


Example tutorial of implementing crawler with requests and lxml
# requests module to request pages
# lxml module's html build selector selector (formatted response response)
# from lxml import html
# import requests
response = requests.get(url).content
# selector = html.formatstring(response)
hrefs = selector.xpath('/html/body//div[@class='feed-item _j_feed_item']/a/@href' )
# Take url = 'https://www.mafengwo.cn/gonglve/ziyouxing/2033.html' as an example
# python 2.7import requestsfrom lxml import htmlimport os
1 # 获取首页中子页的url链接2 def get_page_urls(url):3 response = requests.get(url).content4 # 通过lxml的html来构建选择器5 selector = html.fromstring(response)6 urls = []7 for i in selector.xpath("/html/body//div[@class='feed-item _j_feed_item']/a/@href"):8 urls.append(i)9 return urls
1 # get title from a child's html(div[@class='title'])2 def get_page_a_title(url):3 '''url is ziyouxing's a@href'''4 response = requests.get(url).content5 selector = html.fromstring(response)6 # get xpath by chrome's tool --> /html/body//div[@class='title']/text()7 a_title = selector.xpath("/html/body//div[@class='title']/text()")8 return a_title
1 # 获取页面选择器(通过lxml的html构建) 2 def get_selector(url): 3 response = requests.get(url).content 4 selector = html.fromstring(response) 5 return selector
# 通过chrome的开发者工具分析html页面结构后发现,我们需要获取的文本内容主要显示在div[@class='l-topic']和div[@class='p-section']中
1 # 获取所需的文本内容2 def get_page_content(selector):3 # /html/body/div[2]/div[2]/div[1]/div[@class='l-topic']/p/text()4 page_title = selector.xpath("//div[@class='l-topic']/p/text()")5 # /html/body/div[2]/div[2]/div[1]/div[2]/div[15]/div[@class='p-section']/text()6 page_content = selector.xpath("//div[@class='p-section']/text()")7 return page_title,page_content
1 # 获取页面中的图片url地址2 def get_image_urls(selector):3 imagesrcs = selector.xpath("//img[@class='_j_lazyload']/@src")4 return imagesrcs
# 获取图片的标题
1 def get_image_title(selector, num)2 # num 是从2开始的3 url = "/html/body/div[2]/div[2]/div[1]/div[2]/div["+num+"]/span[@class='img-an']/text()"4 if selector.xpath(url) is not None:5 image_title = selector.xpath(url)6 else:7 image_title = "map"+str(num) # 没有就起一个8 return image_title
# 下载图片
1 def downloadimages(selector,number): 2 '''number是用来计数的''' 3 urls = get_image_urls() 4 num = 2 5 amount = len(urls) 6 for url in urls: 7 image_title = get_image_title(selector, num) 8 filename = "/home/WorkSpace/tour/words/result"+number+"/+"image_title+".jpg" 9 if not os.path.exists(filename):10 os.makedirs(filename)11 print('downloading %s image %s' %(number, image_title))12 with open(filename, 'wb') as f:13 f.write(requests.get(url).content)14 num += 115 print "已经下载了%s张图" %num
# 入口,启动并把获取的数据存入文件中if __name__ =='__main__': url = ''urls = get_page_urls(url)# turn to get response from htmlnumber = 1for i in urls: selector = get_selector(i)# download images downloadimages(selector,number)# get text and write into a filepage_title, page_content = get_page_content(selector) result = page_title+'\n'+page_content+'\n\n'path = "/home/WorkSpace/tour/words/result"+num+"/"if not os.path.exists(filename): os.makedirs(filename) filename = path + "num"+".txt"with open(filename,'wb') as f: f.write(result)print result
This is the end of the crawler. Before crawling the page, you must carefully analyze the html structure. Some pages are generated by js. This page is relatively simple and does not involve js processing. There will be relevant sharing in future essays
The above is the detailed content of Example tutorial of implementing crawler with requests and lxml. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


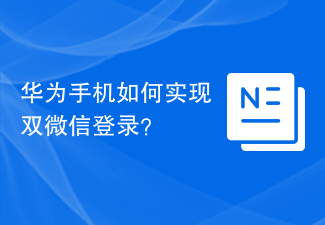
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
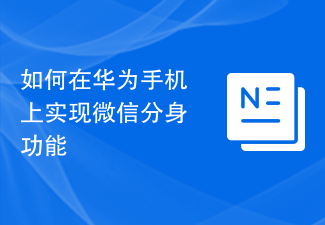
How to implement the WeChat clone function on Huawei mobile phones With the popularity of social software and people's increasing emphasis on privacy and security, the WeChat clone function has gradually become the focus of people's attention. The WeChat clone function can help users log in to multiple WeChat accounts on the same mobile phone at the same time, making it easier to manage and use. It is not difficult to implement the WeChat clone function on Huawei mobile phones. You only need to follow the following steps. Step 1: Make sure that the mobile phone system version and WeChat version meet the requirements. First, make sure that your Huawei mobile phone system version has been updated to the latest version, as well as the WeChat App.
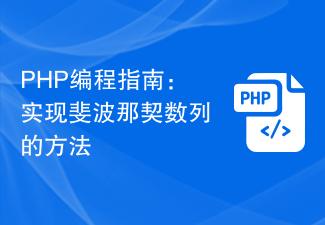
The programming language PHP is a powerful tool for web development, capable of supporting a variety of different programming logics and algorithms. Among them, implementing the Fibonacci sequence is a common and classic programming problem. In this article, we will introduce how to use the PHP programming language to implement the Fibonacci sequence, and attach specific code examples. The Fibonacci sequence is a mathematical sequence defined as follows: the first and second elements of the sequence are 1, and starting from the third element, the value of each element is equal to the sum of the previous two elements. The first few elements of the sequence
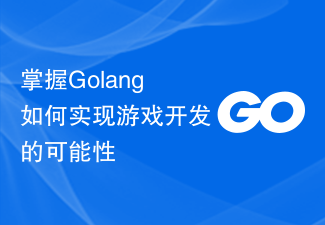
In today's software development field, Golang (Go language), as an efficient, concise and highly concurrency programming language, is increasingly favored by developers. Its rich standard library and efficient concurrency features make it a high-profile choice in the field of game development. This article will explore how to use Golang for game development and demonstrate its powerful possibilities through specific code examples. 1. Golang’s advantages in game development. As a statically typed language, Golang is used in building large-scale game systems.
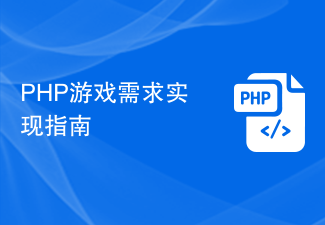
PHP Game Requirements Implementation Guide With the popularity and development of the Internet, the web game market is becoming more and more popular. Many developers hope to use the PHP language to develop their own web games, and implementing game requirements is a key step. This article will introduce how to use PHP language to implement common game requirements and provide specific code examples. 1. Create game characters In web games, game characters are a very important element. We need to define the attributes of the game character, such as name, level, experience value, etc., and provide methods to operate these
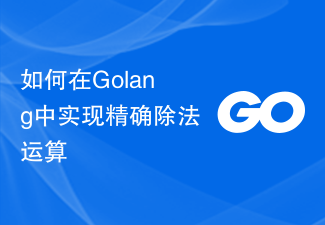
Implementing exact division operations in Golang is a common need, especially in scenarios involving financial calculations or other scenarios that require high-precision calculations. Golang's built-in division operator "/" is calculated for floating point numbers, and sometimes there is a problem of precision loss. In order to solve this problem, we can use third-party libraries or custom functions to implement exact division operations. A common approach is to use the Rat type from the math/big package, which provides a representation of fractions and can be used to implement exact division operations.
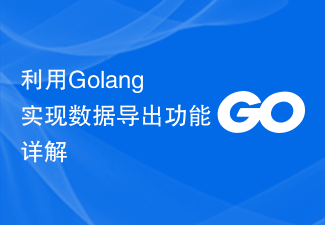
Title: Detailed explanation of data export function using Golang. With the improvement of informatization, many enterprises and organizations need to export data stored in databases into different formats for data analysis, report generation and other purposes. This article will introduce how to use the Golang programming language to implement the data export function, including detailed steps to connect to the database, query data, and export data to files, and provide specific code examples. To connect to the database first, we need to use the database driver provided in Golang, such as da

I'm really sorry that I can't provide real-time programming guidance, but I can provide you with a code example to give you a better understanding of how to use PHP to implement SaaS. The following is an article within 1,500 words, titled "Using PHP to implement SaaS: A comprehensive analysis." In today's information age, SaaS (Software as a Service) has become the mainstream way for enterprises and individuals to use software. It provides a more flexible and convenient way to access software. With SaaS, users don’t need to be on-premises
