Detailed process of implementing car rental system in Java
This article mainly introduces you to the steps of using Java to implement a Dada car rental system. The article gives detailed implementation ideas and sample codes, and provides complete source code at the end for everyone to learn and download. If needed Friends can refer to it, let’s take a look below.
This article introduces the use of Java to write a console version of the "Dada Car Rental System". I won't say much below, let's take a look at the detailed implementation method.
Achieve the goal
Write a console version of "Dada Car Rental System" in java
Realize function
## 1. Display all available rental vehiclesThree major analyzes
Data model analysis
Business model analysis
Display and process analysis
##Achievement effect
Car rental page
First define a Car class, which contains basic functions: car name, number of passengers, cargo capacity, and daily rental. Then create three subclasses, namely passenger car class, truck class and pickup truck class (which can carry both passengers and cargo). They all inherit
Car class. Finally, a main class is needed to start the entire system and call each subclass. Implementation codepackage com.jinger;
public abstract class Car {
public int rent;//日租金
public int people;//载客人数
public int loads;//载货量
public String name;//车名
public int getRent(){
return rent;
}
public void setRent(int rent){
this.rent=rent;
}
public int getPeople(){
return people;
}
public void setPeople(int people){
this.people=people;
}
public int getLoads(){
return loads;
}
public void setLoads(int loads){
this.loads=loads;
}
public String getName(){
return name;
}
public void setName(String name){
this.name=name;
}
}
package com.jinger; public class PassageCar extends Car{ public PassageCar(String name,int people,int rent){ this.setName(name); this.setPeople(people); this.setRent(rent); } public String toString(){ return this.getName()+"\t"+this.getPeople()+"\t\t\t\t"+this.getRent(); } }
package com.jinger; public class Truck extends Car { public Truck(String name,int loads,int rent){ this.setName(name); this.setLoads(loads); this.setRent(rent); } public String toString(){ return this.getName()+"\t\t\t"+this.getLoads()+"\t\t"+this.getRent(); } }
package com.jinger; public class Pickup extends Car { public Pickup(String name,int people,int loads,int rent){ this.setName(name); this.setPeople(people); this.setLoads(loads); this.setRent(rent); } public String toString(){ return this.getName()+"\t"+this.getPeople()+"\t\t"+this.getLoads()+"\t\t"+this.getRent(); } }
package com.jinger; import java.util.*; public class Initial { public static void main(String[] args) { //对各类车实例化并保存到cars数组 Car[] cars={ new PassageCar("奥迪A4",4,500), new PassageCar("马自达6",4,400), new Pickup("皮卡雪6",4,2,450), new PassageCar("金龙",20,800), new Truck("松花江",4,400), new Truck("依维柯",20,1000)}; System.out.println("****欢迎使用达达租车系统!****"); System.out.println("****您确认租车吗?****"+"\n"+"是(请输入1) \t 否(请输入2)"); Scanner in1=new Scanner(System.in); int is=in1.nextInt(); if(is!=1){ System.out.println("****欢迎下次光临!****"); System.exit(0); } if(is==1){ System.out.println("****您可租车的类型及价目表****"); System.out.println("序号"+"\t车名"+"\t载客数(人)"+"\t载货量(吨)"+"\t日租金(元/天)"); //使用循环方式将各类车输出 for(int i=0;i<cars.length;i++){ System.out.println((i+1)+"\t"+cars[i]); } System.out.println("****请输入您的租车数量:****"); int num1=in1.nextInt(); Car[] rentcar=new Car[num1]; int price=0;//总价格 int totalpeople=0;//总人数 int totalloads=0;//总载货量 for(int i=0;i<num1;i++){ System.out.println("****请输入第"+(i+1)+"辆车的序号:****"); int numx=in1.nextInt(); rentcar[i]=cars[numx-1]; } System.out.println("****请输入天数:****"); int day=in1.nextInt(); for(int i=0;i<num1;i++){ price=price+rentcar[i].rent *day; } System.out.println("****您的账单:****"); System.out.println("已选载人车:"); for(int i=0;i<num1;i++){ if(rentcar[i].people!=0){ System.out.println(rentcar[i].name+"\t"); } totalpeople=totalpeople+rentcar[i].people; } System.out.println('\n'); System.out.println("已选载货车:"); for(int i=0;i<num1;i++){ if(rentcar[i].loads!=0){ System.out.println(rentcar[i].name+"\t"); } totalloads=totalloads+rentcar[i].loads; } System.out.println('\n'); System.out.println("共载客:"+totalpeople+"人"); System.out.println("共载货:"+totalloads+"吨"); System.out.println("租车总价格:"+price+"元"); System.out.println('\n'); System.out.println("****感谢您的惠顾,欢迎再次光临!****"); } } }
The idea determines the encoding.
Programming should focus on a top-down, step-by-step design approach.
1.
Java free video tutorial
2. Comprehensive analysis of Java annotations
3. FastJson Tutorial Manual
The above is the detailed content of Detailed process of implementing car rental system in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


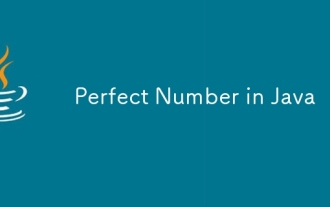
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
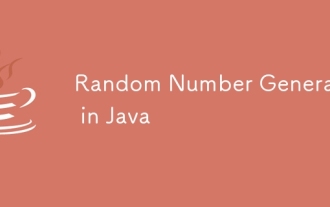
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
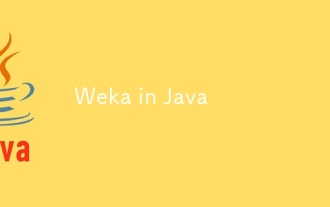
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
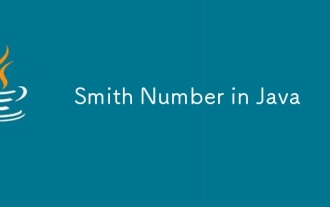
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
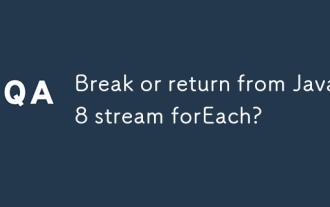
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
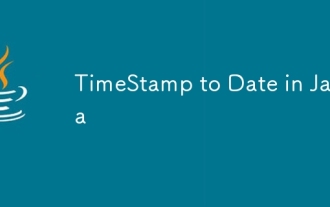
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
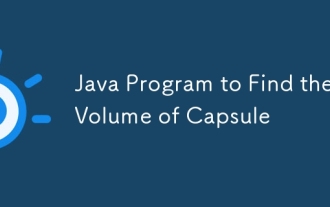
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
