Detailed explanation of Java's method of handling button click events
The following editor will bring you a method of handling button click events in Java. The editor thinks it’s pretty good, so I’ll share it with you now and give it as a reference. Let’s follow the editor and take a look.
Different event sources can generate different types of events. For example, a button can send an ActionEvent object, and a window can send a WindowEvent object.
Overview of the AWT time processing mechanism:
1. The listener object is an object that implements a specific An instance of the listener interface class.
#2. The event source is an object that can register listener objects and send event objects.
#3. When an event occurs, the event source passes the event object to all registered listeners.
#4. The listener object will use the information in the event object to decide how to respond to the event.
Here is an example of a listener:
##
ActionListener listener = ...; JButton button = new JButton("OK"); button.addActionListener(listener);
class MyListener implements ActionListener { ...; public void actionPerformed(ActionEvent event) { //reaction to button click goes here } }
Example: Processing button click events
In order to deepen the understanding of the event delegation model, let’s take a simple example of responding to a button click event. Explain the details you need to know. In this example, you want to place three buttons in a panel and add three listener objects to act as action listeners for the buttons. In this case, as long as the user clicks any button on the panel, the related listener object will receive an ActionEvent object, which indicates that a button has been clicked. In the sample program, the listener object changes the background color of the panel. Before demonstrating how to listen to button click events, we first need to explain how to create buttons and how to add them to the panel. A button can be created by specifying a label string, an icon, or both in the button constructor. Here are two examples:JButton yellowButton = new JButton("Yellow"); JButton blueButton = new JButton(new ImageIcon("blue-ball.gif"));
JButton yellowButton = new JButton("Yellow"); JButton blueButton = new JButton("Blue"); JButton redButton = new JButton("Red"); buttonPanel.add(yellowButton); buttonPanel.add(blueButton); buttonPanel.add(redButton);
class ColorAction implements ActionListener { public ColorAction(Color c) { backgroundColor = c; } public void actionPerformed(actionEvent event) { //set panel background color } private Color backgroundColor; }
ColorAction yelloAction = new ColorAction(Color.YELLOW); ColorAction blueAction = new ColorAction(Color.BLUE); ColorAction redAction = new ColorAction(Color.RED); yellowButton.addActionListener(yellowAction); blueButton.addActionListener(blueAction); redButton.addActionListener(redAction);
class ButtonFrame extends JFrame { ... private class ColorAction implents ActionListener { ... public void actionPerformed(ActionEvent event) { buttonPanel.setBackground(backgroundColor); } private Color backgroundColor; } private Jpanel buttonPanel; }
The above is the detailed content of Detailed explanation of Java's method of handling button click events. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
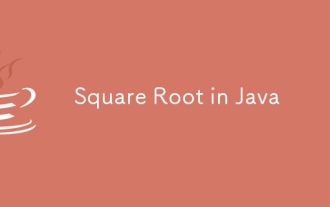
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
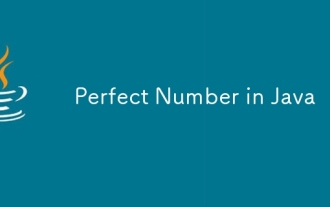
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
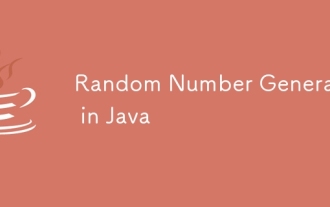
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
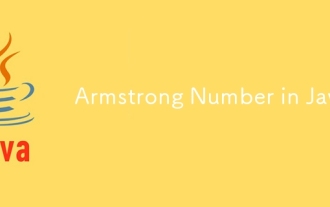
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
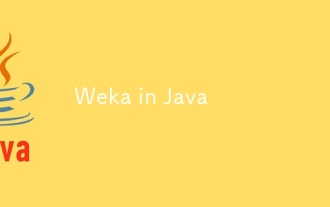
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
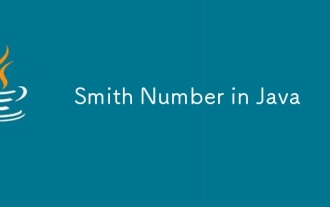
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
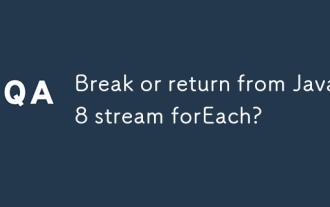
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
