Example introduction of RSA encryption and decryption
RSAsecurity.java
package com.mstf.rsa; import java.security.KeyFactory; import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.PublicKey; import java.security.interfaces.RSAPrivateKey; import java.security.interfaces.RSAPublicKey; import java.security.spec.PKCS8EncodedKeySpec; import java.security.spec.X509EncodedKeySpec; import javax.crypto.Cipher; import com.sun.org.apache.xerces.internal.impl.dv.util.Base64; /*RSA 工具类。提供加密,解密,生成密钥对等方法。 RSA加密原理概述 RSA的安全性依赖于大数的分解,公钥和私钥都是两个大素数(大于100的十进制位)的函数。 据猜测,从一个密钥和密文推断出明文的难度等同于分解两个大素数的积 密钥的产生: 1.选择两个大素数 p,q ,计算 n=p*q; 2.随机选择加密密钥 e ,要求 e 和 (p-1)*(q-1)互质 3.利用 Euclid 算法计算解密密钥 d , 使其满足 e*d = 1(mod(p-1)*(q-1)) (其中 n,d 也要互质) 4:至此得出公钥为 (n,e) 私钥为 (n,d) RSA速度 * 由于进行的都是大数计算,使得RSA最快的情况也比DES慢上100倍,无论 是软件还是硬件实现。 * 速度一直是RSA的缺陷。一般来说只用于少量数据 加密。*/ public class RSAsecurity { public static String src = "admin"; public void priENpubDE() { try { // 初始化秘钥 KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA"); // 秘钥长度 keyPairGenerator.initialize(1024); // 初始化秘钥对 KeyPair keyPair = keyPairGenerator.generateKeyPair(); // 公钥 RSAPublicKey rsaPublicKey = (RSAPublicKey) keyPair.getPublic(); // 私钥 RSAPrivateKey rsaPrivateKey = (RSAPrivateKey) keyPair.getPrivate(); // 2.私钥加密,公钥解密----加密 // 生成私钥 PKCS8EncodedKeySpec pkcs8EncodedKeySpec = new PKCS8EncodedKeySpec(rsaPrivateKey.getEncoded()); KeyFactory keyFactory = KeyFactory.getInstance("RSA"); PrivateKey privateKey = keyFactory.generatePrivate(pkcs8EncodedKeySpec); // Cipher类为加密和解密提供密码功能,通过getinstance实例化对象 Cipher cipher = Cipher.getInstance("RSA"); // 初始化加密 cipher.init(Cipher.ENCRYPT_MODE, privateKey); byte[] result = cipher.doFinal(src.getBytes()); System.out.println("私钥加密,公钥解密----加密:" + Base64.encode(result)); // 3.私钥加密,公钥解密----解密 // 生成公钥 X509EncodedKeySpec x509EncodedKeySpec = new X509EncodedKeySpec(rsaPublicKey.getEncoded()); keyFactory = KeyFactory.getInstance("RSA"); PublicKey publicKey = keyFactory.generatePublic(x509EncodedKeySpec); cipher = Cipher.getInstance("RSA"); // 初始化解密 cipher.init(Cipher.DECRYPT_MODE, publicKey); result = cipher.doFinal(result); System.out.println("私钥加密,公钥解密----解密:" + new String(result)); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void pubENpriDE() { try { // 1.初始化秘钥 KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA"); // 秘钥长度 keyPairGenerator.initialize(512); // 初始化秘钥对 KeyPair keyPair = keyPairGenerator.generateKeyPair(); // 公钥 RSAPublicKey rsaPublicKey = (RSAPublicKey) keyPair.getPublic(); // 私钥 RSAPrivateKey rsaPrivateKey = (RSAPrivateKey) keyPair.getPrivate(); // 2.公钥加密,私钥解密----加密 X509EncodedKeySpec x509EncodedKeySpec = new X509EncodedKeySpec(rsaPublicKey.getEncoded()); KeyFactory keyFactory = KeyFactory.getInstance("RSA"); PublicKey publicKey = keyFactory.generatePublic(x509EncodedKeySpec); // 初始化加密 // Cipher类为加密和解密提供密码功能,通过getinstance实例化对象 Cipher cipher = Cipher.getInstance("RSA"); cipher.init(Cipher.ENCRYPT_MODE, publicKey); // 加密字符串 byte[] result = cipher.doFinal(src.getBytes()); System.out.println("公钥加密,私钥解密----加密:" + Base64.encode(result)); // 3.公钥加密,私钥解密-----解密 PKCS8EncodedKeySpec pkcs8EncodedKeySpec = new PKCS8EncodedKeySpec(rsaPrivateKey.getEncoded()); keyFactory = KeyFactory.getInstance("RSA"); PrivateKey privateKey = keyFactory.generatePrivate(pkcs8EncodedKeySpec); // 初始化解密 cipher.init(Cipher.DECRYPT_MODE, privateKey); // 解密字符串 result = cipher.doFinal(result); System.out.println("公钥加密,私钥解密-----解密:" + new String(result)); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
RSAtest.java
package com.mstf.rsa; import com.mstf.rsa.RSAsecurity; public class RSAtest { public static void main(String[] args) { RSAsecurity rsAsecurity = new RSAsecurity(); System.out.println("私钥加密公钥解密例:"); rsAsecurity.priENpubDE(); System.out.println("公钥加密私钥解密例:"); rsAsecurity.pubENpriDE(); } }
The above is the detailed content of Example introduction of RSA encryption and decryption. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
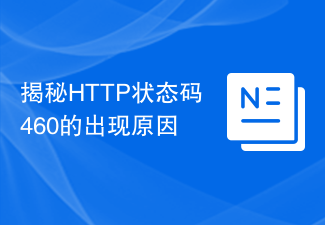
Decrypting HTTP status code 460: Why does this error occur? Introduction: In daily network use, we often encounter various error prompts, including HTTP status codes. These status codes are a mechanism defined by the HTTP protocol to indicate the processing of a request. Among these status codes, there is a relatively rare error code, namely 460. This article will delve into this error code and explain why this error occurs. Definition of HTTP status code 460: First, we need to understand the basics of HTTP status code
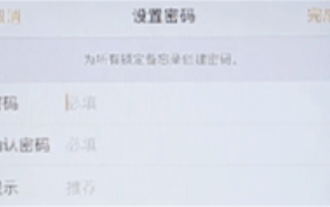
In Apple mobile phones, users can encrypt photo albums according to their own needs. Some users don't know how to set it up. You can add the pictures that need to be encrypted to the memo, and then lock the memo. Next, the editor will introduce the method of setting up the encryption of mobile photo albums for users. Interested users, come and take a look! Apple mobile phone tutorial How to set up iPhone photo album encryption A: After adding the pictures that need to be encrypted to the memo, go to lock the memo for detailed introduction: 1. Enter the photo album, select the picture that needs to be encrypted, and then click [Add to] below. 2. Select [Add to Notes]. 3. Enter the memo, find the memo you just created, enter it, and click the [Send] icon in the upper right corner. 4. Click [Lock Device] below
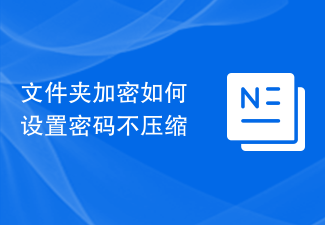
Folder encryption is a common data protection method that encrypts the contents of a folder so that only those who have the decryption password can access the files. When encrypting a folder, there are some common ways to set a password without compressing the file. First, we can use the encryption function that comes with the operating system to set a folder password. For Windows users, you can set it up by following the following steps: Select the folder to be encrypted, right-click the folder, and select "Properties"

In today's work environment, everyone's awareness of confidentiality is getting stronger and stronger, and encryption operations are often performed to protect files when using software. Especially for key documents, the awareness of confidentiality should be increased, and the security of documents should be given top priority at all times. So I don’t know how well everyone understands word decryption. How to operate it specifically? Today we will actually show you the process of word decryption through the explanation below. Friends who need to learn word decryption knowledge should not miss today's course. A decryption operation is first required to protect the file, which means that the file is processed as a protective document. After doing this to a file, a prompt pops up when you open the file again. The way to decrypt the file is to enter the password, so you can directly
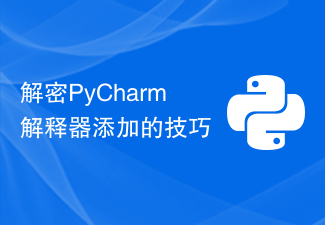
Decrypting the tricks added by the PyCharm interpreter PyCharm is the integrated development environment (IDE) preferred by many Python developers, and it provides many powerful features to improve development efficiency. Among them, the setting of the interpreter is an important part of PyCharm. Correctly setting the interpreter can help developers run the code smoothly and debug the program. This article will introduce some techniques for decrypting the PyCharm interpreter additions, and combine it with specific code examples to show how to correctly configure the interpreter. Adding and selecting interpreters in Py
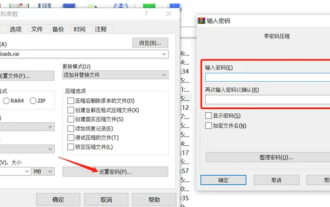
The editor will introduce to you three methods of encryption and compression: Method 1: Encryption The simplest encryption method is to enter the password you want to set when encrypting the file, and the encryption and compression are completed. Method 2: Automatic encryption Ordinary encryption method requires us to enter a password when encrypting each file. If you want to encrypt a large number of compressed packages and the passwords are the same, then we can set automatic encryption in WinRAR, and then just When compressing files normally, WinRAR will add a password to each compressed package. The method is as follows: Open WinRAR, click Options-Settings in the setting interface, switch to [Compression], click Create Default Configuration-Set Password Enter the password we want to set here, click OK to complete the setting, we only need to correct
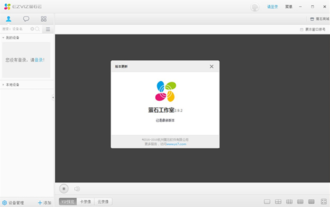
How to de-encrypt videos on EZVIZ Cloud: There are many ways to de-encrypt videos on EZVIZ Cloud, one of which is by using the EZVIZ Cloud Mobile App. Users only need to enter the device list, select the camera to be decrypted and enter the device details page. On the device details page, find the "Settings" option, and then select "Video Encryption" to make relevant settings. In the video encryption settings interface, you can choose the option to turn off video encryption, and save the settings to complete the decryption operation. This simple step allows users to easily decrypt videos and improves the convenience of using the camera. If you use the computer client of EZVIZ Cloud, you can also cancel video encryption through similar steps. Just log in and select the corresponding camera, enter the device details interface, and then look for video addition in the settings.

Original author: Meteor, ChainCatcher Original editor: Marco, ChainCatcher Recently, the full-chain interoperability protocol Analog has entered the public eye with the disclosure of US$16 million in financing. Investment institutions include TribeCapital, NGCVentures, Wintermute, GSR, NEAR, OrangeDAO, and Mike Novogratz’s Alternative asset management companies Samara Asset Group, Balaji Srinivasan, etc. At the end of 2023, Analog caused some excitement in the industry. They released information on the open testnet registration event on the X platform.
