


Sample code for solving the median problem of two ordered lists using JavaScript
Arrange the numbers in a sequence from small to large. At this time, the variable value located in the middle is called the median value.
So, given two ordered lists, how to find their common median?
When you get this problem, the first solution you think of is to merge the two ordered lists, then sort them in ascending order, and finally take out the median value at once.
This approach is very simple and convenient, but it is not very efficient. Because of the sorting, it is an algorithm of O(N*logN).
So, how to optimize?
You can refer to the algorithm for merging ordered linear lists:
1. Use two pointers to point to the current ordered list, and use a new array to receive the comparison of smaller array elements.
2. Compare the array elements pointed to by the two pointers, store the smaller one in the new array, and move the pointer backward. This process will continue until one of the pointers is empty, or the median value has been received by the new array, then the median value will be returned directly.
3.If after phase 2 is completed, a pointer is non-null, and the middle value is not received by the new array at this time, then continue to use the pointer to traverse the ordered list , until the median value is received, return it.
4.The optimized algorithm is O(m+n), and the efficiency is greatly improved.
var findMedianSortedArrays = function(nums1, nums2) { //两个列表的总元素个数 var totalLength = nums1.length + nums2.length; //总元素个数是否为奇数 var isOdd = totalLength % 2 === 0 ? false : true; //两个指针 var p1 = 0; var p2 = 0; //用于接收的新数组 var array = []; //只要指针仍然在范围内 while(p1 < nums1.length && p2 < nums2.length){ //将较小的元素压入新数组,指针后移 if(nums1[p1] < nums2[p2]){ array.push(nums1[p1]); p1++; } else{ array.push(nums2[p2]); p2++; } //如果此时已接收中值,弹出中值,返回 if(array.length === totalLength / 2 + 1){ return (array.pop() + array.pop()) / 2; } if(isOdd && array.length === Math.ceil(totalLength / 2)){ return array.pop(); } } //有一个指针已经出界了 //此时仍然没有接收到中值 //对另一个指针继续遍历 //直到接收中值,弹出中值,并返回 while(p1 < nums1.length){ array.push(nums1[p1]); if(array.length === totalLength / 2 + 1){ return (array.pop() + array.pop()) / 2; } if(isOdd && array.length === Math.ceil(totalLength / 2)){ return array.pop(); } p1++; } while(p2 < nums2.length){ array.push(nums2[p2]); if(array.length === totalLength / 2 + 1){ return (array.pop() + array.pop()) / 2; } if(isOdd && array.length === Math.ceil(totalLength / 2)){ return array.pop(); } p2++; } };
The above is the detailed content of Sample code for solving the median problem of two ordered lists using JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


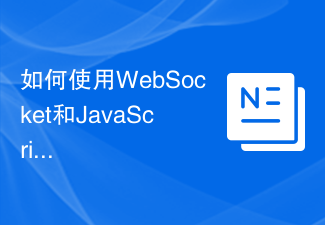
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
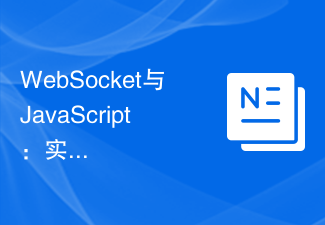
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
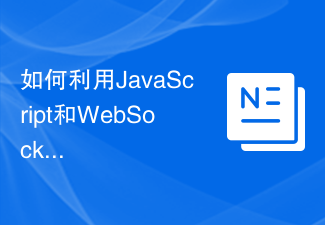
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
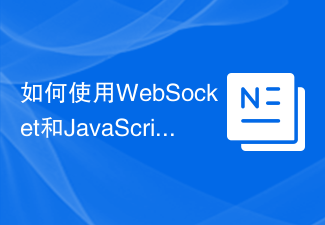
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
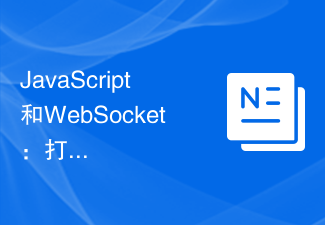
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
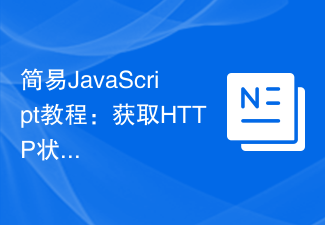
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
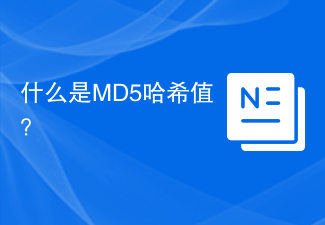
What is the MD5 value? In computer science, MD5 (MessageDigestAlgorithm5) is a commonly used hash function used to digest or encrypt messages. It produces a fixed-length 128-bit binary number, usually represented in 32-bit hexadecimal. The MD5 algorithm was designed by Ronald Rivest in 1991. Although the MD5 algorithm is considered no longer secure in the field of cryptography, it is still widely used in data integrity verification and file verification.
