Introducing techniques to Python beginners
The following are some practical Python tips and tools that I have collected in recent years. I hope they will be helpful to you.
Exchange variables
x = 6 y = 5 x, y = y, x print x >>> 5 print y >>> 6
if statement in line
print "Hello" if True else "World" >>> Hello
connect
The last way below is very cool when binding two objects of different types.
nfc = ["Packers", "49ers"] afc = ["Ravens", "Patriots"] print nfc + afc >>> ['Packers', '49ers', 'Ravens', 'Patriots'] print str(1) + " world" >>> 1 world print `1` + " world" >>> 1 world print 1, "world" >>> 1 world print nfc, 1 >>> ['Packers', '49ers'] 1
Number skills
#除后向下取整 print 5.0//2 >>> 2 # 2的5次方 print 2**5 >> 32
Pay attention to the division of floating point numbers
print .3/.1 >>> 2.9999999999999996 print .3//.1 >>> 2.0
Numerical comparison
This is such a great shorthand method that I have rarely seen in many languages
x = 2 if 3 > x > 1: print x >>> 2 if 1 < x > 0: print x >>> 2
Iterate two lists simultaneously
nfc = ["Packers", "49ers"] afc = ["Ravens", "Patriots"] for teama, teamb in zip(nfc, afc): print teama + " vs. " + teamb >>> Packers vs. Ravens >>> 49ers vs. Patriots
Indexed list iteration
teams = ["Packers", "49ers", "Ravens", "Patriots"] for index, team in enumerate(teams): print index, team >>> 0 Packers >>> 1 49ers >>> 2 Ravens >>> 3 Patriots
List comprehension
Given a list, we can brush out the even list method:
numbers = [1,2,3,4,5,6] even = [] for number in numbers: if number%2 == 0: even.append(number)
Convert to the following:
numbers = [1,2,3,4,5,6] even = [number for number in numbers if number%2 == 0]
Isn’t it awesome, haha.
Dictionary derivation
Similar to list comprehensions, dictionaries can do the same job:
teams = ["Packers", "49ers", "Ravens", "Patriots"] print {key: value for value, key in enumerate(teams)} >>> {'49ers': 1, 'Ravens': 2, 'Patriots': 3, 'Packers': 0}
Initialization list value
items = [0]*3 print items >>> [0,0,0]
Convert list to string
teams = ["Packers", "49ers", "Ravens", "Patriots"] print ", ".join(teams) >>> 'Packers, 49ers, Ravens, Patriots'
Get elements from the dictionary
I admit that try/except code is not elegant, but here is a simple method, try to find the key in the dictionary, and if the corresponding alue is not found, use the second parameter to set its variable value.
data = {'user': 1, 'name': 'Max', 'three': 4} try: is_admin = data['admin'] except KeyError: is_admin = False
替换诚这样:
data = {'user': 1, 'name': 'Max', 'three': 4} is_admin = data.get('admin', False)
Get a subset of the list
Sometimes, you only need part of a list. Here are some ways to get a subset of a list.
x = [1,2,3,4,5,6] #前3个 print x[:3] >>> [1,2,3] #中间4个 print x[1:5] >>> [2,3,4,5] #最后3个 print x[3:] >>> [4,5,6] #奇数项 print x[::2] >>> [1,3,5] #偶数项 print x[1::2] >>> [2,4,6]
60 characters to solve FizzBuzz
Some time ago, Jeff Atwood promoted a simple programming exercise called FizzBuzz. The question is quoted as follows:
Write a program that prints the numbers 1 to 100, replacing the number with "Fizz" for multiples of 3, "Buzz" for multiples of 5, and "FizzBuzz" for numbers that are both multiples of 3 and 5.
Here is a short, interesting way to solve this problem:
for x in range(101):print"fizz"[x%3*4::]+"buzz"[x%5*4::]or x
gather
In addition to Python's built-in data types, the collection module also includes some special use cases. Counter is very practical in some situations. If you participated in this year's Facebook HackerCup, you can even find its practicality.
from collections import Counter print Counter("hello") >>> Counter({'l': 2, 'h': 1, 'e': 1, 'o': 1})
Iteration tools
Like the collections library, there is also a library called itertools, which can really solve certain problems efficiently. One use case is to find all combinations, which can tell you all the impossible combinations of elements in a group
from itertools import combinations teams = ["Packers", "49ers", "Ravens", "Patriots"] for game in combinations(teams, 2): print game >>> ('Packers', '49ers') >>> ('Packers', 'Ravens') >>> ('Packers', 'Patriots') >>> ('49ers', 'Ravens') >>> ('49ers', 'Patriots') >>> ('Ravens', 'Patriots')
False == True
This is a very interesting thing compared to practical technology. In python, True and False are global variables, so:
False = True if False: print "Hello" else: print "World" >>> Hello
If you have any other cool tricks, you can leave a message below, thanks for reading.
The above is the detailed content of Introducing techniques to Python beginners. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
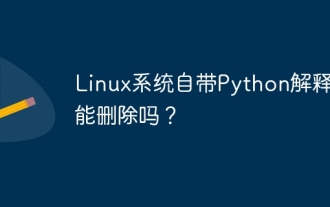
Regarding the problem of removing the Python interpreter that comes with Linux systems, many Linux distributions will preinstall the Python interpreter when installed, and it does not use the package manager...
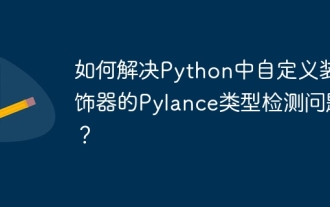
Pylance type detection problem solution when using custom decorator In Python programming, decorator is a powerful tool that can be used to add rows...
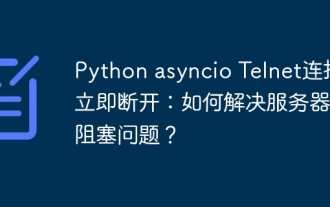
About Pythonasyncio...
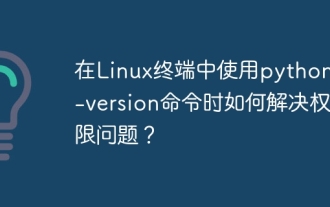
Using python in Linux terminal...
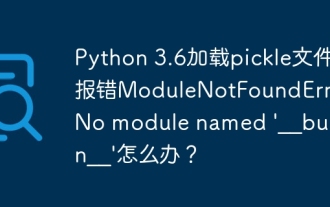
Loading pickle file in Python 3.6 environment error: ModuleNotFoundError:Nomodulenamed...
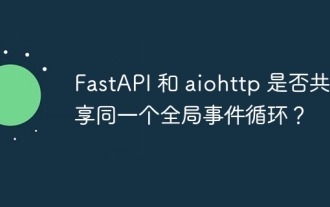
Compatibility issues between Python asynchronous libraries In Python, asynchronous programming has become the process of high concurrency and I/O...
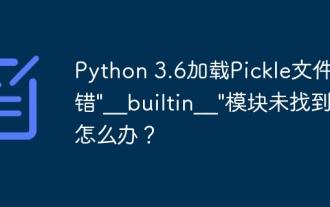
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
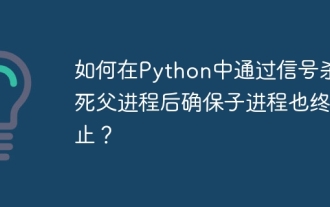
The problem and solution of the child process continuing to run when using signals to kill the parent process. In Python programming, after killing the parent process through signals, the child process still...
