Detailed explanation of Java Annotation features
What is Annotation?
Annotation is translated into Chinese as annotation, which means to provide additional data information in addition to the logic of the program itself. Annotation has no direct impact on the annotated code. It cannot directly interact with the annotated code, but other components can use this information.
Annotation information can be compiled into a class file, or it can be retained in the Java virtual machine so that it can be obtained at runtime. Annotation can even be added to Annotation itself.
Those objects can be added with Annotation
Classes, methods, variables, parameters, and packages can be added Annotation.
Built-in Annotation
@Override OverloadingThe @Deprecated method or type annotated in the parent class is no longer recommended for use
@SuppressWarnings Prevent compile-time warning messages. It needs to receive an array of String as a parameter. The available parameters are:
unchecked
path
serial
finally
- ##fallthrough
@Retention
To determine thelifecycle of the Annotation being saved, you need to receive an Enum object RetentionPolicy as a parameter.
public enum RetentionPolicy { /** * Annotations are to be discarded by the compiler. */ SOURCE, /** * Annotations are to be recorded in the class file by the compiler * but need not be retained by the VM at run time. This is the default * behavior. */ CLASS, /** * Annotations are to be recorded in the class file by the compiler and * retained by the VM at run time, so they may be read reflectively. * * @see java.lang.reflect.AnnotatedElement */ RUNTIME }
@Documented Documented
@Target
Indicates the range that the Annotation can modify, and receives an Enum object EnumType array as parameter.public enum ElementType { /** Class, interface (including annotation type), or enum declaration */ TYPE, /** Field declaration (includes enum constants) */ FIELD, /** Method declaration */ METHOD, /** Parameter declaration */ PARAMETER, /** Constructor declaration */ CONSTRUCTOR, /** Local variable declaration */ LOCAL_VARIABLE, /** Annotation type declaration */ ANNOTATION_TYPE, /** Package declaration */ PACKAGE }
@Inherited
This Annotation can affect subclasses of the annotated class. Customized AnnotationWe can customize Annotation after JSE5.0. Here is a simple example.@Retention(RetentionPolicy.RUNTIME) @Target(ElementType.METHOD) public @interface MethodAnnotation { }
public class Person { public void eat() { System.out.println("eating"); } @MethodAnnotation public void walk() { System.out.print("walking"); } }
Class<Person> personClass = Person.class; Method[] methods = personClass.getMethods(); for(Method method : methods){ if (method.isAnnotationPresent(MethodAnnotation.class)){ method.invoke(personClass.newInstance()); } }
walking
@Target(ElementType.TYPE) public @interface personAnnotation { int id() default 1; String name() default "bowen"; }
@personAnnotation(id = 8, name = "john") public class Person { public void eat() { System.out.println("eating"); } @MethodAnnotation public void walk() { System.out.print("walking"); } }
interface called AnnotatedElement. Java's reflective object classes Class, Constructor, Field, Method and Package all implement this interface. This interface is used to represent the annotated program elements currently running in the Java virtual machine. Through this interface, you can use reflection to read annotations. The AnnotatedElement interface can access annotations marked with RUNTIME. The corresponding methods are getAnnotation, getAnnotations, and isAnnotationPresent. Because Annotation types are compiled and stored in binaries just like classes, the Annotations returned by these methods can be queried just like Querynormal Java objects.
Wide use of AnnotationAnnotation is widely used in variousframeworks and libraries. Here are some typical applications.
JunitJunit is a very famousunit testing framework. When using Junit, you need to be exposed to a lot of annotations.
- @Runwith custom test class Runner
- @ContextConfiguration Set Spring’s ApplicationContext
- @DirtiesContext Reload the ApplicationContext before executing the next test.
- @Before Initialize before calling the test method
- @After Process after calling the test method
- @Test indicates that the method is a test method
- @Ignore can be added to the test class or test method to ignore the operation.
- @BeforeClass: Called before all test methods in the test class are executed, and only called once (the marked method must be
static)
@AfterClass: Called after all test methods in the test class have been executed and only executed once (the marked method must be static)
Spring
Spring is known as configuration hell, and there are many Annotations.
@Service Add annotations to the service class
@Repository Add annotations to the DAO class
@Component Add annotations to component classes
@Autowired Let Spring automatically assemble beans
@Transactional Configure things
@Scope Configure the object survival scope
@Controller Add annotations to the controller class
-
@RequestMapping url path mapping
@PathVariable Maps method parameters to paths
@RequestParam Binds request parameters to method variables
@ModelAttribute is bound to the model
@SessionAttributes is set to the session attribute
Hibernate
@Entity Modifies the entity bean
@Table Maps the entity class to the table in the database
@Column Mapping column
@Id mapping id
@GeneratedValue This field is self-increasing
@Version version control or concurrency Control
@OrderBy Sorting Rules
@Lob Large Object Annotation
Hibernate and a lot more The annotations about the union and the annotations of inheriting are not meaningful to list here.
JSR 303 – Bean Validation
JSR 303 – Bean Validation is a data validation specification, and its verification of Java beans is mainly implemented through Java annotation.
@NullThe element annotated by must be null
@NotNull annotated The element must not be null
@AssertTrueThe annotated element must be true@AssertFalseThe annotated element must be false@Min(value)The annotated element must be a number whose The value must be greater than or equal to the specified minimum value
@Max(value)The annotated element must be a number, and its value must be less than or equal to the specified maximum value
@DecimalMin(value)The annotated element must be a number, and its value must be greater than or equal to the specified minimum value
@DecimalMax(value)The annotated element must be Is a number whose value must be less than or equal to the specified maximum value
@Size(max, min)The size of the annotated element must be within the specified range
@Digits (integer, fraction) The annotated element must be a number, and its value must be within the acceptable range
@Past The annotated element must be Is a past date
@FutureThe annotated element must be a future date
@Pattern(value)The annotated element Must conform to the specified regular expression
In fact, there are many frameworks or libraries that use annotation, so I won’t list them one by one here. I hope everyone can draw inferences. Learn more about annotations in Java.
The above is the detailed content of Detailed explanation of Java Annotation features. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


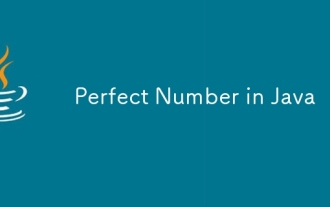
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
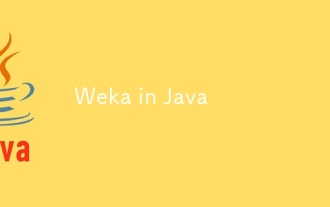
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
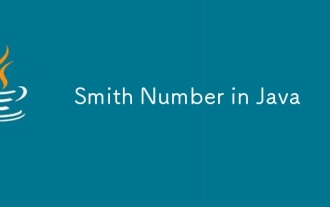
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
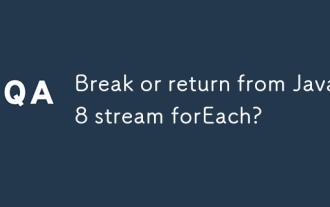
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
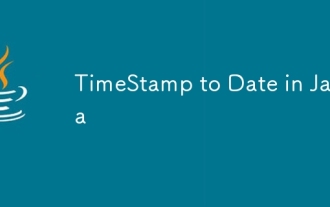
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
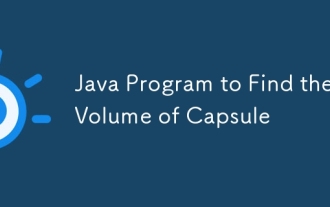
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
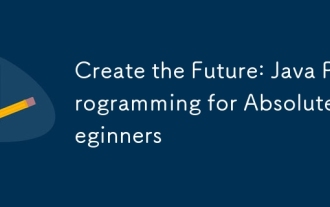
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
