Detailed explanation of Collection in Java
Java collections are toolkits provided by java and include commonly used data structures: collections , linked list, queue, stack, array, mapping, etc. The location of the Java collection toolkit is java.util.*
Java collections can be mainly divided into 4 parts: List list, Set collection, Map mapping, tool class (Iterator iterator, Enumeration enumeration class, Arrays and Collections).
The Java collection framework is as shown below:
As you can see from the above figure, the Java framework is mainly Collection and Map.
1. Collection is an interface, which is a highly abstracted collection. The interface contains basic operations and properties.
Collection contains two branches: List and Set:
1). List is an ordered queue, and each element has its index , the index value of the first element is 0. The implementation classes of List include LinkedList, ArrayList, Vector and Stack.
(1) LinkedList implements the List interface, allowing elements to be empty. LinkedList provides additional get, remove, and insert methods. These operations can enable LinkedList to be used as a stack, queue, or bidirectional queue. .
LinkedList is not thread-safe. If multiple threads access LinkedList at the same time, you must implement access synchronization yourself, or another solution is to construct a synchronized List when creating the List.
(2) ArrayList implements a variable-sized array, allowing all elements to include null. At the same time, ArrayList is not thread-safe.
(3) Vector is similar to ArrayList, but Vector is thread-safe.
(4) Stack inherits from Vector and implements a last-in-first-out stack.
Comparison of Vector, ArrayLis and LinkedList:
(1) Vector is thread-safe, ArrayList and LinkedList are not thread-safe, but thread safety factors are generally not considered, and ArrayList and LinkedList are more efficient .
(2) ArrayList and Vector implement data structures based on dynamic arrays, while LinkedList is a data structure based on linked lists.
(3) Performance of querying, deleting, etc. of arrays and linked lists.
2) Set is a set that does not allow duplicate elements. The implementation classes of set include Hashset and Treeset. HashSet depends on HashMap and is actually implemented through HashMap; TreeSet depends on TreeMap and is implemented through TreeMap.
2. Map is a mapping interface, using key-value key-value pairs.
AbstractMap is an abstract class, which implements most of the API in the Map interface. HashMap, TreeMap and WeakHashMap all inherit from AbstractMap. Although HashTable inherits from Dictionary, But it implements the Map interface.
1), HashTable
(1) HashTable inherits the Map interface and implements a hash table of key-value mapping, any non-empty Object can be used as key or value.
(2) The time overhead of adding data put and removing data get is constant.
(3) Since the object used as a key determines the position of the corresponding value by calculating its hash function , any object used as a key must implement hashCode and equals method. Both hashCode and equals methods are inherited from the root class Object.
(4) HashTable is thread-safe.
2), HashMap
(1) HashMap is similar to HashTable, but HashMap is not thread-safe and allows both key and value to be empty.
(2) When treating HashMap as a Collection, its iteration operation time cost is proportional to the capacity of HashMap. If the iteration performance operation is very important, do not set the initialization capacity of HashMap too high.
3), TreeMap
(1) HashMap quickly searches its content through Hashcode, unordered, while all elements in TreeMap remain In a certain fixed order, orderly.
(2) TreeMap has no tuning options because the tree is always in a balanced state.
4), WeakHashMap
(1) WeakHashMap is an improved HashMap, which implements "weak reference" for the key. If a key is no longer If referenced externally, the key can be recycled by GC.
Summary
(1) If operations involving stacks, queues, etc., you should consider using List; for those that need to quickly insert and delete elements, LinkedList should be used; if fast random access to elements is required, ArrayList should be used.
(2) If the program is in a single-threaded environment, or access is only performed in one thread, consider asynchronous classes, which are more efficient; if multiple threads may operate a class at the same time, synchronization should be used the type.
(3) Pay special attention to the operation of the hash table. The equals and hashCode methods of the key object must be correctly copied.
(4) When using Map, it is best to use HashMap or HashTable to search, update, delete, and add; when traversing Map in natural order or custom key order, it is best to use TreeMap;
(5) Try to return the interface rather than the actual type, such as returning List instead of ArrayList, so that if you need to replace ArrayList with LinkedList in the future, the client code does not need to change. This is programming for abstraction.
Collection interface source code
public interface Collection<E> extends Iterable<E> { int size(); //大小 boolean isEmpty();//是否为空 boolean contains(Object o); //是否包含某个对象 Iterator<E> iterator(); //迭代 Object[] toArray(); //转化为数组 <T> T[] toArray(T[] a); boolean add(E e); //增加对象 boolean remove(Object o); //删除对象 boolean containsAll(Collection<?> c); //判断是否包含相同的Collection boolean addAll(Collection<? extends E> c); //将Collection追加到 boolean removeAll(Collection<?> c); //删除所有相同对象 default boolean removeIf(Predicate<? super E> filter) { Objects.requireNonNull(filter); boolean removed = false; final Iterator<E> each = iterator(); while (each.hasNext()) { if (filter.test(each.next())) { each.remove(); removed = true; } } return removed; } boolean retainAll(Collection<?> c); void clear(); boolean equals(Object o); int hashCode(); @Override default Spliterator<E> spliterator() { return Spliterators.spliterator(this, 0); } default Stream<E> stream() { return StreamSupport.stream(spliterator(), false); } default Stream<E> parallelStream() { return StreamSupport.stream(spliterator(), true); } }
The above is the detailed content of Detailed explanation of Collection in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


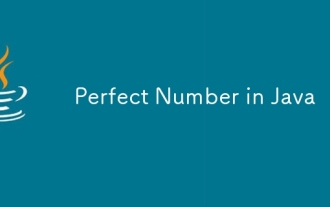
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
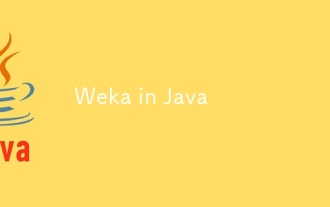
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
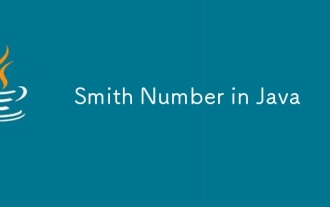
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
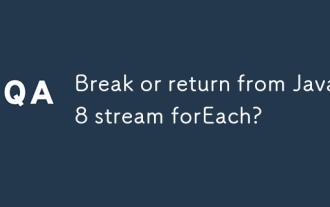
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
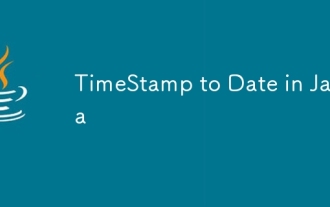
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
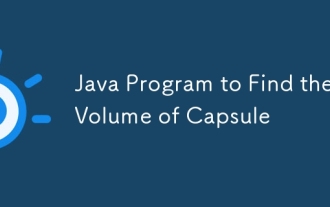
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
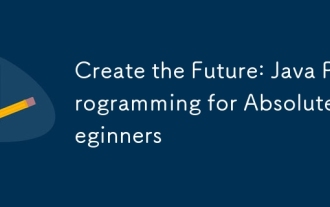
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
