How to use Spring Social to connect to social networks
Social networks have become an indispensable part of people's lives today. Popular social networking sites, such as Facebook, LinkedIn, Twitter, and Sina Weibo, have gathered very high popularity and become sites that many people must visit every day. A large amount of information is shared on social networks. For an application, it is necessary to provide support for social networks. Apps can quickly gain popularity through integration with social networks. Integration with social networks mainly has two functions: the first is a relatively simple user login integration, which allows users to log in to the application using existing accounts on social network websites. The advantage of this is that it can save the user the process of re-registering, and it can also establish a connection with the user's existing social network; the second is the in-depth integration method, which allows users to share relevant content in the application to social networks middle. The advantage of this is that it can maintain user stickiness while promoting the application itself.
Integrating social networks into your application is not a complicated task. Many social networking services provide open APIs that allow third-party applications to integrate. Just refer to the relevant documentation to complete. However, the integration itself requires a certain amount of work. The more websites that need to be integrated, the greater the corresponding workload. As part of the Spring framework component family, Spring Social provides an abstraction of social network services and provides related APIs for operations.
The biggest advantage of using Spring Social is that it already provides support for mainstream social networking sites and only requires simple configuration. For some less commonly used social networking sites, you can also find corresponding components from the community. This article uses a sample application to illustrate the usage of Spring Social.
Sample Application
This example allows users to log in to social networking sites to view information about their friends. This is a web application built using the Spring MVC framework. The application uses an embedded H2 database to store data. The front end uses JSP, JSTL and the tag library provided by Spring, and uses Apache Tiles for page layout. Manage user authentication using Spring Security. The page is styled using the Twitter Bootstrap library. The project is built using Gradle. The front-end library is managed through Bower.
The functions provided by the example are relatively simple, mainly including login and registration pages, and the user's homepage. When logging in, users can choose to use an account with a third-party social network. After logging in, you can view friend information on social networks.
Use existing social network accounts to log in
The most basic function integrated with social networks is to allow users to log in using existing accounts. In addition to registering a new account, users can also use existing accounts from other websites to log in. This method is often called connecting to third-party websites. For users, the usual scenario is to choose a third-party social network to connect on the registration or login page. Then jump to the third-party website for login and authorization. After completing the above steps, a new user will be created. During the connection process, the application can obtain the user's profile information from the third-party social networking site to complete the creation of new users. After the new user is created, the user can log in directly using the account of the third-party social network next time.
Basic configuration
Spring Social provides the implementation of Spring MVC controller (Controller) that handles third-party website user login and registration, which can automatically complete the use of social network accounts Login and registration functionality. Before using these controllers, some basic configuration is required. The configuration of Spring Social is completed by implementing the org.springframework.social.config.annotation.SocialConfigurer interface. Part of the SocialConfig class that implements the SocialConfigurer interface is given. The annotation "@EnableSocial" is used to enable Spring Social related functions. The annotation "@Configuration" indicates that this class also contains configuration information related to the Spring framework. The SocialConfigurer interface has three methods that need to be implemented:
addConnectionFactories: This callback method is used to allow the application to add the implementation of the connection factory corresponding to the social network that needs to be supported.
getUserIdSource: This callback method returns an implementation object of the org.springframework.social.UserIdSource interface, which is used to uniquely identify the current user.
getUsersConnectionRepository: This callback method returns an implementation object of the org.springframework.social.connect.UsersConnectionRepository interface, which is used to manage the correspondence between users and social network service providers.
Specific to the sample application, the implementation of the addConnectionFactories method adds LinkedIn's connection factory implementation represented by the org.springframework.social.linkedin.connect.LinkedInConnectionFactory class. In the implementation of the getUserIdSource method, Spring Security is used to obtain the information of the currently logged in user. The getUsersConnectionRepository method creates an implementation object of the database-based JdbcUsersConnectionRepository class. The LinkedInConnectionFactory class is created in a way that reflects the power of Spring Social. You only need to provide the API key applied at LinkedIn to directly use the functions provided by LinkedIn. Details related to OAuth are encapsulated by Spring Social.
1. Use the basic configuration of Spring Social
@Configuration@EnableSocialpublic class SocialConfig implements SocialConfigurer { @Inject private DataSource dataSource; @Override public void addConnectionFactories(ConnectionFactoryConfigurer cfConfig, Environment env) { cfConfig.addConnectionFactory(new LinkedInConnectionFactory(env.getProperty("linkedin.consumerKey"), env.getProperty("linkedin.consumerSecret"))); } @Override public UserIdSource getUserIdSource() { return new UserIdSource() { @Override public String getUserId() { Authentication authentication = SecurityContextHolder.getContext().getAuthentication(); if (authentication == null) { throw new IllegalStateException("Unable to get a ConnectionRepository: no user signed in"); } return authentication.getName(); } }; } @Override public UsersConnectionRepository getUsersConnectionRepository(ConnectionFactoryLocator connectionFactoryLocator) { return new JdbcUsersConnectionRepository(dataSource, connectionFactoryLocator, Encryptors.noOpText()); } }
Login Controller
The next step after configuring Spring Social is to create the corresponding Spring MVC control Server to handle user registration and login. Spring Social already provides corresponding controller implementation, you only need to create an interface to make requests. The first thing to create is a controller for logging in via a third-party social network. As shown, you only need to create a Spring Bean to create the required controller. This controller is bound to the "/signin" URL by default. Each third-party service provider has a corresponding identifier, such as LinkedIn's identifier "linkedin". The service provider's identifier is often included as part of the URL for different controllers. For example, when a user needs to log in through a LinkedIn account, the corresponding URL is "/signin/linkedin". If logging in via a Twitter account, the corresponding URL is "/signin/twitter".
2. Controller for logging in through third-party social networks
@Beanpublic ProviderSignInController providerSignInController(ConnectionFactoryLocator connectionFactoryLocator, UsersConnectionRepository usersConnectionRepository) { return new ProviderSignInController(connectionFactoryLocator, usersConnectionRepository, new SimpleSignInAdapter(new HttpSessionRequestCache())); }
In the above, the SimpleSignInAdapter class implements the org.springframework.social.connect.web.SignInAdapter interface, and its function is Bridge between the ProviderSignInController class and the login logic of the application itself. After the user completes logging in to the third-party website, the user is automatically logged in to the application through the SignInAdapter interface. The implementation of the SimpleSignInAdapter class is shown below.
3. Implementation of SimpleSignInAdapter class
public class SimpleSignInAdapter implements SignInAdapter { private final RequestCache requestCache; @Inject public SimpleSignInAdapter(RequestCache requestCache) { this.requestCache = requestCache; } @Override public String signIn(String localUserId, Connection<?> connection, NativeWebRequest request) { SignInUtils.signin(localUserId); return extractOriginalUrl(request); } private String extractOriginalUrl(NativeWebRequest request) { HttpServletRequest nativeReq = request.getNativeRequest(HttpServletRequest.class); HttpServletResponse nativeRes = request.getNativeResponse(HttpServletResponse.class); SavedRequest saved = requestCache.getRequest(nativeReq, nativeRes); if (saved == null) { return null; } requestCache.removeRequest(nativeReq, nativeRes); removeAutheticationAttributes(nativeReq.getSession(false)); return saved.getRedirectUrl(); } private void removeAutheticationAttributes(HttpSession session) { if (session == null) { return; } session.removeAttribute(WebAttributes.AUTHENTICATION_EXCEPTION); } }
In the code list 3, the signIn method of the SimpleSignInAdapter class calls the signin method of the SignInUtils class to log in. See the code for its implementation. Listing 4. The return value of the signIn method is the jump URL after successful login.
4. Implementation of the signIn method of the SignInUtils class
public class SignInUtils { public static void signin(String userId) { SecurityContextHolder.getContext().setAuthentication(new UsernamePasswordAuthenticationToken(userId, null, null)); } }
Front-end page
You only need to send a POST request to the URL of the login controller through the page to start the process through LinkedIn Go through the login process. As shown in the figure, the login process can be started by submitting the form. The user will first be directed to the authorization page of the LinkedIn website to authorize the application. After the authorization is completed, the user can register.
5. Login page
<form id="linkedin-signin-form" action="signin/linkedin" method="POST" class="form-signin" role="form"> <h2 class="form-signin-heading">Or Connect by</h2> <input type="hidden" name="${_csrf.parameterName}" value="${_csrf.token}"/> <button type="submit" class="btn btn-primary">LinkedIn</button> </form>
By default, when the third-party website authorization is completed, the user will be directed to the page corresponding to the URL "/signup". On this page, users can add some relevant registration information. At the same time, user profile information obtained from third-party websites, such as the user's name, can be pre-populated. The actions of this page after the form is submitted are handled by the controller shown.
6. Implementation of User Registration Controller
@RequestMapping(value="/signup", method=RequestMethod.POST) public String signup(@Valid @ModelAttribute("signup") SignupForm form, BindingResult formBinding, WebRequest request) { if (formBinding.hasErrors()) { return null; } Account account = createAccount(form, formBinding); if (account != null) { SignInUtils.signin(account.getUsername()); ProviderSignInUtils.handlePostSignUp(account.getUsername(), request); return "redirect:/"; } return null; }
After the user submits the registration form, create a corresponding account based on the information filled in by the user, then log in to the newly registered account and jump back to the home page.
Use API
Spring Social 的另外一个重要的作用在于提供了很多社交网络服务的 API 的封装。当用户通过社交网络的账号登录之后,可以通过相应的 API 获取到相关的信息,或执行一些操作。比如获取到用户的好友信息,或是根据用户的要求发布新的消息。一般来说,很多社交网络服务提供商都有自己的 API 来提供给开发人员使用。不过一般只提供 REST API,并没有 Java 封装。Spring Social 的一个目标是为主流的社交网络提供相应的 Java 封装的 API。对于社区已经有良好的 Java 库的社交网络,如 Twitter,Spring Social 会进行整合与封装;而对于暂时没有 Java 实现的,Spring Social 的组件会提供相应的支持。如示例应用中使用的 LinkedIn 的 Java API 是由 Spring Social 开发的。
在使用 LinkedIn 的 Java API 之前,首先要创建相应的 Spring Bean,如所示。
7. 创建 LinkedIn API 对应的 Bean
@Bean@Scope(value="request", proxyMode=ScopedProxyMode.INTERFACES)public LinkedIn linkedin(ConnectionRepository repository) { Connection<LinkedIn> connection = repository.findPrimaryConnection(LinkedIn.class); return connection != null ? connection.getApi() : null; }
LinkedIn Java API 的核心接口是 org.springframework.social.linkedin.api.LinkedIn。如果当前用户已经连接了 LinkedIn 的账号,就可以通过 ConnectionRepository 接口找到该用户对应的连接的信息,并创建出 API 接口的实现。是展示 LinkedIn 上好友信息的控制器的实现。
8. 展示 LinkedIn 上好友信息的控制器的实现
@Controllerpublic class LinkedInConnectionsController { private LinkedIn linkedIn; @Inject public LinkedInConnectionsController(LinkedIn linkedIn) { this.linkedIn = linkedIn; } @RequestMapping(value="/connections", method= RequestMethod.GET) public String connections(Model model) { model.addAttribute("connections", linkedIn.connectionOperations().getConnections()); return "connections"; } }
通过 LinkedIn 接口的 connectionOperations 方法可以获取到 org.springframework.social.linkedin.api.ConnectionOperations 接口的实现,然后就可以再通过 getConnections 方法获取到包含好友信息的 org.springframework.social.linkedin.api.LinkedInProfile 类的对象列表。然后就可以在网页上展示,如所示。
9. 展示 LinkedIn 好友信息的页面
<ul class="media-list"> <c:forEach items="${connections}" var="connection"> <li class="media"> <a href="" class="pull-left"> <img class="lazy-load" data-original="${connection.profilePictureUrl}" alt="" class="media-object"> </a> <div class="media-body"> <h4 class="media-heading">${connection.firstName} ${connection.lastName}</h4> <p><c:out value="${connection.headline}" /></p> </div> </li> </c:forEach> </ul>
结束语
在社交网络日益流行的今天,Web 应用都应该考虑增加对社交网络的支持。这样既方便了用户,让他们省去了重新注册的麻烦,又可以增加用户粘性,同时可以通过社交网络进行推广。虽然很多社交网站都提供了开放 API 来让应用使用其服务,但是集成所需的工作量是不小的。Spring Social 作为 Spring 框架中的一部分,为在 Web 应用中集成社交网络提供了良好支持。本文详细介绍了如何通过 Spring Social 来实现通过社交网络账号进行登录以及使用社交网络提供的 API。
The above is the detailed content of How to use Spring Social to connect to social networks. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


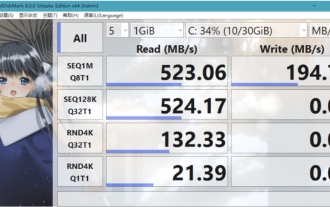
CrystalDiskMark is a small HDD benchmark tool for hard drives that quickly measures sequential and random read/write speeds. Next, let the editor introduce CrystalDiskMark to you and how to use crystaldiskmark~ 1. Introduction to CrystalDiskMark CrystalDiskMark is a widely used disk performance testing tool used to evaluate the read and write speed and performance of mechanical hard drives and solid-state drives (SSD). Random I/O performance. It is a free Windows application and provides a user-friendly interface and various test modes to evaluate different aspects of hard drive performance and is widely used in hardware reviews
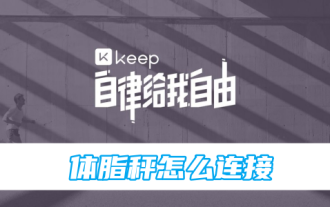
How to connect the keep body fat scale? Keep has a specially designed body fat scale, but most users do not know how to connect the keep body fat scale. Next is the graphic tutorial on the connection method of the keep body fat scale that the editor brings to users. , interested users come and take a look! How to connect the keep body fat scale 1. First open the keep software, go to the main page, click [My] in the lower right corner, and select [Smart Hardware]; 2. Then on the My Smart Devices page, click the [Add Device] button in the middle; 3 , then select the device you want to add interface, select [Smart Body Fat/Weight Scale]; 4. Then on the device model selection page, click the [keep body fat scale] option; 5. Finally, in the interface shown below, finally [Add Now] at the bottom
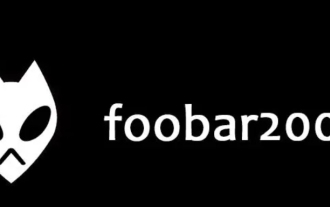
foobar2000 is a software that can listen to music resources at any time. It brings you all kinds of music with lossless sound quality. The enhanced version of the music player allows you to get a more comprehensive and comfortable music experience. Its design concept is to play the advanced audio on the computer The device is transplanted to mobile phones to provide a more convenient and efficient music playback experience. The interface design is simple, clear and easy to use. It adopts a minimalist design style without too many decorations and cumbersome operations to get started quickly. It also supports a variety of skins and Theme, personalize settings according to your own preferences, and create an exclusive music player that supports the playback of multiple audio formats. It also supports the audio gain function to adjust the volume according to your own hearing conditions to avoid hearing damage caused by excessive volume. Next, let me help you
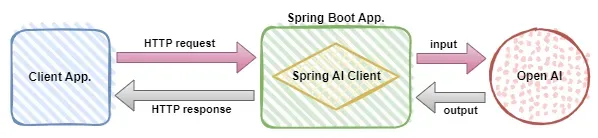
As an industry leader, Spring+AI provides leading solutions for various industries through its powerful, flexible API and advanced functions. In this topic, we will delve into the application examples of Spring+AI in various fields. Each case will show how Spring+AI meets specific needs, achieves goals, and extends these LESSONSLEARNED to a wider range of applications. I hope this topic can inspire you to understand and utilize the infinite possibilities of Spring+AI more deeply. The Spring framework has a history of more than 20 years in the field of software development, and it has been 10 years since the Spring Boot 1.0 version was released. Now, no one can dispute that Spring
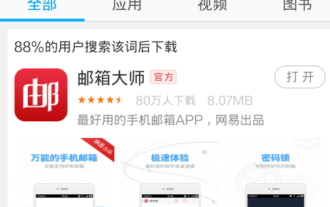
NetEase Mailbox, as an email address widely used by Chinese netizens, has always won the trust of users with its stable and efficient services. NetEase Mailbox Master is an email software specially created for mobile phone users. It greatly simplifies the process of sending and receiving emails and makes our email processing more convenient. So how to use NetEase Mailbox Master, and what specific functions it has. Below, the editor of this site will give you a detailed introduction, hoping to help you! First, you can search and download the NetEase Mailbox Master app in the mobile app store. Search for "NetEase Mailbox Master" in App Store or Baidu Mobile Assistant, and then follow the prompts to install it. After the download and installation is completed, we open the NetEase email account and log in. The login interface is as shown below
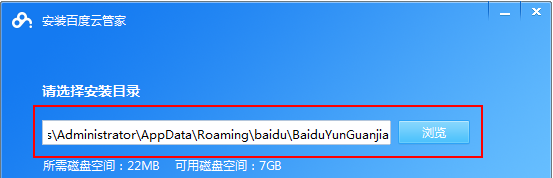
Cloud storage has become an indispensable part of our daily life and work nowadays. As one of the leading cloud storage services in China, Baidu Netdisk has won the favor of a large number of users with its powerful storage functions, efficient transmission speed and convenient operation experience. And whether you want to back up important files, share information, watch videos online, or listen to music, Baidu Cloud Disk can meet your needs. However, many users may not understand the specific use method of Baidu Netdisk app, so this tutorial will introduce in detail how to use Baidu Netdisk app. Users who are still confused can follow this article to learn more. ! How to use Baidu Cloud Network Disk: 1. Installation First, when downloading and installing Baidu Cloud software, please select the custom installation option.
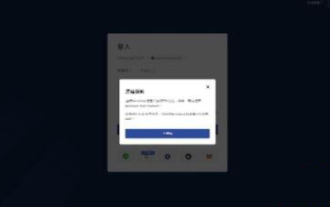
MetaMask (also called Little Fox Wallet in Chinese) is a free and well-received encryption wallet software. Currently, BTCC supports binding to the MetaMask wallet. After binding, you can use the MetaMask wallet to quickly log in, store value, buy coins, etc., and you can also get 20 USDT trial bonus for the first time binding. In the BTCCMetaMask wallet tutorial, we will introduce in detail how to register and use MetaMask, and how to bind and use the Little Fox wallet in BTCC. What is MetaMask wallet? With over 30 million users, MetaMask Little Fox Wallet is one of the most popular cryptocurrency wallets today. It is free to use and can be installed on the network as an extension
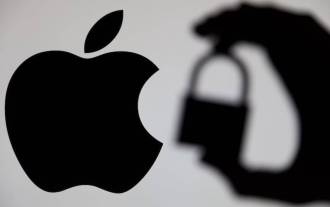
Apple rolled out the iOS 17.4 update on Tuesday, bringing a slew of new features and fixes to iPhones. The update includes new emojis, and EU users will also be able to download them from other app stores. In addition, the update also strengthens the control of iPhone security and introduces more "Stolen Device Protection" setting options to provide users with more choices and protection. "iOS17.3 introduces the "Stolen Device Protection" function for the first time, adding extra security to users' sensitive information. When the user is away from home and other familiar places, this function requires the user to enter biometric information for the first time, and after one hour You must enter information again to access and change certain data, such as changing your Apple ID password or turning off stolen device protection.
