


Example code sharing on how to determine whether two strings are mutually rotated words in Java
This article mainly introduces the relevant knowledge to determine whether string a and b are mutually rotated words, and has a good reference value. Let’s take a look at it with the editor
Rotation word: The new string formed by moving any part of the string str to the back is called the rotation word of the string str.
For example, the rotation words of abc include abc, acb, cba,...
To determine whether str1 and str2 are rotation words for each other, the optimal solution can be that the time complexity is O(n) (n is the length of the string)
The method is as follows:
1. Determine whether the lengths are equal
2. If the lengths are equal, construct a large string, str1+str1 (str1+str1 contains all the rotated words of str1)
3. Use KPM algorithm to determine whether a large string contains str2
The following is the specific algorithm implementation. You must first understand the KPM algorithm
package k; import java.util.Scanner; public class test1 { static int[] next; //next数组 static String str1; //字符串str1 static String str2; //字符串str2 static String str; //字符串str=str1+str1 public static void main(String[] args) { Scanner in = new Scanner(System.in); str1 = in.next(); //获取输入的第一个字符串 str2 = in.next(); //获取输入的第二个字符串 if (str1.length() != str2.length()) //如果长度不相等,那么就肯定不是互为旋转词 System.out.println(str1 + "与" + str2 + "不是互为旋转词"); else { str = str1 + str1; makeNext(); //构建next数组 check(); //判断是否为旋转词 } } private static void check() { int i = 0; int j = 0; while (i < str2.length() && j < str.length()) if (i == -1 || str2.charAt(i) == str.charAt(j)) { i++; j++; } else { i = next[i]; } if (i >= str2.length()) System.out.println(str1 + "与" + str2 + "互为旋转词"); else System.out.println(str1 + "与" + str2 + "不是互为旋转词"); } private static void makeNext() { next = new int[str2.length()]; int i = 0; int k = -1; next[0] = -1; while (i < str2.length() - 1) { while (k >= 0 && str2.charAt(i) != str2.charAt(k)) k = next[k]; i++; k++; if (str2.charAt(i) == str2.charAt(k)) next[i] = next[k]; else next[i] = k; } } }
The above is the detailed content of Example code sharing on how to determine whether two strings are mutually rotated words in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


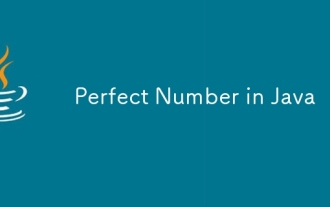
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
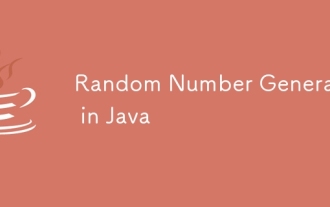
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
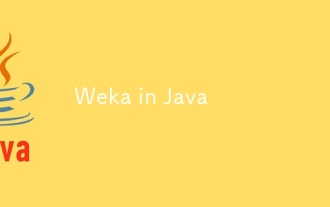
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
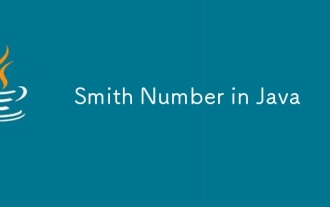
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
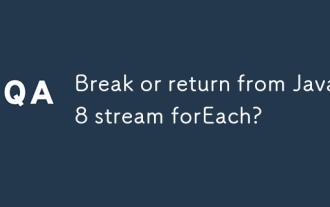
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
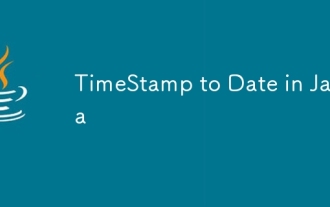
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
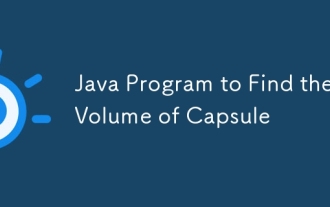
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
