


Introduction and use of basic shell syntax
1. Variables
1. Naming rules for variables: start with a letter or underscore, followed by numbers, letters or underscores. It is best not to name the variable casually, but to be able to guess its meaning when you see the variable name.
2. Variable assignment: x=100
echo $x
Delete variable: unset x
3. Use braces ## to define the boundaries of variable names
#[root@bogon ~]# egon_salary=20000[root@bogon ~]# echo ${egon_salary}yuan
20000yuan
1
[root@bogon ~]# ((x+=1))
[root@bogon ~]# echo $x
11
[root@bogon ~]# x=10
[root@bogon ~]# ((x>=8))
[root@bogon ~]# echo $?
0
4. Calculator in the shell
[root@bogon ~]# echo ${res}%
33%
[root@bogon ~]# [[ 2 > 1 ]][root@bogon ~]# echo $?0 [root@bogon ~]# ((20>10))[root@bogon ~]# echo $?0 [root@bogon ~]# ((20<10))[root@bogon ~]# echo $?1
[root@bogon ~]# [ "abc" = "abc" ][root@bogon ~]# echo $?0 [root@bogon ~]# [[ "abc" = "abc" ]][root@bogon ~]# echo $?0 [root@bogon ~]# (("abc" = "abc"))[root@bogon ~]# echo $?1
[root@bogon ~]# [[ a = a && 1 < 2 ]][root@bogon ~]# echo $?0 [root@bogon ~]# [[ a = a && 1 < 2 ]][root@bogon ~]# echo $?0
[root@bogon ~]# (( a = a || 1 > 2 ))[root@bogon ~]# echo $?1[root@bogon ~]# [[ a = a || 1 > 2 ]][root@bogon ~]# echo $?0
3. Process control
1.if branch
1) Verify user account password:
input your name : zhangcan input password : 123login successful [root@bogon ~]# ./usertest.sh input your name : hha input password : hag user or password error
#! /bin/bashuser='zhangcan'password='123'read -p 'input your name : ' name read -p 'input password : ' codeif [ $name = $user -a $code = $password ];then echo 'login successful'elseecho 'user or password error'fi~
#!/bin/bash #根据用户输入的成绩,判断所属档次,并输出给用户read -p 'input your score : ' scoreif [ $score -ge 90 ];then echo '优秀'elif [ $score -ge 70 -a $score -lt 90 ];then echo '良好'elif [ $score -ge 60 -a $score -lt 70 ];then echo '及格'elif [ $score -lt 60 ];then echo '较差'fi
#!/bin/bashwhile : do read -p 'input your file : ' fileif [ -z $file ];thencontinueelsebreakfi doneif [ -f $file ];then echo "$file is regular file"elif [ -b $file ];then echo "$file is block file"elif [ -d $file ];then echo "$file is directory file"elseecho "$file type unkonw"fi
echo $i doneExample 1: Write a script to test the IPs that can be used in the subnet
#!/bin/bashfor i in {1..50} do ping -c1 192.168.16.$i &> /dev/null # -c1表示ping一次if [ $? -ne 0 ];then echo "192.168.16.$i successful"echo "192.168.16.$i" >> ~/ipavailable.txt fi done~
#!/bin/bashdir='/dev'for i in $(ls $dir) doif [ -h $dir/$i ];then ((link+=1))elif [ -f $dir/$i ];then (( rfile+=1))elif [ -d $dir/$i ];then ((directory+=1))elif [ -b $dir/$i ];then (( block+=1 ))else(( typeunknow+=1)) fi done echo 'block' $block echo 'regular file' $rfile echo 'directory' $directory echo 'link' $link echo 'unknow' $typeunknow
#!/bin/bashfor ((i=1;i<=9;i++)) dofor ((j=1;j<=i;j++)) do echo -n "$i*$j=$[$i*$j]"done echo done
#!/bin/bashuser='zhangcan'password='123'tag=truewhile $tag do read -p 'input your name : ' name read -p 'input your password : ' codeif [[ $name = $user ]] && [[ $code = $password ]];then echo 'login successful'while $tag do read -p '>>: ' cmdif [[ $cmd = 'quit' ]];then tag=falseelse$cmd fi done fi done
The above is the detailed content of Introduction and use of basic shell syntax. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


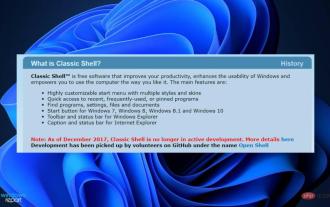
<p>Customizing your operating system is a great way to make your daily life more enjoyable. You can change the user interface, apply custom themes, add widgets, and more. So today we will show you how to install ClassicShell on Windows 11. </p><p>This program has been around for a long time and allows you to modify the operating system. Volunteers have now started running the organization, which disbanded in 2017. The new project is called OpenShell and is currently available on Github for those interested. </p>&a
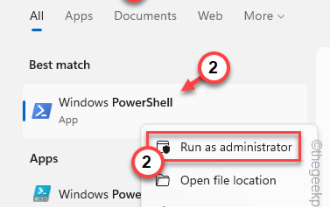
Do you see this error message "Add-AppxPackage: Deployment failed with HRESULT: 0x80073D02, The package cannot be installed because the resource it modifies is currently in use. Error 0x80073D02..." in PowerShell when you run the script? As the error message states, this does occur when the user attempts to re-register one or all WindowsShellExperienceHost applications while the previous process is running. We've got some simple solutions to fix this problem quickly. Fix 1 – Terminate the experience host process You must terminate before executing the powershell command
![Explorer.exe does not start on system startup [Fix]](https://img.php.cn/upload/article/000/887/227/168575230155539.png?x-oss-process=image/resize,m_fill,h_207,w_330)
Nowadays, many Windows users start encountering severe Windows system problems. The problem is that Explorer.exe cannot start after the system is loaded, and users cannot open files or folders. Although, Windows users can open Windows Explorer manually using Command Prompt in some cases and this must be done every time the system restarts or after system startup. This can be problematic and is due to the following factors mentioned below. Corrupted system files. Enable fast startup settings. Outdated or problematic display drivers. Changes were made to some services in the system. Modified registry file. Keeping all the above factors in mind, we have come up with some that will surely help the users
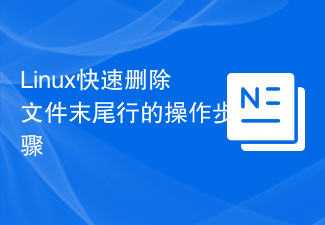
When processing files under Linux systems, it is sometimes necessary to delete lines at the end of the file. This operation is very common in practical applications and can be achieved through some simple commands. This article will introduce the steps to quickly delete the line at the end of the file in Linux system, and provide specific code examples. Step 1: Check the last line of the file. Before performing the deletion operation, you first need to confirm which line is the last line of the file. You can use the tail command to view the last line of the file. The specific command is as follows: tail-n1filena
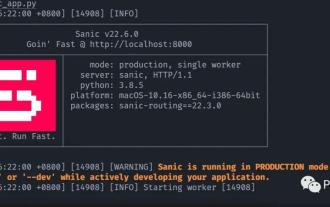
When it comes to API development, you may think of DjangoRESTFramework, Flask, and FastAPI. Yes, they can be used to write APIs. However, the framework shared today allows you to convert existing functions into APIs faster. It is Sanic . Introduction to Sanic Sanic[1] is a Python3.7+ web server and web framework designed to improve performance. It allows the use of the async/await syntax added in Python 3.5, which can effectively avoid blocking and improve response speed. Sanic is committed to providing a simple and fast way to create and launch

Open shell not running on Windows 11 is not a new problem and has been plaguing users since the advent of this new operating system. The cause of the Open-Shell Windows 11 not working issue is not specific. It can be caused by unexpected errors in programs, the presence of viruses or malware, or corrupted system files. For those who don’t know, Open-Shell is the replacement for Classic Shell, which was discontinued in 2017. You can check out our tutorial on how to install Classic Shell on Windows 11. How to replace Windows 11 Start menu

Windows Subsystem for Linux The first option is to use Windows Subsystem for Linux or WSL, which is a compatibility layer for running Linux binary executables natively on Windows systems. It works for most scenarios and allows you to run shell scripts in Windows 11/10. WSL is not automatically available, so you must enable it through your Windows device's developer settings. You can do this by going to Settings > Update & Security > For Developers. Switch to developer mode and confirm the prompt by selecting Yes. Next, look for W
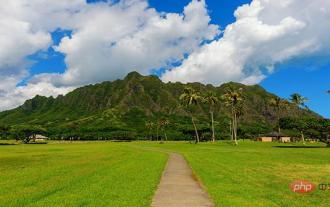
Some examples of Python scripts: enterprise WeChat alarms, FTP clients, SSH clients, Saltstack clients, vCenter clients, obtaining domain name SSL certificate expiration time, sending today's weather forecast and future weather trend charts; some examples of Shell scripts: SVN Full backup, Zabbix monitoring user password expiration, building local YUM, and the readers' needs in the previous article (when the load is high, find out the process scripts with high occupancy and store or push notifications); it is a bit long, so please read it patiently At the end of the article, there is an Easter egg after all. Python script part of enterprise WeChat alarm This script uses enterprise WeChat application to perform WeChat alarm and can be used
