Django advanced learning record
Preface: This blog is a supplement to the previous blogdjangoAdvanced.
1. Rendering
The front-end interface is relatively simple (ugly) and has two functions:
Get the book title from the database eg: New book A
Enter the title of the book in the form, select the publisher, and select the author ( Multiple selection), after completing the input, click Create New Book Submit to create data in the database
2. Implementation
Let’s implement the first function first, printing the book title on the page based on the database data.
1. Add urlrouting
url(r'^book/', views.book),
2. Define the book method in views.py
Django uses the GET method by default, that is, to obtain data; if you want to create/modify data, such as the second function to be implemented later, you need to use the POST method.
def book(request): books = models.Book.objects.all() #找到所有的书 publisher_list = models.Publisher.objects.all() author_list = models.Author.objects.all() print("---->:", request) return render(request, "app01/book.html", {"books":books, "publishers":publisher_list, "authors":author_list})
3. Create book.html under templates/app01:
books is in the database A collection of objects for all books. Use a loop in HTML to display the book titles on the front-end page.
<h2 id="书列表">书列表:</h2> <ul> {% for book in books %} <li>{{ book.name }}</li> {% endfor %} </ul>
Next, implement the second function and create data.
Let’s look at the front-end html first:
<form method="post" action="/payment/book/"> {% csrf_token %} book name:<input type="text" name="name"/> <select name="publisher_id"> {% for publisher in publishers %} <option value="{{ publisher.id }}">{{ publisher.name }}</option> {% endfor %} </select> <select name="author_ids" multiple="multiple"> {% for author in authors %} <option value="{{ author.id }}">{{ author.first_name }}</option> {% endfor %} </select> <div> <input type="submit" value="创建新书"/> </div> </form>
Note:
Because It is to create data, so the submission method must use post, action="/payment/book/" is a url, which means submitting the data to the book method, and the data is encapsulated in the request parameter.
When you select a publishing house, do you need to send the name of the publishing house to the backend? Therefore, I added the value attribute to the option tag to obtain the id of the publisher. When you click submit to submit the data, the id in the value will be submitted to the name attribute of the select tag, name The attribute then submits the data to the background.
You will find that the first line of the html code has {% csrf_token %}. I don’t know what this means yet~_~, I If you remove this code, the data will not be submitted!!
Let’s look at the background book method
def book(request): if request.method == "POST": #若是创建书的数据 print(request.POST) book_name = request.POST.get("name") publisher_id = request.POST.get("publisher_id") # 即使在前端页面选择多个作者只会返回一个值,只能取到最后一个作者的id #author_ids = request.POST.get("author_ids") author_ids = request.POST.getlist("author_ids") #getlist 可取出所有作者的id #生成一个书的对象 new_book = models.Book( name = book_name, publisher_id = publisher_id, publish_date = "2017-3-18" ) new_book.save() #同步到数据库 #new_book.authors.add(1,2) 添加作者 new_book.authors.add(*author_ids) #author_ids为列表,需在前面加上*转化为id print("------->>:", book_name,publisher_id,author_ids) books = models.Book.objects.all() publisher_list = models.Publisher.objects.all() author_list = models.Author.objects.all() print("---->:", request) return render(request, "app01/book.html", {"books":books, "publishers":publisher_list, "authors":author_list})
When I enter the book title in the front-end interface: New Book A, select the second publisher, select the 2nd and 3rd authors, for the convenience of viewing, I print it out in the background:
<QueryDict: {'name': ['新书A'], 'csrfmiddlewaretoken': ['V9OdHSJ10OFSq3r vI41tggns1W2VxwV'], 'publisher_id': ['2'], 'author_ids': ['2', '3']}> ------->>: 新书A 2 ['2', '3'] ---->: <WSGIRequest: POST '/payment/book/'> [18/Mar/2017 14:06:23] "POST /payment/book/ HTTP/1.1" 200 1335
According to the printing results, I know that author_ids is a list. When I add an author to a book, I use the following code:
new_book.authors.add(*author_ids)
Why do I need to add * in front of the list? Not adding * will expose an error! Adding * is to convert the list form ["2", "3"] into the author id form 2,3.
Log in to the admin background to view the new book A just created:
The above is the detailed content of Django advanced learning record. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
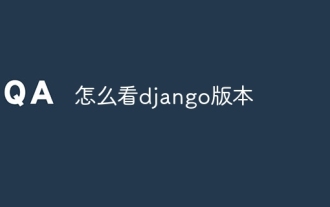
Steps to check the Django version: 1. Open a terminal or command prompt window; 2. Make sure Django has been installed. If Django is not installed, you can use the package management tool to install it and enter the pip install django command; 3. After the installation is complete , you can use python -m django --version to check the Django version.
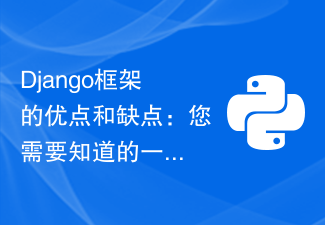
Django is a complete development framework that covers all aspects of the web development life cycle. Currently, this framework is one of the most popular web frameworks worldwide. If you plan to use Django to build your own web applications, then you need to understand the advantages and disadvantages of the Django framework. Here's everything you need to know, including specific code examples. Django advantages: 1. Rapid development-Djang can quickly develop web applications. It provides a rich library and internal
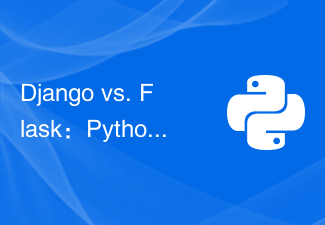
Django and Flask are both leaders in Python Web frameworks, and they both have their own advantages and applicable scenarios. This article will conduct a comparative analysis of these two frameworks and provide specific code examples. Development Introduction Django is a full-featured Web framework, its main purpose is to quickly develop complex Web applications. Django provides many built-in functions, such as ORM (Object Relational Mapping), forms, authentication, management backend, etc. These features allow Django to handle large
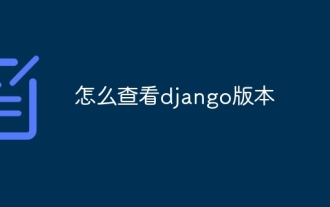
How to check the django version: 1. To check through the command line, enter the "python -m django --version" command in the terminal or command line window; 2. To check in the Python interactive environment, enter "import django print(django. get_version())" code; 3. Check the settings file of the Django project and find a list named INSTALLED_APPS, which contains installed application information.
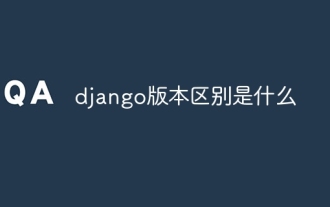
The differences are: 1. Django 1.x series: This is an early version of Django, including versions 1.0, 1.1, 1.2, 1.3, 1.4, 1.5, 1.6, 1.7, 1.8 and 1.9. These versions mainly provide basic web development functions; 2. Django 2.x series: This is the mid-term version of Django, including 2.0, 2.1, 2.2 and other versions; 3. Django 3.x series: This is the latest version series of Django. Including versions 3.0, 3, etc.
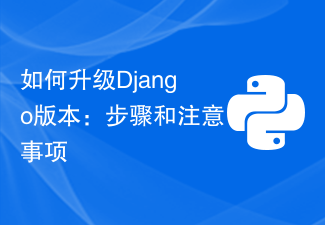
How to upgrade Django version: steps and considerations, specific code examples required Introduction: Django is a powerful Python Web framework that is continuously updated and upgraded to provide better performance and more features. However, for developers using older versions of Django, upgrading Django may face some challenges. This article will introduce the steps and precautions on how to upgrade the Django version, and provide specific code examples. 1. Back up project files before upgrading Djan
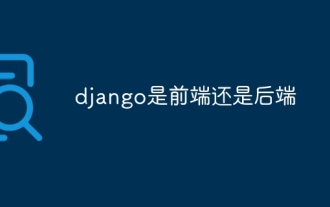
django is the backend. Details: Although Django is primarily a backend framework, it is closely related to front-end development. Through features such as Django's template engine, static file management, and RESTful API, front-end developers can collaborate with back-end developers to build powerful, scalable web applications.
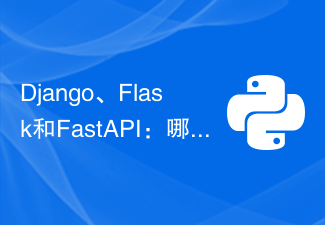
Django, Flask, and FastAPI: Which framework is right for beginners? Introduction: In the field of web application development, there are many excellent Python frameworks to choose from. This article will focus on the three most popular frameworks, Django, Flask and FastAPI. We will evaluate their features and discuss which framework is best for beginners to use. At the same time, we will also provide some specific code examples to help beginners better understand these frameworks. 1. Django: Django
