Detailed introduction to Python timing related operations
This article mainly introduces Python timing related operations, involving time, datetime module usage skills, including Timestamp, time difference, date format and other operation methods, friends in need can refer to the following
The examples in this article describe Python timing related operations. Share it with everyone for your reference, the details are as follows:
Content directory:
1. Timestamp
2. Current time
3. Time difference
4. Time and date formatting symbols in python
5. Example
1. Timestamp
The timestamp is since 1970 The total number of seconds from January 1st (08:00:00 GMT) to the current time. It is also called Unix Timestamp, which can be seen everywhere in the world of Unix and C; the common form is a floating point number, with milliseconds after the decimal point. The subtraction of two timestamps is the time interval (unit: seconds).
Example:
import time time1 = time.time() time.sleep(15) time2 = time.time() print time2 - time1
Among them, time.sleep() is the sleep function, unit: seconds.
2. Current time
>>> import datetime,time >>> now = time.strftime("%Y-%m-%d %H:%M:%S") >>> print now 2016-04-30 17:02:26 >>> now = datetime.datetime.now() >>> print now
3. Time difference
#1 Yesterday 00:00 Until yesterday 23:59
>>> import datetime >>> yestoday = datetime.datetime.now() - datetime.timedelta(days=1) >>> t1 = "%s-00-00-00" % yestoday.strftime("%Y-%m-%d") >>> t2 = "%s-23-59-59" % yestoday.strftime("%Y-%m-%d") >>> print 't1', t1 t1 2016-04-29-00-00-00 >>> print 't2', t2 t2 2016-04-29-23-59-59
#2 Now 10 hours in the future
>>> d1 = datetime.datetime.now() >>> d3 = d1 + datetime.timedelta(hours=10) >>> d3.ctime() 'Sun May 1 03:09:58 2
#3 The number of seconds and microseconds for such a while (note that the seconds and microseconds are taken, not equivalent Conversion)
>>> import datetime >>> starttime = datetime.datetime.now() >>> endtime = datetime.datetime.now() >>> starttime = datetime.datetime.now() >>> endtime = datetime.datetime.now() >>> print endtime - starttime 0:00:07.390988 >>> print (endtime - starttime).seconds 7 >>> print (endtime - starttime).microseconds 390988
Time stamp of the file
>>> import os >>> statinfo=os.stat(r"C:/1.txt") >>> statinfo (33206, 0L, 0, 0, 0, 0, 29L, 1201865413, 1201867904, 1201865413)
Note: Use the return value of os.stat. The last three items in statinfo are the st_atime (access time), st_mtime (modification time) of the file, st_ctime (creation time), for example, get the file modification time:
>>> statinfo.st_mtime 1201865413.8952832
Note: This time is a linux timestamp, which can be converted into an easy-to-understand format:
>>> import time >>> time.localtime(statinfo.st_ctime) (2008, 2, 1, 19, 30, 13, 4, 32, 0)
Note: 19:30:13 on February 1, 2008 (2008-2-1 19:30:13)
##4. Time and date formatting symbols in python
%y Two-digit year representation (00-99) %Y Four-digit year representation (000-9999)
%m Month ( 01-12)
%d One day in the month (0-31)
%H 24-hour hour (0-23)
%I 12-hour hour (01-12)
%M Minutes (00=59)
%S Seconds (00-59)
%a Local simplified week name
%A Local complete week name
%b Local simplified month name
%B Local complete month name
%c Local corresponding date representation and time representation
%j Day within the year (
001-366) %p Local A.M. or Equivalent of P.M.
%U The number of weeks in a year (00-53) Sunday is the beginning of the week
%w The week (0-6), Sunday is the beginning of the week
%W Year The week number in (00-53) Monday is the beginning of the week
%x The corresponding local date representation
%X The corresponding local time representation
%Z The name of the current time zone
%% % The number itself
5. Example
#! coding:utf-8 ''''' 日期相关的操作 ''' from datetime import datetime from datetime import timedelta import calendar DATE_FMT = '%Y-%m-%d' DATETIME_FMT = '%Y-%m-%d %H:%M:%S' DATE_US_FMT = '%d/%m/%Y' ''''' 格式化常用的几个参数 Y : 1999 y :99 m : mouth 02 12 M : minute 00-59 S : second d : day H : hour ''' def dateToStr(date): '''''把datetime类型的时间格式化自己想要的格式''' return datetime.strftime(date, DATETIME_FMT) def strToDate(strdate): '''''把str变成日期用来做一些操作''' return datetime.strptime(strdate, DATETIME_FMT) def timeElement(): '''''获取一个时间对象的各个元素''' now = datetime.today() print 'year: %s month: %s day: %s' %(now.year, now.month, now.day) print 'hour: %s minute: %s second: %s' %(now.hour, now.minute, now.second) print 'weekday: %s ' %(now.weekday()+1) #一周是从0开始的 def timeAdd(): ''''' 时间的加减,前一天后一天等操作 datetime.timedelta([days[, seconds[, microseconds[, milliseconds[, minutes[, hours[, weeks]]]]]]]) 参数可以是正数也可以是负数 得到的对象可以加也可以减 乘以数字和求绝对值 ''' atime = timedelta(days=-1) now = datetime.strptime('2001-01-30 11:01:02', DATETIME_FMT) print now + atime print now - abs(atime) print now - abs(atime)*31 def lastFirday(): today = datetime.today() targetDay = calendar.FRIDAY thisDay = today.weekday() de = (thisDay - targetDay) % 7 res = today - timedelta(days=de) print res def test(): print dateToStr(datetime.today()) print strToDate('2013-01-31 12:00:01') timeElement() timeAdd() lastFirday() if name=='main': test()
Connected to pydev debugger (build 141.1899) 2016-05-18 10:40:26 2013-01-31 12:00:01 year: 2016 month: 5 day: 18 hour: 10 minute: 41 second: 13 weekday: 3 2001-01-29 11:01:02 2001-01-29 11:01:02 2000-12-30 11:01:02 2016-05-13 10:41:37.001000
The above is the detailed content of Detailed introduction to Python timing related operations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
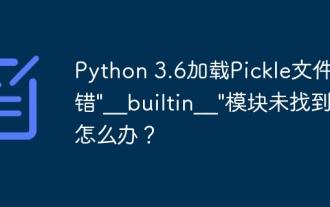
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
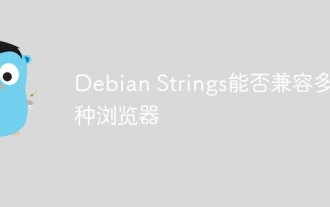
"DebianStrings" is not a standard term, and its specific meaning is still unclear. This article cannot directly comment on its browser compatibility. However, if "DebianStrings" refers to a web application running on a Debian system, its browser compatibility depends on the technical architecture of the application itself. Most modern web applications are committed to cross-browser compatibility. This relies on following web standards and using well-compatible front-end technologies (such as HTML, CSS, JavaScript) and back-end technologies (such as PHP, Python, Node.js, etc.). To ensure that the application is compatible with multiple browsers, developers often need to conduct cross-browser testing and use responsiveness
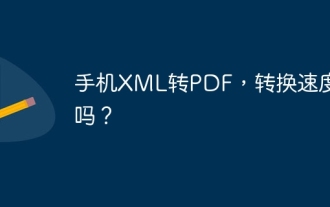
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
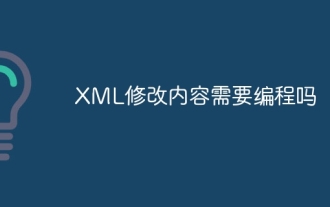
Modifying XML content requires programming, because it requires accurate finding of the target nodes to add, delete, modify and check. The programming language has corresponding libraries to process XML and provides APIs to perform safe, efficient and controllable operations like operating databases.
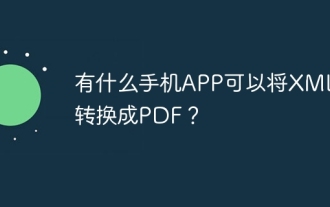
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
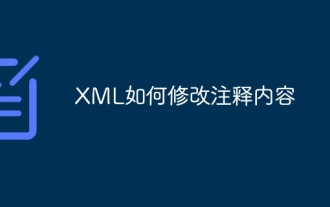
For small XML files, you can directly replace the annotation content with a text editor; for large files, it is recommended to use the XML parser to modify it to ensure efficiency and accuracy. Be careful when deleting XML comments, keeping comments usually helps code understanding and maintenance. Advanced tips provide Python sample code to modify comments using XML parser, but the specific implementation needs to be adjusted according to the XML library used. Pay attention to encoding issues when modifying XML files. It is recommended to use UTF-8 encoding and specify the encoding format.
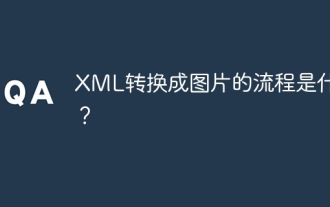
To convert XML images, you need to determine the XML data structure first, then select a suitable graphical library (such as Python's matplotlib) and method, select a visualization strategy based on the data structure, consider the data volume and image format, perform batch processing or use efficient libraries, and finally save it as PNG, JPEG, or SVG according to the needs.
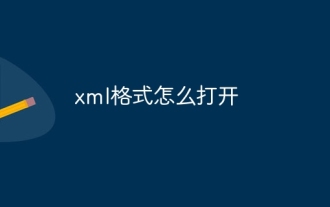
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
