


Detailed introduction to the observer pattern of JavaScript design patterns
Javascript is active in an event-driven environment, such as mouse response, event callback, network request, etc. Observer
mode is also called publisher-subscriber (publisher-subscriber) Pattern
deals with the relationship between objects, their behaviors and states, and manages the relationship between people and tasks.
1. The most common observer pattern
1.1 Event listener
document.body.addEventListener('click', function () { console.log('you clicked me, poor guy!') });
This is the simplest and most common observer pattern, except click In addition to
, there are also load
, blur
, drag
, focus
, mouseover
, etc. An event listener (listener) is different from an event handler (handler). In an event listener, an event can be associated with multiple listeners, and each listener independently processes the monitored message; the event handler is responsible for executing and processing the event. After the associated function, an event can have a processing function:
var dom = $('.dom'); var listener1 = function(e){ //do one thing } var listener2 = function(e){ //do another thing } addEvent(dom,'click',listener1); addEvent(dom,'click',listener2);
In this example of the event listener, listener1
and listener2
are both dom elements The listeners will execute their respective functions when the dom is clicked;
var dom = document.getElementById('dom'); var handler1 = function(e){ //do one thing } var handler2 = function(e){ //do another thing } dom.onclick = handler1; dom.onclick = handler2;
In the example of this event handler, handler1
will not be executed, only handler2
is an assignment operation.
1.2 Animation
The observer mode is widely used in animation. The start, completion, pause, etc. of animation require an observer to determine the behavior and status of the object.
//定义动画 var Animation = function(){ this.onStart = new Publisher; //关于Publisher的设计将在1.3节介绍 this.onComplete = new Publisher; this.onTween = new Publisher; } //定义一个原型方法 Animation.prototype.look = function(){ this.onStart.deliver('animation started!'); this.onTween.deliver('animation is going on!'); this.onComplete.deliver('animation completed!'); }; //实例一个box对象 var box = new Animation(); //定义三个函数作为subscribers var openBox = function(msg){ console.log(msg) } var checkBox = function(msg){ console.log(msg) } var closeBox = function(msg){ console.log(msg) } //订阅事件 openBox.subscribe(box.onStart); checkBox.subscribe(box.onTween); closeBox.subscribe(box.onComplete); //调用方法 box.look() //animation started! //animation is going on! //animation completed!
1.3 Construction of Observer
First, a publisher is needed. First define a constructor and an array for it to save subscriber information:
function Publisher(){ this.subscribes = []; }
The publisher has the function of publishing messages, and defines a prototype function of deliver:
Publisher.prototype.deliver = function(data){ this.subscribes.forEach(function(fn){ fn(data); }); return this; }
Next Construct a subscription method:
Function.prototype.subscribe = function(publisher){ var that = this; var alreadyExists = publisher.subscribes.some(function(el){ return el === that; }); if(!alreadyExists){ publisher.subscribes.push(this); } return this; }
Add the subscribe method directly to the Function prototype so that all functions can call this method. In this way, the construction is completed. For usage methods, please refer to the use case of 1.2 Animation.
A more intuitive explanation (take onStart
as an example): When the box
object executes the look
method, execute onStart.deliver()
, publishes the onStart
event and broadcasts the notification 'animation started!'
. At this time, it has been monitoring onStart
openBox
Listen to the information released by the event and print it out.
1.4 Another way to build an observer
This method imitates the event processing mechanism of nodejs, and the code is relatively concise:
var scope = (function() { //消息列表 var events = {}; return { //订阅消息 on:function(name,hander){ var index = 0; //记录消息时间的索引 if(events[name]){ //消息名已存在,将处理函数放到该消息的事件队列中 index = events[name].push(hander) - 1; }else{ events[name] = [hander]; } //返回当前消息处理事件的移除函数 return function(){ events[name].splice(index,1); } }, //关闭消息 off:function(name){ if(!events[name]) return; //消息存在,删除消息 delete events[name]; }, //消息发布 emit:function(name,msg){ //消息不存在,不处理 if(!events[name]) return; //消息存在,将该事件处理队列中每一个函数都执行一次 events[name].forEach(function(v,i){ v(msg); }); } } })(); var sayHello = scope.on('greeting',function(msg){ console.log('订阅消息:' + msg); }); var greeting = function(msg){ console.log('发布消息:' + msg); scope.emit('greeting', msg); } greeting('hello Panfen!')
1.5 Observer mode in nodejs Implementation plan
There is an events module in nodejs to implement the observer mode. You can refer to Nodejs API-Events to talk about the observer mode. Most modules integrate the events module, so you can directly use emit to launch events and on Listen for events, or define it first like below;
var EventEmitter = require('events').EventEmitter; var life = new EventEmitter(); life.setMaxListeners(11); //设置最大监听数,默认10 //发布和订阅sendName life.on('sendName',function(name){ console.log('say hello to '+name); }); life.emit('sendName','jeff'); //发布和订阅sendName2 function sayBeautiful(name){ console.log(name + ' is beautiful'); } life.on('sendName2',sayBeautiful); life.emit('sendName2','jeff');
Common methods:
hasConfortListener: Used to determine whether the emitted event has a listener
removeListener: Remove the listener
listenerCount: The total number of all listeners for this event
removeAllListeners: Remove All (or one) event listeners
pushand
listen The logic is suitable for situations where you want to separate human behavior from application behavior. For example: when the user clicks a tab on the navigation bar, a submenu containing more options will open. Generally, the user will choose to listen to the click event directly if he knows which element. The disadvantage of this is that it is not the same as the click event. The events are directly tied together. A better approach is to create an observable onTabChange object and associate several observer implementations.
Detailed explanation of the classic JavaScript design pattern, the strategy pattern
##The classic JavaScript design pattern, the simple factory pattern code exampleDetailed Explanation of the Classic Singleton Pattern of JavaScript Design PatternsThe above is the detailed content of Detailed introduction to the observer pattern of JavaScript design patterns. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


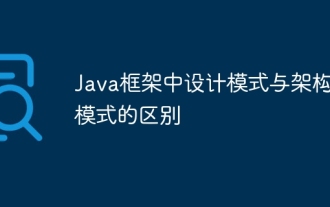
In the Java framework, the difference between design patterns and architectural patterns is that design patterns define abstract solutions to common problems in software design, focusing on the interaction between classes and objects, such as factory patterns. Architectural patterns define the relationship between system structures and modules, focusing on the organization and interaction of system components, such as layered architecture.
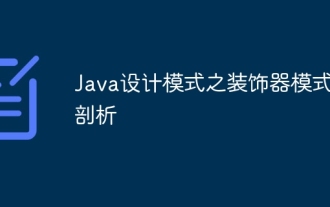
The decorator pattern is a structural design pattern that allows dynamic addition of object functionality without modifying the original class. It is implemented through the collaboration of abstract components, concrete components, abstract decorators and concrete decorators, and can flexibly expand class functions to meet changing needs. In this example, milk and mocha decorators are added to Espresso for a total price of $2.29, demonstrating the power of the decorator pattern in dynamically modifying the behavior of objects.
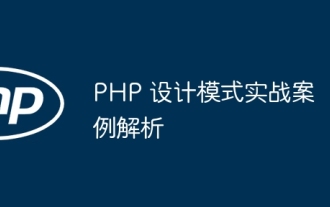
1. Factory pattern: Separate object creation and business logic, and create objects of specified types through factory classes. 2. Observer pattern: allows subject objects to notify observer objects of their state changes, achieving loose coupling and observer pattern.
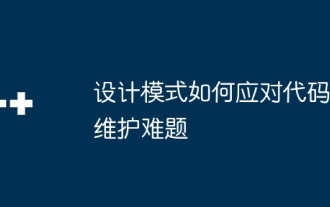
Design patterns solve code maintenance challenges by providing reusable and extensible solutions: Observer Pattern: Allows objects to subscribe to events and receive notifications when they occur. Factory Pattern: Provides a centralized way to create objects without relying on concrete classes. Singleton pattern: ensures that a class has only one instance, which is used to create globally accessible objects.
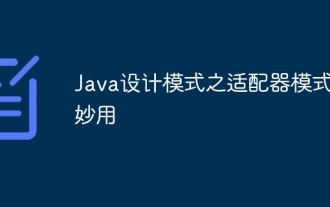
The Adapter pattern is a structural design pattern that allows incompatible objects to work together. It converts one interface into another so that the objects can interact smoothly. The object adapter implements the adapter pattern by creating an adapter object containing the adapted object and implementing the target interface. In a practical case, through the adapter mode, the client (such as MediaPlayer) can play advanced format media (such as VLC), although it itself only supports ordinary media formats (such as MP3).
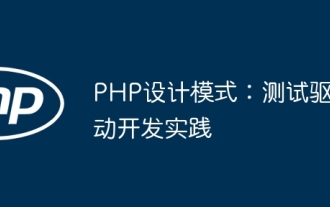
TDD is used to write high-quality PHP code. The steps include: writing test cases, describing the expected functionality and making them fail. Write code so that only the test cases pass without excessive optimization or detailed design. After the test cases pass, optimize and refactor the code to improve readability, maintainability, and scalability.
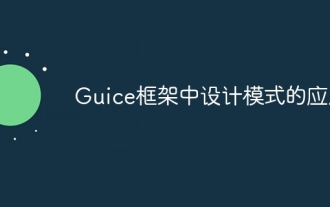
The Guice framework applies a number of design patterns, including: Singleton pattern: ensuring that a class has only one instance through the @Singleton annotation. Factory method pattern: Create a factory method through the @Provides annotation and obtain the object instance during dependency injection. Strategy mode: Encapsulate the algorithm into different strategy classes and specify the specific strategy through the @Named annotation.
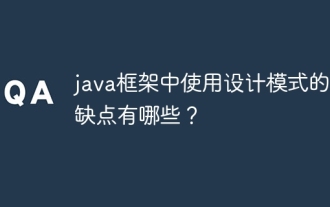
The advantages of using design patterns in Java frameworks include: enhanced code readability, maintainability, and scalability. Disadvantages include complexity, performance overhead, and steep learning curve due to overuse. Practical case: Proxy mode is used to lazy load objects. Use design patterns wisely to take advantage of their advantages and minimize their disadvantages.
