


Automated development using Python - introduction to variables, data types and operation methods
1. Variables
Variable definition: Variables are used to store information to referrenced and manipulated in a computer program.
Used to Store the intermediate operation results of the program running
Identification
Storage
Variables are used in the program A variable name means
The variable name must be a combination of uppercase and lowercase English, numbers and _, and cannot start with a number
-
Sensitive to case
Recommended camel case naming method, such as myFirstName, myLastName
-
Keywords cannot be declared as variables
In Python, the equal sign = is an assignment statement, and any can be Data type is assigned to a variable. The same variable can be assigned repeatedly, and it can be a different type of variable
##myFisrtName = "Jonathan" myLastName ="Ni" |
##Please do not equate the equal sign of the assignment statement with the mathematical equal sign. For example, the following code:
8 x = x + 2
|
Understand mathematically x = x +
2is not established. In the program, the assignment statement first calculates the expression x + 2 on the right side, obtains the result 10, and then assigns it to the variable x. Since the previous value of x was
8, after reassignment, the value of x becomes 10.
It is very important to understand the representation of variables in computer memory, as shown below in declaring variables and assigning values.
a = "ABC" |
- "ABC"## in memory
- #String"ABC" .
-
Constant
is a quantity that cannot be changed, usually represented by a variable name in all uppercase letters.
##
Summary: Inside the computer, any data is regarded as an "object", and Variables are used in programs to point to these data objects, and assigning values to variables associates data with variables.
2. Data types A computer is a machine that can do mathematical calculations. Computer programs naturally deal with various numerical values. In addition to numerical values, computers can also process various data such as text, graphics, audio, video, web pages, etc. Different data requires different data types to be defined. 1. Numbers Integers : The representation method is the same as the mathematical writing method, such as 1, -100, 0, 1000, etc. Or hexadecimal representation, 0xffffff, 0xabcd, etc. Floating point numbers: that is, decimals, such as 0.99, -1.25, 88.88, etc. Or expressed in scientific notation 1.23e8, 1.2e-8, etc. Complex numbers: composed of real part and imaginary part grouped, general form It is x + yj, such as (-5+4j), etc. There is no size limit for numbers. If they exceed a certain range, they are directly expressed as inf(Infinite) 2. StringA string is any text enclosed in single quotes ' or double quotes ", such as 'abc', "XYZ", etc. ' or " itself is just a representation, not part of the string. For example, 'abc' only has three characters: a, b, and c. If ' itself is also a character, it can be enclosed by "", for example "I'm OK"The characters included are I, ', m, space, O, K these 6 characters. Strings containing both ' and " can be identified by the escape character \. For example, 'I\'m \"OK\"!'means I' m "OK"! escape characters can escape many characters, such as \nrepresents a newline, \t represents a tab character, \\ represents the characters \ If there are many line breaks inside the string, you can use '''.....'''The format represents multi-line content. Common string functions#string
method returns the characters. String length.
|
The above is the detailed content of Automated development using Python - introduction to variables, data types and operation methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


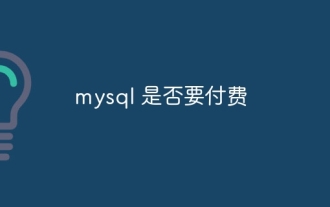
MySQL has a free community version and a paid enterprise version. The community version can be used and modified for free, but the support is limited and is suitable for applications with low stability requirements and strong technical capabilities. The Enterprise Edition provides comprehensive commercial support for applications that require a stable, reliable, high-performance database and willing to pay for support. Factors considered when choosing a version include application criticality, budgeting, and technical skills. There is no perfect option, only the most suitable option, and you need to choose carefully according to the specific situation.
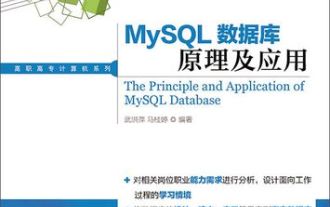
The article introduces the operation of MySQL database. First, you need to install a MySQL client, such as MySQLWorkbench or command line client. 1. Use the mysql-uroot-p command to connect to the server and log in with the root account password; 2. Use CREATEDATABASE to create a database, and USE select a database; 3. Use CREATETABLE to create a table, define fields and data types; 4. Use INSERTINTO to insert data, query data, update data by UPDATE, and delete data by DELETE. Only by mastering these steps, learning to deal with common problems and optimizing database performance can you use MySQL efficiently.
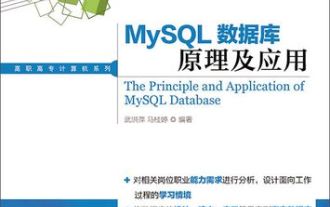
MySQL download file is corrupt, what should I do? Alas, if you download MySQL, you can encounter file corruption. It’s really not easy these days! This article will talk about how to solve this problem so that everyone can avoid detours. After reading it, you can not only repair the damaged MySQL installation package, but also have a deeper understanding of the download and installation process to avoid getting stuck in the future. Let’s first talk about why downloading files is damaged. There are many reasons for this. Network problems are the culprit. Interruption in the download process and instability in the network may lead to file corruption. There is also the problem with the download source itself. The server file itself is broken, and of course it is also broken when you download it. In addition, excessive "passionate" scanning of some antivirus software may also cause file corruption. Diagnostic problem: Determine if the file is really corrupt
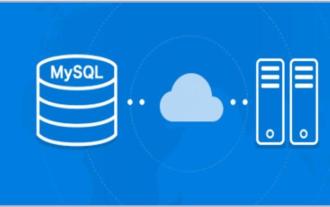
The main reasons for MySQL installation failure are: 1. Permission issues, you need to run as an administrator or use the sudo command; 2. Dependencies are missing, and you need to install relevant development packages; 3. Port conflicts, you need to close the program that occupies port 3306 or modify the configuration file; 4. The installation package is corrupt, you need to download and verify the integrity; 5. The environment variable is incorrectly configured, and the environment variables must be correctly configured according to the operating system. Solve these problems and carefully check each step to successfully install MySQL.
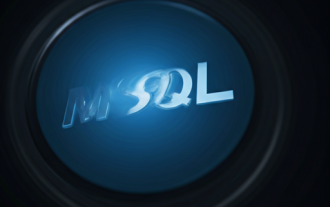
MySQL database performance optimization guide In resource-intensive applications, MySQL database plays a crucial role and is responsible for managing massive transactions. However, as the scale of application expands, database performance bottlenecks often become a constraint. This article will explore a series of effective MySQL performance optimization strategies to ensure that your application remains efficient and responsive under high loads. We will combine actual cases to explain in-depth key technologies such as indexing, query optimization, database design and caching. 1. Database architecture design and optimized database architecture is the cornerstone of MySQL performance optimization. Here are some core principles: Selecting the right data type and selecting the smallest data type that meets the needs can not only save storage space, but also improve data processing speed.
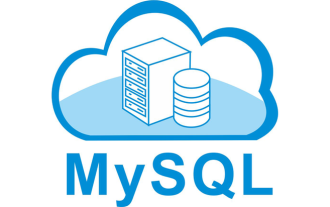
MySQL performance optimization needs to start from three aspects: installation configuration, indexing and query optimization, monitoring and tuning. 1. After installation, you need to adjust the my.cnf file according to the server configuration, such as the innodb_buffer_pool_size parameter, and close query_cache_size; 2. Create a suitable index to avoid excessive indexes, and optimize query statements, such as using the EXPLAIN command to analyze the execution plan; 3. Use MySQL's own monitoring tool (SHOWPROCESSLIST, SHOWSTATUS) to monitor the database health, and regularly back up and organize the database. Only by continuously optimizing these steps can the performance of MySQL database be improved.
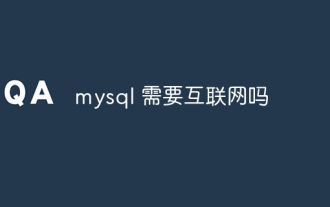
MySQL can run without network connections for basic data storage and management. However, network connection is required for interaction with other systems, remote access, or using advanced features such as replication and clustering. Additionally, security measures (such as firewalls), performance optimization (choose the right network connection), and data backup are critical to connecting to the Internet.
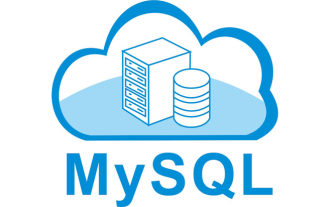
MySQL refused to start? Don’t panic, let’s check it out! Many friends found that the service could not be started after installing MySQL, and they were so anxious! Don’t worry, this article will take you to deal with it calmly and find out the mastermind behind it! After reading it, you can not only solve this problem, but also improve your understanding of MySQL services and your ideas for troubleshooting problems, and become a more powerful database administrator! The MySQL service failed to start, and there are many reasons, ranging from simple configuration errors to complex system problems. Let’s start with the most common aspects. Basic knowledge: A brief description of the service startup process MySQL service startup. Simply put, the operating system loads MySQL-related files and then starts the MySQL daemon. This involves configuration
