


Detailed explanation of Python's garbage collection mechanism
1. Garbage collection mechanism
Garbage collection in Python is mainly based on reference counting, supplemented by generational collection. The drawback of reference counting is the problem of circular references.
In Python, if the number of references to an object is 0, the Python virtual machine will reclaim the memory of this object.
#encoding=utf-8 __author__ = 'kevinlu1010@qq.com' class ClassA(): def __init__(self): print 'object born,id:%s'%str(hex(id(self))) def __del__(self): print 'object del,id:%s'%str(hex(id(self))) def f1(): while True: c1=ClassA() del c1
Executing f1() will output such results in a loop, and the memory occupied by the process will basically not change
object born,id:0x237cf58 object del,id:0x237cf58
c1=ClassA() will create an object. Place it in the 0x237cf58 memory, and the c1 variable points to this memory. At this time, the reference count of this memory is 1
After del c1, the c1 variable no longer points to the 0x237cf58 memory, so the reference count of this memory is reduced by one, equal to 0, so The object is destroyed and the memory is released.
Cause the reference count +1
The object is created, for example a=23
The object is referenced, for example b=a
The object is passed as a parameter to a function, such as func(a )
The object is stored in the container as an element, for example list1=[a,a]
Results in a situation where the reference count is -1
The alias of the object is explicitly destroyed, such as del a
The object's The alias is assigned to a new object, for example a=24
An object leaves its scope, for example, when the f function completes execution, the local variable in the func function (global variables will not)
The container in which the object is located is destroyed , or delete the object from the container
demo
def func(c,d): print 'in func function', sys.getrefcount(c) - 1 print 'init', sys.getrefcount(11) - 1 a = 11 print 'after a=11', sys.getrefcount(11) - 1 b = a print 'after b=1', sys.getrefcount(11) - 1 func(11) print 'after func(a)', sys.getrefcount(11) - 1 list1 = [a, 12, 14] print 'after list1=[a,12,14]', sys.getrefcount(11) - 1 a=12 print 'after a=12', sys.getrefcount(11) - 1 del a print 'after del a', sys.getrefcount(11) - 1 del b print 'after del b', sys.getrefcount(11) - 1 # list1.pop(0) # print 'after pop list1',sys.getrefcount(11)-1 del list1 print 'after del list1', sys.getrefcount(11) - 1
Output:
init 24 after a=11 25 after b=1 26 in func function 28 after func(a) 26 after list1=[a,12,14] 27 after a=12 26 after del a 26 after del b 25 after del list1 24
Question: Why does calling the function increase the reference count by +2
View an object The reference count
sys.getrefcount(a) can view the reference count of the a object, but it is 1 larger than the normal count, because a is passed in when calling the function, which will increase the reference count of a by 1
2. Circular references lead to memory leaks
def f2(): while True: c1=ClassA() c2=ClassA() c1.t=c2 c2.t=c1 del c1 del c2
When f2() is executed, the memory occupied by the process will continue to increase.
object born,id:0x237cf30 object born,id:0x237cf58
After c1 and c2 are created, the reference counts of these two memories are 0x237cf30 (the memory corresponding to c1, recorded as memory 1), 0x237cf58 (the memory corresponding to c2, recorded as memory 2). is 1. After executing c1.t=c2 and c2.t=c1, the reference count of these two pieces of memory becomes 2.
After del c1, the reference count of the object in memory 1 becomes 1, because it is not 0, so the object in memory 1 will not be destroyed, so the number of references to the object in memory 2 is still 2. After del c2, in the same way, the number of references to the object in memory 1 and the object in memory 2 is 1.
Although both of their objects can be destroyed, due to circular references, the garbage collector will not recycle them, which will lead to memory leaks.
3. Garbage collection
deff3(): # print gc.collect() c1=ClassA() c2=ClassA() c1.t=c2 c2.t=c1 del c1 del c2 print gc.garbage print gc.collect() #显式执行垃圾回收 print gc.garbage time.sleep(10) if __name__ == '__main__': gc.set_debug(gc.DEBUG_LEAK) #设置gc模块的日志 f3()
Output:
Python
gc: uncollectable <ClassA instance at 0230E918> gc: uncollectable <ClassA instance at 0230E940> gc: uncollectable <dict 0230B810> gc: uncollectable <dict 02301ED0> object born,id:0x230e918 object born,id:0x230e940
4
The objects after garbage collection will be placed In the gc.garbage list
gc.collect() will return the number of unreachable objects, 4 is equal to two objects and their corresponding dict
There are three situations that will trigger garbage collection:
1. Call gc .collect(),
2. When the counter of the gc module reaches the threshold.
3. When the program exits
4. Analysis of common functions of the gc module
Garbage Collector interface
The gc module provides an interface for developers to set garbage collection options. As mentioned above, one of the defects of using reference counting method to manage memory is circular reference, and one of the main functions of the gc module is to solve the problem of circular reference.
Commonly used functions:
gc.set_debug(flags)
Set the debug log of gc, generally set to gc.DEBUG_LEAK
gc.collect([generation])
Explicit garbage collection, You can enter parameters, 0 means to check only the objects of the first generation, 1 means to check the objects of the first and second generations, 2 means to check the objects of the first, second and third generations. If no parameters are passed, a full collection will be executed, which is equivalent to passing 2 .
Return the number of unreachable objects
gc.set_threshold(threshold0[, threshold1[, threshold2])
Set the frequency of automatic garbage collection.
gc.get_count()
Get the current automatic garbage collection counter and return a list of length 3
The automatic garbage collection mechanism of the gc module
The gc module must be imported and is_enable() =True will start automatic garbage collection.
The main function of this mechanism is to discover and handle unreachable garbage objects.
Garbage collection = garbage check + garbage collection
In Python, the generational collection method is used. Divide the object into three generations. Initially, when the object is created, it is placed in the first generation. If the changed object survives a garbage check of the first generation, it will be placed in the second generation. Similarly, in a second generation garbage check, If the object survives the garbage check, it will be placed in the third generation.
There will be a counter with a length of 3 in the gc module, which can be obtained through gc.get_count().
For example (488,3,0), where 488 refers to the number of memory allocated by Python minus the number of released memory since the last generation garbage check. Note that it is memory allocation, not an increase in the reference count. For example:
print gc.get_count() # (590, 8, 0) a = ClassA() print gc.get_count() # (591, 8, 0) del a print gc.get_count() # (590, 8, 0)
3是指距离上一次二代垃圾检查,一代垃圾检查的次数,同理,0是指距离上一次三代垃圾检查,二代垃圾检查的次数。
gc模快有一个自动垃圾回收的阀值,即通过gc.get_threshold函数获取到的长度为3的元组,例如(700,10,10)
每一次计数器的增加,gc模块就会检查增加后的计数是否达到阀值的数目,如果是,就会执行对应的代数的垃圾检查,然后重置计数器
例如,假设阀值是(700,10,10):
当计数器从(699,3,0)增加到(700,3,0),gc模块就会执行gc.collect(0),即检查一代对象的垃圾,并重置计数器为(0,4,0)
当计数器从(699,9,0)增加到(700,9,0),gc模块就会执行gc.collect(1),即检查一、二代对象的垃圾,并重置计数器为(0,0,1)
当计数器从(699,9,9)增加到(700,9,9),gc模块就会执行gc.collect(2),即检查一、二、三代对象的垃圾,并重置计数器为(0,0,0)
其他
如果循环引用中,两个对象都定义了__del__方法,gc模块不会销毁这些不可达对象,因为gc模块不知道应该先调用哪个对象的__del__方法,所以为了安全起见,gc模块会把对象放到gc.garbage中,但是不会销毁对象。
五.应用
项目中避免循环引用
引入gc模块,启动gc模块的自动清理循环引用的对象机制
由于分代收集,所以把需要长期使用的变量集中管理,并尽快移到二代以后,减少GC检查时的消耗
gc模块唯一处理不了的是循环引用的类都有__del__方法,所以项目中要避免定义__del__方法,如果一定要使用该方法,同时导致了循环引用,需要代码显式调用gc.garbage里面的对象的__del__来打破僵局
The above is the detailed content of Detailed explanation of Python's garbage collection mechanism. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


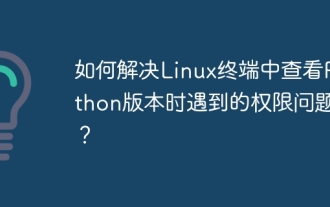
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
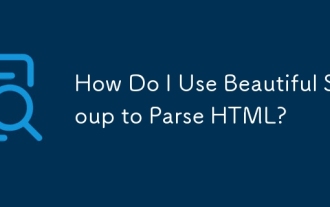
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
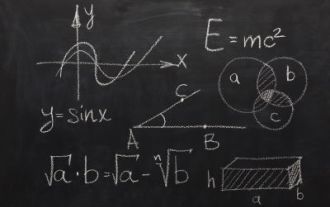
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
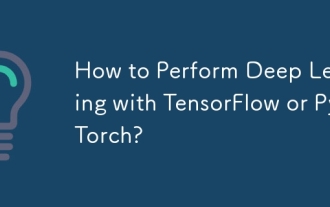
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
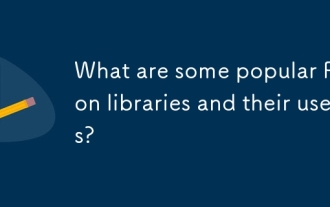
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
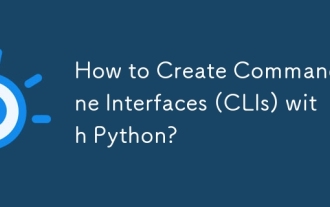
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
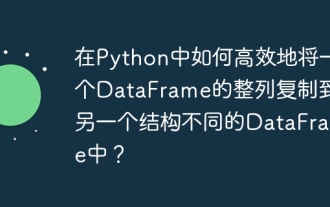
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
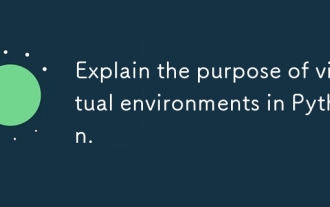
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.
