


Detailed explanation of examples of J2ME 3D graphics technology
3D graphics technology has been increasingly used in various fields, of course, this also includes the J2ME field. J2ME provides us with an optional package such as JSR184, which is a set of APIs that implement 3D graphics programming on mobile phones. At the same time, with the development of mobile device hardware, there are now more and more mobile phones that support this optional package, such as Sony Ericsson's K series, S series, etc.
It just so happened that I briefly studied 3D graphics some time ago, so I recently started studying Mobile 3D. Here I will share what I have learned with everyone. I hope it can be helpful to everyone. I also hope that everyone can learn JSR184 together.
Let’s get down to business. First, let’s imagine how we observe the world in real life. We observe through our eyes, and we live in a world composed of a three-dimensional coordinate system. In Mobile3D, there is also a World class that allows you to construct the world you want as you wish. Of course, it is more professional here. In 3D graphics, we call it "scene"; there is also a Camera class to serve as your eyes. You can Set its position, angle and other parameters to display different images.
How to realize the display of 3D images in Mobile 3D? First, you need to create or load a 3D model, then set a series of parameters such as the environment and rendering method in the scene as needed, and then generate and set up a camera and adjust the light you want. Adjust the position and angle you need. OK, what else do you need? Press the shutter, this step is called "rendering" and everything is done. It sounds simple and is actually not difficult. In fact, it is not difficult either.
Let’s explain these steps step by step:
First of all, let’s talk about the establishment of the model. In Mobile 3D, it is the same as most 3D programming APIs. There are two ways: 1. Real-time calculation Generate; 2 external modeling import. Since the external modeling import will import the environment information at the same time, I will give you a detailed introduction later. Here I will focus on introducing the "real-time calculation generation" part, which will help you understand the working principle of Mobile 3D. Mobile 3D provides us with two classes, VertexArray and VertexBuffer, which are used to save the vertex information of the 3D model.
Among them, the VertexArray class has more uses and is more flexible. There are three most commonly used uses of this class. 1. Save vertex coordinate information; 2. Save normal information; 3. Save texture information. Someone may ask how this class manages three different things? Let's analyze this class. First, the constructor of this class has 3 parameters: 1. The number of elements to be included in this instance; 2. The number of elements to be included in each element; 3. The number of bytes occupied by each sub-element. . This seems to make it clear why this class can be used for 3 things.
In addition, this class also has a more commonly used method set(int index, int length, short[] array0). This method is used to store data to instances
objects of this class. , the first parameter refers to which element to start from; the second parameter refers to how many elements to set; and the third parameter is the actual setting.
The following is a brief introduction to the VertexBuffer class, which is the class that actually saves the
frame information of polygons. Modify the class to create graphics by setting vertex positions, discovery, and map information. Among them
setPositions(VertexBuffer v, float s, float[]b)
is used to set the vertex position. In this method you will find that there are 3 parameters, the first one is not used As mentioned, it is the coordinate information of the vertex. The last two are used for operations such as coordinate offset. The operation is the following mathematical formula:
v'=v*s+b
Also There is a
setNormals(vertexBuffer norm)
method to set normals. There is also a very important method
setTexCo
ords(int, VertexArray, float, float[])
In addition to the first parameter in this method, the last The three are the same as
setPositions(VertexBuffer v, float s, float[]b)
. The first parameter is the starting element number. Isn’t this a bit abstract? Let me give you an example so that you can understand.
short x = 20; short y = 20; short z = 20; short fx = (short) -x; short fy = (short) -y; short fz = (short) -z; //定点坐标 short[] vert = {x,y,z, fx,y,z, x,fy,z, fx,fy,z, //D fx,y,fz, x,y,fz, fx,fy,fz, x,fy,fz, //C fx,y,z, fx,y,fz, fx,fy,z, fx,fy,fz, //B x,y,fz, x,y,z, x,fy,fz, x,fy,z, //F x,y,fz, fx,y,fz, x,y,z, fx,y,z, //A x,fy,z, fx,fy,z, x,fy,fz, fx,fy,fz}; //E try{vertArray= new VertexArray(vert.length/3,3,2); vertArray.set(0,vert.length/3,vert); }catch( Exception e){System.out. PRint ln("vert");} //发线 byte[] norm = { 0,0,127, 0,0,127, 0,0,127, 0,0,127, 0,0,-127, 0,0,-127, 0,0,-127, 0,0,-127, -127,0,0, -127,0,0, -127,0,0, -127,0,0, 127,0,0, 127,0,0, 127,0,0, 127,0,0, 0,127,0, 0,127,0, 0,127,0, 0,127,0, 0,-127,0, 0,-127,0, 0,-127,0, 0,-127,0}; try{normArray=new VertexArray(norm.length/3,3,1); normArray.set(0,norm.length/3,norm); }catch(Exception e){System.out.println("norm");e.printStackTrace();} //给出顶点们对应 图片 上的点(vert和tex 数组 是一一对应的) short[] tex = { 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1 }; try{ texArray=new VertexArray(tex.length/2,2,2); texArray.set(0,tex.length/2,tex); }catch(Exception e){System.out.println("tex");} //建立正方体 vb=new VertexBuffer(); vb.setPositions(vertArray,1.0f, null ); vb.setNormals(normArray); vb.setTexCoords(0,texArray,1.0f,null);
In the above code, I created all the vertex and face information needed to create a cube, but everyone should note that the corresponding model is not generated here. The reason is that we have not set up other information to generate the model. Let's take a look at the TriangleStripArray class. This class is the information class of the triangles needed to form the surface. Anyone familiar with 3D graphics knows that constructing 3D graphics is through multiple surfaces. To construct a 3D solid, triangular surfaces are a more commonly used method. I won’t elaborate on the specific content here.
Next we also need to set some environment and material information. The classes used here are Appearance, Texture2D, and Material. Let’s look at an example first:
appearnce=new Appearance();
//创建帖图 Texture2D texture=new Texture2D(image2d); texture.setBl end Color(Texture2D.FUNC_DECAL); texture.setWrapping(Texture2D.WRAP_REPEAT,Texture2D.WRAP_REPEAT); texture.setFiltering(Texture2D.FILTER_NEAREST,Texture2D.FILTER_NEAREST); material=new Material(); material.setColor(Material.D IF FUSE, 0xFFFFFFFF); material.setColor(Material.SPECULAR, 0xFFFFFFFF); material.setShininess(100.0f); appearnce.setTexture(0,texture); appearnce.setMaterial(material); mesh=new Mesh(vb,tsa,appearnce); mesh.setAppearance(0,appearnce);
I personally feel that the Appearance class is somewhat similar to the VertexBuffer class, and is also the holder of multiple attributes; one thing to emphasize here is Appearance The settings of the class are far more than what is given above, and there are many settings (such as FOG, which is fog settings). Texture2D is a texture class. Use it to set the texture information, such as whether the texture is tiled. The name Material means the material. Here you can set the "reflectivity", "color" and other information. In addition, here I will also introduce a method of setting rendering parameters
//设置poly模式设置 PolygonMode polygonMode=new PolygonMode(); polygonMode.setShading(PolygonMode.SHADE_SMOOTH); polygonMode.setCulling(PolygonMode.CULL_NONE); //生成外貌 appearnce=new Appearance(); appearnce.setPolygonMode(polygonMode);
Looking at the code just given, it seems simpler than the above, right? In fact, PolygonMode has already done a lot of work for us. The setting is very similar to the use of Poly in 3D MAX.
The code just now also gives a Mesh class, which is the final model we want for this type of material. After establishing the model, we need to create the Camera. In Camera, I will only briefly introduce two methods here: setParallel(float, float, float, float) and setPerspective(float, float, float, float). Let's first look at setParallel(float, float, float, float). This method is to set the Camera's view method to a flat view; the first parameter is to set the height of the viewing angle. Pay attention to the height, not the angle, because Here is the flat view; the second parameter is the aspect ratio of the Camera, for example, our TV is 4:3 and the widescreen movie is 16:9; the third and fourth parameters are the nearest and farthest rendering range respectively. The same setPerspective sets the Camera to a perspective view, which is closer to the observation angle in our daily life. The last three parameters of this method are the same as the last three parameters of setParallel, and the first parameter is Angle, you should not ignore this angle issue here. This angle is an important parameter for calculating projection in perspective.
It seems that everything is set up, but it is not the case. So far we have only prepared all the materials we need. Let's take a look at the management mechanism of Mobile 3D. People who are familiar with 3D graphics know that most 3D software and 3D APIs manage materials through a tree structure. The advantage of this is that each model, model group, camera and other elements As a node, you can set your own rotation axis and other attributes, and you can move according to the animation information you set. In Mobile 3D, we stipulate that the root node of the tree structure must be an instance object of the World class. The camera and light are special and can not be placed in this tree, but set through objects of the Graphics3D class
【Related Recommendations】
3. Li Yanhui XHTML video tutorial
The above is the detailed content of Detailed explanation of examples of J2ME 3D graphics technology. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


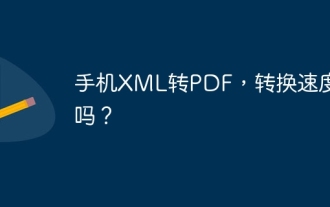
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
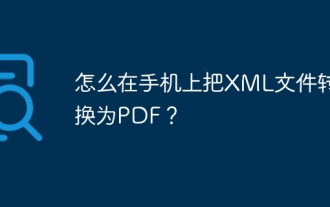
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
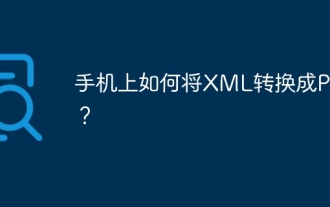
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
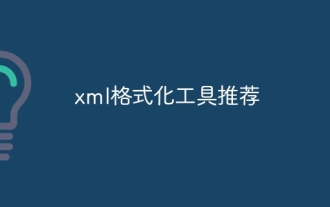
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
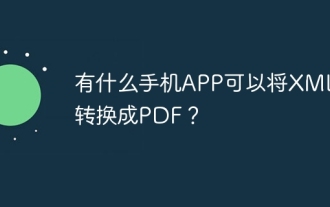
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.

To open a web.xml file, you can use the following methods: Use a text editor (such as Notepad or TextEdit) to edit commands using an integrated development environment (such as Eclipse or NetBeans) (Windows: notepad web.xml; Mac/Linux: open -a TextEdit web.xml)
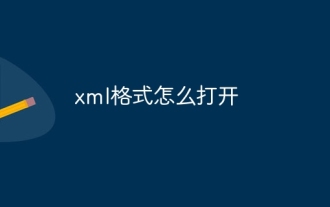
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
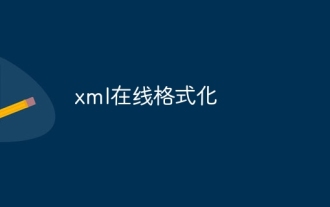
XML Online Format Tools automatically organizes messy XML code into easy-to-read and maintain formats. By parsing the syntax tree of XML and applying formatting rules, these tools optimize the structure of the code, enhancing its maintainability and teamwork efficiency.
