JS--ES 2015/6 new features summary
ES 2015/6 has quite a lot of new content. Here is only an outline (not necessarily comprehensive) list of these features. In fact, there will be a lot of knowledge in every point you dig into. This article aims to summarize it, so it will not conduct in-depth discussion and research on these characteristics. Then, if I have time, I will write a few separate blogs to dig deeper into commonly used points and have in-depth exchanges with everyone.
Arrow function
Arrow function is a short form of function implemented through =>
syntax. Similar syntax is available in C#/JAVA8/CoffeeScript. Unlike functions, arrow functions share the same this
with their execution context. If an arrow function appears inside a function object, it shares arguments
variables with the function.
// Expression bodiesvar odds = evens.map(v => v + 1);var nums = evens.map((v, i) => v + i);// Statement bodiesnums.forEach(v => { if (v % 5 === 0) fives.push(v);});// Lexical thisvar bob = { _name: "Bob", _friends: ['jim'], printFriends() { this._friends.forEach(f => console.log(this._name + " knows " + f)); // Bob knows jim }};// Lexical argumentsfunction square() { let example = () => { let numbers = []; for (let number of arguments) { numbers.push(number * number); } return numbers; }; return example();}square(2, 4, 7.5, 8, 11.5, 21); // returns: [4, 16, 56.25, 64, 132.25, 441]
Class Class
Javascript Class
does not introduce a new object-oriented approach The object inheritance model is based on syntactic sugar of prototypal inheritance. It provides a simpler and clearer syntax for creating objects and handling inheritance.
class Rectangle { constructor(height, width) { this.height = height; this.width = width; }}
The class does not declare promotion, you must ensure that it has been declared before calling.
Constructor constructor
is a special method used to create and initialize instances of a class.
Static methodstatic
The keyword is used to declare a static method
Create a subclassextends
The keyword is used to create a subclass, please note here: extends cannot be used to extend regular objects (non-constructible/non-constructible). If you want to inherit regular objects, you can use Object.setPrototypeOf()
.
Call super classsuper
Keyword can be used to call methods in the parent class
Mix-ins
Mix
Enhancement The object literal
can be implemented in the literal form to define prototype, key-value pair abbreviation, definition method, etc., dynamic attribute names.
var obj = { // Sets the prototype. "__proto__" or '__proto__' would also work. __proto__: theProtoObj, // Computed property name does not set prototype or trigger early error for // duplicate __proto__ properties. ['__proto__']: somethingElse, // Shorthand for ‘handler: handler’ handler, // Methods toString() { // Super calls return "d " + super.toString(); }, // Computed (dynamic) property names [ "prop_" + (() => 42)() ]: 42};
Template string
Template string provides syntactic sugar for constructing strings, in Prel/python, etc. Languages also have similar features.
// Basic literal string creation `This is a pretty little template string.` // Multiline strings `In ES5 this is not legal.` // Interpolate variable bindings var name = "Bob", time = "today"; `Hello ${name}, how are you ${time}?` // Unescaped template strings String.raw`In ES5 "\n" is a line-feed.` // Construct an HTTP request prefix is used to interpret the replacements and construction GET`http://foo.org/bar?a=${a}&b=${b} Content-Type: application/json X-Credentials: ${credentials} { "foo": ${foo}, "bar": ${bar}}`(myOnReadyStateChangeHandler);
Destructuring assignment
The Destructuring method is a Javascript expression, which makes it possible to extract a value from an array or a property from an object into a different variable.
// list matching var [a, ,b] = [1,2,3]; a === 1; b === 3; // object matching (用新变量名赋值) var { op: a, lhs: { op: b }, rhs: c } = getASTNode() // object matching shorthand // binds `op`, `lhs` and `rhs` in scope var {op, lhs, rhs} = getASTNode() // Can be used in parameter position function g({name: x}) { console.log(x); } g({name: 5}) // Fail-soft destructuring var [a] = []; a === undefined; // Fail-soft destructuring with defaults var [a = 1] = []; a === 1; // 变量可以先赋予默认值。当要提取的对象没有对应的属性,变量就被赋予默认值。 var {a = 10, b = 5} = {a: 3}; console.log(a); // 3 console.log(b); // 5 // Destructuring + defaults arguments function r({x, y, w = 10, h = 10}) { return x + y + w + h; } r({x:1, y:2}) === 23 // 对象属性计算名和解构 let key = "z"; let { [key]: foo } = { z: "bar" }; console.log(foo); // "bar"
Default + Rest + Spread
Provide default values for function parameters& ...
Fixed number of parameters
function f(x, y=12) { // y is 12 if not passed (or passed as undefined) return x + y; } f(3) == 15 function f(x, ...y) { // y is an Array return x * y.length; } f(3, "hello", true) == 6 function f(x, y, z) { return x + y + z; } // Pass each elem of array as argument f(...[1,2,3]) == 6
Let + Const
let
is used to declare block-level scope variables. const
is used to declare constants.
function f() { { let x; { // this is ok since it's a block scoped name const x = "sneaky"; // error, was just defined with `const` above x = "foo"; } // this is ok since it was declared with `let` x = "bar"; // error, already declared above in this block let x = "inner"; } }
Iterator
Custom iterators can be created through symbol.iterator.
let fibonacci = { [Symbol.iterator]() { let pre = 0, cur = 1; return { next() { [pre, cur] = [cur, pre + cur]; return { done: false, value: cur } } } } } for (var n of fibonacci) { // truncate the sequence at 1000 if (n > 1000) break; console.log(n); }
Generators
Normal functions use function declarations, while generator functions use function* declarations.
Inside the generator function, there is a syntax similar to return: the keyword yield. The difference between the two is that a normal function can only return once, while a generator function can yield multiple times (of course it can also yield only once). During the execution of the generator, it will pause immediately when encountering a yield expression, and the execution state can be resumed later.
function* quips(name) { yield "你好 " + name + "!"; yield "希望你能喜欢这篇介绍ES6的译文"; if (name.startsWith("X")) { yield "你的名字 " + name + " 首字母是X,这很酷!"; } yield "我们下次再见!"; }
Unicode
// same as ES5.1 "
The above is the detailed content of JS--ES 2015/6 new features summary. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
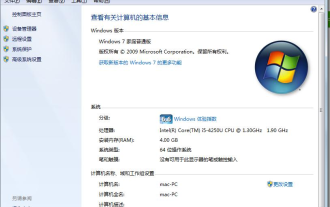
Everyone knows that there are many versions of win7 system, such as win7 ultimate version, win7 professional version, win7 home version, etc. Many users are entangled between the home version and the ultimate version, and don’t know which version to choose, so today I will Let me tell you about the differences between Win7 Family Meal and Win7 Ultimate. Let’s take a look. 1. Experience Different Home Basic Edition makes your daily operations faster and simpler, and allows you to access your most frequently used programs and documents faster and more conveniently. Home Premium gives you the best entertainment experience, making it easy to enjoy and share your favorite TV shows, photos, videos, and music. The Ultimate Edition integrates all the functions of each edition and has all the entertainment functions and professional features of Windows 7 Home Premium.
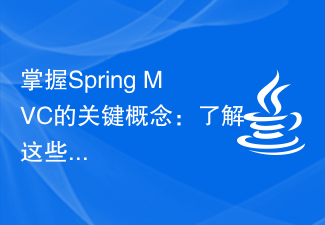
Understand the key features of SpringMVC: To master these important concepts, specific code examples are required. SpringMVC is a Java-based web application development framework that helps developers build flexible and scalable structures through the Model-View-Controller (MVC) architectural pattern. web application. Understanding and mastering the key features of SpringMVC will enable us to develop and manage our web applications more efficiently. This article will introduce some important concepts of SpringMVC
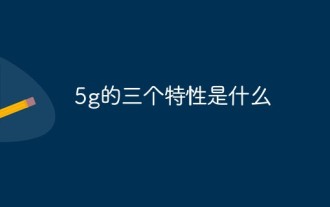
The three characteristics of 5g are: 1. High speed; in practical applications, the speed of 5G network is more than 10 times that of 4G network. 2. Low latency; the latency of 5G network is about tens of milliseconds, which is faster than human reaction speed. 3. Broad connection; the emergence of 5G network, combined with other technologies, will create a new scene of the Internet of Everything.
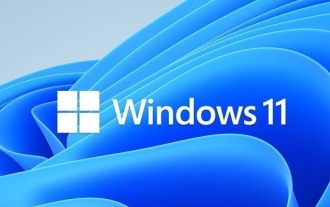
Some people want to update win11, but they don’t know if there are many bugs in win11 and whether the update will cause problems. In fact, there are bugs in win11 now, but they have little impact on use. Are there many bugs in win11? Answer: There are still many bugs in win11. However, these bugs have little impact on daily use. If the user has high requirements for daily use, it is recommended to use it later. Summary of win11 bugs 1. Resource Manager 1. Sometimes memory overflow occurs, resulting in high memory usage of the Resource Manager. 2. This situation will cause the memory to occupy more than 70%, causing the computer to freeze or even crash. 2. Conflict and crash 1. Some applications are not compatible enough, causing conflicts with each other. 2. Although there are relatively few conflict procedures,
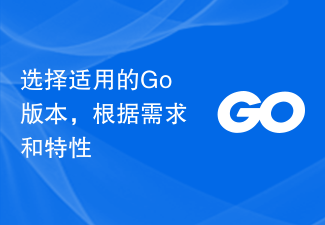
With the rapid development of the Internet, programming languages are constantly evolving and updating. Among them, Go language, as an open source programming language, has attracted much attention in recent years. The Go language is designed to be simple, efficient, safe, and easy to develop and deploy. It has the characteristics of high concurrency, fast compilation and memory safety, making it widely used in fields such as web development, cloud computing and big data. However, there are currently different versions of the Go language available. When choosing a suitable Go language version, we need to consider both requirements and features. head
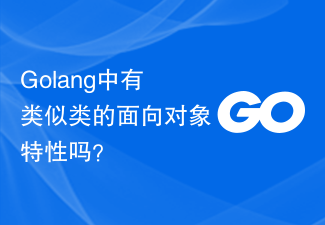
There is no concept of a class in the traditional sense in Golang (Go language), but it provides a data type called a structure, through which object-oriented features similar to classes can be achieved. In this article, we'll explain how to use structures to implement object-oriented features and provide concrete code examples. Definition and use of structures First, let's take a look at the definition and use of structures. In Golang, structures can be defined through the type keyword and then used where needed. Structures can contain attributes
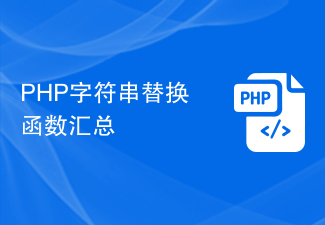
As a powerful programming language, PHP provides a wealth of string processing functions. With the development of the Internet, string processing has increasingly become an indispensable part of Web development. In PHP, the string replacement function is used to search and replace specific text in a string. The following is a summary of commonly used string replacement functions in PHP. str_replace The str_replace function is one of the most commonly used string replacement functions in PHP. It can replace a certain substring in a string. The syntax of this function is as follows
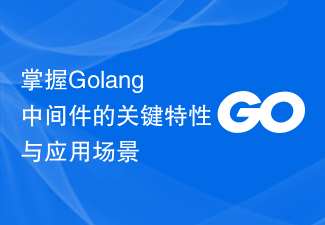
As a fast and efficient programming language, Golang is also widely used in the field of web development. Among them, middleware, as an important design pattern, can help developers better organize and manage code, and improve the reusability and maintainability of code. This article will introduce the key features and application scenarios of middleware in Golang, and illustrate its usage through specific code examples. 1. The concept and function of middleware. As a plug-in component, middleware is located in the request-response processing chain of the application. It is used
