


Detailed introduction to JavaScript: What exactly does this point to? (pictures and text)
JavaScript is a scripting language that supports advanced features such as functional programming, closures, and prototype-based inheritance. JavaScript seems to be easy to get started with at first, but as you use it more deeply, you will find that JavaScript is actually very difficult to master, and some basic concepts are confusing. Among them, the this keyword in JavaScript is a relatively confusing concept. In different scenarios, this will be transformed into different objects. There is a view that only by correctly mastering the this keyword in JavaScript can you enter the threshold of the JavaScript language. In mainstream object-oriented languages (such as Java, C#, etc.), the meaning of this is clear and specific, that is, it points to the current object. Usually bound at compile time. This in JavaScript is bound at runtime, which is the essential reason why the this keyword in JavaScript has multiple meanings.
Due to the nature of JavaScript binding at runtime, this in JavaScript can be the global object, the current object or any object , which completely depends on how the function is called. There are several ways to call functions in JavaScript: as an object method, as a function, as a constructor, and using apply or call. As the saying goes, words are not as good as words, and expressions are not as good as pictures. In order to better understand what JavaScript this points to? The following is a picture to explain:
I call the above picture "JavaScript this decision tree" (in non-strict mode). The following is an example to illustrate how this diagram can help us judge this:
var point = { x : 0, y : 0, moveTo : function(x, y) { this.x = this.x + x; this.y = this.y + y; } }; //决策树解释:point.moveTo(1,1)函数不是new进行调用,进入否决策, //是用dot(.)进行调用,则指向.moveTo之前的调用对象,即point point.moveTo(1,1); //this 绑定到当前对象,即point对象
point.moveTo() function’s judgment process in "JavaScript this decision tree" is as follows :
1) Is the point.moveTo function call using new? This is obviously not the case. Go to the "No" branch, that is, is the function called with dot(.)? ;
2) The point.moveTo function is called using dot(.), that is, it enters the "Yes" branch, that is, this here points to the previous object point in point.moveTo;
Illustration of what point.moveTo function this points to is shown below:
For another example, look at the following code:
function func(x) { this.x = x; } func(5); //this是全局对象window,x为全局变量 //决策树解析:func()函数是用new进行调用的么?为否,进入func()函数是用dot进行调用的么?为否,则 this指向全局对象window x;//x => 5
func( ) The process of function determination in "JavaScript this decision tree" is as follows:
1) Is the func(5) function call using new? This is obviously not the case. Go to the "No" branch, that is, is the function called with dot(.)? ;
2) The func(5) function is not called with dot(.), that is, it enters the "No" branch, that is, this here points to the global variable window, then this.x is actually window.x ;
The analysis diagram illustrating what this points to in the func function is as shown below:
For the method of direct calling as a function, let’s look at a complicated Example:
var point = { x : 0, y : 0, moveTo : function(x, y) { // 内部函数 var moveX = function(x) { this.x = x;//this 指向什么?window }; // 内部函数 var moveY = function(y) { this.y = y;//this 指向什么?window }; moveX(x); moveY(y); } }; point.moveTo(1,1); point.x; //=>0 point.y; //=>0 x; //=>1 y; //=>1
point.moveTo(1,1) function actually calls the moveX() and moveY() functions internally. The this inside the moveX() function is in "JavaScript this decision tree## The judgment process in #" is as follows:
1) Is the moveX(1) function call using new? This is obviously not the case. Go to the "No" branch, that is, is the function called with dot(.)? ;2) The moveX(1) function is not called with dot(.), that is, it enters the "No" branch, that is, this here points to the global variable window, then this.x is actually window.x ;Let’s take a look at an example of calling as a constructor:function Point(x,y){ this.x = x; // this ? this.y = y; // this ? } var np=new Point(1,1); np.x;//1 var p=Point(2,2); p.x;//error, p是一个空对象undefined window.x;//2
The process of judgment in JavaScript this decision tree" is as follows:
1) Is the call to var np=new Point(1,1) using new? This is obviously, entering the "yes" branch, that is, this points to np; 2) Then this.x=1, that is, np.x=1; Point(2,2) function The process of determining this in var p= Point(2,2) in "JavaScript this decision tree" is as follows:
1) var p= Point(2, 2) Is the call made using new? This is obviously not the case. Go to the "No" branch, that is, is the function called with dot(.)? ;2) The Point(2,2) function is not called using dot(.)? If the judgment is no, it enters the "No" branch, that is, this here points to the global variable window, then this.x is actually window.x;3) this.x=2 means window.x=2 .Finally, let’s look at an example of a function being called using call and apply:function Point(x, y){ this.x = x; this.y = y; this.moveTo = function(x, y){ this.x = x; this.y = y; } } var p1 = new Point(0, 0); var p2 = {x: 0, y: 0}; p1.moveTo.apply(p2, [10, 10]);//apply实际上为p2.moveTo(10,10) p2.x//10
JavaScript this decision The decision-making process in the tree" is as follows:
We know that the two methods apply and call are extremely powerful. They allow switching the context of function execution, that is, what this is bound to. object. p1.moveTo.apply(p2,[10,10]) is actually p2.moveTo(10,10). Then p2.moveTo(10,10) can be interpreted as:1) Is the p2.moveTo(10,10) function call using new? This is obviously not the case. Go to the "No" branch, that is, is the function called with dot(.)? ;
2) The p2.moveTo(10,10) function is called using dot(.), that is, it enters the "yes" branch, that is, this here points to p2.moveTo(10,10). The previous object p2, so p2. "Function in JavaScript can be executed as an ordinary function or as a method of an object. This is the main reason why this has such rich meaning. When a function is executed, an execution environment (ExecutionContext) will be created. The function's All actions occur in this execution environment. When building this execution environment, JavaScript first creates
argumentsvariables, which contain the parameters passed in when calling the function. Then it creates the scope chain. , first initialize the formal parameter list of the function, the value is the corresponding value in the
argumentsvariable, if there is no corresponding value in thearguments
variable, the formal parameter is initialized to
undefined. If the function contains internal functions, initialize these internal functions. If not, continue to initialize the local variables defined in the function. It should be noted that these variables are initialized to
undefinedat this time, and their assignment operations After the execution environment (ExecutionContext) is successfully created, the function will not be executed. This is very important for us to understand the variable scope in JavaScript. In view of the length, we will not discuss this topic here. Finally,
this##. #Variable assignment, as mentioned above, will be assigned to thethis
global object, current object, etc., depending on the function calling method. At this point, the execution environment (ExecutionContext) of the function is successfully created, and the function begins to execute line by line. The required variables are all read from the previously constructed execution environment (ExecutionContext). "Understanding this paragraph will be of great benefit to understanding Javascript functions.
The above is the detailed content of Detailed introduction to JavaScript: What exactly does this point to? (pictures and text). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


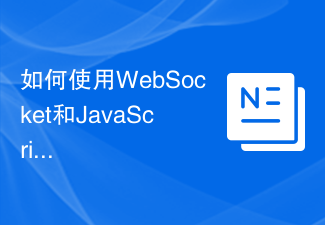
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
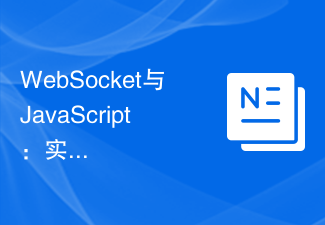
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
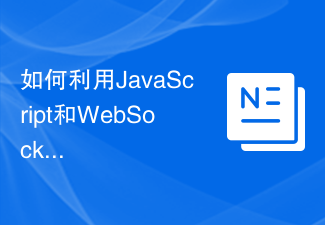
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
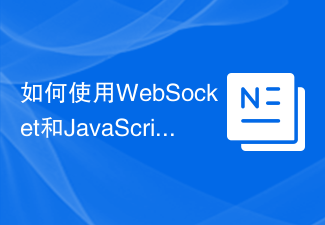
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
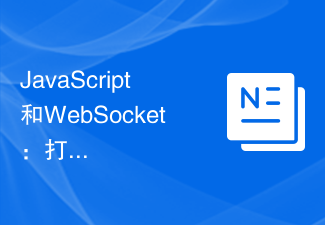
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
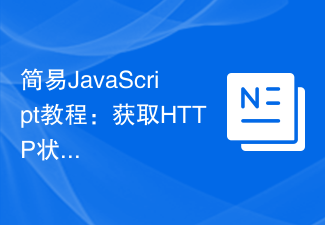
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
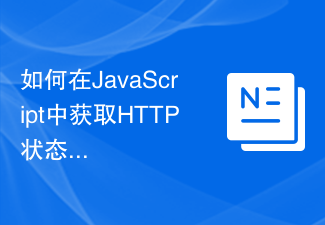
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
