A brief introduction to arrays in JavaScript
Array
(1), definition of array
Array is an ordered collection of values
JavaScript array is untyped; array elements can be of any type, And different elements of the same array may also have different types.
Each value is called an element, and each element has a position in the array.
(2). Create an array.
1. Use array literals to create an array. The simplest way is to separate the array elements with commas in square brackets.
eg:var empty = []; //Array with no elements
eg:var primes = [2,3,4,5,7]; //Array with 5 values Array
eg:var misc = [1.1,true,"a",]; //There are 3 elements of different types and a comma at the end. The value in the array literal does not have to be a constant, it can be anything expression.
eg:var base = 1602;
var table = [base+1,base+2,base+3];
If a certain element in the array literal is omitted value, omitted elements will be assigned the value undefined.
var count = [1,,3]; //The array has 3 elements, and the middle element has a value of undefined
var undefs = [,,]; //The array has two elements elements, all are undefined. The syntax of array literals allows an optional trailing comma, so [,,] has only two elements instead of three.
2. Calling the constructor Array() is another way to create an array. The constructor can be called in three ways.
①There are no parameters when calling: var a = new Array(); This method creates an empty array without any elements, which is equivalent to the array literal [].
②There is a numeric parameter when calling, which specifies the length: var a = new Array(10) When the number of required elements is known in advance, this form of Array() constructor can be used to pre-allocate An array space.
③ Display and specify two or more array elements or a non-numeric element of the array: var a = new Array(5,4,3,2,1,”test”); In this form, The parameters of the constructor will become the elements of the new array. Using array literals is much simpler than using the Array() constructor like this.
(3), Reading and writing of array elements
Use the [] operator to access an element in the array element. Array references are on the left side of the square brackets. Enclosed in square brackets is an arbitrary expression that returns a nonnegative integer value. Use this syntax to both read and write an element of an array.
1. You can access a specific element by specifying the array name and index number. Eg:mycars[0]
2. If you need to modify the value in the existing array, just add a new value to the specified index: Eg:mycars[0]="Opel";
(4), Array length
Definition: The length attribute can set or return the number of elements in the array. (Starting from 1)
The length property of an array is always greater than the subscript of the last element defined in the array
1. For those regular elements that have consecutive elements and start with element 0 For arrays, the length attribute declares the number of elements in the array.
The length property of the array is initialized when the array is created using the constructor Array(). When a new element is added to the array, the value of length is updated if necessary.
Setting the length property can change the size of the array. If set to a value smaller than its current value, the array will be truncated and its trailing elements will be lost. If the set value is greater than its current value, the array will grow and new elements will be added to the end of the array, with their value being undefined.
eg:[].length ==0
[1,2,3].length ==3
(5). Check whether it is an array
1. Use the instanceof operator to determine whether an object is an array
Instanceof is a binary operator. The left operand is an object. If not, it returns false. The right operand is a function object or Function constructor, returns false if not. The principle is to determine whether the prototype chain of the object of the left operand has the prototype attribute of the constructor of the right operand.
eg: arr instanceof Array
2. You can use Array.isArray(arr)
This is a new Array method in ES5, which is a static method of the Array object Function used to determine whether an object is an array.
The above is the detailed content of A brief introduction to arrays in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


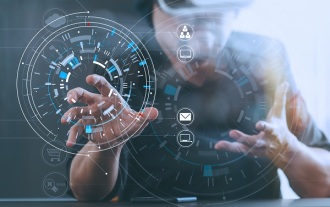
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
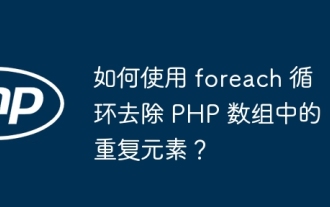
The method of using a foreach loop to remove duplicate elements from a PHP array is as follows: traverse the array, and if the element already exists and the current position is not the first occurrence, delete it. For example, if there are duplicate records in the database query results, you can use this method to remove them and obtain results without duplicate records.

The performance comparison of PHP array key value flipping methods shows that the array_flip() function performs better than the for loop in large arrays (more than 1 million elements) and takes less time. The for loop method of manually flipping key values takes a relatively long time.
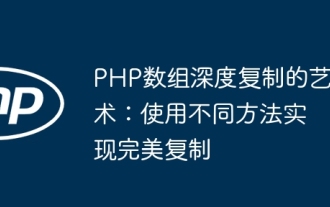
Methods for deep copying arrays in PHP include: JSON encoding and decoding using json_decode and json_encode. Use array_map and clone to make deep copies of keys and values. Use serialize and unserialize for serialization and deserialization.
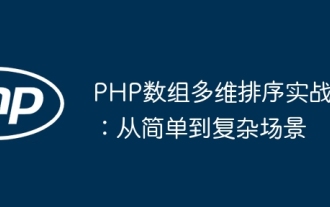
Multidimensional array sorting can be divided into single column sorting and nested sorting. Single column sorting can use the array_multisort() function to sort by columns; nested sorting requires a recursive function to traverse the array and sort it. Practical cases include sorting by product name and compound sorting by sales volume and price.
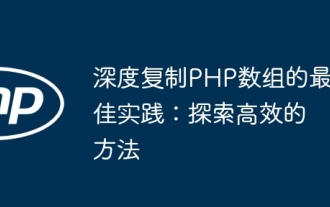
The best practice for performing an array deep copy in PHP is to use json_decode(json_encode($arr)) to convert the array to a JSON string and then convert it back to an array. Use unserialize(serialize($arr)) to serialize the array to a string and then deserialize it to a new array. Use the RecursiveIteratorIterator to recursively traverse multidimensional arrays.
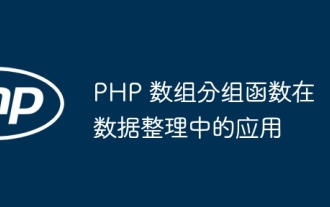
PHP's array_group_by function can group elements in an array based on keys or closure functions, returning an associative array where the key is the group name and the value is an array of elements belonging to the group.
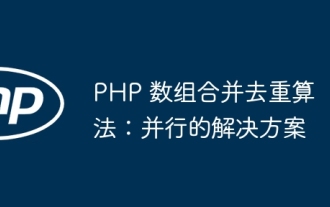
The PHP array merging and deduplication algorithm provides a parallel solution, dividing the original array into small blocks for parallel processing, and the main process merges the results of the blocks to deduplicate. Algorithmic steps: Split the original array into equally allocated small blocks. Process each block for deduplication in parallel. Merge block results and deduplicate again.
