Detailed explanation of function Decorator examples in JavaScript
This article mainly introduces the JavaScript decorator function (Decorator), and analyzes the function, implementation and usage of the JavaScript decorator function (Decorator) in the form of examples. Friends in need can refer to this article
The example describes the Javascript decorator function (Decorator). Share it with everyone for your reference, the details are as follows:
The decorator function (Decorator) is used to dynamically add a certain function, responsibility, etc. to the object during runtime. Compared with extending the functions of objects through inheritance, decorators are more flexible. First, we can dynamically select a decorator for the object instead of hardcore inheriting the object to implement a certain function point. Secondly: The inheritance method may lead to a large number of subclasses, which is a bit redundant just to add a single function point.
The following are several commonly used decorator function examples. Please check github for related codes.
1 Dynamically add onload listening function
function addLoadEvent(fn) { var oldEvent = window.onload; if(typeof window.onload != 'function') { window.onload = fn; }else { window.onload = function() { oldEvent(); fn(); }; } } function fn1() { console.log('onloadFunc 1'); } function fn2() { console.log('onloadFunc 2'); } function fn3() { console.log('onloadFunc 3'); } addLoadEvent(fn1); addLoadEvent(fn2); addLoadEvent(fn3);
2 Pre-execution function and post-execution function
Function.prototype.before = function(beforfunc) { var self = this; var outerArgs = Array.prototype.slice.call(arguments, 1); return function() { var innerArgs = Array.prototype.slice.call(arguments); beforfunc.apply(this, innerArgs); self.apply(this, outerArgs); }; }; Function.prototype.after = function(afterfunc) { var self = this; var outerArgs = Array.prototype.slice.call(arguments, 1); return function() { var innerArgs = Array.prototype.slice.call(arguments); self.apply(this, outerArgs); afterfunc.apply(this, innerArgs); }; }; var func = function(name){ console.log('I am ' + name); }; var beforefunc = function(age){ console.log('I am ' + age + ' years old'); }; var afterfunc = function(gender){ console.log('I am a ' + gender); }; var beforeFunc = func.before(beforefunc, 'Andy'); var afterFunc = func.after(afterfunc, 'Andy'); beforeFunc('12'); afterFunc('boy');
The execution result is printed on the console as follows:
I am 12 years old I am Andy I am Andy I am a boy
3 Function execution time calculation
function log(func){ return function(...args){ const start = Date.now(); let result = func(...args); const used = Date.now() - start; console.log(`call ${func.name} (${args}) used ${used} ms.`); return result; }; } function calculate(times){ let sum = 0; let i = 1; while(i < times){ sum += i; i++; } return sum; } runCalculate = log(calculate); let result = runCalculate(100000); console.log(result);
Note: I used ES2015 (ES6) syntax here. If you are interested, you can check the previous related content about ES6.
Of course, decorator functions are not limited to these uses. The Nodejs framework Koa used by Tmall is based on decorator functions and ES2015 Generator. I hope this article can serve as a starting point for you to write more elegant JS code.
The above is the detailed content of Detailed explanation of function Decorator examples in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


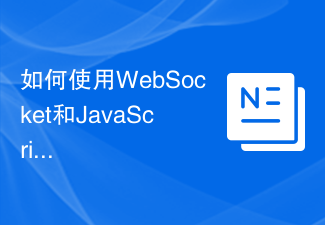
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
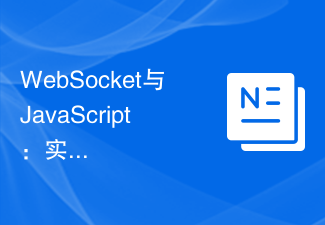
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
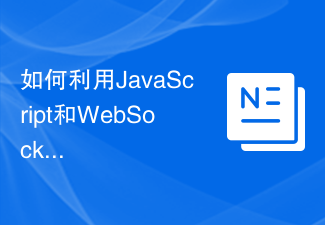
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
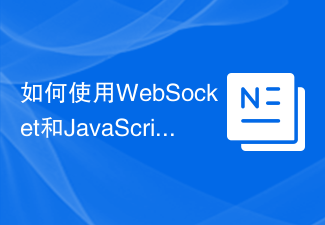
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
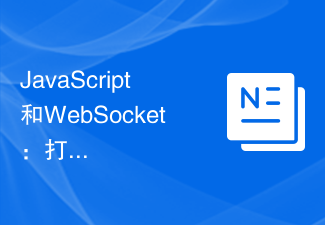
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
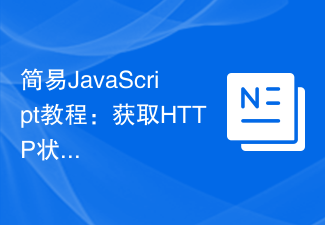
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
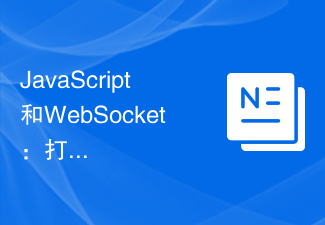
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
