2.items())
However, this efficiency is not high. According to the above analysis guidance, it actually calls items to first return the corresponding list, then performs list addition, and finally initializes the list into a dictionary.
2, dd = dict(dict1, **dict2)
The keys of the dictionary must be strings. In Python 2 (the interpreter is CPython), we can use non-string keys as keys, but don't be fooled: this hack just happens to work in Python 2 using the standard CPython runtime environment.
The above statement is equivalent to
dd = dict1.copy()
dd.update(dict2)
where dd.update(dict2) is Equivalent to
for k in dict2
dd[k] = dict2[k]
It can be seen that the function of update can not only add non-existing elements, but also modify existing ones. The element value of the key.
And from the above, we know that dictionaries can also be merged through update and for...in.
Sort
dict = { : , : , : , : sorted(dict.items(), key= sorted(dict.items(), key= d: d[1]) ls = list(dict.keys()) ls.sort() for k in ls: print(k, dict[k]) for k in sorted(dict.keys()): print(k, dict[k])
The above is the detailed content of python dict dictionary detailed description. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


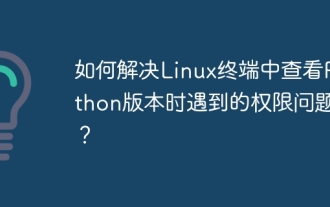
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
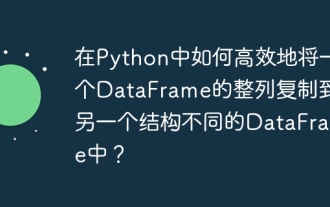
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
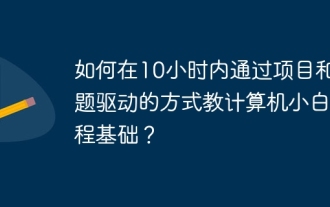
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
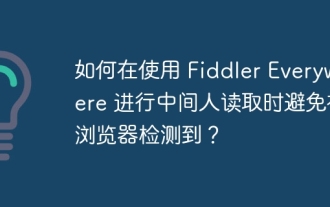
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
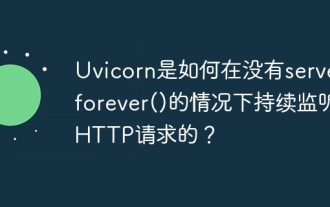
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
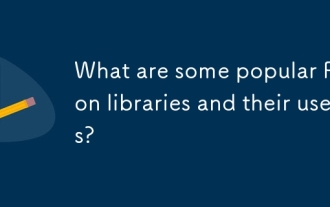
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
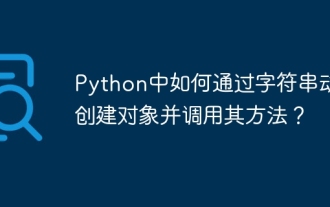
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
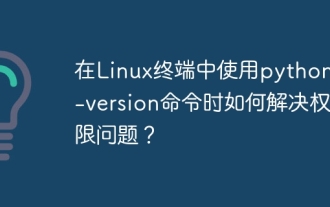
Using python in Linux terminal...
