What is ado.net and its simple implementation details
1. Introduction
Ado.net is the data provider of .net framework. It mainly consists of five objects: SqlConnection, SqlCommand, SqlDataAdapter, SqlDataReader and DataSet. The structure is as follows
1.SqlConnection class represents a connection to a sql server database
The connection string format generally has the following two forms, specifically Parameters can refer to msdn
1) Persist Security Info=False;Integrated Security=true;Initial Catalog=Northwind;server=(local)
2) Server=.;Database=demodb;User =sa;Password=123;
Create a connection as follows
SqlConnection conn = new SqlConnection(connString);
2.SqlCommand class represents the database execution command Object
1) Used to set the SQL script or stored procedure, timeout, parameters and transactions that need to be executed.
2) The creation method is as follows
SqlConnection conn = new SqlConnection();
## CreateCommand(); ##
//Method 2
SqlCommand cmd = new
SqlCommand(); cmd.CommandText = "select * from table" ;## cmd.Connection = conn;
3) Several main methods ExecuteNonQuery: Execute sql statements on the connection and return the number of affected rows, mainly performing add, delete and modify operations
ExecuteReader: Execute the query and return the SqlDataReader object
ExecuteScalar: Execute the query and return the first row and column of the result set
3.SqlDataAdapterClass is used to fill DataSet and update database data commands and database connections
This class has 4 constructors as follows
public SqlDataAdapter();
## public SqlDataAdapter(SqlCommand selectCommand);
public SqlDataAdapter(string selectCommandText, SqlConnection selectConnection);
##public SqlDataAdapter(string selectCommandText, string selectConnectionString);
4.SqlDataReaderclass provides a data stream-only way to read
5.DataSetclass represents the cache of data in memory
2. Simple implementation of ADO.NETThe following implements an add, delete, modify, Check the example
public class EasySqlHelper { //web.config来配置 //private static string connString = ConfigurationManager.AppSettings["SqlConnectionString"]; private static string connString = "Server=.;Database=demodb;User=sa;Password=123;"; public static int ExecuteNonQuery(string sql) { using (SqlConnection conn = new SqlConnection(connString)) { using (SqlCommand cmd = new SqlCommand(sql, conn)) { if (conn.State != ConnectionState.Open) { conn.Open(); } return cmd.ExecuteNonQuery(); } } } public static SqlDataReader ExecuteReader(string sql) { SqlConnection conn = new SqlConnection(connString); SqlCommand cmd = new SqlCommand(sql, conn); SqlDataReader rdr = null; try { if (conn.State != ConnectionState.Open) { conn.Open(); } rdr = cmd.ExecuteReader(); } catch (SqlException ex) { conn.Dispose(); cmd.Dispose(); if (rdr != null) { rdr.Dispose(); } throw ex; } finally { cmd.Dispose(); } return rdr; } public static DataTable ExecuteDataTable(string sql) { using (SqlConnection conn = new SqlConnection(connString)) { using (SqlCommand cmd = new SqlCommand(sql, conn)) { if (conn.State != ConnectionState.Open) { conn.Open(); } SqlDataAdapter adp = new SqlDataAdapter(cmd); DataSet ds = new DataSet(); adp.Fill(ds); return ds.Tables[0]; } } } }
The above code uses using to ensure resource release. All classes that implement the IDisposable interface can be released using using, even if it occurs when calling the object's method. Exceptions are also released. 3. Create data source class instances of different providers
The above code is only valid for sql server. If you want to implement different databases such as Oracle, you need to write another one. Set of codes, .Net provides the DbProviderFactory class to create different database instances.
At the same time, the above five major objects should also be replaced with DbConnection, DbCommand, DbDataReader, and DbDataAdapter to abstract the specific sql server objects into more specific objects that have nothing to do with the database type.
//// <summary> /// 连接信息 /// </summary> public class ConnectionInfo { private string _connectionString; private string _providerName; /// <summary> /// 连接字符串 /// </summary> public string ConnectionString { get { return _connectionString; } } /// <summary> /// 提供程序的固定名称 /// </summary> public string ProviderName { get { return _providerName; } } public ConnectionInfo(string connectionString, string providerName) { _connectionString = connectionString; _providerName = providerName; } } public class MySqlHelper { private static DbProviderFactory dbProvider; private static readonly ConnectionInfo connInfo = new ConnectionInfo("Server=.;Database=demodb;User=sa;Password=123;", "System.Data.SqlClient"); private static void GetProvider() { dbProvider = DbProviderFactories.GetFactory(connInfo.ProviderName); } static MySqlHelper() { GetProvider(); } public static int ExecuteNonQuery(string sql, DbParameter[] parameters) { int flag = 0; using (DbConnection conn = dbProvider.CreateConnection()) { conn.ConnectionString = connInfo.ConnectionString; conn.Open(); using (DbCommand cmd = conn.CreateCommand()) { cmd.CommandText = sql; if (parameters != null && parameters.Length > 0) { cmd.Parameters.AddRange(parameters); } flag = cmd.ExecuteNonQuery(); } } return flag; } public static void ExecuteReader(string sql, DbParameter[] parameters, Action<IDataReader> action) { IDataReader rdr = null; using (DbConnection conn = dbProvider.CreateConnection()) { conn.ConnectionString = connInfo.ConnectionString; conn.Open(); using (DbCommand cmd = conn.CreateCommand()) { cmd.CommandText = sql; if (parameters != null && parameters.Length > 0) { cmd.Parameters.AddRange(parameters); } rdr = cmd.ExecuteReader(); action(rdr); rdr.Close(); } } } public static DataTable ExecuteDataTable(string sql, DbParameter[] parameters) { DataTable dt = null; using (DbConnection conn = dbProvider.CreateConnection()) { conn.ConnectionString = connInfo.ConnectionString; conn.Open(); using (DbCommand cmd = conn.CreateCommand()) { cmd.CommandText = sql; if (parameters != null && parameters.Length > 0) { cmd.Parameters.AddRange(parameters); } IDataReader rdr = cmd.ExecuteReader(); dt = new DataTable(); dt.Load(rdr); rdr.Close(); } return dt; } } }
4. Other third-party frameworks
There are Dapper, IBatis.Net, etc., you can refer to them for reference
【Related Recommendations】
1.
ASP.NET Free Video TutorialC#Use Ado.Net to update and add data to Excel tables MethodADO.NET reads the EXCEL implementation code ((c#))ADO.NET calls the stored procedure ado.net connection vs database codeThe above is the detailed content of What is ado.net and its simple implementation details. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


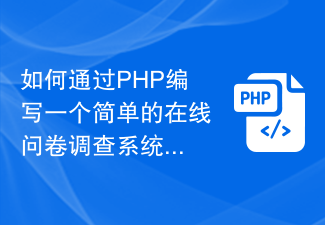
How to write a simple online questionnaire system through PHP. With the development and popularization of the Internet, more and more people are beginning to tend to conduct various surveys and questionnaires through the Internet. In order to meet this demand, we can write a simple online questionnaire system through PHP language. This article will introduce how to use PHP to implement a basic questionnaire system and provide specific code examples. Database design First, we need to design a database to store questionnaire-related data. We can use MySQL data
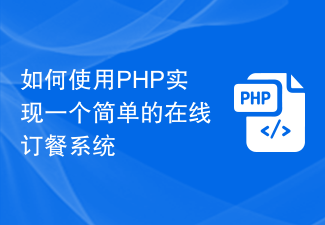
How to use PHP to implement a simple online ordering system. With the popularity of the Internet, the ordering industry is gradually developing online. In order to meet the needs of users, the development of online ordering systems has become very important. This article will introduce how to use PHP language to implement a simple online ordering system and provide specific code examples. Define the database structure First, we need to define the database structure to store order information. Create a data table named "orders" and define the following fields: order_id: order ID
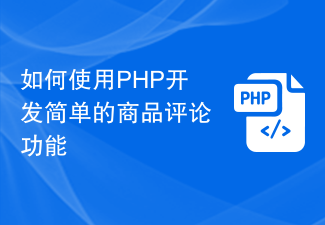
How to use PHP to develop a simple product review function. With the rise of e-commerce, the product review function has become an indispensable function to facilitate communication between users and consumers' evaluation of products. This article will introduce how to use PHP to develop a simple product review function, and attach specific code examples. Create a database First, we need to create a database to store product review information. Create a database called "product_comments" and within it a file called "comment
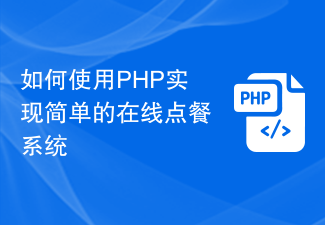
Today, we will implement a simple online ordering system using the PHP programming language. PHP is a popular server-side scripting language that is ideal for developing web-based applications. We'll show you how to use PHP to create a simple ordering system where users can select items on the website, add them to their shopping cart, and complete the transaction. Before starting, we need to create a new PHP project and install the necessary dependencies such as database and server environment. We will use MySQ
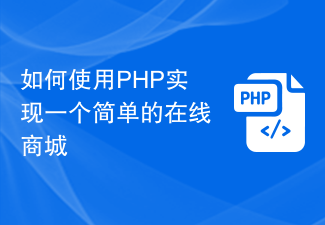
How to use PHP to implement a simple online mall With the development of the Internet, e-commerce has become a common way of shopping. Many people want to learn how to build their own online store. This article will introduce how to use PHP language to implement a simple online mall and give specific code examples. 1. Set up the environment First, we need to set up a PHP development environment locally. It is recommended to use XAMPP or WAMP tools to build an integrated development environment, which can avoid tedious configuration work. 2. Create database connection
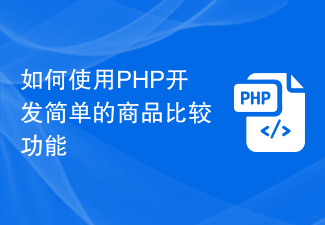
How to use PHP to develop a simple product comparison function requires specific code examples. With the development of e-commerce, users often encounter difficulties in selecting products when shopping, such as not knowing which brand of product to choose is better and which store has the best price. More affordable etc. In order to solve this problem, we can develop a simple product comparison function to help users easily compare the attributes of products and make choices. This article will introduce how to use PHP to implement this function and give specific code examples. First, we need to create a product
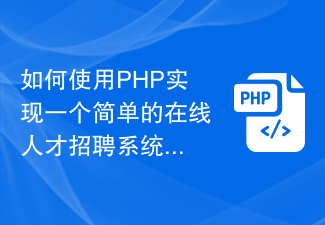
How to use PHP to implement a simple online talent recruitment system Talent recruitment is an important link in enterprise development. With the development of the Internet, more and more companies have begun to adopt online talent recruitment systems to simplify the recruitment process and improve efficiency. In this article, we will introduce how to use PHP language to implement a simple online talent recruitment system and provide specific code examples. 1. Requirements analysis Before implementing the online talent recruitment system, it is first necessary to clarify the system requirements. A simple talent recruitment system usually includes the following functions:
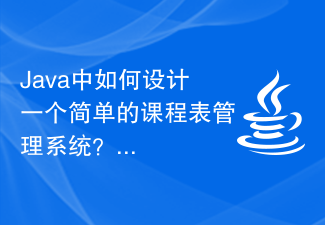
How to design a simple curriculum management system in Java? With the development of education and the diversification of courses, schools and educational institutions need an efficient course management system to handle daily course arrangements and adjustments. As a widely used programming language, Java provides us with a wealth of tools and libraries to design and develop a simple and practical course schedule management system. Before designing a curriculum management system, we need to clarify the functional requirements of the system. Generally speaking, a curriculum management system needs to implement the following functions: Manage courses
